Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / MS / Internal / Shaping / CharacterShapingProperties.cs / 1 / CharacterShapingProperties.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: CharacterShapingProperties class // // History: // 10/26/2004: Garyyang Created the file // 1/25/2004: garyyang Move it to internal namespace // //--------------------------------------------------------------------------- using System.Runtime.InteropServices; namespace MS.Internal.Shaping { ////// Properties per character /// [StructLayout(LayoutKind.Sequential)] internal struct CharacterShapingProperties { ////// Constructor /// /// reserved bits used by shaping engine /// flag indicating whether the code point can be glyphed alone internal CharacterShapingProperties(byte engineReserved, bool canGlyphAlone) { _value = (canGlyphAlone ? CanGlyphAloneFlag : (ushort) 0); _value |= engineReserved; } ////// Get or Set CanGlyphAlone flag for the charactrer /// ///internal bool CanGlyphAlone { get { return (_value & CanGlyphAloneFlag) != 0; } set { if (value) { _value |= CanGlyphAloneFlag; } else { _value &= (CanGlyphAloneFlag ^ 0xFFFF); } } } /// /// Get or Set bits reserved for shaping engine use /// ///internal byte EngineReserved { get { return (byte)(_value & EngineReservedValueMask); } set { _value = (ushort)((_value & (EngineReservedValueMask ^ 0xFFFF)) | value); } } /// /// Compares two CharacterShapingProperties for equality. /// ///Returns true if the arguments have identical properties, false if not. public static bool operator ==( CharacterShapingProperties left, CharacterShapingProperties right) { return left._value == right._value; } ////// Compares two CharacterShapingProperties for inequality. /// ///Returns true if the arguments are not equal, false if they are equal. public static bool operator !=( CharacterShapingProperties left, CharacterShapingProperties right) { return !(left == right); } ////// Compares the specified object with this. /// ///Returns true if the specified object is a CharacterShapingProperties with the same /// value as this object. public override bool Equals(object o) { if (o != null && o is CharacterShapingProperties) { return (CharacterShapingProperties)o == this; } else { return false; } } ////// Returns a hash code based on the property flags. /// public override int GetHashCode() { return _value; } private ushort _value; // CanGlyphAloneFlag is store at the 9th bit private const ushort CanGlyphAloneFlag = 0x0100; private const ushort EngineReservedValueMask = 0x00FF; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: CharacterShapingProperties class // // History: // 10/26/2004: Garyyang Created the file // 1/25/2004: garyyang Move it to internal namespace // //--------------------------------------------------------------------------- using System.Runtime.InteropServices; namespace MS.Internal.Shaping { ////// Properties per character /// [StructLayout(LayoutKind.Sequential)] internal struct CharacterShapingProperties { ////// Constructor /// /// reserved bits used by shaping engine /// flag indicating whether the code point can be glyphed alone internal CharacterShapingProperties(byte engineReserved, bool canGlyphAlone) { _value = (canGlyphAlone ? CanGlyphAloneFlag : (ushort) 0); _value |= engineReserved; } ////// Get or Set CanGlyphAlone flag for the charactrer /// ///internal bool CanGlyphAlone { get { return (_value & CanGlyphAloneFlag) != 0; } set { if (value) { _value |= CanGlyphAloneFlag; } else { _value &= (CanGlyphAloneFlag ^ 0xFFFF); } } } /// /// Get or Set bits reserved for shaping engine use /// ///internal byte EngineReserved { get { return (byte)(_value & EngineReservedValueMask); } set { _value = (ushort)((_value & (EngineReservedValueMask ^ 0xFFFF)) | value); } } /// /// Compares two CharacterShapingProperties for equality. /// ///Returns true if the arguments have identical properties, false if not. public static bool operator ==( CharacterShapingProperties left, CharacterShapingProperties right) { return left._value == right._value; } ////// Compares two CharacterShapingProperties for inequality. /// ///Returns true if the arguments are not equal, false if they are equal. public static bool operator !=( CharacterShapingProperties left, CharacterShapingProperties right) { return !(left == right); } ////// Compares the specified object with this. /// ///Returns true if the specified object is a CharacterShapingProperties with the same /// value as this object. public override bool Equals(object o) { if (o != null && o is CharacterShapingProperties) { return (CharacterShapingProperties)o == this; } else { return false; } } ////// Returns a hash code based on the property flags. /// public override int GetHashCode() { return _value; } private ushort _value; // CanGlyphAloneFlag is store at the 9th bit private const ushort CanGlyphAloneFlag = 0x0100; private const ushort EngineReservedValueMask = 0x00FF; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
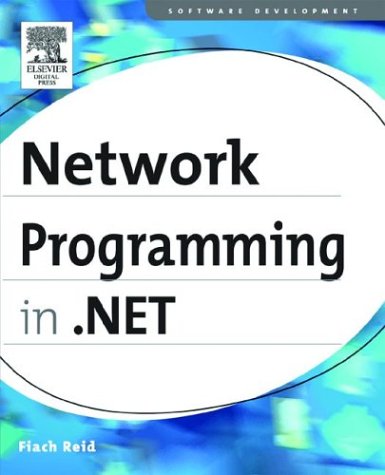
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LayoutManager.cs
- EventSetter.cs
- RegexStringValidator.cs
- DataGridRelationshipRow.cs
- DataObjectAttribute.cs
- ToolStripSystemRenderer.cs
- CheckBoxAutomationPeer.cs
- WindowsSysHeader.cs
- SByteConverter.cs
- CombinedTcpChannel.cs
- ParallelTimeline.cs
- UrlParameterReader.cs
- shaperfactory.cs
- HttpBrowserCapabilitiesBase.cs
- TreeViewBindingsEditor.cs
- PathTooLongException.cs
- SendingRequestEventArgs.cs
- WebBrowserDocumentCompletedEventHandler.cs
- DSASignatureFormatter.cs
- CultureSpecificStringDictionary.cs
- ErrorsHelper.cs
- VBIdentifierNameEditor.cs
- GlyphShapingProperties.cs
- QueryAccessibilityHelpEvent.cs
- Compiler.cs
- GeneralTransform.cs
- CodeTypeMember.cs
- TrackingServices.cs
- JournalNavigationScope.cs
- ControlsConfig.cs
- TaiwanLunisolarCalendar.cs
- ComponentSerializationService.cs
- XmlSchemaCollection.cs
- DesignerView.xaml.cs
- FixedPageProcessor.cs
- PanelDesigner.cs
- GenericQueueSurrogate.cs
- InputScopeConverter.cs
- IndentTextWriter.cs
- MultipartContentParser.cs
- HashCodeCombiner.cs
- ExtractorMetadata.cs
- ControlType.cs
- SourceFilter.cs
- SharingService.cs
- DurableMessageDispatchInspector.cs
- DescendentsWalkerBase.cs
- SqlConnectionFactory.cs
- EventProviderWriter.cs
- TransportElement.cs
- DynamicMethod.cs
- XmlParserContext.cs
- AssertUtility.cs
- QueueProcessor.cs
- Preprocessor.cs
- XPathNodeHelper.cs
- MD5CryptoServiceProvider.cs
- util.cs
- LocalizabilityAttribute.cs
- PropertyEmitterBase.cs
- BrowserCapabilitiesFactoryBase.cs
- _NestedSingleAsyncResult.cs
- CompatibleComparer.cs
- GZipStream.cs
- WpfPayload.cs
- NameValuePair.cs
- Exception.cs
- TextPenaltyModule.cs
- Calendar.cs
- XamlClipboardData.cs
- HttpModuleAction.cs
- FieldInfo.cs
- XmlAnyElementAttributes.cs
- ViewCellSlot.cs
- IxmlLineInfo.cs
- LicenseProviderAttribute.cs
- UpWmlPageAdapter.cs
- PlaceHolder.cs
- EdmComplexTypeAttribute.cs
- Accessible.cs
- Int32EqualityComparer.cs
- CodeDirectoryCompiler.cs
- DataContractJsonSerializerOperationBehavior.cs
- XmlSignatureProperties.cs
- Enumerable.cs
- DataComponentGenerator.cs
- Crc32Helper.cs
- CodeDesigner.cs
- DataGridViewTopRowAccessibleObject.cs
- InputProcessorProfilesLoader.cs
- UIntPtr.cs
- WindowsGraphics.cs
- fixedPageContentExtractor.cs
- InternalsVisibleToAttribute.cs
- TableHeaderCell.cs
- SqlMethodAttribute.cs
- TextEndOfParagraph.cs
- _NegoStream.cs
- EncryptedHeader.cs
- DelegatingConfigHost.cs