Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Objects / ObjectParameterCollection.cs / 1 / ObjectParameterCollection.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupowner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Globalization; using System.Data; using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Objects.Internal; using System.Text; namespace System.Data.Objects { ////// This class represents a collection of query parameters at the object layer. /// public sealed class ObjectParameterCollection : ICollection{ // Note: There are NO public constructors for this class - it is for internal // ObjectQuery use only, but must be public so that an instance thereof can be // a public property on ObjectQuery . #region Internal Constructors // --------------------- // Internal Constructors // --------------------- #region ObjectParameterCollection (ClrPerspective) /// /// This internal constructor creates a new query parameter collection and /// initializes the internal parameter storage. /// internal ObjectParameterCollection(ClrPerspective perspective) { EntityUtil.CheckArgumentNull(perspective, "perspective"); // The perspective is required to do type-checking on parameters as they // are added to the collection. this._perspective = perspective; // Create a new list to store the parameters. _parameters = new List(); } #endregion #endregion #region Private Fields // -------------- // Private Fields // -------------- /// /// Can parameters be added or removed from this collection? /// private bool _locked; ////// The internal storage for the query parameters in the collection. /// private List_parameters; /// /// A CLR perspective necessary to do type-checking on parameters as they /// are added to the collection. /// private ClrPerspective _perspective; ////// A string that can be used to represent the current state of this parameter collection in an ObjectQuery cache key. /// private string _cacheKey; #endregion #region Public Properties // ----------------- // Public Properties // ----------------- ////// The number of parameters currently in the collection. /// public int Count { get { return this._parameters.Count; } } ////// This collection is read-write - parameters may be added, removed /// and [somewhat] modified at will (value only) - provided that the /// implementation the collection belongs to has not locked its parameters /// because it's command definition has been prepared. /// bool ICollection.IsReadOnly { get { return (this._locked); } } #endregion #region Public Indexers // --------------- // Public Indexers // --------------- /// /// This indexer allows callers to retrieve parameters by name. If no /// parameter by the given name exists, an exception is thrown. For /// safe existence-checking, use the Contains method instead. /// /// /// The name of the parameter to find. /// ////// The parameter object with the specified name. /// ////// If no parameter with the specified name is found in the collection. /// public ObjectParameter this[string name] { get { int index = this.IndexOf(name); if (index == -1) { throw EntityUtil.ArgumentOutOfRange(System.Data.Entity.Strings.ObjectParameterCollection_ParameterNameNotFound(name), "name"); } return this._parameters[index]; } } #endregion #region Public Methods // -------------- // Public Methods // -------------- #region Add ////// This method adds the specified parameter object to the collection. If /// the parameter object already exists in the collection, an exception is /// thrown. /// /// /// The parameter object to add to the collection. /// ////// /// If the value of the parameter argument is null. /// ////// If the parameter argument already exists in the collection. This /// behavior differs from that of most collections which allow duplicate /// entries. /// ////// If another parameter with the same name as the parameter argument /// already exists in the collection. Note that the lookup is case- /// insensitive. This behavior differs from that of most collections, /// and is more like that of a Dictionary. /// ////// If the type of the specified parameter is invalid. /// public void Add (ObjectParameter parameter) { EntityUtil.CheckArgumentNull(parameter, "parameter"); CheckUnlocked(); if (this.Contains(parameter)) { throw EntityUtil.Argument(System.Data.Entity.Strings.ObjectParameterCollection_ParameterAlreadyExists(parameter.Name), "parameter"); } if (this.Contains(parameter.Name)) { throw EntityUtil.Argument(System.Data.Entity.Strings.ObjectParameterCollection_DuplicateParameterName(parameter.Name), "parameter"); } if (!parameter.ValidateParameterType(this._perspective)) { throw EntityUtil.ArgumentOutOfRange(System.Data.Entity.Strings.ObjectParameter_InvalidParameterType(parameter.ParameterType.FullName), "parameter"); } this._parameters.Add(parameter); this._cacheKey = null; } #endregion #region Clear ////// This method empties the entire parameter collection. /// ///public void Clear() { CheckUnlocked(); this._parameters.Clear(); this._cacheKey = null; } #endregion #region Contains (ObjectParameter) /// /// This methods checks for the existence of a given parameter object in the /// collection by reference. /// /// /// The parameter object to look for in the collection. /// ////// True if the parameter object was found in the collection, false otherwise. /// Note that this is a reference-based lookup, which means that if the para- /// meter argument has the same name as a parameter object in the collection, /// this method will only return true if it's the same object. /// ////// If the value of the parameter argument is null. /// public bool Contains (ObjectParameter parameter) { EntityUtil.CheckArgumentNull(parameter, "parameter"); return this._parameters.Contains(parameter); } #endregion #region Contains (string) ////// This method checks for the existence of a given parameter in the collection /// by name. /// /// /// The name of the parameter to look for in the collection. /// ////// True if a parameter with the specified name was found in the collection, /// false otherwise. Note that the lookup is case-insensitive. /// ////// If the value of the parameter argument is null. /// public bool Contains (string name) { EntityUtil.CheckArgumentNull(name, "name"); if (this.IndexOf(name) != -1) { return true; } return false; } #endregion #region CopyTo ////// This method allows the parameters in the collection to be copied into a /// supplied array, beginning at the specified index therein. /// /// /// The array into which to copy the parameters. /// /// /// The index in the array at which to start copying the parameters. /// ///public void CopyTo (ObjectParameter[] array, int index) { this._parameters.CopyTo(array, index); } #endregion #region Remove /// /// This method removes an instance of a parameter from the collection by /// reference if it exists in the collection. To remove a parameter by name, /// first use the Contains(name) method or this[name] indexer to retrieve /// the parameter instance, then remove it using this method. /// /// /// The parameter object to remove from the collection. /// ////// True if the parameter object was found and removed from the collection, /// false otherwise. Note that this is a reference-based lookup, which means /// that if the parameter argument has the same name as a parameter object /// in the collection, this method will remove it only if it's the same object. /// ////// If the value of the parameter argument is null. /// public bool Remove (ObjectParameter parameter) { EntityUtil.CheckArgumentNull(parameter, "parameter"); CheckUnlocked(); bool removed = this._parameters.Remove(parameter); // If the specified parameter was found in the collection and removed, // clear out the cached string representation of this parameter collection // so that the next call to GetCacheKey (if any) will regenerate it based on // the new state of this collection. if (removed) { this._cacheKey = null; } return removed; } #endregion #region GetEnumerator ////// These methods return enumerator instances, which allow the collection to /// be iterated through and traversed. /// IEnumeratorIEnumerable .GetEnumerator() { return ((System.Collections.Generic.ICollection )this._parameters).GetEnumerator(); } IEnumerator IEnumerable.GetEnumerator() { return ((System.Collections.ICollection)this._parameters).GetEnumerator(); } #endregion #endregion #region Internal Methods // --------------- // Internal Methods // --------------- /// /// Retrieves a string that may be used to represent this parameter collection in an ObjectQuery cache key. /// If this collection has not changed since the last call to this method, the same string instance is returned. /// Note that this string is used by various ObjectQueryImplementations to version the parameter collection. /// ///A string that may be used to represent this parameter collection in an ObjectQuery cache key. internal string GetCacheKey() { if (null == this._cacheKey) { if(this._parameters.Count > 0) { // Future Enhancement: If the separate branch for a single parameter does not have a measurable perf advantage, remove it. if (1 == this._parameters.Count) { // if its one parameter only, there is no need to use stringbuilder ObjectParameter theParam = this._parameters[0]; this._cacheKey = "@@1" + theParam.Name + ":" + theParam.ParameterType.FullName; } else { // Future Enhancement: Investigate whether precalculating the required size of the string builder is a better time/space tradeoff. StringBuilder keyBuilder = new StringBuilder(this._parameters.Count * 20); keyBuilder.Append("@@"); keyBuilder.Append(this._parameters.Count); for (int idx = 0; idx < this._parameters.Count; idx++) { // // if (idx > 0) { keyBuilder.Append(";"); } ObjectParameter thisParam = this._parameters[idx]; keyBuilder.Append(thisParam.Name); keyBuilder.Append(":"); keyBuilder.Append(thisParam.ParameterType.FullName); } this._cacheKey = keyBuilder.ToString(); } } } return this._cacheKey; } ////// Locks or unlocks this parameter collection, allowing its contents to be added to, removed from, or cleared. /// Calling this method consecutively with the same value has no effect but does not throw an exception. /// /// Iftrue , this parameter collection is now locked; otherwise it is unlocked internal void SetReadOnly(bool isReadOnly) { this._locked = isReadOnly; } ////// Creates a new copy of the specified parameter collection containing copies of its element /// The parameter collection to copy ///s. /// If the specified argument is null , thennull is returned. ///The new collection containing copies of internal static ObjectParameterCollection DeepCopy(ObjectParameterCollection copyParams) { if (null == copyParams) { return null; } ObjectParameterCollection retParams = new ObjectParameterCollection(copyParams._perspective); foreach (ObjectParameter param in copyParams) { retParams.Add(param.ShallowCopy()); } return retParams; } #endregion #region Private Methods // --------------- // Private Methods // --------------- ///parameters, if is non-null; otherwise null ./// This private method checks for the existence of a given parameter object /// by name by iterating through the list and comparing each parameter name /// to the specified name. This is a case-insensitive lookup. /// private int IndexOf (string name) { int index = 0; foreach (ObjectParameter parameter in this._parameters) { if (0 == String.Compare(name, parameter.Name, StringComparison.OrdinalIgnoreCase)) { return index; } index++; } return -1; } ////// This method successfully returns only if the parameter collection is not considered 'locked'; /// otherwise an private void CheckUnlocked() { if (this._locked) { throw EntityUtil.InvalidOperation(Entity.Strings.ObjectParameterCollection_ParametersLocked); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //is thrown. /// // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupowner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Globalization; using System.Data; using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Objects.Internal; using System.Text; namespace System.Data.Objects { ////// This class represents a collection of query parameters at the object layer. /// public sealed class ObjectParameterCollection : ICollection{ // Note: There are NO public constructors for this class - it is for internal // ObjectQuery use only, but must be public so that an instance thereof can be // a public property on ObjectQuery . #region Internal Constructors // --------------------- // Internal Constructors // --------------------- #region ObjectParameterCollection (ClrPerspective) /// /// This internal constructor creates a new query parameter collection and /// initializes the internal parameter storage. /// internal ObjectParameterCollection(ClrPerspective perspective) { EntityUtil.CheckArgumentNull(perspective, "perspective"); // The perspective is required to do type-checking on parameters as they // are added to the collection. this._perspective = perspective; // Create a new list to store the parameters. _parameters = new List(); } #endregion #endregion #region Private Fields // -------------- // Private Fields // -------------- /// /// Can parameters be added or removed from this collection? /// private bool _locked; ////// The internal storage for the query parameters in the collection. /// private List_parameters; /// /// A CLR perspective necessary to do type-checking on parameters as they /// are added to the collection. /// private ClrPerspective _perspective; ////// A string that can be used to represent the current state of this parameter collection in an ObjectQuery cache key. /// private string _cacheKey; #endregion #region Public Properties // ----------------- // Public Properties // ----------------- ////// The number of parameters currently in the collection. /// public int Count { get { return this._parameters.Count; } } ////// This collection is read-write - parameters may be added, removed /// and [somewhat] modified at will (value only) - provided that the /// implementation the collection belongs to has not locked its parameters /// because it's command definition has been prepared. /// bool ICollection.IsReadOnly { get { return (this._locked); } } #endregion #region Public Indexers // --------------- // Public Indexers // --------------- /// /// This indexer allows callers to retrieve parameters by name. If no /// parameter by the given name exists, an exception is thrown. For /// safe existence-checking, use the Contains method instead. /// /// /// The name of the parameter to find. /// ////// The parameter object with the specified name. /// ////// If no parameter with the specified name is found in the collection. /// public ObjectParameter this[string name] { get { int index = this.IndexOf(name); if (index == -1) { throw EntityUtil.ArgumentOutOfRange(System.Data.Entity.Strings.ObjectParameterCollection_ParameterNameNotFound(name), "name"); } return this._parameters[index]; } } #endregion #region Public Methods // -------------- // Public Methods // -------------- #region Add ////// This method adds the specified parameter object to the collection. If /// the parameter object already exists in the collection, an exception is /// thrown. /// /// /// The parameter object to add to the collection. /// ////// /// If the value of the parameter argument is null. /// ////// If the parameter argument already exists in the collection. This /// behavior differs from that of most collections which allow duplicate /// entries. /// ////// If another parameter with the same name as the parameter argument /// already exists in the collection. Note that the lookup is case- /// insensitive. This behavior differs from that of most collections, /// and is more like that of a Dictionary. /// ////// If the type of the specified parameter is invalid. /// public void Add (ObjectParameter parameter) { EntityUtil.CheckArgumentNull(parameter, "parameter"); CheckUnlocked(); if (this.Contains(parameter)) { throw EntityUtil.Argument(System.Data.Entity.Strings.ObjectParameterCollection_ParameterAlreadyExists(parameter.Name), "parameter"); } if (this.Contains(parameter.Name)) { throw EntityUtil.Argument(System.Data.Entity.Strings.ObjectParameterCollection_DuplicateParameterName(parameter.Name), "parameter"); } if (!parameter.ValidateParameterType(this._perspective)) { throw EntityUtil.ArgumentOutOfRange(System.Data.Entity.Strings.ObjectParameter_InvalidParameterType(parameter.ParameterType.FullName), "parameter"); } this._parameters.Add(parameter); this._cacheKey = null; } #endregion #region Clear ////// This method empties the entire parameter collection. /// ///public void Clear() { CheckUnlocked(); this._parameters.Clear(); this._cacheKey = null; } #endregion #region Contains (ObjectParameter) /// /// This methods checks for the existence of a given parameter object in the /// collection by reference. /// /// /// The parameter object to look for in the collection. /// ////// True if the parameter object was found in the collection, false otherwise. /// Note that this is a reference-based lookup, which means that if the para- /// meter argument has the same name as a parameter object in the collection, /// this method will only return true if it's the same object. /// ////// If the value of the parameter argument is null. /// public bool Contains (ObjectParameter parameter) { EntityUtil.CheckArgumentNull(parameter, "parameter"); return this._parameters.Contains(parameter); } #endregion #region Contains (string) ////// This method checks for the existence of a given parameter in the collection /// by name. /// /// /// The name of the parameter to look for in the collection. /// ////// True if a parameter with the specified name was found in the collection, /// false otherwise. Note that the lookup is case-insensitive. /// ////// If the value of the parameter argument is null. /// public bool Contains (string name) { EntityUtil.CheckArgumentNull(name, "name"); if (this.IndexOf(name) != -1) { return true; } return false; } #endregion #region CopyTo ////// This method allows the parameters in the collection to be copied into a /// supplied array, beginning at the specified index therein. /// /// /// The array into which to copy the parameters. /// /// /// The index in the array at which to start copying the parameters. /// ///public void CopyTo (ObjectParameter[] array, int index) { this._parameters.CopyTo(array, index); } #endregion #region Remove /// /// This method removes an instance of a parameter from the collection by /// reference if it exists in the collection. To remove a parameter by name, /// first use the Contains(name) method or this[name] indexer to retrieve /// the parameter instance, then remove it using this method. /// /// /// The parameter object to remove from the collection. /// ////// True if the parameter object was found and removed from the collection, /// false otherwise. Note that this is a reference-based lookup, which means /// that if the parameter argument has the same name as a parameter object /// in the collection, this method will remove it only if it's the same object. /// ////// If the value of the parameter argument is null. /// public bool Remove (ObjectParameter parameter) { EntityUtil.CheckArgumentNull(parameter, "parameter"); CheckUnlocked(); bool removed = this._parameters.Remove(parameter); // If the specified parameter was found in the collection and removed, // clear out the cached string representation of this parameter collection // so that the next call to GetCacheKey (if any) will regenerate it based on // the new state of this collection. if (removed) { this._cacheKey = null; } return removed; } #endregion #region GetEnumerator ////// These methods return enumerator instances, which allow the collection to /// be iterated through and traversed. /// IEnumeratorIEnumerable .GetEnumerator() { return ((System.Collections.Generic.ICollection )this._parameters).GetEnumerator(); } IEnumerator IEnumerable.GetEnumerator() { return ((System.Collections.ICollection)this._parameters).GetEnumerator(); } #endregion #endregion #region Internal Methods // --------------- // Internal Methods // --------------- /// /// Retrieves a string that may be used to represent this parameter collection in an ObjectQuery cache key. /// If this collection has not changed since the last call to this method, the same string instance is returned. /// Note that this string is used by various ObjectQueryImplementations to version the parameter collection. /// ///A string that may be used to represent this parameter collection in an ObjectQuery cache key. internal string GetCacheKey() { if (null == this._cacheKey) { if(this._parameters.Count > 0) { // Future Enhancement: If the separate branch for a single parameter does not have a measurable perf advantage, remove it. if (1 == this._parameters.Count) { // if its one parameter only, there is no need to use stringbuilder ObjectParameter theParam = this._parameters[0]; this._cacheKey = "@@1" + theParam.Name + ":" + theParam.ParameterType.FullName; } else { // Future Enhancement: Investigate whether precalculating the required size of the string builder is a better time/space tradeoff. StringBuilder keyBuilder = new StringBuilder(this._parameters.Count * 20); keyBuilder.Append("@@"); keyBuilder.Append(this._parameters.Count); for (int idx = 0; idx < this._parameters.Count; idx++) { // // if (idx > 0) { keyBuilder.Append(";"); } ObjectParameter thisParam = this._parameters[idx]; keyBuilder.Append(thisParam.Name); keyBuilder.Append(":"); keyBuilder.Append(thisParam.ParameterType.FullName); } this._cacheKey = keyBuilder.ToString(); } } } return this._cacheKey; } ////// Locks or unlocks this parameter collection, allowing its contents to be added to, removed from, or cleared. /// Calling this method consecutively with the same value has no effect but does not throw an exception. /// /// Iftrue , this parameter collection is now locked; otherwise it is unlocked internal void SetReadOnly(bool isReadOnly) { this._locked = isReadOnly; } ////// Creates a new copy of the specified parameter collection containing copies of its element /// The parameter collection to copy ///s. /// If the specified argument is null , thennull is returned. ///The new collection containing copies of internal static ObjectParameterCollection DeepCopy(ObjectParameterCollection copyParams) { if (null == copyParams) { return null; } ObjectParameterCollection retParams = new ObjectParameterCollection(copyParams._perspective); foreach (ObjectParameter param in copyParams) { retParams.Add(param.ShallowCopy()); } return retParams; } #endregion #region Private Methods // --------------- // Private Methods // --------------- ///parameters, if is non-null; otherwise null ./// This private method checks for the existence of a given parameter object /// by name by iterating through the list and comparing each parameter name /// to the specified name. This is a case-insensitive lookup. /// private int IndexOf (string name) { int index = 0; foreach (ObjectParameter parameter in this._parameters) { if (0 == String.Compare(name, parameter.Name, StringComparison.OrdinalIgnoreCase)) { return index; } index++; } return -1; } ////// This method successfully returns only if the parameter collection is not considered 'locked'; /// otherwise an private void CheckUnlocked() { if (this._locked) { throw EntityUtil.InvalidOperation(Entity.Strings.ObjectParameterCollection_ParametersLocked); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.is thrown. ///
Link Menu
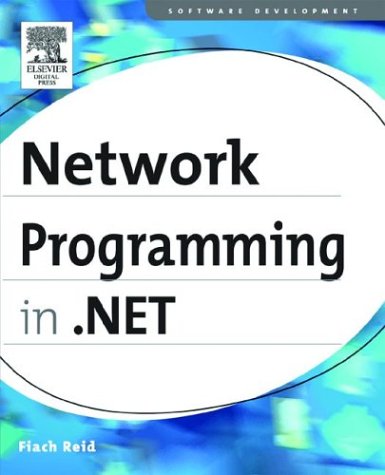
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IIS7ConfigurationLoader.cs
- ComponentResourceKey.cs
- PrimitiveSchema.cs
- TypeSource.cs
- SimpleBitVector32.cs
- BitmapEffectInputConnector.cs
- MbpInfo.cs
- IndexerNameAttribute.cs
- TextSelectionHelper.cs
- SystemInfo.cs
- CqlLexer.cs
- BrowserDefinition.cs
- SafeEventLogWriteHandle.cs
- Visual3D.cs
- TextElementEditingBehaviorAttribute.cs
- TreeViewCancelEvent.cs
- XmlSchemaSimpleTypeRestriction.cs
- LocalizabilityAttribute.cs
- SoapFormatExtensions.cs
- CompilerError.cs
- AuthorizationSection.cs
- ClientSettingsStore.cs
- NullExtension.cs
- AppDomainAttributes.cs
- MemberAccessException.cs
- SqlDelegatedTransaction.cs
- EntityDataSourceReferenceGroup.cs
- DocumentCollection.cs
- FrameSecurityDescriptor.cs
- FontStyles.cs
- XsltQilFactory.cs
- OracleCommandBuilder.cs
- CodePropertyReferenceExpression.cs
- XsltException.cs
- PolygonHotSpot.cs
- RoleGroupCollection.cs
- TypeEnumerableViewSchema.cs
- ConfigXmlAttribute.cs
- DateTimeStorage.cs
- Annotation.cs
- TextElementCollectionHelper.cs
- MultiAsyncResult.cs
- CodeRegionDirective.cs
- TextRangeEditLists.cs
- SpnEndpointIdentityExtension.cs
- DocumentOutline.cs
- EndpointDesigner.cs
- ToolStripArrowRenderEventArgs.cs
- ButtonStandardAdapter.cs
- DesignerTextViewAdapter.cs
- DataKey.cs
- GridViewDeleteEventArgs.cs
- DataGridLength.cs
- PersonalizationEntry.cs
- RecognizeCompletedEventArgs.cs
- DllNotFoundException.cs
- NamedPermissionSet.cs
- PropertyIdentifier.cs
- AutomationPatternInfo.cs
- HwndStylusInputProvider.cs
- TargetInvocationException.cs
- ICollection.cs
- DropShadowBitmapEffect.cs
- XpsFontSerializationService.cs
- AndMessageFilterTable.cs
- HttpWebRequestElement.cs
- GridEntryCollection.cs
- PopupRootAutomationPeer.cs
- ParallelTimeline.cs
- HtmlUtf8RawTextWriter.cs
- PageTheme.cs
- BinaryMessageEncodingBindingElement.cs
- ModelItemDictionary.cs
- FocusTracker.cs
- MasterPageCodeDomTreeGenerator.cs
- TimeSpanOrInfiniteConverter.cs
- ListViewItemSelectionChangedEvent.cs
- ServerProtocol.cs
- DisposableCollectionWrapper.cs
- WebPartConnectionsCancelVerb.cs
- CatalogZoneBase.cs
- BindingValueChangedEventArgs.cs
- RoleExceptions.cs
- MeasureData.cs
- MsmqNonTransactedPoisonHandler.cs
- XmlQueryRuntime.cs
- ToggleProviderWrapper.cs
- QuaternionConverter.cs
- BasePropertyDescriptor.cs
- SqlTransaction.cs
- ToolStripItem.cs
- PanelStyle.cs
- TableSectionStyle.cs
- ChangePasswordAutoFormat.cs
- CompositeTypefaceMetrics.cs
- SimpleTableProvider.cs
- ContractBase.cs
- PrinterResolution.cs
- CharAnimationUsingKeyFrames.cs
- DeviceContext.cs