Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / Stylus / StylusButton.cs / 1 / StylusButton.cs
using System; using System.Globalization; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// StylusButton class /// public class StylusButton { ///////////////////////////////////////////////////////////////////// internal StylusButton(string name, Guid id) { _name = name; _guid = id; } ///////////////////////////////////////////////////////////////////// ////// Returns the hardware Guid of the StylusDevice button. /// public Guid Guid { get { return _guid; } } ///////////////////////////////////////////////////////////////////// ////// Returns the current state of the button. /// public StylusButtonState StylusButtonState { // get { StylusPointCollection stylusPoints = StylusDevice.GetStylusPoints(null); if (stylusPoints == null || stylusPoints.Count == 0) return CachedButtonState; return (StylusButtonState)stylusPoints[stylusPoints.Count - 1].GetPropertyValue(new StylusPointProperty(Guid, true)); } } internal StylusButtonState CachedButtonState { get { return _cachedButtonState; } set { _cachedButtonState = value; } } ///////////////////////////////////////////////////////////////////// ////// Returns the name of the button. /// public string Name { get { return _name; } } ///////////////////////////////////////////////////////////////////// ////// Returns StylusDevice object that owns this button. /// public StylusDevice StylusDevice { get { return _stylusDevice; } } ///////////////////////////////////////////////////////////////////// /// internal void SetOwner(StylusDevice stylusDevice) { _stylusDevice = stylusDevice; } ///////////////////////////////////////////////////////////////////// ////// Returns the friendly representation of the button object /// ///public override string ToString() { return String.Format(CultureInfo.CurrentCulture, "{0}({1})", base.ToString(), this.Name); } ///////////////////////////////////////////////////////////////////// StylusDevice _stylusDevice; string _name; Guid _guid; StylusButtonState _cachedButtonState = StylusButtonState.Up; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Globalization; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// /// name of the tablet /// StylusButton class /// public class StylusButton { ///////////////////////////////////////////////////////////////////// internal StylusButton(string name, Guid id) { _name = name; _guid = id; } ///////////////////////////////////////////////////////////////////// ////// Returns the hardware Guid of the StylusDevice button. /// public Guid Guid { get { return _guid; } } ///////////////////////////////////////////////////////////////////// ////// Returns the current state of the button. /// public StylusButtonState StylusButtonState { // get { StylusPointCollection stylusPoints = StylusDevice.GetStylusPoints(null); if (stylusPoints == null || stylusPoints.Count == 0) return CachedButtonState; return (StylusButtonState)stylusPoints[stylusPoints.Count - 1].GetPropertyValue(new StylusPointProperty(Guid, true)); } } internal StylusButtonState CachedButtonState { get { return _cachedButtonState; } set { _cachedButtonState = value; } } ///////////////////////////////////////////////////////////////////// ////// Returns the name of the button. /// public string Name { get { return _name; } } ///////////////////////////////////////////////////////////////////// ////// Returns StylusDevice object that owns this button. /// public StylusDevice StylusDevice { get { return _stylusDevice; } } ///////////////////////////////////////////////////////////////////// /// internal void SetOwner(StylusDevice stylusDevice) { _stylusDevice = stylusDevice; } ///////////////////////////////////////////////////////////////////// ////// Returns the friendly representation of the button object /// ///public override string ToString() { return String.Format(CultureInfo.CurrentCulture, "{0}({1})", base.ToString(), this.Name); } ///////////////////////////////////////////////////////////////////// StylusDevice _stylusDevice; string _name; Guid _guid; StylusButtonState _cachedButtonState = StylusButtonState.Up; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. name of the tablet
Link Menu
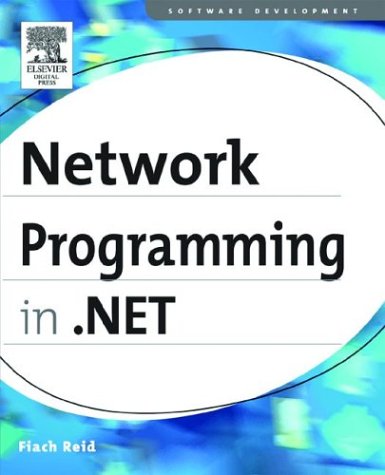
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeFileMappingHandle.cs
- MultiSelectRootGridEntry.cs
- LookupBindingPropertiesAttribute.cs
- DbTransaction.cs
- ComponentCollection.cs
- TransformPatternIdentifiers.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- MailAddress.cs
- Rfc2898DeriveBytes.cs
- CodeAttributeDeclarationCollection.cs
- HandlerBase.cs
- StateBag.cs
- SqlUserDefinedTypeAttribute.cs
- Regex.cs
- DataSetMappper.cs
- WmfPlaceableFileHeader.cs
- CodeCompileUnit.cs
- ClientUtils.cs
- Ipv6Element.cs
- BufferedReadStream.cs
- ProfileSection.cs
- ReaderOutput.cs
- RegexMatchCollection.cs
- RefreshPropertiesAttribute.cs
- SignatureDescription.cs
- StateChangeEvent.cs
- AutomationEventArgs.cs
- StatusBarItemAutomationPeer.cs
- Unit.cs
- updateconfighost.cs
- DataSourceControl.cs
- ItemCollection.cs
- ComNativeDescriptor.cs
- GACMembershipCondition.cs
- BindingFormattingDialog.cs
- WebPartCancelEventArgs.cs
- XmlDataImplementation.cs
- XMLSchema.cs
- ConfigurationValidatorBase.cs
- MatrixStack.cs
- SafeNativeMethods.cs
- TableLayoutPanel.cs
- OracleTimeSpan.cs
- FileVersion.cs
- SevenBitStream.cs
- entityreference_tresulttype.cs
- Control.cs
- SoapAttributeAttribute.cs
- SingleObjectCollection.cs
- CodeMethodMap.cs
- DelegateBodyWriter.cs
- StateMachineSubscription.cs
- NetworkStream.cs
- ConfigXmlElement.cs
- SendingRequestEventArgs.cs
- RecipientInfo.cs
- ExtendLockCommand.cs
- RepeatInfo.cs
- CounterSetInstance.cs
- RuleSettingsCollection.cs
- PenContext.cs
- Form.cs
- InternalConfigHost.cs
- _LazyAsyncResult.cs
- Screen.cs
- XsdDuration.cs
- _AcceptOverlappedAsyncResult.cs
- Tuple.cs
- Helper.cs
- SQLInt64.cs
- DataBindEngine.cs
- BitStack.cs
- X509PeerCertificateAuthentication.cs
- TrackingProfile.cs
- Panel.cs
- IsolatedStorageException.cs
- TextRangeEditTables.cs
- SqlGatherConsumedAliases.cs
- PolygonHotSpot.cs
- HandlerMappingMemo.cs
- LogReserveAndAppendState.cs
- DESCryptoServiceProvider.cs
- InstanceLockException.cs
- BStrWrapper.cs
- TableRowCollection.cs
- CodeObject.cs
- BamlTreeNode.cs
- SafeFileHandle.cs
- EntityViewContainer.cs
- TranslateTransform3D.cs
- ControlParser.cs
- GenericIdentity.cs
- BrowserCapabilitiesFactoryBase.cs
- RoleService.cs
- SecondaryIndexDefinition.cs
- ApplicationInfo.cs
- FileFormatException.cs
- ReadOnlyHierarchicalDataSourceView.cs
- Logging.cs
- control.ime.cs