Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Collections / Generic / IList.cs / 1305376 / IList.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Interface: IList ** **[....] ** ** ** Purpose: Base interface for all generic lists. ** ** ===========================================================*/ namespace System.Collections.Generic { using System; using System.Collections; using System.Runtime.CompilerServices; using System.Diagnostics.Contracts; // An IList is an ordered collection of objects. The exact ordering // is up to the implementation of the list, ranging from a sorted // order to insertion order. // Note that T[] : IList, and we want to ensure that if you use // IList , we ensure a YourValueType[] can be used // without jitting. Hence the TypeDependencyAttribute on SZArrayHelper. // This is a special hack internally though - see VM\compile.cpp. // The same attribute is on IEnumerable and ICollection . [TypeDependencyAttribute("System.SZArrayHelper")] [ContractClass(typeof(IListContract<>))] public interface IList : ICollection { // The Item property provides methods to read and edit entries in the List. T this[int index] { get; set; } // Returns the index of a particular item, if it is in the list. // Returns -1 if the item isn't in the list. int IndexOf(T item); // Inserts value into the list at position index. // index must be non-negative and less than or equal to the // number of elements in the list. If index equals the number // of items in the list, then value is appended to the end. void Insert(int index, T item); // Removes the item at position index. void RemoveAt(int index); } [ContractClassFor(typeof(IList<>))] internal abstract class IListContract : IList { T IList .this[int index] { get { //Contract.Requires(index >= 0); //Contract.Requires(index < ((ICollection )this).Count); return default(T); } set { //Contract.Requires(index >= 0); //Contract.Requires(index < ((ICollection )this).Count); } } IEnumerator System.Collections.IEnumerable.GetEnumerator() { Contract.Ensures(Contract.Result () != null); return default(IEnumerator); } IEnumerator IEnumerable .GetEnumerator() { Contract.Ensures(Contract.Result >() != null); return default(IEnumerator ); } [Pure] int IList .IndexOf(T value) { Contract.Ensures(Contract.Result () >= -1); Contract.Ensures(Contract.Result () < ((ICollection )this).Count); return default(int); } void IList .Insert(int index, T value) { //Contract.Requires(index >= 0); //Contract.Requires(index <= ((ICollection )this).Count); // For inserting immediately after the end. //Contract.Ensures(((ICollection )this).Count == Contract.OldValue(((ICollection )this).Count) + 1); // Not threadsafe } void IList .RemoveAt(int index) { //Contract.Requires(index >= 0); //Contract.Requires(index < ((ICollection )this).Count); //Contract.Ensures(((ICollection )this).Count == Contract.OldValue(((ICollection )this).Count) - 1); // Not threadsafe } #region ICollection Members void ICollection .Add(T value) { //Contract.Ensures(((ICollection )this).Count == Contract.OldValue(((ICollection )this).Count) + 1); // Not threadsafe } bool ICollection .IsReadOnly { get { return default(bool); } } int ICollection .Count { get { Contract.Ensures(Contract.Result () >= 0); return default(int); } } void ICollection .Clear() { // For fixed-sized collections like arrays, Clear will not change the Count property. // But we can't express that in a contract because we have no IsFixedSize property on // our generic collection interfaces. } bool ICollection .Contains(T value) { return default(bool); } void ICollection .CopyTo(T[] array, int startIndex) { //Contract.Requires(array != null); //Contract.Requires(startIndex >= 0); //Contract.Requires(startIndex + ((ICollection )this).Count <= array.Length); } bool ICollection .Remove(T value) { // No information if removal fails. return default(bool); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
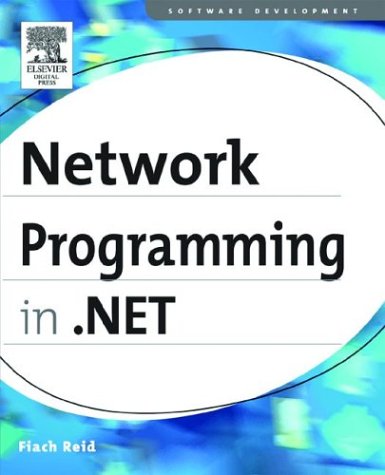
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebConfigurationFileMap.cs
- FixedSOMSemanticBox.cs
- SingleResultAttribute.cs
- InstanceData.cs
- DataError.cs
- WinInetCache.cs
- PanelDesigner.cs
- GcSettings.cs
- HttpHandlerActionCollection.cs
- ButtonBaseAutomationPeer.cs
- ActivationArguments.cs
- SchemaObjectWriter.cs
- _HTTPDateParse.cs
- DeviceSpecific.cs
- MenuBindingsEditorForm.cs
- TableChangeProcessor.cs
- XmlUtf8RawTextWriter.cs
- WinFormsSpinner.cs
- MenuEventArgs.cs
- SynchronizationLockException.cs
- AQNBuilder.cs
- QueryOptionExpression.cs
- TableChangeProcessor.cs
- Queue.cs
- FeatureSupport.cs
- SingleResultAttribute.cs
- _Win32.cs
- SecurityDocument.cs
- TrackingMemoryStream.cs
- PointKeyFrameCollection.cs
- DataTableCollection.cs
- ToolStripControlHost.cs
- EntityDesignerDataSourceView.cs
- TemplateControl.cs
- DataGridViewAdvancedBorderStyle.cs
- IERequestCache.cs
- ArraySortHelper.cs
- LongValidatorAttribute.cs
- StateManagedCollection.cs
- IndexedGlyphRun.cs
- ContextStaticAttribute.cs
- ProcessInfo.cs
- SortDescription.cs
- Rotation3DKeyFrameCollection.cs
- SelectionChangedEventArgs.cs
- StreamResourceInfo.cs
- ManagedIStream.cs
- SerializerDescriptor.cs
- StateWorkerRequest.cs
- BindingCompleteEventArgs.cs
- BitmapDecoder.cs
- ConfigurationLoaderException.cs
- AuthenticatingEventArgs.cs
- DataSourceCacheDurationConverter.cs
- NTAccount.cs
- DataTableMappingCollection.cs
- RawKeyboardInputReport.cs
- MergeFailedEvent.cs
- UInt16Converter.cs
- COM2PropertyDescriptor.cs
- SendMailErrorEventArgs.cs
- BindingsCollection.cs
- _NetworkingPerfCounters.cs
- StorageEntityContainerMapping.cs
- FixUpCollection.cs
- arc.cs
- FileDialog_Vista.cs
- ListViewItemSelectionChangedEvent.cs
- GenericTextProperties.cs
- ConsumerConnectionPointCollection.cs
- FixedSchema.cs
- SqlMetaData.cs
- CompositeDataBoundControl.cs
- UserControl.cs
- XmlReaderDelegator.cs
- DateTimeSerializationSection.cs
- SimpleHandlerFactory.cs
- SupportingTokenDuplexChannel.cs
- SecurityProtocol.cs
- KeyInterop.cs
- HttpWriter.cs
- Terminate.cs
- ConfigXmlText.cs
- TreeViewTemplateSelector.cs
- SurrogateSelector.cs
- TrackPoint.cs
- CapabilitiesAssignment.cs
- EnumUnknown.cs
- WebServiceTypeData.cs
- DocumentsTrace.cs
- IPHostEntry.cs
- ToolStripScrollButton.cs
- AdornerHitTestResult.cs
- PageHandlerFactory.cs
- RootBrowserWindow.cs
- CompositeKey.cs
- CompiledQueryCacheEntry.cs
- SqlCacheDependencyDatabase.cs
- SelectiveScrollingGrid.cs
- CorrelationResolver.cs