Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Mapping / StorageSetMapping.cs / 1305376 / StorageSetMapping.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; using System.Data.Common.Utils; using System.Diagnostics; using System.Linq; namespace System.Data.Mapping { using Triple = Pair>; /// /// Represents the Mapping metadata for an Extent in ES space. /// ////// For Example if conceptually you could represent the ES MSL file as following /// --Mapping /// --EntityContainerMapping ( CNorthwind-->SNorthwind ) /// --EntitySetMapping /// --EntityTypeMapping /// --TableMappingFragment /// --EntityTypeMapping /// --TableMappingFragment /// --AssociationSetMapping /// --AssociationTypeMapping /// --TableMappingFragment /// --EntityContainerMapping ( CMyDatabase-->SMyDatabase ) /// --CompositionSetMapping /// --CompositionTypeMapping /// This class represents the metadata for all the extent map elements in the /// above example namely EntitySetMapping, AssociationSetMapping and CompositionSetMapping. /// The SetMapping elements that are children of the EntityContainerMapping element /// can be accessed through the properties on this type. /// internal abstract class StorageSetMapping { #region Constructors ////// Construct the new ExtentMapping object. /// /// Extent metadata object /// The EntityContainer mapping that contains this extent mapping internal StorageSetMapping(EntitySetBase extent, StorageEntityContainerMapping entityContainerMapping) { this.m_entityContainerMapping = entityContainerMapping; this.m_extent = extent; this.m_typeMappings = new List(); } #endregion #region Fields private StorageEntityContainerMapping m_entityContainerMapping; //The EntityContainer mapping that contains this extent mapping private EntitySetBase m_extent; //The extent for which this mapping represents. private List m_typeMappings; //Set of type mappings that make up the Set Mapping //Unless this is a EntitySetMapping with inheritance, //you would have a single type mapping per set private string m_queryView; //User defined Query View for the EntitySet private int m_startLineNumber; //Line Number for Set Mapping element start tag private int m_startLinePosition; //Line position for Set Mapping element start tag private bool m_hasModificationFunctionMapping; // has modificationfunctionmapping for set mapping //Stores type-Specific user-defined QueryViews private Dictionary m_typeSpecificQueryViews = new Dictionary (Triple.PairComparer.Instance); #endregion #region Properties /// /// The set for which this mapping is for /// internal EntitySetBase Set { get { return this.m_extent; } } ////////// TypeMappings that make up this set type. ///// For AssociationSet and CompositionSet there will be one type( atleast thats what ///// we expect as of now). EntitySet could have mappings for multiple Entity types. ///// internal ReadOnlyCollectionTypeMappings { get { return this.m_typeMappings.AsReadOnly(); } } internal StorageEntityContainerMapping EntityContainerMapping { get { return m_entityContainerMapping; } } /// /// Whether the SetMapping has empty content /// Returns true if there no table Mapping fragments /// internal virtual bool HasNoContent { get { if (QueryView != null) { return false; } foreach (StorageTypeMapping typeMap in TypeMappings) { foreach (StorageMappingFragment mapFragment in typeMap.MappingFragments) { foreach (StoragePropertyMapping propertyMap in mapFragment.AllProperties) { return false; } } } return true; } } internal string QueryView { get { return m_queryView; } set { m_queryView = value; } } ////// Line Number in MSL file where the Set Mapping Element's Start Tag is present. /// internal int StartLineNumber { get { return m_startLineNumber; } set { m_startLineNumber = value; } } ////// Line Position in MSL file where the Set Mapping Element's Start Tag is present. /// internal int StartLinePosition { get { return m_startLinePosition; } set { m_startLinePosition = value; } } internal bool HasModificationFunctionMapping { get { return m_hasModificationFunctionMapping; } set { m_hasModificationFunctionMapping = value; } } #endregion #region Methods ////// Add type mapping as a child under this SetMapping /// /// internal void AddTypeMapping(StorageTypeMapping typeMapping) { this.m_typeMappings.Add(typeMapping); } ////// This method is primarily for debugging purposes. /// Will be removed shortly. /// internal abstract void Print(int index); internal bool ContainsTypeSpecificQueryView(Triple key) { return m_typeSpecificQueryViews.ContainsKey(key); } ////// Stores a type-specific user-defiend QueryView so that it can be loaded /// into StorageMappingItemCollection's view cache. /// internal void AddTypeSpecificQueryView(Triple key, string viewString) { Debug.Assert(!m_typeSpecificQueryViews.ContainsKey(key), "Query View already present for the given Key"); m_typeSpecificQueryViews.Add(key, viewString); } internal ReadOnlyCollectionGetTypeSpecificQVKeys() { return new ReadOnlyCollection (m_typeSpecificQueryViews.Keys.ToList()); } internal string GetTypeSpecificQueryView(Triple key) { Debug.Assert(m_typeSpecificQueryViews.ContainsKey(key)); return m_typeSpecificQueryViews[key]; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; using System.Data.Common.Utils; using System.Diagnostics; using System.Linq; namespace System.Data.Mapping { using Triple = Pair>; /// /// Represents the Mapping metadata for an Extent in ES space. /// ////// For Example if conceptually you could represent the ES MSL file as following /// --Mapping /// --EntityContainerMapping ( CNorthwind-->SNorthwind ) /// --EntitySetMapping /// --EntityTypeMapping /// --TableMappingFragment /// --EntityTypeMapping /// --TableMappingFragment /// --AssociationSetMapping /// --AssociationTypeMapping /// --TableMappingFragment /// --EntityContainerMapping ( CMyDatabase-->SMyDatabase ) /// --CompositionSetMapping /// --CompositionTypeMapping /// This class represents the metadata for all the extent map elements in the /// above example namely EntitySetMapping, AssociationSetMapping and CompositionSetMapping. /// The SetMapping elements that are children of the EntityContainerMapping element /// can be accessed through the properties on this type. /// internal abstract class StorageSetMapping { #region Constructors ////// Construct the new ExtentMapping object. /// /// Extent metadata object /// The EntityContainer mapping that contains this extent mapping internal StorageSetMapping(EntitySetBase extent, StorageEntityContainerMapping entityContainerMapping) { this.m_entityContainerMapping = entityContainerMapping; this.m_extent = extent; this.m_typeMappings = new List(); } #endregion #region Fields private StorageEntityContainerMapping m_entityContainerMapping; //The EntityContainer mapping that contains this extent mapping private EntitySetBase m_extent; //The extent for which this mapping represents. private List m_typeMappings; //Set of type mappings that make up the Set Mapping //Unless this is a EntitySetMapping with inheritance, //you would have a single type mapping per set private string m_queryView; //User defined Query View for the EntitySet private int m_startLineNumber; //Line Number for Set Mapping element start tag private int m_startLinePosition; //Line position for Set Mapping element start tag private bool m_hasModificationFunctionMapping; // has modificationfunctionmapping for set mapping //Stores type-Specific user-defined QueryViews private Dictionary m_typeSpecificQueryViews = new Dictionary (Triple.PairComparer.Instance); #endregion #region Properties /// /// The set for which this mapping is for /// internal EntitySetBase Set { get { return this.m_extent; } } ////////// TypeMappings that make up this set type. ///// For AssociationSet and CompositionSet there will be one type( atleast thats what ///// we expect as of now). EntitySet could have mappings for multiple Entity types. ///// internal ReadOnlyCollectionTypeMappings { get { return this.m_typeMappings.AsReadOnly(); } } internal StorageEntityContainerMapping EntityContainerMapping { get { return m_entityContainerMapping; } } /// /// Whether the SetMapping has empty content /// Returns true if there no table Mapping fragments /// internal virtual bool HasNoContent { get { if (QueryView != null) { return false; } foreach (StorageTypeMapping typeMap in TypeMappings) { foreach (StorageMappingFragment mapFragment in typeMap.MappingFragments) { foreach (StoragePropertyMapping propertyMap in mapFragment.AllProperties) { return false; } } } return true; } } internal string QueryView { get { return m_queryView; } set { m_queryView = value; } } ////// Line Number in MSL file where the Set Mapping Element's Start Tag is present. /// internal int StartLineNumber { get { return m_startLineNumber; } set { m_startLineNumber = value; } } ////// Line Position in MSL file where the Set Mapping Element's Start Tag is present. /// internal int StartLinePosition { get { return m_startLinePosition; } set { m_startLinePosition = value; } } internal bool HasModificationFunctionMapping { get { return m_hasModificationFunctionMapping; } set { m_hasModificationFunctionMapping = value; } } #endregion #region Methods ////// Add type mapping as a child under this SetMapping /// /// internal void AddTypeMapping(StorageTypeMapping typeMapping) { this.m_typeMappings.Add(typeMapping); } ////// This method is primarily for debugging purposes. /// Will be removed shortly. /// internal abstract void Print(int index); internal bool ContainsTypeSpecificQueryView(Triple key) { return m_typeSpecificQueryViews.ContainsKey(key); } ////// Stores a type-specific user-defiend QueryView so that it can be loaded /// into StorageMappingItemCollection's view cache. /// internal void AddTypeSpecificQueryView(Triple key, string viewString) { Debug.Assert(!m_typeSpecificQueryViews.ContainsKey(key), "Query View already present for the given Key"); m_typeSpecificQueryViews.Add(key, viewString); } internal ReadOnlyCollectionGetTypeSpecificQVKeys() { return new ReadOnlyCollection (m_typeSpecificQueryViews.Keys.ToList()); } internal string GetTypeSpecificQueryView(Triple key) { Debug.Assert(m_typeSpecificQueryViews.ContainsKey(key)); return m_typeSpecificQueryViews[key]; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
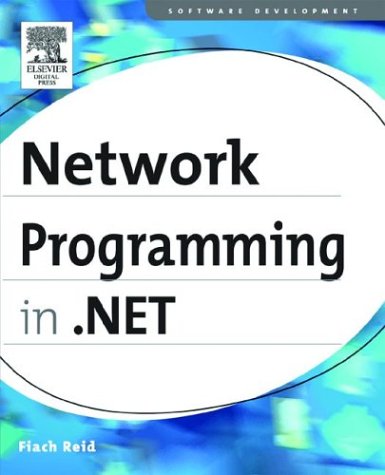
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ValidationSummaryDesigner.cs
- RuleCache.cs
- SemanticResultKey.cs
- BindingExpression.cs
- TreeNode.cs
- UDPClient.cs
- ApplicationFileCodeDomTreeGenerator.cs
- PeerNameRecord.cs
- NameTable.cs
- ByteAnimation.cs
- MessageSmuggler.cs
- RsaSecurityToken.cs
- OracleRowUpdatedEventArgs.cs
- CompilerResults.cs
- CompiledXpathExpr.cs
- SingleStorage.cs
- InputLanguageSource.cs
- nulltextcontainer.cs
- SelectionWordBreaker.cs
- OleDbDataAdapter.cs
- ModelProperty.cs
- LockCookie.cs
- DefaultValueConverter.cs
- UserNameSecurityTokenAuthenticator.cs
- AsyncWaitHandle.cs
- InstallerTypeAttribute.cs
- GridView.cs
- XmlDataSourceView.cs
- ColorMap.cs
- TableLayoutPanelDesigner.cs
- DES.cs
- SemanticBasicElement.cs
- CompilerResults.cs
- TypeSemantics.cs
- Decoder.cs
- PointConverter.cs
- RectangleF.cs
- ConstructorNeedsTagAttribute.cs
- ClientOperation.cs
- Point.cs
- ToolStripRenderer.cs
- SamlNameIdentifierClaimResource.cs
- RadioButtonBaseAdapter.cs
- TabControl.cs
- LocalFileSettingsProvider.cs
- HttpStreams.cs
- IxmlLineInfo.cs
- FrameworkTemplate.cs
- NamedObjectList.cs
- PriorityChain.cs
- PersistenceTask.cs
- DataGridState.cs
- ToolStripControlHost.cs
- CapabilitiesState.cs
- DataControlButton.cs
- XsdDataContractExporter.cs
- KeyConverter.cs
- WebPartEditVerb.cs
- ValidatorCollection.cs
- FlowPanelDesigner.cs
- SQLInt32.cs
- ComboBoxDesigner.cs
- control.ime.cs
- FileChangesMonitor.cs
- XmlSchemaFacet.cs
- ServiceModelSectionGroup.cs
- WindowsScroll.cs
- Speller.cs
- MenuItemStyle.cs
- HMACSHA512.cs
- PasswordRecovery.cs
- CompiledRegexRunner.cs
- Stroke2.cs
- HttpFormatExtensions.cs
- WebServiceResponse.cs
- OutputCacheSettingsSection.cs
- TransactionManager.cs
- PageParser.cs
- PropertyEmitterBase.cs
- ImageList.cs
- ValueConversionAttribute.cs
- OraclePermissionAttribute.cs
- AccessDataSource.cs
- DataGridParentRows.cs
- CallbackValidatorAttribute.cs
- CodeGotoStatement.cs
- ASCIIEncoding.cs
- FromRequest.cs
- EntitySetBaseCollection.cs
- WindowsListViewItemCheckBox.cs
- ZoneIdentityPermission.cs
- FormsAuthenticationUser.cs
- ping.cs
- XmlDocument.cs
- Msec.cs
- RandomNumberGenerator.cs
- SchemaTypeEmitter.cs
- ChangePassword.cs
- SQLSingle.cs
- PathBox.cs