Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Diagnostics / PerformanceData / PerfProviderCollection.cs / 1305376 / PerfProviderCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Diagnostics.PerformanceData { using System; using System.Threading; using System.ComponentModel; using System.Collections.Generic; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using Microsoft.Win32; using Microsoft.Win32.SafeHandles; internal sealed class PerfProvider { internal Guid m_providerGuid; internal Int32 m_counterSet; internal SafePerfProviderHandle m_hProvider; //// [System.Security.SecurityCritical] internal PerfProvider(Guid providerGuid) { m_providerGuid = providerGuid; uint Status = UnsafeNativeMethods.PerfStartProvider(ref m_providerGuid, null, out m_hProvider); // ERROR_INVALID_PARAMETER, ERROR_OUTOFMEMORY if (Status != (uint) UnsafeNativeMethods.ERROR_SUCCESS) { throw new Win32Exception((int) Status); } } } internal static class PerfProviderCollection { // Internal global PERFLIB V2 provider collection that contains a collection of PerfProvider objects. // Use mutex to serialize collection initialization/update. // private static Object s_hiddenInternalSyncObject; private static List// // // s_providerList = new List (); private static Dictionary
Link Menu
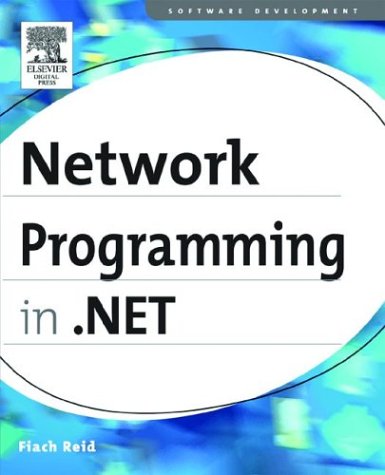
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SuppressMessageAttribute.cs
- GridSplitterAutomationPeer.cs
- RemotingConfigParser.cs
- RootBrowserWindowProxy.cs
- RelationshipEndMember.cs
- TextTreeRootNode.cs
- CodePropertyReferenceExpression.cs
- QueryStringParameter.cs
- GroupBoxAutomationPeer.cs
- EventWaitHandle.cs
- TcpHostedTransportConfiguration.cs
- RayMeshGeometry3DHitTestResult.cs
- QueryConverter.cs
- SoapAttributeAttribute.cs
- infer.cs
- GeneralTransform.cs
- LassoSelectionBehavior.cs
- DispatcherHookEventArgs.cs
- SqlPersonalizationProvider.cs
- precedingquery.cs
- DbConnectionPoolGroupProviderInfo.cs
- DataGridCellAutomationPeer.cs
- DynamicDocumentPaginator.cs
- RowBinding.cs
- BrowserCapabilitiesFactoryBase.cs
- SupportsEventValidationAttribute.cs
- UnsafeNativeMethodsTablet.cs
- SmtpDigestAuthenticationModule.cs
- CodeGroup.cs
- ListViewAutomationPeer.cs
- ResourceCategoryAttribute.cs
- ObjectQueryState.cs
- FocusManager.cs
- DictionaryBase.cs
- TopClause.cs
- ErrorsHelper.cs
- StrongNameUtility.cs
- Triplet.cs
- Size.cs
- HeaderElement.cs
- ReferenceEqualityComparer.cs
- AttributeCollection.cs
- CryptoStream.cs
- Point3DCollection.cs
- DescendentsWalker.cs
- JsonWriter.cs
- Int32AnimationBase.cs
- SmtpCommands.cs
- ExceptionUtil.cs
- compensatingcollection.cs
- XPathDocument.cs
- IndicFontClient.cs
- RowCache.cs
- XamlTemplateSerializer.cs
- ColumnCollectionEditor.cs
- loginstatus.cs
- HtmlTableCellCollection.cs
- Int32EqualityComparer.cs
- ScriptingRoleServiceSection.cs
- TcpTransportManager.cs
- FaultReason.cs
- BmpBitmapEncoder.cs
- TdsParserStaticMethods.cs
- TextDecorationCollectionConverter.cs
- PropertyRecord.cs
- FixedStringLookup.cs
- PropertyEmitterBase.cs
- XmlLanguage.cs
- SecurityHelper.cs
- Vector3DAnimationUsingKeyFrames.cs
- HandlerFactoryCache.cs
- ReflectionHelper.cs
- MarshalDirectiveException.cs
- DocumentPageView.cs
- ConfigPathUtility.cs
- BehaviorDragDropEventArgs.cs
- ArrayExtension.cs
- hwndwrapper.cs
- CheckBox.cs
- TextElementEnumerator.cs
- SQLBinaryStorage.cs
- FontFamilyConverter.cs
- AddInAttribute.cs
- QueueAccessMode.cs
- PropertySegmentSerializationProvider.cs
- RootBrowserWindowAutomationPeer.cs
- RootBrowserWindow.cs
- EdmItemError.cs
- KeyPressEvent.cs
- HostProtectionPermission.cs
- xsdvalidator.cs
- AuthStoreRoleProvider.cs
- LinkDescriptor.cs
- ControlCachePolicy.cs
- DictionarySectionHandler.cs
- TextServicesProperty.cs
- ComboBox.cs
- TypeGeneratedEventArgs.cs
- HttpRequest.cs
- CollectionViewGroupRoot.cs