Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Design / MessageFilters / PanningMessageFilter.cs / 1305376 / PanningMessageFilter.cs
namespace System.Workflow.ComponentModel.Design { using System; using System.Drawing; using System.Windows.Forms; using System.ComponentModel.Design; #region Class PanningMessageFilter ///This behavior needs and stores coordinates in client coordinates internal sealed class PanningMessageFilter : WorkflowDesignerMessageFilter { #region Members and Constructor private static Cursor PanBeganCursor = new Cursor(typeof(WorkflowView), "Resources.panClosed.cur"); private static Cursor PanReadyCursor = new Cursor(typeof(WorkflowView), "Resources.panOpened.cur"); private Point panPoint = Point.Empty; private bool panningActive = false; private CommandID previousCommand; private Cursor previousCursor = Cursors.Default; internal PanningMessageFilter() { } #endregion #region MessageFilter Overrides protected override void Initialize(WorkflowView parentView) { base.Initialize(parentView); StoreUIState(); RefreshUIState(); } protected override void Dispose(bool disposing) { try { RestoreUIState(); } finally { base.Dispose(disposing); } } protected override bool OnShowContextMenu(Point menuPoint) { IMenuCommandService menuCommandService = (IMenuCommandService)GetService(typeof(IMenuCommandService)); if (menuCommandService != null) menuCommandService.ShowContextMenu(WorkflowMenuCommands.ZoomMenu, menuPoint.X, menuPoint.Y); return true; } protected override bool OnMouseEnter(MouseEventArgs eventArgs) { RefreshUIState(); return true; } protected override bool OnMouseDown(MouseEventArgs eventArgs) { if (eventArgs.Button == MouseButtons.Left) SetPanPoint(new Point(eventArgs.X, eventArgs.Y)); return true; } protected override bool OnMouseMove(MouseEventArgs eventArgs) { if (this.panningActive && (eventArgs.Button & MouseButtons.Left) > 0) { Size panSize = new Size(eventArgs.X - this.panPoint.X, eventArgs.Y - this.panPoint.Y); WorkflowView parentView = ParentView; parentView.ScrollPosition = new Point(parentView.ScrollPosition.X - panSize.Width, parentView.ScrollPosition.Y - panSize.Height); SetPanPoint(new Point(eventArgs.X, eventArgs.Y)); } return true; } protected override bool OnMouseUp(MouseEventArgs eventArgs) { SetPanPoint(Point.Empty); return true; } protected override bool OnDragEnter(DragEventArgs eventArgs) { ParentView.RemoveDesignerMessageFilter(this); return false; } protected override bool OnKeyDown(KeyEventArgs eventArgs) { if (eventArgs.KeyValue == (int)Keys.Escape) ParentView.RemoveDesignerMessageFilter(this); return true; } #endregion #region Helpers private void SetPanPoint(Point value) { this.panPoint = value; this.panningActive = (this.panPoint != Point.Empty); ParentView.Capture = this.panningActive; RefreshUIState(); } private void RefreshUIState() { //Update the cursor ParentView.Cursor = (this.panningActive) ? PanningMessageFilter.PanBeganCursor : PanningMessageFilter.PanReadyCursor; //Update the menu command IMenuCommandService menuCommandService = GetService(typeof(IMenuCommandService)) as IMenuCommandService; if (menuCommandService != null) { CommandID[] affectedCommands = new CommandID[] { WorkflowMenuCommands.ZoomIn, WorkflowMenuCommands.ZoomOut, WorkflowMenuCommands.Pan, WorkflowMenuCommands.DefaultFilter }; foreach (CommandID affectedCommand in affectedCommands) { MenuCommand menuCommand = menuCommandService.FindCommand(affectedCommand); if (menuCommand != null && menuCommand.Enabled) menuCommand.Checked = (menuCommand.CommandID == WorkflowMenuCommands.Pan); } } } private void StoreUIState() { IMenuCommandService menuCommandService = GetService(typeof(IMenuCommandService)) as IMenuCommandService; if (menuCommandService != null) { foreach (CommandID affectedCommand in CommandSet.NavigationToolCommandIds) { MenuCommand menuCommand = menuCommandService.FindCommand(affectedCommand); if (menuCommand != null && menuCommand.Enabled && menuCommand.Checked) { this.previousCommand = menuCommand.CommandID; break; } } } this.previousCursor = ParentView.Cursor; } private void RestoreUIState() { IMenuCommandService menuCommandService = GetService(typeof(IMenuCommandService)) as IMenuCommandService; if (menuCommandService != null) { foreach (CommandID affectedCommand in CommandSet.NavigationToolCommandIds) { MenuCommand menuCommand = menuCommandService.FindCommand(affectedCommand); if (menuCommand != null && menuCommand.Enabled) menuCommand.Checked = (menuCommand.CommandID == this.previousCommand); } } ParentView.Cursor = this.previousCursor; } #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Workflow.ComponentModel.Design { using System; using System.Drawing; using System.Windows.Forms; using System.ComponentModel.Design; #region Class PanningMessageFilter ///This behavior needs and stores coordinates in client coordinates internal sealed class PanningMessageFilter : WorkflowDesignerMessageFilter { #region Members and Constructor private static Cursor PanBeganCursor = new Cursor(typeof(WorkflowView), "Resources.panClosed.cur"); private static Cursor PanReadyCursor = new Cursor(typeof(WorkflowView), "Resources.panOpened.cur"); private Point panPoint = Point.Empty; private bool panningActive = false; private CommandID previousCommand; private Cursor previousCursor = Cursors.Default; internal PanningMessageFilter() { } #endregion #region MessageFilter Overrides protected override void Initialize(WorkflowView parentView) { base.Initialize(parentView); StoreUIState(); RefreshUIState(); } protected override void Dispose(bool disposing) { try { RestoreUIState(); } finally { base.Dispose(disposing); } } protected override bool OnShowContextMenu(Point menuPoint) { IMenuCommandService menuCommandService = (IMenuCommandService)GetService(typeof(IMenuCommandService)); if (menuCommandService != null) menuCommandService.ShowContextMenu(WorkflowMenuCommands.ZoomMenu, menuPoint.X, menuPoint.Y); return true; } protected override bool OnMouseEnter(MouseEventArgs eventArgs) { RefreshUIState(); return true; } protected override bool OnMouseDown(MouseEventArgs eventArgs) { if (eventArgs.Button == MouseButtons.Left) SetPanPoint(new Point(eventArgs.X, eventArgs.Y)); return true; } protected override bool OnMouseMove(MouseEventArgs eventArgs) { if (this.panningActive && (eventArgs.Button & MouseButtons.Left) > 0) { Size panSize = new Size(eventArgs.X - this.panPoint.X, eventArgs.Y - this.panPoint.Y); WorkflowView parentView = ParentView; parentView.ScrollPosition = new Point(parentView.ScrollPosition.X - panSize.Width, parentView.ScrollPosition.Y - panSize.Height); SetPanPoint(new Point(eventArgs.X, eventArgs.Y)); } return true; } protected override bool OnMouseUp(MouseEventArgs eventArgs) { SetPanPoint(Point.Empty); return true; } protected override bool OnDragEnter(DragEventArgs eventArgs) { ParentView.RemoveDesignerMessageFilter(this); return false; } protected override bool OnKeyDown(KeyEventArgs eventArgs) { if (eventArgs.KeyValue == (int)Keys.Escape) ParentView.RemoveDesignerMessageFilter(this); return true; } #endregion #region Helpers private void SetPanPoint(Point value) { this.panPoint = value; this.panningActive = (this.panPoint != Point.Empty); ParentView.Capture = this.panningActive; RefreshUIState(); } private void RefreshUIState() { //Update the cursor ParentView.Cursor = (this.panningActive) ? PanningMessageFilter.PanBeganCursor : PanningMessageFilter.PanReadyCursor; //Update the menu command IMenuCommandService menuCommandService = GetService(typeof(IMenuCommandService)) as IMenuCommandService; if (menuCommandService != null) { CommandID[] affectedCommands = new CommandID[] { WorkflowMenuCommands.ZoomIn, WorkflowMenuCommands.ZoomOut, WorkflowMenuCommands.Pan, WorkflowMenuCommands.DefaultFilter }; foreach (CommandID affectedCommand in affectedCommands) { MenuCommand menuCommand = menuCommandService.FindCommand(affectedCommand); if (menuCommand != null && menuCommand.Enabled) menuCommand.Checked = (menuCommand.CommandID == WorkflowMenuCommands.Pan); } } } private void StoreUIState() { IMenuCommandService menuCommandService = GetService(typeof(IMenuCommandService)) as IMenuCommandService; if (menuCommandService != null) { foreach (CommandID affectedCommand in CommandSet.NavigationToolCommandIds) { MenuCommand menuCommand = menuCommandService.FindCommand(affectedCommand); if (menuCommand != null && menuCommand.Enabled && menuCommand.Checked) { this.previousCommand = menuCommand.CommandID; break; } } } this.previousCursor = ParentView.Cursor; } private void RestoreUIState() { IMenuCommandService menuCommandService = GetService(typeof(IMenuCommandService)) as IMenuCommandService; if (menuCommandService != null) { foreach (CommandID affectedCommand in CommandSet.NavigationToolCommandIds) { MenuCommand menuCommand = menuCommandService.FindCommand(affectedCommand); if (menuCommand != null && menuCommand.Enabled) menuCommand.Checked = (menuCommand.CommandID == this.previousCommand); } } ParentView.Cursor = this.previousCursor; } #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
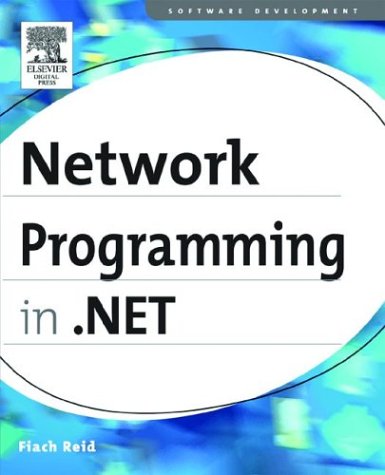
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SizeChangedInfo.cs
- QueryOutputWriterV1.cs
- QilSortKey.cs
- NamespaceQuery.cs
- FixedSOMTableCell.cs
- OrderedDictionary.cs
- Int16Storage.cs
- LambdaCompiler.Binary.cs
- CopyOfAction.cs
- WindowsRichEditRange.cs
- DomainConstraint.cs
- storepermission.cs
- HttpCookie.cs
- DataGridViewSelectedCellCollection.cs
- ReadonlyMessageFilter.cs
- XmlDataCollection.cs
- TypeElement.cs
- ListSourceHelper.cs
- LabelInfo.cs
- UrlAuthorizationModule.cs
- ChtmlTextWriter.cs
- ObjectSet.cs
- ToolStripControlHost.cs
- CustomValidator.cs
- IRCollection.cs
- IsolatedStorageFile.cs
- PropertyPathWorker.cs
- RawStylusActions.cs
- ProcessRequestArgs.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- ConfigurationErrorsException.cs
- XslNumber.cs
- AttachedAnnotation.cs
- EntityDataSourceContainerNameConverter.cs
- ExpressionConverter.cs
- ArrangedElementCollection.cs
- XsdDataContractImporter.cs
- SqlDataAdapter.cs
- Membership.cs
- DescendantOverDescendantQuery.cs
- ReadOnlyHierarchicalDataSource.cs
- InputReportEventArgs.cs
- TextReader.cs
- QueryHandler.cs
- RawAppCommandInputReport.cs
- ResourceProviderFactory.cs
- Matrix3DConverter.cs
- StackOverflowException.cs
- CatalogPart.cs
- UrlMappingsModule.cs
- RoutedUICommand.cs
- SqlTrackingService.cs
- _LocalDataStore.cs
- NoResizeHandleGlyph.cs
- WizardStepBase.cs
- AppSettingsExpressionBuilder.cs
- RowsCopiedEventArgs.cs
- _NTAuthentication.cs
- ChangePassword.cs
- ProtocolsConfigurationHandler.cs
- ChangeConflicts.cs
- NavigatingCancelEventArgs.cs
- ConsumerConnectionPointCollection.cs
- BaseDataList.cs
- InkCanvasSelection.cs
- AnnotationStore.cs
- EditorAttribute.cs
- ComplusTypeValidator.cs
- DictionarySectionHandler.cs
- MethodCallExpression.cs
- AssemblyBuilderData.cs
- WorkflowPrinting.cs
- SingleObjectCollection.cs
- __ComObject.cs
- Attributes.cs
- Help.cs
- InvalidFilterCriteriaException.cs
- ToolboxComponentsCreatedEventArgs.cs
- ConnectionInterfaceCollection.cs
- EditableLabelControl.cs
- ActivityStateQuery.cs
- RenderContext.cs
- CacheOutputQuery.cs
- PerfProviderCollection.cs
- HttpProfileBase.cs
- FlowThrottle.cs
- XmlNamespaceDeclarationsAttribute.cs
- ButtonBase.cs
- RelationHandler.cs
- Debug.cs
- ArgumentException.cs
- DomainLiteralReader.cs
- InternalBase.cs
- DirectoryNotFoundException.cs
- ListenerTraceUtility.cs
- MarkupProperty.cs
- ImmComposition.cs
- OptimalBreakSession.cs
- WsdlHelpGeneratorElement.cs
- PriorityItem.cs