Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / Ink / InkSerializedFormat / MultiByteCodec.cs / 1305600 / MultiByteCodec.cs
using MS.Utility; using System; using System.Runtime.InteropServices; using System.Security; using System.Globalization; using System.Windows; using System.Windows.Input; using System.Windows.Ink; using System.Collections.Generic; using MS.Internal.Ink.InkSerializedFormat; using System.Diagnostics; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink.InkSerializedFormat { ////// MultiByteCodec /// internal class MultiByteCodec { ////// MultiByteCodec /// internal MultiByteCodec() { } ////// Encode /// /// /// internal void Encode(uint data, Listoutput) { if (output == null) { throw new ArgumentNullException("output"); } while (data > 0x7f) { byte byteToAdd = (byte)(0x80 | (byte)data & 0x7f); output.Add(byteToAdd); data >>= 7; } byte finalByteToAdd = (byte)(data & 0x7f); output.Add(finalByteToAdd); } /// /// SignEncode /// /// /// internal void SignEncode(int data, Listoutput) { uint xfData = 0; if (data < 0) { xfData = (uint)( (-data << 1) | 0x01 ); } else { xfData = (uint)data << 1; } Encode(xfData, output); } /// /// Decode /// /// /// /// ///internal uint Decode(byte[] input, int inputIndex, ref uint data) { Debug.Assert(input != null); Debug.Assert(inputIndex < input.Length); // We care about first 5 bytes uint cb = (input.Length - inputIndex > 5) ? 5 : (uint)(input.Length - inputIndex); uint index = 0; data = 0; while ((index < cb) && (input[index] > 0x7f)) { int leftShift = (int)(index * 7); data |= (uint)((input[index] & 0x7f) << leftShift); ++index; } if (index < cb) { int leftShift = (int)(index * 7); data |= (uint)((input[index] & 0x7f) << leftShift); } else { throw new ArgumentException(StrokeCollectionSerializer.ISFDebugMessage("invalid input in MultiByteCodec.Decode")); } return (index + 1); } /// /// SignDecode /// /// /// /// ///internal uint SignDecode(byte[] input, int inputIndex, ref int data) { Debug.Assert(input != null); //already validated at the AlgoModule level if (inputIndex >= input.Length) { throw new ArgumentOutOfRangeException("inputIndex"); } uint xfData = 0; uint cb = Decode(input, inputIndex, ref xfData); data = (0 != (0x01 & xfData)) ? -(int)(xfData >> 1) : (int)(xfData >> 1); return cb; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
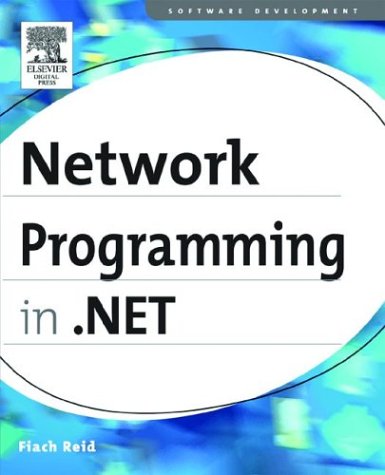
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UriParserTemplates.cs
- WindowsTokenRoleProvider.cs
- WebServiceClientProxyGenerator.cs
- Int32RectConverter.cs
- CodeRemoveEventStatement.cs
- LabelLiteral.cs
- EnumValidator.cs
- DataException.cs
- CharacterHit.cs
- UnsafeNativeMethods.cs
- AnnotationObservableCollection.cs
- HostedHttpRequestAsyncResult.cs
- NonBatchDirectoryCompiler.cs
- ErrorHandlingAcceptor.cs
- InfoCardRequestException.cs
- ResourceBinder.cs
- XmlSchema.cs
- ContextMenuStripGroup.cs
- FileUtil.cs
- DetailsViewCommandEventArgs.cs
- TreeNodeStyle.cs
- OdbcInfoMessageEvent.cs
- Brush.cs
- XmlUTF8TextWriter.cs
- FileDialog.cs
- XmlWriterSettings.cs
- DataGridViewIntLinkedList.cs
- InternalCache.cs
- AnimationLayer.cs
- BrushConverter.cs
- XmlDictionaryString.cs
- EventLog.cs
- InstanceKeyCompleteException.cs
- TreeViewItem.cs
- FieldToken.cs
- PointLight.cs
- AutoGeneratedField.cs
- DesignerActionTextItem.cs
- TrailingSpaceComparer.cs
- ObjectListShowCommandsEventArgs.cs
- Char.cs
- SecureStringHasher.cs
- FormViewRow.cs
- EqualityComparer.cs
- TokenBasedSetEnumerator.cs
- EventWaitHandleSecurity.cs
- XXXInfos.cs
- ToolbarAUtomationPeer.cs
- SortDescriptionCollection.cs
- TimeIntervalCollection.cs
- TabletDeviceInfo.cs
- M3DUtil.cs
- SqlConnectionPoolGroupProviderInfo.cs
- BitStack.cs
- WizardForm.cs
- DispatchWrapper.cs
- InkCanvasAutomationPeer.cs
- AdvancedBindingPropertyDescriptor.cs
- WasAdminWrapper.cs
- EdmFunction.cs
- XmlSchemaAny.cs
- WebPartConnectionsEventArgs.cs
- EmptyEnumerator.cs
- ParameterCollection.cs
- CannotUnloadAppDomainException.cs
- FtpWebResponse.cs
- WebPartUtil.cs
- DecoderNLS.cs
- PngBitmapEncoder.cs
- StorageSetMapping.cs
- DBConnection.cs
- FamilyMap.cs
- Material.cs
- RawStylusInputCustomData.cs
- DEREncoding.cs
- ElementHostAutomationPeer.cs
- SmtpTransport.cs
- DecoderNLS.cs
- DecimalAnimationBase.cs
- ConstructorNeedsTagAttribute.cs
- _Connection.cs
- BatchStream.cs
- SqlUtil.cs
- SessionStateItemCollection.cs
- SafeTokenHandle.cs
- CodeComment.cs
- util.cs
- HitTestWithGeometryDrawingContextWalker.cs
- CssTextWriter.cs
- WebPartDisplayModeEventArgs.cs
- DataKeyCollection.cs
- SqlDataSourceQueryEditor.cs
- LoginView.cs
- AsymmetricKeyExchangeDeformatter.cs
- DocumentAutomationPeer.cs
- HandlerBase.cs
- Metafile.cs
- HtmlInputButton.cs
- ToolboxComponentsCreatedEventArgs.cs
- ForwardPositionQuery.cs