Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / WebControls / SqlDesignerDataSourceView.cs / 1 / SqlDesignerDataSourceView.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.WebControls { using System; using System.Collections; using System.Data; using System.Web.UI.WebControls; ////// SqlDesignerDataSourceView is the designer view associated with a SqlDataSourceDesigner. /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags = System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public class SqlDesignerDataSourceView : DesignerDataSourceView { private SqlDataSourceDesigner _owner; public SqlDesignerDataSourceView(SqlDataSourceDesigner owner, string viewName) : base(owner, viewName) { _owner = owner; } public override bool CanDelete { get { return (_owner.SqlDataSource.DeleteCommand.Length > 0); } } public override bool CanInsert { get { return (_owner.SqlDataSource.InsertCommand.Length > 0); } } public override bool CanPage { get { return false; } } public override bool CanRetrieveTotalRowCount { get { return false; } } public override bool CanSort { get { return (_owner.SqlDataSource.DataSourceMode == SqlDataSourceMode.DataSet) || (_owner.SqlDataSource.SortParameterName.Length > 0); } } public override bool CanUpdate { get { return (_owner.SqlDataSource.UpdateCommand.Length > 0); } } public override IDataSourceViewSchema Schema { get { DataTable schemaTable = _owner.LoadSchema(); if (schemaTable == null) { return null; } return new DataSetViewSchema(schemaTable); } } public override IEnumerable GetDesignTimeData(int minimumRows, out bool isSampleData) { DataTable schemaTable = _owner.LoadSchema(); if (schemaTable != null) { isSampleData = true; return DesignTimeData.GetDesignTimeDataSource(DesignTimeData.CreateSampleDataTable(new DataView(schemaTable), true), minimumRows); } // Couldn't find design-time schema, use base implementation return base.GetDesignTimeData(minimumRows, out isSampleData); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
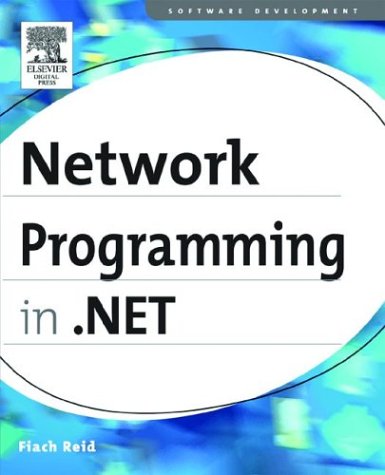
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataService.cs
- AsyncWaitHandle.cs
- ComboBoxItem.cs
- DES.cs
- FileCodeGroup.cs
- PromptStyle.cs
- DragEvent.cs
- ControlValuePropertyAttribute.cs
- TextElementCollection.cs
- TextRunProperties.cs
- DetailsViewUpdatedEventArgs.cs
- DetailsViewUpdatedEventArgs.cs
- SingleConverter.cs
- GridViewPageEventArgs.cs
- SimpleWorkerRequest.cs
- DataGridViewColumnEventArgs.cs
- ToolboxItem.cs
- StylusDownEventArgs.cs
- XmlCollation.cs
- SqlCacheDependencyDatabaseCollection.cs
- SoapEnumAttribute.cs
- NativeMethods.cs
- SettingsProperty.cs
- KeySplineConverter.cs
- CodeArgumentReferenceExpression.cs
- QueryCacheManager.cs
- OleDbParameterCollection.cs
- ConnectionString.cs
- CompressionTracing.cs
- LiteralLink.cs
- PassportPrincipal.cs
- DataBoundLiteralControl.cs
- MessageTraceRecord.cs
- SessionIDManager.cs
- XamlDesignerSerializationManager.cs
- SupportsEventValidationAttribute.cs
- CharConverter.cs
- DataObjectSettingDataEventArgs.cs
- TripleDES.cs
- ProviderConnectionPoint.cs
- GenerateHelper.cs
- codemethodreferenceexpression.cs
- Baml2006KeyRecord.cs
- GlobalProxySelection.cs
- GridItemPattern.cs
- HttpCapabilitiesSectionHandler.cs
- SemaphoreSlim.cs
- XmlIgnoreAttribute.cs
- TextElementEnumerator.cs
- SrgsNameValueTag.cs
- SiteMapProvider.cs
- SystemWebSectionGroup.cs
- WSSecurityOneDotZeroSendSecurityHeader.cs
- RequestQueue.cs
- WebServiceData.cs
- ResponseStream.cs
- TextAutomationPeer.cs
- Boolean.cs
- OutputCacheModule.cs
- MessageDecoder.cs
- EmissiveMaterial.cs
- BaseCodePageEncoding.cs
- PTUtility.cs
- HttpCachePolicy.cs
- PointLight.cs
- StatusBarItemAutomationPeer.cs
- ResourceReader.cs
- OleDbDataAdapter.cs
- UnitControl.cs
- DataControlButton.cs
- CurrencyManager.cs
- GiveFeedbackEvent.cs
- WinFormsUtils.cs
- WebControlsSection.cs
- ToolboxItemAttribute.cs
- MultiAsyncResult.cs
- MaskDesignerDialog.cs
- AuthenticationSection.cs
- StopRoutingHandler.cs
- HScrollBar.cs
- RuntimeHelpers.cs
- mediaeventargs.cs
- EventToken.cs
- SessionPageStatePersister.cs
- DomainLiteralReader.cs
- WindowsTreeView.cs
- EventHandlerService.cs
- ReliabilityContractAttribute.cs
- Point4D.cs
- StylusButton.cs
- FigureParagraph.cs
- SequenceRange.cs
- MenuEventArgs.cs
- SiteMapPathDesigner.cs
- PropertyEmitter.cs
- MatrixCamera.cs
- Clause.cs
- Registry.cs
- Native.cs
- FormParameter.cs