Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / Utility / TraceLog.cs / 1 / TraceLog.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Log of recent actions. Use this to debug those nasty problems // that don't repro on demand and don't have enough information in a crash // dump. // // In the class(es) of interest, add a TraceLog object. At points of // interest, call TraceLog.Add to record a string in the log. After the // crash, call TraceLog.WriteLog (or simply examine the log directly in // the debugger). Log entries are timestamped. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Globalization; namespace MS.Internal.Utility { internal class TraceLog { // create an unbounded trace log internal TraceLog() : this(Int32.MaxValue) {} // create a trace log that remembers the last 'size' actions internal TraceLog(int size) { _size = size; _log = new ArrayList(); } // add an entry to the log. Args are just like String.Format internal void Add(string message, params object[] args) { // create timestamped message string string s = DateTime.Now.Ticks.ToString(CultureInfo.InvariantCulture) + " " + String.Format(CultureInfo.InvariantCulture, message, args); // if log is full, discard the oldest message if (_log.Count == _size) _log.RemoveAt(0); // add the new message _log.Add(s); } // write the log to the console internal void WriteLog() { for (int k=0; k<_log.Count; ++k) Console.WriteLine(_log[k]); } // return a printable id for the object internal static string IdFor(object o) { if (o == null) return "NULL"; else return String.Format(CultureInfo.InvariantCulture, "{0}.{1}", o.GetType().Name, o.GetHashCode()); } ArrayList _log; int _size; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Log of recent actions. Use this to debug those nasty problems // that don't repro on demand and don't have enough information in a crash // dump. // // In the class(es) of interest, add a TraceLog object. At points of // interest, call TraceLog.Add to record a string in the log. After the // crash, call TraceLog.WriteLog (or simply examine the log directly in // the debugger). Log entries are timestamped. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Globalization; namespace MS.Internal.Utility { internal class TraceLog { // create an unbounded trace log internal TraceLog() : this(Int32.MaxValue) {} // create a trace log that remembers the last 'size' actions internal TraceLog(int size) { _size = size; _log = new ArrayList(); } // add an entry to the log. Args are just like String.Format internal void Add(string message, params object[] args) { // create timestamped message string string s = DateTime.Now.Ticks.ToString(CultureInfo.InvariantCulture) + " " + String.Format(CultureInfo.InvariantCulture, message, args); // if log is full, discard the oldest message if (_log.Count == _size) _log.RemoveAt(0); // add the new message _log.Add(s); } // write the log to the console internal void WriteLog() { for (int k=0; k<_log.Count; ++k) Console.WriteLine(_log[k]); } // return a printable id for the object internal static string IdFor(object o) { if (o == null) return "NULL"; else return String.Format(CultureInfo.InvariantCulture, "{0}.{1}", o.GetType().Name, o.GetHashCode()); } ArrayList _log; int _size; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
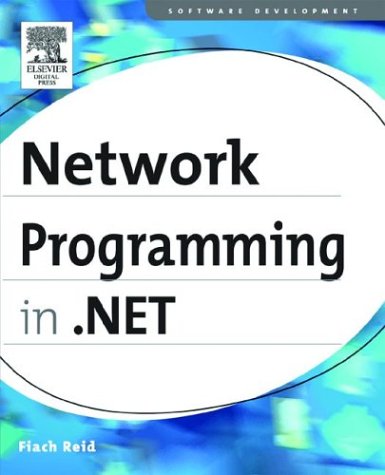
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PassportIdentity.cs
- InvalidFilterCriteriaException.cs
- Hex.cs
- XmlDataImplementation.cs
- Compiler.cs
- Geometry3D.cs
- ConnectionPointCookie.cs
- DataStreamFromComStream.cs
- GregorianCalendar.cs
- FontUnit.cs
- Compensate.cs
- FunctionMappingTranslator.cs
- IndicShape.cs
- TerminatorSinks.cs
- AlignmentXValidation.cs
- XMLSchema.cs
- LinearQuaternionKeyFrame.cs
- ChannelSinkStacks.cs
- ObjectListItemCollection.cs
- DeclaredTypeElement.cs
- RSAPKCS1SignatureDeformatter.cs
- PersonalizationEntry.cs
- ServiceBehaviorElementCollection.cs
- WebPart.cs
- DataSourceGeneratorException.cs
- DesigntimeLicenseContextSerializer.cs
- SecureUICommand.cs
- BindingExpression.cs
- ActivitiesCollection.cs
- XmlAttributeCollection.cs
- indexingfiltermarshaler.cs
- ScrollViewer.cs
- MultiByteCodec.cs
- _SingleItemRequestCache.cs
- RequestCachePolicyConverter.cs
- RelationHandler.cs
- XmlChildNodes.cs
- DbParameterCollectionHelper.cs
- Encoding.cs
- XdrBuilder.cs
- mda.cs
- XmlSchemaComplexContentRestriction.cs
- EntityDataSourceSelectingEventArgs.cs
- AspNetCacheProfileAttribute.cs
- SplashScreen.cs
- RunClient.cs
- TypeLibConverter.cs
- SoapExtensionStream.cs
- WebException.cs
- ColumnCollection.cs
- TextEndOfLine.cs
- XmlSchemaAttributeGroup.cs
- PrintSchema.cs
- SimpleType.cs
- _CommandStream.cs
- Pair.cs
- Application.cs
- ListViewAutomationPeer.cs
- SoapServerMessage.cs
- SqlCacheDependencySection.cs
- TraceHandlerErrorFormatter.cs
- RequiredFieldValidator.cs
- elementinformation.cs
- PolyLineSegment.cs
- control.ime.cs
- GridViewUpdateEventArgs.cs
- XmlLanguage.cs
- GeometryModel3D.cs
- HelloMessageCD1.cs
- MetabaseServerConfig.cs
- TransformDescriptor.cs
- serverconfig.cs
- XmlNodeChangedEventManager.cs
- DBCSCodePageEncoding.cs
- DefaultSerializationProviderAttribute.cs
- CompModSwitches.cs
- ClipboardData.cs
- PassportAuthenticationModule.cs
- CredentialCache.cs
- Delay.cs
- MailDefinition.cs
- PropertyDescriptors.cs
- StringUtil.cs
- ButtonStandardAdapter.cs
- Events.cs
- HttpsHostedTransportConfiguration.cs
- DbFunctionCommandTree.cs
- DefaultShape.cs
- QuadraticBezierSegment.cs
- SecurityHeaderTokenResolver.cs
- HybridWebProxyFinder.cs
- BuildManagerHost.cs
- connectionpool.cs
- EncoderParameter.cs
- XmlIlGenerator.cs
- AspNetSynchronizationContext.cs
- PathTooLongException.cs
- XmlComplianceUtil.cs
- SafeRegistryHandle.cs
- RelationshipEndMember.cs