Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Annotations / AnnotationComponentChooser.cs / 1305600 / AnnotationComponentChooser.cs
//---------------------------------------------------------------------------- //// Copyright(C) Microsoft Corporation. All rights reserved. // // // Description: // AnnotationComponentChooser // // History: // 04/01/2004 axelk: Created AnnotationComponentChooser.cs // // Copyright(C) 2002 by Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Reflection; using System.Windows; using System.Windows.Controls; using MS.Internal.Annotations; using MS.Internal.Annotations.Component; namespace System.Windows.Annotations { ////// Instances of this class choose an IAnnotationComponent for a given AttachedAnnotation. /// The AnnotationService.Chooser DP is used to set such instances on the application tree. /// internal sealed class AnnotationComponentChooser { #region Public Statics /* * This member is not used in V1. Its only used to set no chooser but in V1 we don't * expose changing the chooser. We have one and only one chooser. * ////// Singleton to set no chooser to be used. /// public static readonly AnnotationComponentChooser None = new NoAnnotationComponentChooser(); * */ #endregion Public Statics #region Constructors ////// Return a default AnnotationComponentChooser /// public AnnotationComponentChooser() { } #endregion Constructors #region Public Methods ////// Choose an IAnnotationComponent for a given IAttachedAnnotation. Implementation in AnnotationComponentChooser knows /// about all out-of-box IAnnotationComponents. The default mapping will be stated here later. /// Subclasses can overwrite this method to return application specific mapping. /// Note: In future release this method should be made virtual. /// /// The IAttachedAnnotation that needs an IAnnotationComponent ///public IAnnotationComponent ChooseAnnotationComponent(IAttachedAnnotation attachedAnnotation) { if (attachedAnnotation == null) throw new ArgumentNullException("attachedAnnotation"); IAnnotationComponent ac = null; // Text StickyNote if (attachedAnnotation.Annotation.AnnotationType == StickyNoteControl.TextSchemaName) { ac = new StickyNoteControl(StickyNoteType.Text) as IAnnotationComponent; } // Ink StickyNote else if (attachedAnnotation.Annotation.AnnotationType == StickyNoteControl.InkSchemaName) { ac = new StickyNoteControl(StickyNoteType.Ink) as IAnnotationComponent; } // Highlight else if (attachedAnnotation.Annotation.AnnotationType == HighlightComponent.TypeName) { ac = new HighlightComponent() as IAnnotationComponent; } return ac; } #endregion Public Methods #region Private Classes /* * This class won't be used in V1. We have one and only one chooser. There's no way to change it. * /// /// There is only one instance of this class (in AnnotationComponentChooser.None), it always returns null for any given IAttachedAnnotation. /// It does not throw an exception for a null attached annotation. /// It indicates that no choosing should be performed in the subtree that the instance is attached to. /// private class NoAnnotationComponentChooser : AnnotationComponentChooser { public override IAnnotationComponent ChooseAnnotationComponent(IAttachedAnnotation attachedAnnotation) { return null; } } * */ #endregion Private Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
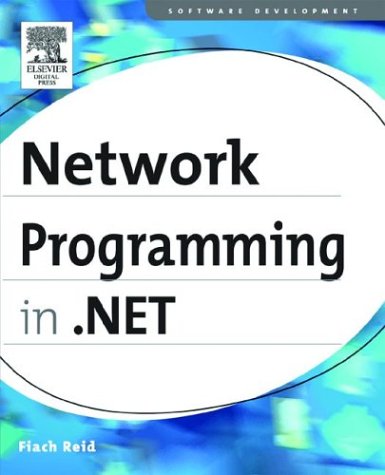
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HeaderCollection.cs
- DebuggerService.cs
- AttributeSetAction.cs
- ArrayWithOffset.cs
- TypeFieldSchema.cs
- ConfigXmlSignificantWhitespace.cs
- SqlAliaser.cs
- SingleSelectRootGridEntry.cs
- Constraint.cs
- PiiTraceSource.cs
- CompiledRegexRunnerFactory.cs
- EventToken.cs
- BinaryReader.cs
- SchemaTypeEmitter.cs
- TreeViewBindingsEditor.cs
- OrderToken.cs
- ChameleonKey.cs
- SchemaAttDef.cs
- MailWebEventProvider.cs
- StylusPointPropertyInfoDefaults.cs
- GlobalDataBindingHandler.cs
- ObjectStateEntryDbDataRecord.cs
- ExtenderProvidedPropertyAttribute.cs
- CodeSubDirectory.cs
- ContextMenuService.cs
- milrender.cs
- AutoResetEvent.cs
- WebPartAuthorizationEventArgs.cs
- ScheduleChanges.cs
- SettingsProperty.cs
- IncomingWebRequestContext.cs
- PolyBezierSegment.cs
- TwoPhaseCommitProxy.cs
- ScrollItemProviderWrapper.cs
- TrackingMemoryStream.cs
- DataGridViewMethods.cs
- StateMachineTimers.cs
- Path.cs
- ScrollBar.cs
- GeneralTransform3DCollection.cs
- DesignerFrame.cs
- FilteredReadOnlyMetadataCollection.cs
- ObjectItemCollection.cs
- StandardMenuStripVerb.cs
- Processor.cs
- EntityCollection.cs
- ClaimSet.cs
- Transform.cs
- complextypematerializer.cs
- AvTrace.cs
- CodeNamespaceImport.cs
- TextBoxDesigner.cs
- CustomAttributeBuilder.cs
- LinqDataSourceInsertEventArgs.cs
- LoginCancelEventArgs.cs
- EventOpcode.cs
- WebRequestModuleElementCollection.cs
- ComponentDispatcher.cs
- RelationshipEnd.cs
- ColumnTypeConverter.cs
- ExtensionDataReader.cs
- PolyLineSegmentFigureLogic.cs
- BuildTopDownAttribute.cs
- TabletDeviceInfo.cs
- WSSecurityJan2004.cs
- WorkflowInstanceExtensionProvider.cs
- CustomErrorsSection.cs
- ModifierKeysConverter.cs
- ViewStateException.cs
- GatewayIPAddressInformationCollection.cs
- JsonXmlDataContract.cs
- SqlDesignerDataSourceView.cs
- Label.cs
- MulticastNotSupportedException.cs
- Ipv6Element.cs
- FamilyMapCollection.cs
- RegistryKey.cs
- NativeRecognizer.cs
- SaveRecipientRequest.cs
- XmlException.cs
- VsPropertyGrid.cs
- BinaryCommonClasses.cs
- IIS7UserPrincipal.cs
- RestHandler.cs
- InputReportEventArgs.cs
- ZipIORawDataFileBlock.cs
- ApplicationServicesHostFactory.cs
- JapaneseCalendar.cs
- Condition.cs
- WinEventWrap.cs
- ShutDownListener.cs
- Type.cs
- processwaithandle.cs
- DataReceivedEventArgs.cs
- RawStylusInputCustomData.cs
- HostProtectionPermission.cs
- ProcessHostServerConfig.cs
- XmlBinaryWriter.cs
- HasCopySemanticsAttribute.cs
- CommonGetThemePartSize.cs