Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / ComboBoxDesigner.cs / 1 / ComboBoxDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Design.ComboBoxDesigner..ctor()")] namespace System.Windows.Forms.Design { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Windows.Forms.Design.Behavior; ////// /// internal class ComboBoxDesigner : ControlDesigner { private EventHandler propChanged = null; // Delegate used to dirty the selectionUIItem when needed. private DesignerActionListCollection _actionLists; ////// Provides a designer that can design components /// that extend ComboBox. ////// /// Adds a baseline SnapLine to the list of SnapLines related /// to this control. /// public override IList SnapLines { get { ArrayList snapLines = base.SnapLines as ArrayList; //a single text-baseline for the label (and linklabel) control int baseline = DesignerUtils.GetTextBaseline(Control, System.Drawing.ContentAlignment.TopLeft); baseline += 3; snapLines.Add(new SnapLine(SnapLineType.Baseline, baseline, SnapLinePriority.Medium)); return snapLines; } } ////// /// Disposes of this object. /// protected override void Dispose(bool disposing) { if (disposing) { // Hook up the property change notification so that we can dirty the SelectionUIItem when needed. if (propChanged != null) { ((ComboBox)Control).StyleChanged -= propChanged; } } base.Dispose(disposing); } ////// /// Called by the host when we're first initialized. /// public override void Initialize(IComponent component) { base.Initialize(component); AutoResizeHandles = true; // Hook up the property change notification so that we can dirty the SelectionUIItem when needed. propChanged = new EventHandler(this.OnControlPropertyChanged); ((ComboBox)Control).StyleChanged += propChanged; } ////// /// We override this so we can clear the text field set by controldesigner. /// public override void InitializeNewComponent(IDictionary defaultValues) { base.InitializeNewComponent(defaultValues); // in Whidbey, formattingEnabled is TRUE ((ComboBox) this.Component).FormattingEnabled = true; PropertyDescriptor textProp = TypeDescriptor.GetProperties(Component)["Text"]; if (textProp != null && textProp.PropertyType == typeof(string) && !textProp.IsReadOnly && textProp.IsBrowsable) { textProp.SetValue(Component, ""); } } ////// /// For controls, we sync their property changed event so our component can track their location. /// private void OnControlPropertyChanged(object sender, EventArgs e) { if (BehaviorService != null) { BehaviorService.SyncSelection(); } } ////// /// Retrieves a set of rules concerning the movement capabilities of a component. /// This should be one or more flags from the SelectionRules class. If no designer /// provides rules for a component, the component will not get any UI services. /// public override SelectionRules SelectionRules { get { SelectionRules rules = base.SelectionRules; object component = Component; PropertyDescriptor propStyle = TypeDescriptor.GetProperties(component)["DropDownStyle"]; if (propStyle != null) { ComboBoxStyle style = (ComboBoxStyle)propStyle.GetValue(component); // Height is not user-changable for these styles if (style == ComboBoxStyle.DropDown || style == ComboBoxStyle.DropDownList) rules &= ~(SelectionRules.TopSizeable | SelectionRules.BottomSizeable); } return rules; } } public override DesignerActionListCollection ActionLists { get { if (_actionLists == null) { _actionLists = new DesignerActionListCollection(); _actionLists.Add(new ListControlBoundActionList(this)); } return _actionLists; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
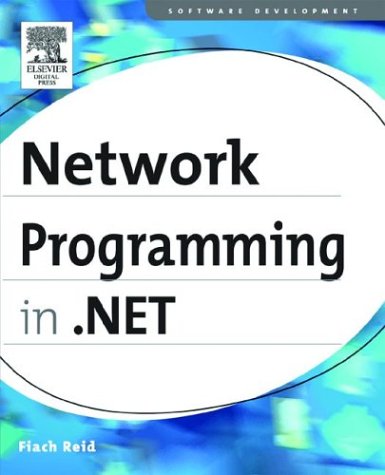
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DetailsViewModeEventArgs.cs
- DataGridViewColumnCollectionDialog.cs
- ChangePasswordDesigner.cs
- AnimationClock.cs
- Attributes.cs
- EventPropertyMap.cs
- RegistrySecurity.cs
- BuildResultCache.cs
- XmlLinkedNode.cs
- OleDbParameter.cs
- PrintControllerWithStatusDialog.cs
- Attributes.cs
- WebPartDisplayModeEventArgs.cs
- AnnotationMap.cs
- DbMetaDataCollectionNames.cs
- LogicalCallContext.cs
- UnsafeNativeMethods.cs
- VarRefManager.cs
- SqlCaseSimplifier.cs
- CommandDesigner.cs
- CodeAccessPermission.cs
- FlowLayout.cs
- RelationshipNavigation.cs
- EntityDataSourceWrapper.cs
- MultiPropertyDescriptorGridEntry.cs
- FolderBrowserDialog.cs
- DateTimeConstantAttribute.cs
- PageContent.cs
- COM2FontConverter.cs
- RevocationPoint.cs
- CompositeDataBoundControl.cs
- IndentedTextWriter.cs
- XmlReader.cs
- ArcSegment.cs
- RegistryConfigurationProvider.cs
- WorkflowDebuggerSteppingAttribute.cs
- SqlServices.cs
- XmlSchemaElement.cs
- TextParagraphProperties.cs
- RenderOptions.cs
- ConsoleKeyInfo.cs
- XmlSerializationReader.cs
- StateRuntime.cs
- NamespaceList.cs
- DataServicePagingProviderWrapper.cs
- RecognizeCompletedEventArgs.cs
- RefreshPropertiesAttribute.cs
- MessageOperationFormatter.cs
- ReachPageContentCollectionSerializer.cs
- ExpressionDumper.cs
- ContentOperations.cs
- Axis.cs
- NotifyCollectionChangedEventArgs.cs
- BinaryObjectReader.cs
- ApplicationTrust.cs
- ValueExpressions.cs
- NullEntityWrapper.cs
- DataTableReader.cs
- ExpressionNode.cs
- EntryPointNotFoundException.cs
- SQLMoneyStorage.cs
- DataGridPageChangedEventArgs.cs
- CachingHintValidation.cs
- ComplexType.cs
- AggregateNode.cs
- Control.cs
- EntityDataSourceWizardForm.cs
- DocComment.cs
- CollectionBase.cs
- PointCollectionConverter.cs
- safePerfProviderHandle.cs
- FigureParaClient.cs
- TraceSource.cs
- SHA256.cs
- SafeFileMappingHandle.cs
- WindowsContainer.cs
- Route.cs
- CodeObject.cs
- MatrixTransform.cs
- Figure.cs
- DataGridViewCellMouseEventArgs.cs
- CLRBindingWorker.cs
- EntityCommandCompilationException.cs
- EndpointNameMessageFilter.cs
- ZoneIdentityPermission.cs
- ServiceDiscoveryBehavior.cs
- MediaContextNotificationWindow.cs
- DocumentViewerConstants.cs
- WebHeaderCollection.cs
- FunctionNode.cs
- SingleStorage.cs
- InlineUIContainer.cs
- NullRuntimeConfig.cs
- EvidenceBase.cs
- AsymmetricAlgorithm.cs
- ComboBoxAutomationPeer.cs
- Label.cs
- SizeAnimationClockResource.cs
- Point3DAnimation.cs
- Deflater.cs