Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / Configuration / ConfigUtil.cs / 1 / ConfigUtil.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Threading; using System.Configuration; using System.Xml; using System.Web.Compilation; using System.Web.Util; internal class ConfigUtil { private ConfigUtil() { } internal static void CheckBaseType(Type expectedBaseType, Type userBaseType, string propertyName, ConfigurationElement configElement) { // Make sure the base type is valid if (!expectedBaseType.IsAssignableFrom(userBaseType)) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_type_to_inherit_from, userBaseType.FullName, expectedBaseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, XmlNode node, bool checkAptcaBit, bool ignoreCase) { // We should get either a propertyName/configElement or node, but not both. // They are used only for error reporting. Debug.Assert((propertyName != null) != (node != null)); Type val; try { val = BuildManager.GetType(typeName, true /*throwOnError*/, ignoreCase); } catch (Exception e) { if (e is ThreadAbortException || e is StackOverflowException || e is OutOfMemoryException) { throw; } if (node != null) { throw new ConfigurationErrorsException(e.Message, e, node); } else { if (configElement != null) { throw new ConfigurationErrorsException(e.Message, e, configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } else { throw new ConfigurationErrorsException(e.Message, e); } } } // If we're not in full trust, only allow types that have the APTCA bit (ASURT 139687), // unless the checkAptcaBit flag is false if (checkAptcaBit) { if (node != null) { HttpRuntime.FailIfNoAPTCABit(val, node); } else { HttpRuntime.FailIfNoAPTCABit(val, configElement != null ? configElement.ElementInformation : null, propertyName); } } return val; } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement) { return GetType(typeName, propertyName, configElement, true /*checkAptcaBit*/); } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, bool checkAptcaBit) { return GetType(typeName, propertyName, configElement, checkAptcaBit, false); } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, bool checkAptcaBit, bool ignoreCase) { return GetType(typeName, propertyName, configElement, null /*node*/, checkAptcaBit, ignoreCase); } internal static Type GetType(string typeName, XmlNode node) { return GetType(typeName, node, false /*ignoreCase*/); } internal static Type GetType(string typeName, XmlNode node, bool ignoreCase) { return GetType(typeName, null, null, node, true /*checkAptcaBit*/, ignoreCase); } internal static void CheckAssignableType(Type baseType, Type type, ConfigurationElement configElement, string propertyName) { if (!baseType.IsAssignableFrom(type)) { throw new ConfigurationErrorsException( SR.GetString(SR.Type_doesnt_inherit_from_type, type.FullName, baseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static void CheckAssignableType(Type baseType, Type baseType2, Type type, ConfigurationElement configElement, string propertyName) { if (!baseType.IsAssignableFrom(type) && !baseType2.IsAssignableFrom(type)) { throw new ConfigurationErrorsException( SR.GetString(SR.Type_doesnt_inherit_from_type, type.FullName, baseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static bool IsTypeHandlerOrFactory(Type t) { return typeof(IHttpHandler).IsAssignableFrom(t) || typeof(IHttpHandlerFactory).IsAssignableFrom(t); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Threading; using System.Configuration; using System.Xml; using System.Web.Compilation; using System.Web.Util; internal class ConfigUtil { private ConfigUtil() { } internal static void CheckBaseType(Type expectedBaseType, Type userBaseType, string propertyName, ConfigurationElement configElement) { // Make sure the base type is valid if (!expectedBaseType.IsAssignableFrom(userBaseType)) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_type_to_inherit_from, userBaseType.FullName, expectedBaseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, XmlNode node, bool checkAptcaBit, bool ignoreCase) { // We should get either a propertyName/configElement or node, but not both. // They are used only for error reporting. Debug.Assert((propertyName != null) != (node != null)); Type val; try { val = BuildManager.GetType(typeName, true /*throwOnError*/, ignoreCase); } catch (Exception e) { if (e is ThreadAbortException || e is StackOverflowException || e is OutOfMemoryException) { throw; } if (node != null) { throw new ConfigurationErrorsException(e.Message, e, node); } else { if (configElement != null) { throw new ConfigurationErrorsException(e.Message, e, configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } else { throw new ConfigurationErrorsException(e.Message, e); } } } // If we're not in full trust, only allow types that have the APTCA bit (ASURT 139687), // unless the checkAptcaBit flag is false if (checkAptcaBit) { if (node != null) { HttpRuntime.FailIfNoAPTCABit(val, node); } else { HttpRuntime.FailIfNoAPTCABit(val, configElement != null ? configElement.ElementInformation : null, propertyName); } } return val; } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement) { return GetType(typeName, propertyName, configElement, true /*checkAptcaBit*/); } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, bool checkAptcaBit) { return GetType(typeName, propertyName, configElement, checkAptcaBit, false); } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, bool checkAptcaBit, bool ignoreCase) { return GetType(typeName, propertyName, configElement, null /*node*/, checkAptcaBit, ignoreCase); } internal static Type GetType(string typeName, XmlNode node) { return GetType(typeName, node, false /*ignoreCase*/); } internal static Type GetType(string typeName, XmlNode node, bool ignoreCase) { return GetType(typeName, null, null, node, true /*checkAptcaBit*/, ignoreCase); } internal static void CheckAssignableType(Type baseType, Type type, ConfigurationElement configElement, string propertyName) { if (!baseType.IsAssignableFrom(type)) { throw new ConfigurationErrorsException( SR.GetString(SR.Type_doesnt_inherit_from_type, type.FullName, baseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static void CheckAssignableType(Type baseType, Type baseType2, Type type, ConfigurationElement configElement, string propertyName) { if (!baseType.IsAssignableFrom(type) && !baseType2.IsAssignableFrom(type)) { throw new ConfigurationErrorsException( SR.GetString(SR.Type_doesnt_inherit_from_type, type.FullName, baseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static bool IsTypeHandlerOrFactory(Type t) { return typeof(IHttpHandler).IsAssignableFrom(t) || typeof(IHttpHandlerFactory).IsAssignableFrom(t); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
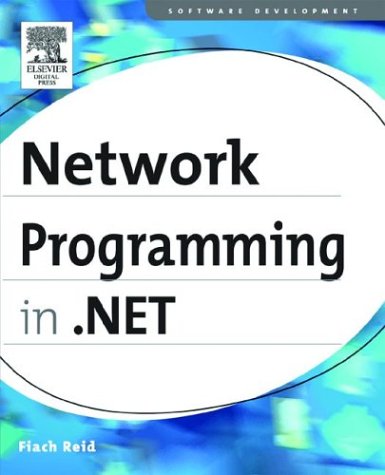
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- COM2TypeInfoProcessor.cs
- AffineTransform3D.cs
- StructureChangedEventArgs.cs
- _emptywebproxy.cs
- LayoutEditorPart.cs
- SqlBuffer.cs
- AttachedPropertyBrowsableAttribute.cs
- XmlReaderSettings.cs
- StringConcat.cs
- FormViewInsertEventArgs.cs
- DefaultObjectSerializer.cs
- ByteKeyFrameCollection.cs
- ChangeNode.cs
- MonthCalendarDesigner.cs
- XmlAggregates.cs
- WorkflowInstance.cs
- TaskFileService.cs
- Tracking.cs
- CodeAccessSecurityEngine.cs
- ListCommandEventArgs.cs
- Stylesheet.cs
- ReadWriteObjectLock.cs
- invalidudtexception.cs
- DoubleCollectionConverter.cs
- CharacterShapingProperties.cs
- DataBindingCollectionEditor.cs
- _LazyAsyncResult.cs
- XmlDeclaration.cs
- Boolean.cs
- KeyToListMap.cs
- FullTrustAssembliesSection.cs
- FreezableCollection.cs
- TdsValueSetter.cs
- XmlAttributeCollection.cs
- TextAutomationPeer.cs
- SqlProfileProvider.cs
- QuaternionAnimation.cs
- OdbcConnectionStringbuilder.cs
- UnmanagedMemoryStreamWrapper.cs
- SecurityValidationBehavior.cs
- ServiceReference.cs
- UIElementIsland.cs
- NumericExpr.cs
- UpdateCompiler.cs
- linebase.cs
- TouchPoint.cs
- DropDownHolder.cs
- SynchronizedDisposablePool.cs
- ContextMenuAutomationPeer.cs
- DecodeHelper.cs
- Int32CollectionConverter.cs
- TitleStyle.cs
- SortedDictionary.cs
- ClientSettingsProvider.cs
- ping.cs
- ListViewInsertedEventArgs.cs
- Propagator.Evaluator.cs
- WindowsProgressbar.cs
- SerializationFieldInfo.cs
- _SafeNetHandles.cs
- SystemIPGlobalProperties.cs
- Roles.cs
- TreeBuilder.cs
- UIHelper.cs
- DocumentPageTextView.cs
- HttpHandlerAction.cs
- SystemException.cs
- LineProperties.cs
- SqlDataSourceStatusEventArgs.cs
- ZoomComboBox.cs
- CodeAccessPermission.cs
- MD5CryptoServiceProvider.cs
- _emptywebproxy.cs
- XamlNamespaceHelper.cs
- PriorityRange.cs
- SecurityRuntime.cs
- UnmanagedMemoryStream.cs
- ProfilePropertyNameValidator.cs
- SoapSchemaExporter.cs
- TextSelectionProcessor.cs
- GrammarBuilderPhrase.cs
- GACMembershipCondition.cs
- HelloMessage11.cs
- SchemaDeclBase.cs
- XhtmlConformanceSection.cs
- TreeView.cs
- CodeTypeMember.cs
- BinaryNegotiation.cs
- ColorBlend.cs
- Cursors.cs
- FontDialog.cs
- DbModificationClause.cs
- DeploymentSectionCache.cs
- ControlPropertyNameConverter.cs
- XmlSchemaAll.cs
- XmlName.cs
- ToolStripAdornerWindowService.cs
- RelationshipEnd.cs
- XmlElementAttributes.cs
- Exception.cs