Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Configuration / System / Configuration / ErrorsHelper.cs / 1 / ErrorsHelper.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Specialized; using System.Collections.Generic; static internal class ErrorsHelper { static internal int GetErrorCount(Listerrors) { return (errors != null) ? errors.Count : 0; } static internal bool GetHasErrors(List errors) { return GetErrorCount(errors) > 0; } static internal void AddError(ref List errors, ConfigurationException e) { Debug.Assert(e != null, "e != null"); // Create on demand if (errors == null) { errors = new List (); } ConfigurationErrorsException ce = e as ConfigurationErrorsException; if (ce == null) { errors.Add(e); } else { ICollection col = ce.ErrorsGeneric; if (col.Count == 1) { errors.Add(e); } else { errors.AddRange(col); } } } static internal void AddErrors(ref List errors, ICollection coll) { if (coll == null || coll.Count == 0) { // Nothing to do here, bail return; } foreach (ConfigurationException e in coll) { AddError(ref errors, e); } } static internal ConfigurationErrorsException GetErrorsException(List errors) { if (errors == null) { return null; } Debug.Assert(errors.Count != 0, "errors.Count != 0"); return new ConfigurationErrorsException(errors); } static internal void ThrowOnErrors(List errors) { ConfigurationErrorsException e = GetErrorsException(errors); if (e != null) { throw e; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Specialized; using System.Collections.Generic; static internal class ErrorsHelper { static internal int GetErrorCount(Listerrors) { return (errors != null) ? errors.Count : 0; } static internal bool GetHasErrors(List errors) { return GetErrorCount(errors) > 0; } static internal void AddError(ref List errors, ConfigurationException e) { Debug.Assert(e != null, "e != null"); // Create on demand if (errors == null) { errors = new List (); } ConfigurationErrorsException ce = e as ConfigurationErrorsException; if (ce == null) { errors.Add(e); } else { ICollection col = ce.ErrorsGeneric; if (col.Count == 1) { errors.Add(e); } else { errors.AddRange(col); } } } static internal void AddErrors(ref List errors, ICollection coll) { if (coll == null || coll.Count == 0) { // Nothing to do here, bail return; } foreach (ConfigurationException e in coll) { AddError(ref errors, e); } } static internal ConfigurationErrorsException GetErrorsException(List errors) { if (errors == null) { return null; } Debug.Assert(errors.Count != 0, "errors.Count != 0"); return new ConfigurationErrorsException(errors); } static internal void ThrowOnErrors(List errors) { ConfigurationErrorsException e = GetErrorsException(errors); if (e != null) { throw e; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
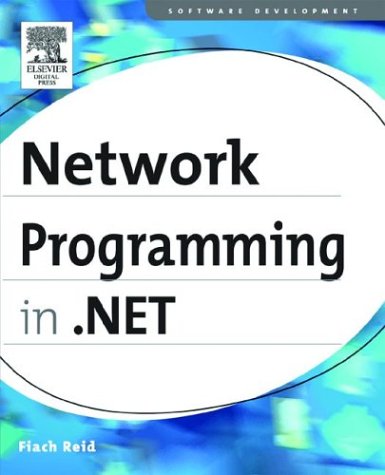
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CmsInterop.cs
- TabControlToolboxItem.cs
- DockAndAnchorLayout.cs
- TextHidden.cs
- PlatformCulture.cs
- IdentityManager.cs
- PriorityChain.cs
- HwndSubclass.cs
- SerializableReadOnlyDictionary.cs
- FixedSOMLineRanges.cs
- hresults.cs
- MouseDevice.cs
- IRCollection.cs
- ObjectToIdCache.cs
- BinHexDecoder.cs
- ElementMarkupObject.cs
- OleAutBinder.cs
- ContextActivityUtils.cs
- ExpressionBuilderCollection.cs
- ApplicationProxyInternal.cs
- FlowDocumentPaginator.cs
- DataSourceXmlTextReader.cs
- XmlSchemaValidationException.cs
- QuadraticBezierSegment.cs
- OperandQuery.cs
- LineSegment.cs
- BitmapEffectDrawingContextWalker.cs
- CorrelationManager.cs
- ErrorFormatter.cs
- SqlPersonalizationProvider.cs
- ExtendedProtectionPolicyElement.cs
- PerformanceCountersElement.cs
- DeclaredTypeValidatorAttribute.cs
- Fonts.cs
- XmlNamedNodeMap.cs
- SqlClientWrapperSmiStream.cs
- SqlDataSourceView.cs
- Hyperlink.cs
- StateWorkerRequest.cs
- DispatchChannelSink.cs
- DelegateTypeInfo.cs
- BevelBitmapEffect.cs
- AutomationFocusChangedEventArgs.cs
- ServiceModelPerformanceCounters.cs
- SafeBitVector32.cs
- TreeNodeClickEventArgs.cs
- EditorPart.cs
- EventDescriptor.cs
- DetailsViewPagerRow.cs
- FilteredReadOnlyMetadataCollection.cs
- EnumerableRowCollection.cs
- MenuItem.cs
- ObjectTag.cs
- ObjectStateFormatter.cs
- webbrowsersite.cs
- StringFunctions.cs
- XPathNodeIterator.cs
- CallContext.cs
- ButtonChrome.cs
- mda.cs
- PrimitiveXmlSerializers.cs
- BitVec.cs
- SurrogateSelector.cs
- StringValidatorAttribute.cs
- ContextStaticAttribute.cs
- AdjustableArrowCap.cs
- TabItemWrapperAutomationPeer.cs
- DialogResultConverter.cs
- EntityModelBuildProvider.cs
- ACE.cs
- SqlUserDefinedAggregateAttribute.cs
- ListViewInsertedEventArgs.cs
- CodeNamespaceCollection.cs
- SettingsPropertyValueCollection.cs
- XPathSelfQuery.cs
- TypeDescriptionProviderAttribute.cs
- InvalidPrinterException.cs
- SubMenuStyleCollectionEditor.cs
- PointAnimation.cs
- VisualStateManager.cs
- NavigationWindow.cs
- SchemaSetCompiler.cs
- OdbcParameter.cs
- ConfigXmlSignificantWhitespace.cs
- PlatformCulture.cs
- XmlSchemaAnyAttribute.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- FlowDecision.cs
- HtmlElementErrorEventArgs.cs
- ConvertEvent.cs
- Deflater.cs
- FormClosingEvent.cs
- ButtonFieldBase.cs
- ProtocolsConfigurationHandler.cs
- LineSegment.cs
- HitTestParameters.cs
- UpdateCompiler.cs
- MDIClient.cs
- ZipIOExtraFieldElement.cs
- DocumentEventArgs.cs