Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / Configuration / BrowserCapabilitiesFactoryBase.cs / 3 / BrowserCapabilitiesFactoryBase.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Base class for browser capabilities object: just a read-only dictionary * holder that supports Init() * * */ using System.Web.UI; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Globalization; using System.Security.Permissions; using System.Text; using System.Text.RegularExpressions; using System.Web.Util; namespace System.Web.Configuration { [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class BrowserCapabilitiesFactoryBase { private IDictionary _matchedHeaders; private IDictionary _browserElements; private object _lock = new object(); public BrowserCapabilitiesFactoryBase() { } [EditorBrowsable(EditorBrowsableState.Advanced)] protected IDictionary BrowserElements { get { if (_browserElements == null) lock (_lock) { if (_browserElements == null) { _browserElements = Hashtable.Synchronized(new Hashtable(StringComparer.OrdinalIgnoreCase)); PopulateBrowserElements(_browserElements); } } return _browserElements; } } [EditorBrowsable(EditorBrowsableState.Advanced)] protected virtual void PopulateBrowserElements(IDictionary dictionary) { } internal IDictionary InternalGetMatchedHeaders() { return MatchedHeaders; } internal IDictionary InternalGetBrowserElements() { return BrowserElements; } [EditorBrowsable(EditorBrowsableState.Advanced)] protected IDictionary MatchedHeaders { get { if (_matchedHeaders == null) lock (_lock) { if (_matchedHeaders == null) { _matchedHeaders = Hashtable.Synchronized(new Hashtable(24, StringComparer.OrdinalIgnoreCase)); PopulateMatchedHeaders(_matchedHeaders); } } return _matchedHeaders; } } [EditorBrowsable(EditorBrowsableState.Advanced)] protected virtual void PopulateMatchedHeaders(IDictionary dictionary) { } internal int CompareFilters(string filter1, string filter2) { bool isFilter1DefaultFilter = String.IsNullOrEmpty(filter1); bool isFilter2DefaultFilter = String.IsNullOrEmpty(filter2); IDictionary browsers = BrowserElements; bool filter1Exists = (browsers.Contains(filter1)) || isFilter1DefaultFilter; bool filter2Exists = (browsers.Contains(filter2)) || isFilter2DefaultFilter; if (!filter1Exists) { if (!filter2Exists) { return 0; } else { return -1; } } else { if (!filter2Exists) { return 1; } } if (isFilter1DefaultFilter && !isFilter2DefaultFilter) { return 1; } if (isFilter2DefaultFilter && !isFilter1DefaultFilter) { return -1; } if (isFilter1DefaultFilter && isFilter2DefaultFilter) { return 0; } int filter1Depth = (int)((Triplet)BrowserElements[filter1]).Third; int filter2Depth = (int)((Triplet)BrowserElements[filter2]).Third; return filter2Depth - filter1Depth; } public virtual void ConfigureBrowserCapabilities(NameValueCollection headers, HttpBrowserCapabilities browserCaps) { } // CodeGenerator will override this function to declare custom browser capabilities public virtual void ConfigureCustomCapabilities(NameValueCollection headers, HttpBrowserCapabilities browserCaps) { } internal static string GetBrowserCapKey(IDictionary headers, HttpRequest request) { StringBuilder sb = new StringBuilder(); foreach(String key in headers.Keys) { if (key.Length == 0) { sb.Append(HttpCapabilitiesEvaluator.GetUserAgent(request)); } else { sb.Append(request.Headers[key]); } sb.Append("\n"); } return sb.ToString(); } internal HttpBrowserCapabilities GetHttpBrowserCapabilities(HttpRequest request) { if (request == null) throw new ArgumentNullException("request"); NameValueCollection headers = request.Headers; HttpBrowserCapabilities browserCaps = new HttpBrowserCapabilities(); Hashtable values = new Hashtable(180, StringComparer.OrdinalIgnoreCase); values[String.Empty] = HttpCapabilitiesEvaluator.GetUserAgent(request); browserCaps.Capabilities = values; ConfigureBrowserCapabilities(headers, browserCaps); ConfigureCustomCapabilities(headers, browserCaps); return browserCaps; } protected bool IsBrowserUnknown(HttpCapabilitiesBase browserCaps) { // We want to ignore the "Default" node, which will also be matched. if(browserCaps.Browsers == null || browserCaps.Browsers.Count <= 1) { return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Base class for browser capabilities object: just a read-only dictionary * holder that supports Init() * * */ using System.Web.UI; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Globalization; using System.Security.Permissions; using System.Text; using System.Text.RegularExpressions; using System.Web.Util; namespace System.Web.Configuration { [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class BrowserCapabilitiesFactoryBase { private IDictionary _matchedHeaders; private IDictionary _browserElements; private object _lock = new object(); public BrowserCapabilitiesFactoryBase() { } [EditorBrowsable(EditorBrowsableState.Advanced)] protected IDictionary BrowserElements { get { if (_browserElements == null) lock (_lock) { if (_browserElements == null) { _browserElements = Hashtable.Synchronized(new Hashtable(StringComparer.OrdinalIgnoreCase)); PopulateBrowserElements(_browserElements); } } return _browserElements; } } [EditorBrowsable(EditorBrowsableState.Advanced)] protected virtual void PopulateBrowserElements(IDictionary dictionary) { } internal IDictionary InternalGetMatchedHeaders() { return MatchedHeaders; } internal IDictionary InternalGetBrowserElements() { return BrowserElements; } [EditorBrowsable(EditorBrowsableState.Advanced)] protected IDictionary MatchedHeaders { get { if (_matchedHeaders == null) lock (_lock) { if (_matchedHeaders == null) { _matchedHeaders = Hashtable.Synchronized(new Hashtable(24, StringComparer.OrdinalIgnoreCase)); PopulateMatchedHeaders(_matchedHeaders); } } return _matchedHeaders; } } [EditorBrowsable(EditorBrowsableState.Advanced)] protected virtual void PopulateMatchedHeaders(IDictionary dictionary) { } internal int CompareFilters(string filter1, string filter2) { bool isFilter1DefaultFilter = String.IsNullOrEmpty(filter1); bool isFilter2DefaultFilter = String.IsNullOrEmpty(filter2); IDictionary browsers = BrowserElements; bool filter1Exists = (browsers.Contains(filter1)) || isFilter1DefaultFilter; bool filter2Exists = (browsers.Contains(filter2)) || isFilter2DefaultFilter; if (!filter1Exists) { if (!filter2Exists) { return 0; } else { return -1; } } else { if (!filter2Exists) { return 1; } } if (isFilter1DefaultFilter && !isFilter2DefaultFilter) { return 1; } if (isFilter2DefaultFilter && !isFilter1DefaultFilter) { return -1; } if (isFilter1DefaultFilter && isFilter2DefaultFilter) { return 0; } int filter1Depth = (int)((Triplet)BrowserElements[filter1]).Third; int filter2Depth = (int)((Triplet)BrowserElements[filter2]).Third; return filter2Depth - filter1Depth; } public virtual void ConfigureBrowserCapabilities(NameValueCollection headers, HttpBrowserCapabilities browserCaps) { } // CodeGenerator will override this function to declare custom browser capabilities public virtual void ConfigureCustomCapabilities(NameValueCollection headers, HttpBrowserCapabilities browserCaps) { } internal static string GetBrowserCapKey(IDictionary headers, HttpRequest request) { StringBuilder sb = new StringBuilder(); foreach(String key in headers.Keys) { if (key.Length == 0) { sb.Append(HttpCapabilitiesEvaluator.GetUserAgent(request)); } else { sb.Append(request.Headers[key]); } sb.Append("\n"); } return sb.ToString(); } internal HttpBrowserCapabilities GetHttpBrowserCapabilities(HttpRequest request) { if (request == null) throw new ArgumentNullException("request"); NameValueCollection headers = request.Headers; HttpBrowserCapabilities browserCaps = new HttpBrowserCapabilities(); Hashtable values = new Hashtable(180, StringComparer.OrdinalIgnoreCase); values[String.Empty] = HttpCapabilitiesEvaluator.GetUserAgent(request); browserCaps.Capabilities = values; ConfigureBrowserCapabilities(headers, browserCaps); ConfigureCustomCapabilities(headers, browserCaps); return browserCaps; } protected bool IsBrowserUnknown(HttpCapabilitiesBase browserCaps) { // We want to ignore the "Default" node, which will also be matched. if(browserCaps.Browsers == null || browserCaps.Browsers.Count <= 1) { return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
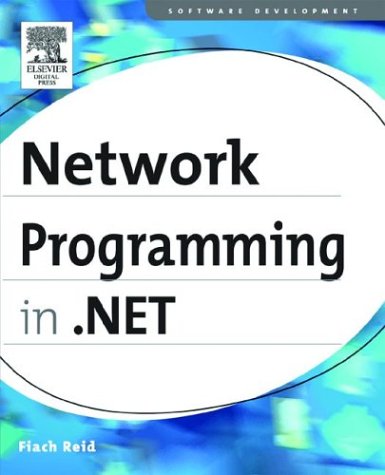
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProfessionalColorTable.cs
- WindowsContainer.cs
- GridViewUpdatedEventArgs.cs
- Models.cs
- xmlsaver.cs
- WindowsGraphics.cs
- CodeAttributeArgumentCollection.cs
- DropSource.cs
- ChtmlTextWriter.cs
- NetworkInformationPermission.cs
- OleDbStruct.cs
- ParsedAttributeCollection.cs
- OperationInfo.cs
- NamespaceTable.cs
- RichTextBoxAutomationPeer.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- KnownTypesProvider.cs
- LicenseException.cs
- InternalDispatchObject.cs
- GeneralTransform3DTo2DTo3D.cs
- Accessible.cs
- ConfigXmlWhitespace.cs
- HttpCapabilitiesEvaluator.cs
- SchemaImporterExtension.cs
- PanningMessageFilter.cs
- CalendarButtonAutomationPeer.cs
- TypedReference.cs
- Evidence.cs
- ConfigurationLocation.cs
- RectAnimationClockResource.cs
- VScrollBar.cs
- SocketAddress.cs
- MostlySingletonList.cs
- DefaultShape.cs
- CodeAssignStatement.cs
- DictionaryContent.cs
- SoapSchemaMember.cs
- AlternationConverter.cs
- DeobfuscatingStream.cs
- ExtenderControl.cs
- RefreshEventArgs.cs
- TrustExchangeException.cs
- SettingsBindableAttribute.cs
- PrintPreviewGraphics.cs
- HtmlInputButton.cs
- DesignerActionService.cs
- DropSource.cs
- ParameterCollection.cs
- GeneralTransform2DTo3D.cs
- TrackingRecord.cs
- CacheChildrenQuery.cs
- SynchronizedInputPattern.cs
- RepeatBehavior.cs
- TypeForwardedToAttribute.cs
- ImageListStreamer.cs
- Splitter.cs
- NoneExcludedImageIndexConverter.cs
- ThreadInterruptedException.cs
- ShaderRenderModeValidation.cs
- MarkupObject.cs
- BrushConverter.cs
- ToolStripRenderer.cs
- ObjRef.cs
- Match.cs
- PixelShader.cs
- RelatedPropertyManager.cs
- SqlCacheDependencyDatabaseCollection.cs
- StyleBamlTreeBuilder.cs
- CreatingCookieEventArgs.cs
- RowType.cs
- PageRanges.cs
- SoapReflectionImporter.cs
- Guid.cs
- DBConnection.cs
- CodeRegionDirective.cs
- FixedTextBuilder.cs
- COM2ICategorizePropertiesHandler.cs
- TextBoxView.cs
- UserPreferenceChangedEventArgs.cs
- CachedRequestParams.cs
- RegistrySecurity.cs
- COAUTHIDENTITY.cs
- PackagePart.cs
- TextStore.cs
- BamlMapTable.cs
- ICollection.cs
- StreamReader.cs
- EpmCustomContentDeSerializer.cs
- Polygon.cs
- EncryptedPackage.cs
- ElementHost.cs
- TransformPatternIdentifiers.cs
- GeneratedView.cs
- EnvelopedSignatureTransform.cs
- TreeNodeBindingDepthConverter.cs
- ChangeProcessor.cs
- altserialization.cs
- BooleanAnimationBase.cs
- _NegoStream.cs
- ContentType.cs