Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Query / ResultAssembly / BitVec.cs / 2 / BitVec.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- namespace System.Data.Query.ResultAssembly { using System.Diagnostics; using System.Text; ////// BitVector helper class; used to keep track of the used columns /// in the result assembly. /// ////// BitVec can be a struct because it contains a readonly reference to an int[]. /// This code is a copy of System.Collections.BitArray so that we can have an efficient implementation of Minus. /// internal struct BitVec { private readonly int[] m_array; private readonly int m_length; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal BitVec(int length) { Debug.Assert(0 < length, "zero length"); m_array = new int[(length + 31) / 32]; m_length = length; } internal int Count { get { return m_length; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Set(int index) { Debug.Assert(unchecked((uint)index < (uint)m_length), "index out of range"); m_array[index / 32] |= (1 << (index % 32)); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void ClearAll() { for (int i = 0; i < m_array.Length; i++) { m_array[i] = 0; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal bool IsEmpty() { for (int i = 0; i < m_array.Length; i++) { if (0 != m_array[i]) { return false; } } return true; } internal bool IsSet(int index) { Debug.Assert(unchecked((uint)index < (uint)m_length), "index out of range"); return (m_array[index / 32] & (1 << (index % 32))) != 0; } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Or(BitVec value) { Debug.Assert(m_length == value.m_length, "unequal sized bitvec"); for (int i = 0; i < m_array.Length; i++) { m_array[i] |= value.m_array[i]; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Minus(BitVec value) { Debug.Assert(m_length == value.m_length, "unequal sized bitvec"); for (int i = 0; i < m_array.Length; i++) { m_array[i] &= ~value.m_array[i]; } } public override string ToString() { StringBuilder sb = new StringBuilder(3 * Count); string separator = string.Empty; for (int i = 0; i < Count; i++) { if (IsSet(i)) { sb.Append(separator); sb.Append(i); separator = ","; } } return sb.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- namespace System.Data.Query.ResultAssembly { using System.Diagnostics; using System.Text; ////// BitVector helper class; used to keep track of the used columns /// in the result assembly. /// ////// BitVec can be a struct because it contains a readonly reference to an int[]. /// This code is a copy of System.Collections.BitArray so that we can have an efficient implementation of Minus. /// internal struct BitVec { private readonly int[] m_array; private readonly int m_length; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal BitVec(int length) { Debug.Assert(0 < length, "zero length"); m_array = new int[(length + 31) / 32]; m_length = length; } internal int Count { get { return m_length; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Set(int index) { Debug.Assert(unchecked((uint)index < (uint)m_length), "index out of range"); m_array[index / 32] |= (1 << (index % 32)); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void ClearAll() { for (int i = 0; i < m_array.Length; i++) { m_array[i] = 0; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal bool IsEmpty() { for (int i = 0; i < m_array.Length; i++) { if (0 != m_array[i]) { return false; } } return true; } internal bool IsSet(int index) { Debug.Assert(unchecked((uint)index < (uint)m_length), "index out of range"); return (m_array[index / 32] & (1 << (index % 32))) != 0; } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Or(BitVec value) { Debug.Assert(m_length == value.m_length, "unequal sized bitvec"); for (int i = 0; i < m_array.Length; i++) { m_array[i] |= value.m_array[i]; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Minus(BitVec value) { Debug.Assert(m_length == value.m_length, "unequal sized bitvec"); for (int i = 0; i < m_array.Length; i++) { m_array[i] &= ~value.m_array[i]; } } public override string ToString() { StringBuilder sb = new StringBuilder(3 * Count); string separator = string.Empty; for (int i = 0; i < Count; i++) { if (IsSet(i)) { sb.Append(separator); sb.Append(i); separator = ","; } } return sb.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
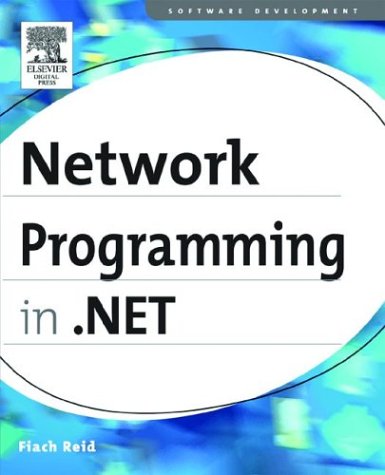
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TraceSource.cs
- MethodImplAttribute.cs
- InternalBase.cs
- ObjectDataSourceView.cs
- _NestedSingleAsyncResult.cs
- TextInfo.cs
- WebBrowserNavigatedEventHandler.cs
- FixedNode.cs
- GetPageNumberCompletedEventArgs.cs
- PatternMatcher.cs
- ReadOnlyCollection.cs
- CapabilitiesUse.cs
- Stackframe.cs
- ISAPIWorkerRequest.cs
- CalendarDateChangedEventArgs.cs
- XmlDownloadManager.cs
- XsltInput.cs
- IndexingContentUnit.cs
- HttpHandlerActionCollection.cs
- ExceptionUtil.cs
- Missing.cs
- DesignerSerializerAttribute.cs
- StartUpEventArgs.cs
- RequestSecurityTokenForRemoteTokenFactory.cs
- CharStorage.cs
- WindowsEditBoxRange.cs
- KeySplineConverter.cs
- SwitchElementsCollection.cs
- WebPartTransformer.cs
- XmlSchemaDatatype.cs
- counter.cs
- EntityParameterCollection.cs
- PersonalizableTypeEntry.cs
- SettingsPropertyIsReadOnlyException.cs
- Model3D.cs
- DataPager.cs
- SimpleBitVector32.cs
- TabletDeviceInfo.cs
- ResumeStoryboard.cs
- MatrixTransform.cs
- ProviderSettings.cs
- ReferentialConstraint.cs
- TextTreeRootNode.cs
- ClassGenerator.cs
- HttpDebugHandler.cs
- CryptoKeySecurity.cs
- Error.cs
- CommandConverter.cs
- AspNetHostingPermission.cs
- XmlComplianceUtil.cs
- AssemblyContextControlItem.cs
- AutomationPropertyInfo.cs
- AttachmentCollection.cs
- WorkflowInstanceExtensionCollection.cs
- InheritedPropertyChangedEventArgs.cs
- PathGeometry.cs
- AnnotationComponentChooser.cs
- FormViewUpdatedEventArgs.cs
- Stacktrace.cs
- RootBuilder.cs
- ListBoxItemWrapperAutomationPeer.cs
- WpfWebRequestHelper.cs
- RtfFormatStack.cs
- RootBuilder.cs
- DataGridViewCellParsingEventArgs.cs
- InkCollectionBehavior.cs
- DesignTimeVisibleAttribute.cs
- UserValidatedEventArgs.cs
- CookieProtection.cs
- LabelEditEvent.cs
- GridView.cs
- EntityDescriptor.cs
- ADMembershipUser.cs
- ReferencedCollectionType.cs
- ADMembershipProvider.cs
- WindowVisualStateTracker.cs
- SecureConversationServiceCredential.cs
- CodeIdentifiers.cs
- Comparer.cs
- SqlMultiplexer.cs
- BlurBitmapEffect.cs
- WindowsPen.cs
- UrlPath.cs
- ZipIOModeEnforcingStream.cs
- Pair.cs
- ArraySegment.cs
- SaveFileDialog.cs
- fixedPageContentExtractor.cs
- MarkupExtensionParser.cs
- XmlAttributeHolder.cs
- EntityExpressionVisitor.cs
- SQLDecimalStorage.cs
- HttpWebRequestElement.cs
- CounterSample.cs
- LiteralTextContainerControlBuilder.cs
- Compiler.cs
- SimpleType.cs
- ReferenceSchema.cs
- QualificationDataAttribute.cs
- SystemIcmpV6Statistics.cs