Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Documents / TextEditorTables.cs / 1 / TextEditorTables.cs
//---------------------------------------------------------------------------- // // File: TextEditorTables.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: A Component of TextEditor supporting Table editing commands // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; using System.Globalization; using System.Threading; using System.ComponentModel; using System.Text; using System.Collections; // ArrayList using System.Runtime.InteropServices; using System.Windows.Threading; using System.Windows.Input; using System.Windows.Controls; // ScrollChangedEventArgs using System.Windows.Controls.Primitives; // CharacterCasing, TextBoxBase using System.Windows.Media; using System.Windows.Markup; using MS.Utility; using MS.Win32; using MS.Internal.Documents; using MS.Internal.Commands; // CommandHelpers ////// Text editing service for controls. /// internal static class TextEditorTables { //----------------------------------------------------- // // Class Internal Methods // //----------------------------------------------------- #region Class Internal Methods // Registers all text editing command handlers for a given control type internal static void _RegisterClassHandlers(Type controlType, bool registerEventListeners) { var onTableCommand = new ExecutedRoutedEventHandler(OnTableCommand); var onQueryStatusNYI = new CanExecuteRoutedEventHandler(OnQueryStatusNYI); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertTable , onTableCommand, onQueryStatusNYI, SRID.KeyInsertTable, SRID.KeyInsertTableDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertRows , onTableCommand, onQueryStatusNYI, SRID.KeyInsertRows, SRID.KeyInsertRowsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertColumns , onTableCommand, onQueryStatusNYI, SRID.KeyInsertColumns, SRID.KeyInsertColumnsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.DeleteRows , onTableCommand, onQueryStatusNYI, SRID.KeyDeleteRows, SRID.KeyDeleteRowsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.DeleteColumns , onTableCommand, onQueryStatusNYI, SRID.KeyDeleteColumns, SRID.KeyDeleteColumnsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.MergeCells , onTableCommand, onQueryStatusNYI, SRID.KeyMergeCells, SRID.KeyMergeCellsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.SplitCell , onTableCommand, onQueryStatusNYI, SRID.KeySplitCell, SRID.KeySplitCellDisplayString); } #endregion Class Internal Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods private static void OnTableCommand(object target, ExecutedRoutedEventArgs args) { TextEditor This = TextEditor._GetTextEditor(target); if (This == null || !This._IsEnabled || This.IsReadOnly || !This.AcceptsRichContent || !(This.Selection is TextSelection)) { return; } TextEditorTyping._FlushPendingInputItems(This); // Forget previously suggested horizontal position TextEditorSelection._ClearSuggestedX(This); // Execute the command if (args.Command == EditingCommands.InsertTable) { ((TextSelection)This.Selection).InsertTable(/*rowCount:*/4, /*columnCount:*/4); } else if (args.Command == EditingCommands.InsertRows) { ((TextSelection)This.Selection).InsertRows(+1); } else if (args.Command == EditingCommands.InsertColumns) { ((TextSelection)This.Selection).InsertColumns(+1); } else if (args.Command == EditingCommands.DeleteRows) { ((TextSelection)This.Selection).DeleteRows(); } else if (args.Command == EditingCommands.DeleteColumns) { ((TextSelection)This.Selection).DeleteColumns(); } else if (args.Command == EditingCommands.MergeCells) { ((TextSelection)This.Selection).MergeCells(); } else if (args.Command == EditingCommands.SplitCell) { ((TextSelection)This.Selection).SplitCell(1000, 1000); // Split all ways to possible maximum } } // ---------------------------------------------------------- // // Misceleneous Commands // // ---------------------------------------------------------- #region Misceleneous Commands ////// StartInputCorrection command QueryStatus handler /// private static void OnQueryStatusNYI(object target, CanExecuteRoutedEventArgs args) { TextEditor This = TextEditor._GetTextEditor(target); if (This == null) { return; } args.CanExecute = true; } #endregion Misceleneous Commands #endregion Private methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: TextEditorTables.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: A Component of TextEditor supporting Table editing commands // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; using System.Globalization; using System.Threading; using System.ComponentModel; using System.Text; using System.Collections; // ArrayList using System.Runtime.InteropServices; using System.Windows.Threading; using System.Windows.Input; using System.Windows.Controls; // ScrollChangedEventArgs using System.Windows.Controls.Primitives; // CharacterCasing, TextBoxBase using System.Windows.Media; using System.Windows.Markup; using MS.Utility; using MS.Win32; using MS.Internal.Documents; using MS.Internal.Commands; // CommandHelpers ////// Text editing service for controls. /// internal static class TextEditorTables { //----------------------------------------------------- // // Class Internal Methods // //----------------------------------------------------- #region Class Internal Methods // Registers all text editing command handlers for a given control type internal static void _RegisterClassHandlers(Type controlType, bool registerEventListeners) { var onTableCommand = new ExecutedRoutedEventHandler(OnTableCommand); var onQueryStatusNYI = new CanExecuteRoutedEventHandler(OnQueryStatusNYI); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertTable , onTableCommand, onQueryStatusNYI, SRID.KeyInsertTable, SRID.KeyInsertTableDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertRows , onTableCommand, onQueryStatusNYI, SRID.KeyInsertRows, SRID.KeyInsertRowsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertColumns , onTableCommand, onQueryStatusNYI, SRID.KeyInsertColumns, SRID.KeyInsertColumnsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.DeleteRows , onTableCommand, onQueryStatusNYI, SRID.KeyDeleteRows, SRID.KeyDeleteRowsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.DeleteColumns , onTableCommand, onQueryStatusNYI, SRID.KeyDeleteColumns, SRID.KeyDeleteColumnsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.MergeCells , onTableCommand, onQueryStatusNYI, SRID.KeyMergeCells, SRID.KeyMergeCellsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.SplitCell , onTableCommand, onQueryStatusNYI, SRID.KeySplitCell, SRID.KeySplitCellDisplayString); } #endregion Class Internal Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods private static void OnTableCommand(object target, ExecutedRoutedEventArgs args) { TextEditor This = TextEditor._GetTextEditor(target); if (This == null || !This._IsEnabled || This.IsReadOnly || !This.AcceptsRichContent || !(This.Selection is TextSelection)) { return; } TextEditorTyping._FlushPendingInputItems(This); // Forget previously suggested horizontal position TextEditorSelection._ClearSuggestedX(This); // Execute the command if (args.Command == EditingCommands.InsertTable) { ((TextSelection)This.Selection).InsertTable(/*rowCount:*/4, /*columnCount:*/4); } else if (args.Command == EditingCommands.InsertRows) { ((TextSelection)This.Selection).InsertRows(+1); } else if (args.Command == EditingCommands.InsertColumns) { ((TextSelection)This.Selection).InsertColumns(+1); } else if (args.Command == EditingCommands.DeleteRows) { ((TextSelection)This.Selection).DeleteRows(); } else if (args.Command == EditingCommands.DeleteColumns) { ((TextSelection)This.Selection).DeleteColumns(); } else if (args.Command == EditingCommands.MergeCells) { ((TextSelection)This.Selection).MergeCells(); } else if (args.Command == EditingCommands.SplitCell) { ((TextSelection)This.Selection).SplitCell(1000, 1000); // Split all ways to possible maximum } } // ---------------------------------------------------------- // // Misceleneous Commands // // ---------------------------------------------------------- #region Misceleneous Commands ////// StartInputCorrection command QueryStatus handler /// private static void OnQueryStatusNYI(object target, CanExecuteRoutedEventArgs args) { TextEditor This = TextEditor._GetTextEditor(target); if (This == null) { return; } args.CanExecute = true; } #endregion Misceleneous Commands #endregion Private methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
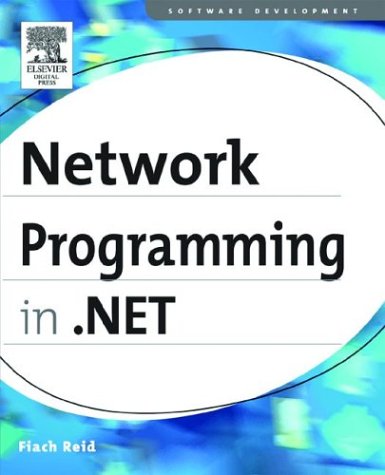
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FixedHyperLink.cs
- GridEntry.cs
- ExceptionUtil.cs
- InputScope.cs
- QuaternionIndependentAnimationStorage.cs
- CodeTypeReferenceExpression.cs
- FullTextBreakpoint.cs
- ScaleTransform3D.cs
- DeferredSelectedIndexReference.cs
- ScriptingJsonSerializationSection.cs
- SolidColorBrush.cs
- pingexception.cs
- Wildcard.cs
- HandleTable.cs
- XmlSchemaSimpleTypeRestriction.cs
- InheritanceContextChangedEventManager.cs
- ReadOnlyDataSourceView.cs
- Helpers.cs
- XsltSettings.cs
- XhtmlBasicLiteralTextAdapter.cs
- RowToParametersTransformer.cs
- WMIInterop.cs
- PackagePart.cs
- DateTimeFormatInfoScanner.cs
- RenderCapability.cs
- FixedSOMGroup.cs
- Regex.cs
- SapiInterop.cs
- ByteAnimation.cs
- SecurityCriticalDataForSet.cs
- UpdatePanel.cs
- EmbeddedObject.cs
- HttpMethodConstraint.cs
- DbMetaDataColumnNames.cs
- EntityCollection.cs
- GridViewItemAutomationPeer.cs
- ToolStripDropDown.cs
- Soap.cs
- LinkButton.cs
- Menu.cs
- StringFreezingAttribute.cs
- ExpressionConverter.cs
- AttachedPropertyInfo.cs
- SchemaElementLookUpTable.cs
- ModuleConfigurationInfo.cs
- StickyNoteHelper.cs
- FileDetails.cs
- MdiWindowListItemConverter.cs
- BinaryNode.cs
- SpellerStatusTable.cs
- RepeaterItemCollection.cs
- HebrewCalendar.cs
- _IPv6Address.cs
- AssertUtility.cs
- MenuItemCollection.cs
- Size3DValueSerializer.cs
- WindowHideOrCloseTracker.cs
- GestureRecognizer.cs
- CqlGenerator.cs
- PieceNameHelper.cs
- ListControlBuilder.cs
- ContentElementAutomationPeer.cs
- DefaultTraceListener.cs
- Compress.cs
- LoadGrammarCompletedEventArgs.cs
- ProjectedSlot.cs
- CacheEntry.cs
- ImmutableObjectAttribute.cs
- GridViewItemAutomationPeer.cs
- HttpConfigurationSystem.cs
- DocumentAutomationPeer.cs
- Int16.cs
- ExtractCollection.cs
- TargetControlTypeAttribute.cs
- PropertyCollection.cs
- EUCJPEncoding.cs
- AssociationSet.cs
- CodeNamespaceCollection.cs
- EditorZone.cs
- SignatureHelper.cs
- EdgeProfileValidation.cs
- StateBag.cs
- Semaphore.cs
- XmlSchemaProviderAttribute.cs
- SystemWebExtensionsSectionGroup.cs
- AudioFileOut.cs
- RadioButtonBaseAdapter.cs
- UnsafeNativeMethods.cs
- DragDropHelper.cs
- ProxyWebPart.cs
- SecuritySessionSecurityTokenAuthenticator.cs
- DataControlHelper.cs
- ChannelSinkStacks.cs
- PageAsyncTask.cs
- StringToken.cs
- PrtTicket_Base.cs
- ParseHttpDate.cs
- LayoutTableCell.cs
- _AutoWebProxyScriptWrapper.cs
- IdentifierCreationService.cs