Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / UI / PageHandlerFactory.cs / 1 / PageHandlerFactory.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Handler Factory implementation for Page files * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.UI { using System.Runtime.Serialization.Formatters; using System.IO; using System.Security.Permissions; using System.Web.Compilation; using System.Web.Util; using Debug=System.Web.Util.Debug; /* * Handler Factory implementation for ASP.NET files */ [PermissionSet(SecurityAction.LinkDemand, Unrestricted = true)] [PermissionSet(SecurityAction.InheritanceDemand, Unrestricted=true)] public class PageHandlerFactory : IHttpHandlerFactory2 { private bool _isInheritedInstance; protected internal PageHandlerFactory() { // Check whether this is the exact PageHandlerFactory, or a derived class _isInheritedInstance = (GetType() != typeof(PageHandlerFactory)); } public virtual IHttpHandler GetHandler(HttpContext context, string requestType, string virtualPath, string path) { Debug.Trace("PageHandlerFactory", "PageHandlerFactory: " + virtualPath); // This should never get called in ISAPI mode but currently is in integrated mode // Debug.Assert(false); return GetHandlerHelper(context, requestType, VirtualPath.CreateNonRelative(virtualPath), path); } IHttpHandler IHttpHandlerFactory2.GetHandler(HttpContext context, String requestType, VirtualPath virtualPath, String physicalPath) { // If it's a derived class, we must call the old (less efficient) GetHandler, in // case it was overriden if (_isInheritedInstance) { return GetHandler(context, requestType, virtualPath.VirtualPathString, physicalPath); } return GetHandlerHelper(context, requestType, virtualPath, physicalPath); } public virtual void ReleaseHandler(IHttpHandler handler) { } private IHttpHandler GetHandlerHelper(HttpContext context, string requestType, VirtualPath virtualPath, string physicalPath) { Page page = BuildManager.CreateInstanceFromVirtualPath( virtualPath, typeof(Page), context, true /*allowCrossApp*/, true /*noAssert*/) as Page; if (page == null) return null; page.TemplateControlVirtualPath = virtualPath; return page; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Handler Factory implementation for Page files * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.UI { using System.Runtime.Serialization.Formatters; using System.IO; using System.Security.Permissions; using System.Web.Compilation; using System.Web.Util; using Debug=System.Web.Util.Debug; /* * Handler Factory implementation for ASP.NET files */ [PermissionSet(SecurityAction.LinkDemand, Unrestricted = true)] [PermissionSet(SecurityAction.InheritanceDemand, Unrestricted=true)] public class PageHandlerFactory : IHttpHandlerFactory2 { private bool _isInheritedInstance; protected internal PageHandlerFactory() { // Check whether this is the exact PageHandlerFactory, or a derived class _isInheritedInstance = (GetType() != typeof(PageHandlerFactory)); } public virtual IHttpHandler GetHandler(HttpContext context, string requestType, string virtualPath, string path) { Debug.Trace("PageHandlerFactory", "PageHandlerFactory: " + virtualPath); // This should never get called in ISAPI mode but currently is in integrated mode // Debug.Assert(false); return GetHandlerHelper(context, requestType, VirtualPath.CreateNonRelative(virtualPath), path); } IHttpHandler IHttpHandlerFactory2.GetHandler(HttpContext context, String requestType, VirtualPath virtualPath, String physicalPath) { // If it's a derived class, we must call the old (less efficient) GetHandler, in // case it was overriden if (_isInheritedInstance) { return GetHandler(context, requestType, virtualPath.VirtualPathString, physicalPath); } return GetHandlerHelper(context, requestType, virtualPath, physicalPath); } public virtual void ReleaseHandler(IHttpHandler handler) { } private IHttpHandler GetHandlerHelper(HttpContext context, string requestType, VirtualPath virtualPath, string physicalPath) { Page page = BuildManager.CreateInstanceFromVirtualPath( virtualPath, typeof(Page), context, true /*allowCrossApp*/, true /*noAssert*/) as Page; if (page == null) return null; page.TemplateControlVirtualPath = virtualPath; return page; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
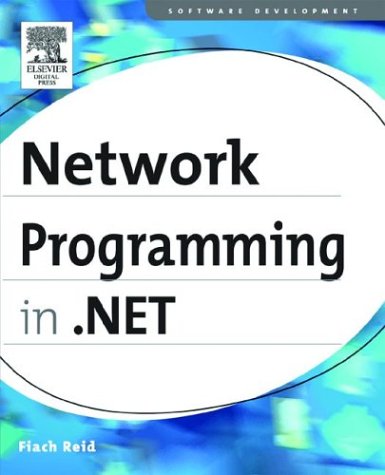
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SharedDp.cs
- SqlNotificationRequest.cs
- SortKey.cs
- StylusPointPropertyInfo.cs
- BackEase.cs
- Deserializer.cs
- Pen.cs
- TreeViewAutomationPeer.cs
- ZoneMembershipCondition.cs
- NewArray.cs
- Assembly.cs
- TouchesCapturedWithinProperty.cs
- FamilyMapCollection.cs
- ForceCopyBuildProvider.cs
- QueryOperationResponseOfT.cs
- ellipse.cs
- ValidatorCollection.cs
- Popup.cs
- Int64Storage.cs
- TextEditorLists.cs
- SizeKeyFrameCollection.cs
- UpWmlPageAdapter.cs
- ClrPerspective.cs
- MultiBindingExpression.cs
- StateWorkerRequest.cs
- NeedSkipTokenVisitor.cs
- HWStack.cs
- CodeExpressionStatement.cs
- TriggerActionCollection.cs
- EntityDataSourceConfigureObjectContext.cs
- RIPEMD160.cs
- BoundPropertyEntry.cs
- Vector3DIndependentAnimationStorage.cs
- TextContainerChangedEventArgs.cs
- DesignerAdapterAttribute.cs
- ConsoleCancelEventArgs.cs
- RegexCompiler.cs
- IteratorFilter.cs
- ListViewItem.cs
- View.cs
- ModuleBuilder.cs
- MessageBuffer.cs
- SplashScreen.cs
- BasicExpressionVisitor.cs
- FormsAuthenticationConfiguration.cs
- UnmanagedHandle.cs
- MinimizableAttributeTypeConverter.cs
- NetworkInformationPermission.cs
- SafeLibraryHandle.cs
- ServiceOperationWrapper.cs
- ResourceAssociationSetEnd.cs
- WebPartVerbCollection.cs
- SvcMapFile.cs
- shaperfactoryquerycacheentry.cs
- PixelShader.cs
- DictionaryContent.cs
- NotificationContext.cs
- RemotingSurrogateSelector.cs
- XmlMembersMapping.cs
- GraphicsContext.cs
- LOSFormatter.cs
- EllipticalNodeOperations.cs
- TemplatedMailWebEventProvider.cs
- ElementFactory.cs
- SocketException.cs
- RectangleGeometry.cs
- DataView.cs
- CurrentChangingEventManager.cs
- RotateTransform.cs
- ThousandthOfEmRealDoubles.cs
- ErrorsHelper.cs
- ServiceOperationParameter.cs
- XmlDigitalSignatureProcessor.cs
- SeekableReadStream.cs
- ProcessHostServerConfig.cs
- RegisteredScript.cs
- QueryResponse.cs
- Compress.cs
- JumpTask.cs
- SimpleModelProvider.cs
- CodeCatchClause.cs
- RowUpdatingEventArgs.cs
- DataGridViewRowPostPaintEventArgs.cs
- PropertyPathWorker.cs
- QueryStringConverter.cs
- Behavior.cs
- DiagnosticSection.cs
- NonValidatingSecurityTokenAuthenticator.cs
- HtmlLiteralTextAdapter.cs
- CheckedPointers.cs
- ToolboxBitmapAttribute.cs
- CapabilitiesUse.cs
- RequestResizeEvent.cs
- HwndSource.cs
- Binding.cs
- HwndSource.cs
- QuotaThrottle.cs
- HighContrastHelper.cs
- HitTestParameters3D.cs
- EditorZoneBase.cs