Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / documents / HostedElements.cs / 1 / HostedElements.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: HostedElements.cs // // Enumerator class for returning descendants of TextBlock and FlowDocumentPage // // History: // 01/17/2005 : arathira - created. // //--------------------------------------------------------------------------- using System.Collections; using MS.Internal.Documents; using System.Collections.Generic; using System.Collections.ObjectModel; using System; using System.Diagnostics; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Documents { ////// Enumerator class for implementation of IContentHost on TextBlock and FlowDocumentPage. /// Used to iterate through descendants of the content host /// internal class HostedElements : IEnumerator{ //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors internal HostedElements(ReadOnlyCollection textSegments) { _textSegments = textSegments; _currentPosition = null; _currentTextSegment = 0; } #endregion Constructors //-------------------------------------------------------------------- // // IDisposable Methods // //------------------------------------------------------------------- #region IDisposable Methods void IDisposable.Dispose() { _textSegments = null; } #endregion IDisposable Methods //-------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods public bool MoveNext() { // If _textSegments has been disposed, throw exception if (_textSegments == null) { throw new ObjectDisposedException("HostedElements"); } if (_textSegments.Count == 0) return false; // Verify that current position matches with current text segment, and set it if it's null. if (_currentPosition == null) { // We should be at the start Debug.Assert(_currentTextSegment == 0); // Type check that we can create _currentPosition here if (_textSegments[0].Start is TextPointer) { _currentPosition = new TextPointer(_textSegments[0].Start as TextPointer); } else { // We cannot search, return false _currentPosition = null; return false; } } else if (_currentTextSegment < _textSegments.Count) { // We have not yet reached the end of the container. Assert that our position matches with // our current text segment Debug.Assert(((ITextPointer)_currentPosition).CompareTo(_textSegments[_currentTextSegment].Start) >= 0 && ((ITextPointer)_currentPosition).CompareTo(_textSegments[_currentTextSegment].End) < 0); // This means that we are in the middle of content, so the previous search would have returned // something. We want to now move beyond that position _currentPosition.MoveToNextContextPosition(LogicalDirection.Forward); } // Search strarting from the position in the current segment while (_currentTextSegment < _textSegments.Count) { Debug.Assert(((ITextPointer)_currentPosition).CompareTo(_textSegments[_currentTextSegment].Start) >= 0); while (((ITextPointer)_currentPosition).CompareTo(_textSegments[_currentTextSegment].End) < 0) { if (_currentPosition.GetPointerContext(LogicalDirection.Forward) == TextPointerContext.ElementStart || _currentPosition.GetPointerContext(LogicalDirection.Forward) == TextPointerContext.EmbeddedElement) { return true; } else { _currentPosition.MoveToNextContextPosition(LogicalDirection.Forward); } } // We have reached the end of a segement. Update counters _currentTextSegment++; if (_currentTextSegment < _textSegments.Count) { // Move to the next segment if (_textSegments[_currentTextSegment].Start is TextPointer) { _currentPosition = new TextPointer(_textSegments[_currentTextSegment].Start as TextPointer); } else { // This state is invalid, set to null and return false _currentPosition = null; return false; } } } // We have reached the end without finding an element. Return false. return false; } #endregion Private Methods //------------------------------------------------------------------- // // Private Properties // //-------------------------------------------------------------------- #region Private Properties object IEnumerator.Current { get { return this.Current; } } void IEnumerator.Reset() { throw new NotImplementedException(); } public IInputElement Current { get { // Disable PRESharp warning 6503: "Property get methods should not throw exceptions". // HostedElements must throw exception if Current property is incorrectly accessed if (_textSegments == null) { // Collection was modified #pragma warning suppress 6503 // IEnumerator.Current is documented to throw this exception throw new InvalidOperationException(SR.Get(SRID.EnumeratorCollectionDisposed)); } if (_currentPosition == null) { // Enumerator not started. Call MoveNext to see if we can move ahead #pragma warning suppress 6503 // IEnumerator.Current is documented to throw this exception throw new InvalidOperationException(SR.Get(SRID.EnumeratorNotStarted)); } IInputElement currentElement = null; switch (_currentPosition.GetPointerContext(LogicalDirection.Forward)) { case TextPointerContext.ElementStart: Debug.Assert(_currentPosition.GetAdjacentElementFromOuterPosition(LogicalDirection.Forward) is IInputElement); currentElement = _currentPosition.GetAdjacentElementFromOuterPosition(LogicalDirection.Forward); break; case TextPointerContext.EmbeddedElement: Debug.Assert(_currentPosition.GetAdjacentElement(LogicalDirection.Forward) is IInputElement); currentElement = (IInputElement)_currentPosition.GetAdjacentElement(LogicalDirection.Forward); break; default: // Throw exception because this function should only be called after MoveNext, and not // if MoveNext returns false Debug.Assert(false, "Invalid state in HostedElements.cs"); break; } return currentElement; } } #endregion Private Properties //------------------------------------------------------------------- // // Private Fields // //------------------------------------------------------------------- #region Private Fields ReadOnlyCollection _textSegments; TextPointer _currentPosition; int _currentTextSegment; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: HostedElements.cs // // Enumerator class for returning descendants of TextBlock and FlowDocumentPage // // History: // 01/17/2005 : arathira - created. // //--------------------------------------------------------------------------- using System.Collections; using MS.Internal.Documents; using System.Collections.Generic; using System.Collections.ObjectModel; using System; using System.Diagnostics; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Documents { /// /// Enumerator class for implementation of IContentHost on TextBlock and FlowDocumentPage. /// Used to iterate through descendants of the content host /// internal class HostedElements : IEnumerator{ //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors internal HostedElements(ReadOnlyCollection textSegments) { _textSegments = textSegments; _currentPosition = null; _currentTextSegment = 0; } #endregion Constructors //-------------------------------------------------------------------- // // IDisposable Methods // //------------------------------------------------------------------- #region IDisposable Methods void IDisposable.Dispose() { _textSegments = null; } #endregion IDisposable Methods //-------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods public bool MoveNext() { // If _textSegments has been disposed, throw exception if (_textSegments == null) { throw new ObjectDisposedException("HostedElements"); } if (_textSegments.Count == 0) return false; // Verify that current position matches with current text segment, and set it if it's null. if (_currentPosition == null) { // We should be at the start Debug.Assert(_currentTextSegment == 0); // Type check that we can create _currentPosition here if (_textSegments[0].Start is TextPointer) { _currentPosition = new TextPointer(_textSegments[0].Start as TextPointer); } else { // We cannot search, return false _currentPosition = null; return false; } } else if (_currentTextSegment < _textSegments.Count) { // We have not yet reached the end of the container. Assert that our position matches with // our current text segment Debug.Assert(((ITextPointer)_currentPosition).CompareTo(_textSegments[_currentTextSegment].Start) >= 0 && ((ITextPointer)_currentPosition).CompareTo(_textSegments[_currentTextSegment].End) < 0); // This means that we are in the middle of content, so the previous search would have returned // something. We want to now move beyond that position _currentPosition.MoveToNextContextPosition(LogicalDirection.Forward); } // Search strarting from the position in the current segment while (_currentTextSegment < _textSegments.Count) { Debug.Assert(((ITextPointer)_currentPosition).CompareTo(_textSegments[_currentTextSegment].Start) >= 0); while (((ITextPointer)_currentPosition).CompareTo(_textSegments[_currentTextSegment].End) < 0) { if (_currentPosition.GetPointerContext(LogicalDirection.Forward) == TextPointerContext.ElementStart || _currentPosition.GetPointerContext(LogicalDirection.Forward) == TextPointerContext.EmbeddedElement) { return true; } else { _currentPosition.MoveToNextContextPosition(LogicalDirection.Forward); } } // We have reached the end of a segement. Update counters _currentTextSegment++; if (_currentTextSegment < _textSegments.Count) { // Move to the next segment if (_textSegments[_currentTextSegment].Start is TextPointer) { _currentPosition = new TextPointer(_textSegments[_currentTextSegment].Start as TextPointer); } else { // This state is invalid, set to null and return false _currentPosition = null; return false; } } } // We have reached the end without finding an element. Return false. return false; } #endregion Private Methods //------------------------------------------------------------------- // // Private Properties // //-------------------------------------------------------------------- #region Private Properties object IEnumerator.Current { get { return this.Current; } } void IEnumerator.Reset() { throw new NotImplementedException(); } public IInputElement Current { get { // Disable PRESharp warning 6503: "Property get methods should not throw exceptions". // HostedElements must throw exception if Current property is incorrectly accessed if (_textSegments == null) { // Collection was modified #pragma warning suppress 6503 // IEnumerator.Current is documented to throw this exception throw new InvalidOperationException(SR.Get(SRID.EnumeratorCollectionDisposed)); } if (_currentPosition == null) { // Enumerator not started. Call MoveNext to see if we can move ahead #pragma warning suppress 6503 // IEnumerator.Current is documented to throw this exception throw new InvalidOperationException(SR.Get(SRID.EnumeratorNotStarted)); } IInputElement currentElement = null; switch (_currentPosition.GetPointerContext(LogicalDirection.Forward)) { case TextPointerContext.ElementStart: Debug.Assert(_currentPosition.GetAdjacentElementFromOuterPosition(LogicalDirection.Forward) is IInputElement); currentElement = _currentPosition.GetAdjacentElementFromOuterPosition(LogicalDirection.Forward); break; case TextPointerContext.EmbeddedElement: Debug.Assert(_currentPosition.GetAdjacentElement(LogicalDirection.Forward) is IInputElement); currentElement = (IInputElement)_currentPosition.GetAdjacentElement(LogicalDirection.Forward); break; default: // Throw exception because this function should only be called after MoveNext, and not // if MoveNext returns false Debug.Assert(false, "Invalid state in HostedElements.cs"); break; } return currentElement; } } #endregion Private Properties //------------------------------------------------------------------- // // Private Fields // //------------------------------------------------------------------- #region Private Fields ReadOnlyCollection _textSegments; TextPointer _currentPosition; int _currentTextSegment; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
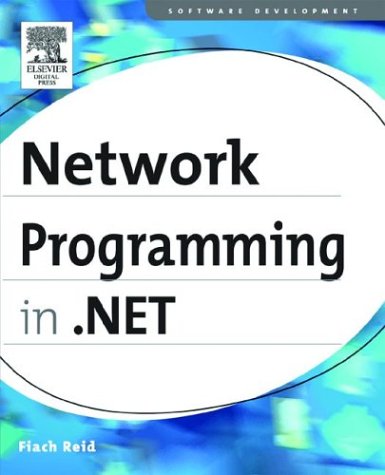
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Margins.cs
- CallbackValidator.cs
- SafeThemeHandle.cs
- NullNotAllowedCollection.cs
- IxmlLineInfo.cs
- CodeDirectoryCompiler.cs
- DelegatingConfigHost.cs
- SwitchLevelAttribute.cs
- CombinedTcpChannel.cs
- WebPartMovingEventArgs.cs
- WindowVisualStateTracker.cs
- SelectionHighlightInfo.cs
- SessionPageStatePersister.cs
- XamlReaderHelper.cs
- PropertyInformationCollection.cs
- CanonicalXml.cs
- DataControlFieldHeaderCell.cs
- UInt16Storage.cs
- ClientOptions.cs
- CursorConverter.cs
- SymmetricKey.cs
- ListenerServiceInstallComponent.cs
- ServiceCredentials.cs
- DynamicQueryableWrapper.cs
- SerializationInfo.cs
- ProgressBarBrushConverter.cs
- BackStopAuthenticationModule.cs
- EventProviderWriter.cs
- EntityDataSourceWrapper.cs
- LinkLabel.cs
- ReflectEventDescriptor.cs
- Triplet.cs
- XmlSchemaObjectCollection.cs
- PrintDialog.cs
- ThicknessAnimationUsingKeyFrames.cs
- XomlCompiler.cs
- ByteAnimationUsingKeyFrames.cs
- XmlIgnoreAttribute.cs
- DataGridViewRowConverter.cs
- WebPartDisplayModeCancelEventArgs.cs
- WebConfigurationHostFileChange.cs
- InputMethod.cs
- FontWeight.cs
- SyntaxCheck.cs
- ZoneIdentityPermission.cs
- SQLSingleStorage.cs
- NameValueCollection.cs
- CommonEndpointBehaviorElement.cs
- XsltException.cs
- _SSPIWrapper.cs
- SmtpException.cs
- Converter.cs
- DllNotFoundException.cs
- XmlNamespaceMapping.cs
- JsonWriter.cs
- VBIdentifierDesigner.xaml.cs
- SafeProcessHandle.cs
- InkCanvasSelectionAdorner.cs
- MdImport.cs
- WindowsRichEditRange.cs
- PreservationFileReader.cs
- ZipIOExtraFieldZip64Element.cs
- ImageIndexConverter.cs
- CustomAssemblyResolver.cs
- ToolStripDropDownDesigner.cs
- PersonalizationDictionary.cs
- FixedStringLookup.cs
- ResourceDescriptionAttribute.cs
- MatrixTransform.cs
- DataGridViewDataErrorEventArgs.cs
- Int32RectValueSerializer.cs
- ConvertersCollection.cs
- UpDownEvent.cs
- ListViewUpdatedEventArgs.cs
- _NegotiateClient.cs
- PathSegment.cs
- EntityProviderServices.cs
- CodeMethodInvokeExpression.cs
- InternalConfigHost.cs
- LineServicesRun.cs
- AnonymousIdentificationSection.cs
- KeysConverter.cs
- TextEditorTables.cs
- SqlServices.cs
- AppSettingsSection.cs
- EntityDataSourceSelectedEventArgs.cs
- WebContext.cs
- Fault.cs
- ListItemCollection.cs
- FileUtil.cs
- TabControl.cs
- XmlSchemaAttributeGroupRef.cs
- ActivityPreviewDesigner.cs
- InstancePersistence.cs
- TextRangeEdit.cs
- ToolStripRenderer.cs
- ReachUIElementCollectionSerializerAsync.cs
- MenuStrip.cs
- StylusPointPropertyId.cs
- XmlAutoDetectWriter.cs