Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / HWStack.cs / 1305376 / HWStack.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; namespace System.Xml { // This stack is designed to minimize object creation for the // objects being stored in the stack by allowing them to be // re-used over time. It basically pushes the objects creating // a high water mark then as Pop() is called they are not removed // so that next time Push() is called it simply returns the last // object that was already on the stack. internal class HWStack : ICloneable { internal HWStack(int GrowthRate) : this (GrowthRate, int.MaxValue) {} internal HWStack(int GrowthRate, int limit) { this.growthRate = GrowthRate; this.used = 0; this.stack = new Object[GrowthRate]; this.size = GrowthRate; this.limit = limit; } internal Object Push() { if (this.used == this.size) { if (this.limit <= this.used) { throw new XmlException(Res.Xml_StackOverflow, string.Empty); } Object[] newstack = new Object[this.size + this.growthRate]; if (this.used > 0) { System.Array.Copy(this.stack, 0, newstack, 0, this.used); } this.stack = newstack; this.size += this.growthRate; } return this.stack[this.used++]; } internal Object Pop() { if (0 < this.used) { this.used--; Object result = this.stack[this.used]; return result; } return null; } [System.Runtime.TargetedPatchingOptOutAttribute("Performance critical to inline across NGen image boundaries")] internal object Peek() { return this.used > 0 ? this.stack[this.used - 1] : null; } [System.Runtime.TargetedPatchingOptOutAttribute("Performance critical to inline across NGen image boundaries")] internal void AddToTop(object o) { if (this.used > 0) { this.stack[this.used - 1] = o; } } internal Object this[int index] { get { if (index >= 0 && index < this.used) { Object result = this.stack[index]; return result; } else { throw new IndexOutOfRangeException(); } } set { if (index >= 0 && index < this.used) { this.stack[index] = value; } else { throw new IndexOutOfRangeException(); } } } internal int Length { get { return this.used;} } // // ICloneable // private HWStack(object[] stack, int growthRate, int used, int size) { this.stack = stack; this.growthRate = growthRate; this.used = used; this.size = size; } public object Clone() { return new HWStack((object[]) this.stack.Clone(), this.growthRate, this.used, this.size); } private Object[] stack; private int growthRate; private int used; private int size; private int limit; }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; namespace System.Xml { // This stack is designed to minimize object creation for the // objects being stored in the stack by allowing them to be // re-used over time. It basically pushes the objects creating // a high water mark then as Pop() is called they are not removed // so that next time Push() is called it simply returns the last // object that was already on the stack. internal class HWStack : ICloneable { internal HWStack(int GrowthRate) : this (GrowthRate, int.MaxValue) {} internal HWStack(int GrowthRate, int limit) { this.growthRate = GrowthRate; this.used = 0; this.stack = new Object[GrowthRate]; this.size = GrowthRate; this.limit = limit; } internal Object Push() { if (this.used == this.size) { if (this.limit <= this.used) { throw new XmlException(Res.Xml_StackOverflow, string.Empty); } Object[] newstack = new Object[this.size + this.growthRate]; if (this.used > 0) { System.Array.Copy(this.stack, 0, newstack, 0, this.used); } this.stack = newstack; this.size += this.growthRate; } return this.stack[this.used++]; } internal Object Pop() { if (0 < this.used) { this.used--; Object result = this.stack[this.used]; return result; } return null; } [System.Runtime.TargetedPatchingOptOutAttribute("Performance critical to inline across NGen image boundaries")] internal object Peek() { return this.used > 0 ? this.stack[this.used - 1] : null; } [System.Runtime.TargetedPatchingOptOutAttribute("Performance critical to inline across NGen image boundaries")] internal void AddToTop(object o) { if (this.used > 0) { this.stack[this.used - 1] = o; } } internal Object this[int index] { get { if (index >= 0 && index < this.used) { Object result = this.stack[index]; return result; } else { throw new IndexOutOfRangeException(); } } set { if (index >= 0 && index < this.used) { this.stack[index] = value; } else { throw new IndexOutOfRangeException(); } } } internal int Length { get { return this.used;} } // // ICloneable // private HWStack(object[] stack, int growthRate, int used, int size) { this.stack = stack; this.growthRate = growthRate; this.used = used; this.size = size; } public object Clone() { return new HWStack((object[]) this.stack.Clone(), this.growthRate, this.used, this.size); } private Object[] stack; private int growthRate; private int used; private int size; private int limit; }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
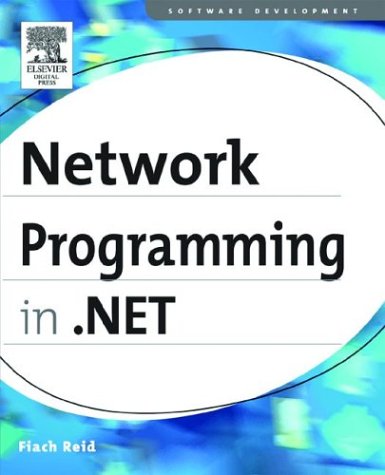
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MappingMetadataHelper.cs
- XmlSchemaComplexContent.cs
- ProviderConnectionPoint.cs
- CultureMapper.cs
- InvalidOleVariantTypeException.cs
- ListViewPagedDataSource.cs
- ManipulationVelocities.cs
- MatrixStack.cs
- PublishLicense.cs
- TaskCanceledException.cs
- FilePrompt.cs
- ClosableStream.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- ConfigDefinitionUpdates.cs
- ConnectionManagementElementCollection.cs
- InvokeHandlers.cs
- DropShadowBitmapEffect.cs
- XmlDeclaration.cs
- TraceLog.cs
- DBConnection.cs
- ArgIterator.cs
- OAVariantLib.cs
- ConfigurationConverterBase.cs
- SettingsPropertyValueCollection.cs
- PixelShader.cs
- PageStatePersister.cs
- TraceHandlerErrorFormatter.cs
- TextSpan.cs
- SafeProcessHandle.cs
- Error.cs
- InternalDispatchObject.cs
- StringFunctions.cs
- QuaternionRotation3D.cs
- TreeNodeCollection.cs
- ToolBar.cs
- SQLDecimalStorage.cs
- SqlClientPermission.cs
- ObjectQueryProvider.cs
- TextServicesCompartmentContext.cs
- GenericXmlSecurityTokenAuthenticator.cs
- Row.cs
- TrackingStringDictionary.cs
- DigestComparer.cs
- ContentDesigner.cs
- SystemWebCachingSectionGroup.cs
- QilTargetType.cs
- FixedPage.cs
- Parser.cs
- isolationinterop.cs
- sqlser.cs
- SafeProcessHandle.cs
- MultiBindingExpression.cs
- TextRunProperties.cs
- DiscoveryDocument.cs
- ContextProperty.cs
- _NestedSingleAsyncResult.cs
- XmlCustomFormatter.cs
- WebPartConnectionsCancelEventArgs.cs
- BaseParaClient.cs
- DeflateStreamAsyncResult.cs
- InitializerFacet.cs
- XmlSerializerFactory.cs
- SoapAttributeOverrides.cs
- WebPartZoneDesigner.cs
- DefaultTraceListener.cs
- DependencyObjectType.cs
- ActivityMarkupSerializationProvider.cs
- Queue.cs
- GradientBrush.cs
- FontInfo.cs
- LayoutUtils.cs
- PropertyDescriptor.cs
- PropertyTabAttribute.cs
- SqlUnionizer.cs
- CaseExpr.cs
- MSG.cs
- FormViewDeletedEventArgs.cs
- SchemeSettingElement.cs
- QilStrConcatenator.cs
- loginstatus.cs
- ProfileSection.cs
- EditorResources.cs
- FontWeightConverter.cs
- DrawingVisual.cs
- ParallelTimeline.cs
- FormViewUpdatedEventArgs.cs
- LicenseContext.cs
- LayoutDump.cs
- StringBlob.cs
- ExportOptions.cs
- LogStore.cs
- OdbcDataReader.cs
- DocumentOrderComparer.cs
- SQLChars.cs
- WebPartConnectionsCancelEventArgs.cs
- WebControlsSection.cs
- BuildProviderAppliesToAttribute.cs
- HttpPostedFile.cs
- BookmarkScopeHandle.cs
- HijriCalendar.cs