Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Documents / DocumentStructures / SemanticBasicElement.cs / 1 / SemanticBasicElement.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // History: // 05/12/2005 : [....] - created. // // //--------------------------------------------------------------------------- using MS.Internal.Documents; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Reflection; using System.Security.Permissions; using System.Windows.Controls.Primitives; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Shapes; using System.Windows.Markup; [assembly: XmlnsDefinition( "http://schemas.microsoft.com/xps/2005/06/documentstructure", "System.Windows.Documents.DocumentStructures")] namespace System.Windows.Documents.DocumentStructures { ////// /// public class SemanticBasicElement : BlockElement { ////// /// internal SemanticBasicElement() { _elementList = new List(); } internal List BlockElementList { get { return _elementList; } } /// /// /// internal List_elementList; } /// /// /// public class SectionStructure : SemanticBasicElement, IAddChildInternal { ////// /// public SectionStructure() { _elementType = FixedElement.ElementType.Section; } void IAddChild.AddChild(object value) { if (value is ParagraphStructure || value is FigureStructure || value is ListStructure || value is TableStructure ) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType4, value.GetType(), typeof(ParagraphStructure), typeof(FigureStructure), typeof(ListStructure), typeof(TableStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class ParagraphStructure : SemanticBasicElement, IAddChildInternal { ////// /// public ParagraphStructure() { _elementType = FixedElement.ElementType.Paragraph; } void IAddChild.AddChild(object value) { if (value is NamedElement) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType1, value.GetType(), typeof(NamedElement)), "value"); } void IAddChild.AddText(string text) { } } //We are keeping these classes around for V.Next #if DEBUG ////// /// internal class Inline : SemanticBasicElement { ////// /// public Inline() { _elementType = FixedElement.ElementType.Inline; } } ////// /// internal class Span : Inline, IAddChild { ////// /// public Span() { _elementType = FixedElement.ElementType.Span; } void IAddChild.AddChild(object value) { if (value is Inline || value is NamedElement) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType2, value.GetType(), typeof(ParagraphStructure), typeof(NamedElement)), "value"); } void IAddChild.AddText(string text) { //throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, typeof(string)), "text"); } } ////// /// internal class Run : Inline, IAddChild { ////// /// public Run() { _elementType = FixedElement.ElementType.Run; } void IAddChild.AddChild(object value) { throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType()), "value"); } void IAddChild.AddText(string text) { } } #endif ////// /// public class FigureStructure : SemanticBasicElement, IAddChildInternal { ////// /// public FigureStructure() { _elementType = FixedElement.ElementType.Figure; } void IAddChild.AddChild(object value) { if (value is NamedElement) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(NamedElement)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class ListStructure : SemanticBasicElement, IAddChildInternal { ////// /// public ListStructure() { _elementType = FixedElement.ElementType.List; } void IAddChild.AddChild(object value) { if (value is ListItemStructure) { _elementList.Add((ListItemStructure)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(ListItemStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class ListItemStructure : SemanticBasicElement, IAddChildInternal { ////// /// public ListItemStructure() { _elementType = FixedElement.ElementType.ListItem; } void IAddChild.AddChild(object value) { if (value is ParagraphStructure || value is TableStructure || value is ListStructure || value is FigureStructure) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType4, value.GetType(), typeof(ParagraphStructure), typeof(TableStructure), typeof(ListStructure), typeof(FigureStructure)), "value"); } void IAddChild.AddText(string text) { } ////// /// public String Marker { get { return _markerName; } set { _markerName = value; } } private String _markerName; } ////// /// public class TableStructure : SemanticBasicElement, IAddChildInternal { ////// /// public TableStructure() { _elementType = FixedElement.ElementType.Table; } void IAddChild.AddChild(object value) { if (value is TableRowGroupStructure) { _elementList.Add((TableRowGroupStructure)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(TableRowGroupStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class TableRowGroupStructure : SemanticBasicElement, IAddChildInternal { ////// /// public TableRowGroupStructure() { _elementType = FixedElement.ElementType.TableRowGroup; } void IAddChild.AddChild(object value) { if (value is TableRowStructure) { _elementList.Add((TableRowStructure)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(TableRowStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class TableRowStructure : SemanticBasicElement, IAddChildInternal { ////// /// public TableRowStructure() { _elementType = FixedElement.ElementType.TableRow; } void IAddChild.AddChild(object value) { if (value is TableCellStructure) { _elementList.Add((TableCellStructure)value); return; } throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(TableCellStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// /// public class TableCellStructure : SemanticBasicElement, IAddChildInternal { ////// /// public TableCellStructure() { _elementType = FixedElement.ElementType.TableCell; _rowSpan = 1; _columnSpan = 1; } void IAddChild.AddChild(object value) { if (value is ParagraphStructure || value is TableStructure || value is ListStructure || value is FigureStructure) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType4, value.GetType(), typeof(ParagraphStructure), typeof(TableStructure), typeof(ListStructure), typeof(FigureStructure)), "value"); } void IAddChild.AddText(string text) { } ////// /// public int RowSpan { get { return _rowSpan; } set {_rowSpan = value; } } ////// /// public int ColumnSpan { get { return _columnSpan; } set {_columnSpan = value; } } private int _rowSpan; private int _columnSpan; } //We are keeping these classes for V.Next #if DEBUG ////// Header will not participate in the selection fow. /// internal class Header : SemanticBasicElement, IAddChild { ////// /// public Header() { _elementType = FixedElement.ElementType.Header; } void IAddChild.AddChild(object value) { if (value is NamedElement || value is ParagraphStructure) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType2, value.GetType(), typeof(NamedElement), typeof(ParagraphStructure)), "value"); } void IAddChild.AddText(string text) { } } ////// Footer will not participate in the selection fow. /// internal class Footer : SemanticBasicElement, IAddChild { ////// /// public Footer() { _elementType = FixedElement.ElementType.Footer; } void IAddChild.AddChild(object value) { if (value is NamedElement || value is ParagraphStructure) { _elementList.Add((BlockElement)value); return; } throw new ArgumentException(SR.Get(SRID.DocumentStructureUnexpectedParameterType2, value.GetType(), typeof(NamedElement), typeof(ParagraphStructure)), "value"); } void IAddChild.AddText(string text) { } } #endif } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
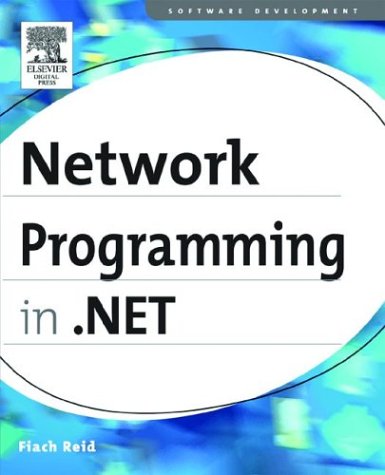
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Queue.cs
- SectionInput.cs
- FixUpCollection.cs
- UiaCoreApi.cs
- XsdBuilder.cs
- UserNamePasswordValidationMode.cs
- MutexSecurity.cs
- DesignerVerbCollection.cs
- ObjectToIdCache.cs
- MimeTypeAttribute.cs
- CalendarDesigner.cs
- FileDetails.cs
- DataGridViewBand.cs
- MenuAutomationPeer.cs
- WaitForChangedResult.cs
- BoundColumn.cs
- XmlSchemaObjectCollection.cs
- InternalSafeNativeMethods.cs
- uribuilder.cs
- HttpHandlerAction.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- itemelement.cs
- AvTraceFormat.cs
- WpfWebRequestHelper.cs
- RTTypeWrapper.cs
- RankException.cs
- FlowPosition.cs
- BackStopAuthenticationModule.cs
- EllipseGeometry.cs
- HttpUnhandledOperationInvoker.cs
- ProcessRequestArgs.cs
- UserControlAutomationPeer.cs
- DrawingCollection.cs
- PanelStyle.cs
- ServiceHostFactory.cs
- ParameterBuilder.cs
- BitmapCacheBrush.cs
- SecUtil.cs
- TryCatchDesigner.xaml.cs
- XsdCachingReader.cs
- EventLogTraceListener.cs
- IList.cs
- XmlSortKeyAccumulator.cs
- RoleGroup.cs
- ContentOperations.cs
- GeneratedContractType.cs
- ProcessInfo.cs
- HtmlControl.cs
- DocumentStatusResources.cs
- LinkTarget.cs
- WSHttpBindingBaseElement.cs
- Label.cs
- RegistrySecurity.cs
- TextCharacters.cs
- TypeResolver.cs
- ConfigurationSection.cs
- JulianCalendar.cs
- BuildProvider.cs
- SafeNativeMethods.cs
- MessageRpc.cs
- StylusPoint.cs
- MobileUITypeEditor.cs
- SerializeAbsoluteContext.cs
- TypeLoader.cs
- SessionPageStatePersister.cs
- Control.cs
- SqlParameter.cs
- Executor.cs
- VideoDrawing.cs
- MetadataUtilsSmi.cs
- ReferentialConstraint.cs
- SystemWebCachingSectionGroup.cs
- DataGridViewTopRowAccessibleObject.cs
- AppDomainFactory.cs
- DataPagerCommandEventArgs.cs
- PointHitTestResult.cs
- SaveFileDialog.cs
- Dispatcher.cs
- VirtualizingPanel.cs
- UnknownBitmapEncoder.cs
- CodeNamespaceCollection.cs
- ManagedWndProcTracker.cs
- CrossSiteScriptingValidation.cs
- TextTreeObjectNode.cs
- DisplayMemberTemplateSelector.cs
- TabControl.cs
- TextServicesManager.cs
- WebEventTraceProvider.cs
- CriticalFinalizerObject.cs
- DirectoryInfo.cs
- QuaternionConverter.cs
- PageParser.cs
- XamlFigureLengthSerializer.cs
- SingleBodyParameterMessageFormatter.cs
- SettingsSavedEventArgs.cs
- ActionFrame.cs
- NonParentingControl.cs
- BinaryMessageFormatter.cs
- PixelFormatConverter.cs
- MediaPlayerState.cs