Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Base / System / Collections / Specialized / NotifyCollectionChangedEventArgs.cs / 1 / NotifyCollectionChangedEventArgs.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: NotifyCollectionChanged event arguments // // Specs: [....]/connecteddata/Specs/INotifyCollectionChanged.mht // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Windows; using MS.Internal; // Invariant.Assert namespace System.Collections.Specialized { ////// This enum describes the action that caused a CollectionChanged event. /// public enum NotifyCollectionChangedAction { ///One or more items were added to the collection. Add, ///One or more items were removed from the collection. Remove, ///One or more items were replaced in the collection. Replace, ///One or more items were moved within the collection. Move, ///The contents of the collection changed dramatically. Reset, } ////// Arguments for the CollectionChanged event. /// A collection that supports INotifyCollectionChangedThis raises this event /// whenever an item is added or removed, or when the contents of the collection /// changes dramatically. /// public class NotifyCollectionChangedEventArgs : EventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// Construct a NotifyCollectionChangedEventArgs that describes a reset change. /// /// The action that caused the event (must be Reset). public NotifyCollectionChangedEventArgs(NotifyCollectionChangedAction action) { if (action != NotifyCollectionChangedAction.Reset) throw new ArgumentException(SR.Get(SRID.WrongActionForCtor, NotifyCollectionChangedAction.Reset), "action"); InitializeAdd(action, null, -1); } ////// Construct a NotifyCollectionChangedEventArgs that describes a one-item change. /// /// The action that caused the event; can only be Reset, Add or Remove action. /// The item affected by the change. public NotifyCollectionChangedEventArgs(NotifyCollectionChangedAction action, object changedItem) { if ((action != NotifyCollectionChangedAction.Add) && (action != NotifyCollectionChangedAction.Remove) && (action != NotifyCollectionChangedAction.Reset)) throw new ArgumentException(SR.Get(SRID.MustBeResetAddOrRemoveActionForCtor), "action"); if (action == NotifyCollectionChangedAction.Reset) { if (changedItem != null) throw new ArgumentException(SR.Get(SRID.ResetActionRequiresNullItem), "action"); InitializeAdd(action, null, -1); } else { InitializeAddOrRemove(action, new object[]{changedItem}, -1); } } ////// Construct a NotifyCollectionChangedEventArgs that describes a one-item change. /// /// The action that caused the event. /// The item affected by the change. /// The index where the change occurred. public NotifyCollectionChangedEventArgs(NotifyCollectionChangedAction action, object changedItem, int index) { if ((action != NotifyCollectionChangedAction.Add) && (action != NotifyCollectionChangedAction.Remove) && (action != NotifyCollectionChangedAction.Reset)) throw new ArgumentException(SR.Get(SRID.MustBeResetAddOrRemoveActionForCtor), "action"); if (action == NotifyCollectionChangedAction.Reset) { if (changedItem != null) throw new ArgumentException(SR.Get(SRID.ResetActionRequiresNullItem), "action"); if (index != -1) throw new ArgumentException(SR.Get(SRID.ResetActionRequiresIndexMinus1), "action"); InitializeAdd(action, null, -1); } else { InitializeAddOrRemove(action, new object[]{changedItem}, index); } } ////// Construct a NotifyCollectionChangedEventArgs that describes a multi-item change. /// /// The action that caused the event. /// The items affected by the change. public NotifyCollectionChangedEventArgs(NotifyCollectionChangedAction action, IList changedItems) { if ((action != NotifyCollectionChangedAction.Add) && (action != NotifyCollectionChangedAction.Remove) && (action != NotifyCollectionChangedAction.Reset)) throw new ArgumentException(SR.Get(SRID.MustBeResetAddOrRemoveActionForCtor), "action"); if (action == NotifyCollectionChangedAction.Reset) { if (changedItems != null) throw new ArgumentException(SR.Get(SRID.ResetActionRequiresNullItem), "action"); InitializeAdd(action, null, -1); } else { if (changedItems == null) throw new ArgumentNullException("changedItems"); InitializeAddOrRemove(action, changedItems, -1); } } ////// Construct a NotifyCollectionChangedEventArgs that describes a multi-item change (or a reset). /// /// The action that caused the event. /// The items affected by the change. /// The index where the change occurred. public NotifyCollectionChangedEventArgs(NotifyCollectionChangedAction action, IList changedItems, int startingIndex) { if ((action != NotifyCollectionChangedAction.Add) && (action != NotifyCollectionChangedAction.Remove) && (action != NotifyCollectionChangedAction.Reset)) throw new ArgumentException(SR.Get(SRID.MustBeResetAddOrRemoveActionForCtor), "action"); if (action == NotifyCollectionChangedAction.Reset) { if (changedItems != null) throw new ArgumentException(SR.Get(SRID.ResetActionRequiresNullItem), "action"); if (startingIndex != -1) throw new ArgumentException(SR.Get(SRID.ResetActionRequiresIndexMinus1), "action"); InitializeAdd(action, null, -1); } else { if (changedItems == null) throw new ArgumentNullException("changedItems"); if (startingIndex < -1) throw new ArgumentException(SR.Get(SRID.IndexCannotBeNegative), "startingIndex"); InitializeAddOrRemove(action, changedItems, startingIndex); } } ////// Construct a NotifyCollectionChangedEventArgs that describes a one-item Replace event. /// /// Can only be a Replace action. /// The new item replacing the original item. /// The original item that is replaced. public NotifyCollectionChangedEventArgs(NotifyCollectionChangedAction action, object newItem, object oldItem) { if (action != NotifyCollectionChangedAction.Replace) throw new ArgumentException(SR.Get(SRID.WrongActionForCtor, NotifyCollectionChangedAction.Replace), "action"); InitializeMoveOrReplace(action, new object[]{newItem}, new object[]{oldItem}, -1, -1); } ////// Construct a NotifyCollectionChangedEventArgs that describes a one-item Replace event. /// /// Can only be a Replace action. /// The new item replacing the original item. /// The original item that is replaced. /// The index of the item being replaced. public NotifyCollectionChangedEventArgs(NotifyCollectionChangedAction action, object newItem, object oldItem, int index) { if (action != NotifyCollectionChangedAction.Replace) throw new ArgumentException(SR.Get(SRID.WrongActionForCtor, NotifyCollectionChangedAction.Replace), "action"); InitializeMoveOrReplace(action, new object[]{newItem}, new object[]{oldItem}, index, index); } ////// Construct a NotifyCollectionChangedEventArgs that describes a multi-item Replace event. /// /// Can only be a Replace action. /// The new items replacing the original items. /// The original items that are replaced. public NotifyCollectionChangedEventArgs(NotifyCollectionChangedAction action, IList newItems, IList oldItems) { if (action != NotifyCollectionChangedAction.Replace) throw new ArgumentException(SR.Get(SRID.WrongActionForCtor, NotifyCollectionChangedAction.Replace), "action"); if (newItems == null) throw new ArgumentNullException("newItems"); if (oldItems == null) throw new ArgumentNullException("oldItems"); InitializeMoveOrReplace(action, newItems, oldItems, -1, -1); } ////// Construct a NotifyCollectionChangedEventArgs that describes a multi-item Replace event. /// /// Can only be a Replace action. /// The new items replacing the original items. /// The original items that are replaced. /// The starting index of the items being replaced. public NotifyCollectionChangedEventArgs(NotifyCollectionChangedAction action, IList newItems, IList oldItems, int startingIndex) { if (action != NotifyCollectionChangedAction.Replace) throw new ArgumentException(SR.Get(SRID.WrongActionForCtor, NotifyCollectionChangedAction.Replace), "action"); if (newItems == null) throw new ArgumentNullException("newItems"); if (oldItems == null) throw new ArgumentNullException("oldItems"); InitializeMoveOrReplace(action, newItems, oldItems, startingIndex, startingIndex); } ////// Construct a NotifyCollectionChangedEventArgs that describes a one-item Move event. /// /// Can only be a Move action. /// The item affected by the change. /// The new index for the changed item. /// The old index for the changed item. public NotifyCollectionChangedEventArgs(NotifyCollectionChangedAction action, object changedItem, int index, int oldIndex) { if (action != NotifyCollectionChangedAction.Move) throw new ArgumentException(SR.Get(SRID.WrongActionForCtor, NotifyCollectionChangedAction.Move), "action"); if (index < 0) throw new ArgumentException(SR.Get(SRID.IndexCannotBeNegative), "index"); object[] changedItems= new object[] {changedItem}; InitializeMoveOrReplace(action, changedItems, changedItems, index, oldIndex); } ////// Construct a NotifyCollectionChangedEventArgs that describes a multi-item Move event. /// /// The action that caused the event. /// The items affected by the change. /// The new index for the changed items. /// The old index for the changed items. public NotifyCollectionChangedEventArgs(NotifyCollectionChangedAction action, IList changedItems, int index, int oldIndex) { if (action != NotifyCollectionChangedAction.Move) throw new ArgumentException(SR.Get(SRID.WrongActionForCtor, NotifyCollectionChangedAction.Move), "action"); if (index < 0) throw new ArgumentException(SR.Get(SRID.IndexCannotBeNegative), "index"); InitializeMoveOrReplace(action, changedItems, changedItems, index, oldIndex); } private void InitializeAddOrRemove(NotifyCollectionChangedAction action, IList changedItems, int startingIndex) { if (action == NotifyCollectionChangedAction.Add) InitializeAdd(action, changedItems, startingIndex); else if (action == NotifyCollectionChangedAction.Remove) InitializeRemove(action, changedItems, startingIndex); else Invariant.Assert(false, "Unsupported action: {0}", action.ToString()); } private void InitializeAdd(NotifyCollectionChangedAction action, IList newItems, int newStartingIndex) { _action = action; _newItems = (newItems == null) ? null : ArrayList.ReadOnly(newItems); _newStartingIndex = newStartingIndex; } private void InitializeRemove(NotifyCollectionChangedAction action, IList oldItems, int oldStartingIndex) { _action = action; _oldItems = (oldItems == null) ? null : ArrayList.ReadOnly(oldItems); _oldStartingIndex= oldStartingIndex; } private void InitializeMoveOrReplace(NotifyCollectionChangedAction action, IList newItems, IList oldItems, int startingIndex, int oldStartingIndex) { InitializeAdd(action, newItems, startingIndex); InitializeRemove(action, oldItems, oldStartingIndex); } //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// The action that caused the event. /// public NotifyCollectionChangedAction Action { get { return _action; } } ////// The items affected by the change. /// public IList NewItems { get { return _newItems; } } ////// The old items affected by the change (for Replace events). /// public IList OldItems { get { return _oldItems; } } ////// The index where the change occurred. /// public int NewStartingIndex { get { return _newStartingIndex; } } ////// The old index where the change occurred (for Move events). /// public int OldStartingIndex { get { return _oldStartingIndex; } } //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ private NotifyCollectionChangedAction _action; private IList _newItems, _oldItems; private int _newStartingIndex = -1; private int _oldStartingIndex = -1; } ////// The delegate to use for handlers that receive the CollectionChanged event. /// public delegate void NotifyCollectionChangedEventHandler(object sender, NotifyCollectionChangedEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
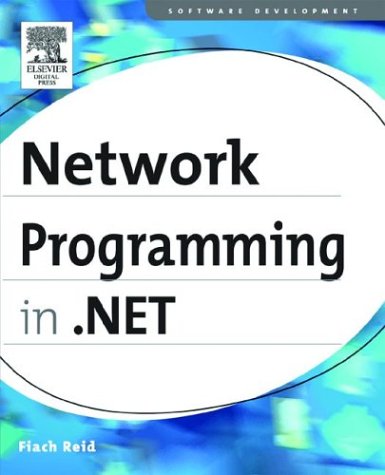
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Version.cs
- RewritingSimplifier.cs
- ToolboxItemImageConverter.cs
- DataGridColumnCollectionEditor.cs
- HtmlShimManager.cs
- DataBindEngine.cs
- RuntimeResourceSet.cs
- CreateUserWizardStep.cs
- AdapterUtil.cs
- Menu.cs
- ScaleTransform.cs
- ProbeDuplexCD1AsyncResult.cs
- RemotingConfigParser.cs
- DbProviderFactory.cs
- StrokeIntersection.cs
- TreeNodeCollectionEditor.cs
- FtpCachePolicyElement.cs
- ChildTable.cs
- DescendantOverDescendantQuery.cs
- TreeNode.cs
- NameNode.cs
- _SslStream.cs
- StoreItemCollection.cs
- EventRecordWrittenEventArgs.cs
- MetafileHeader.cs
- RewritingValidator.cs
- RepeatButtonAutomationPeer.cs
- XmlReader.cs
- XmlNodeList.cs
- PropertyBuilder.cs
- SqlTypesSchemaImporter.cs
- WebSysDefaultValueAttribute.cs
- AddInDeploymentState.cs
- CAGDesigner.cs
- ObjectConverter.cs
- ReceiveErrorHandling.cs
- ColorKeyFrameCollection.cs
- DrawingContextDrawingContextWalker.cs
- IssuanceLicense.cs
- SimpleHandlerFactory.cs
- AspNetSynchronizationContext.cs
- CompilerScope.cs
- HtmlButton.cs
- DataGridLinkButton.cs
- loginstatus.cs
- DataServiceBehavior.cs
- CompilerHelpers.cs
- ParamArrayAttribute.cs
- MonikerProxyAttribute.cs
- MetadataCache.cs
- ManagementOperationWatcher.cs
- SecurityImpersonationBehavior.cs
- DateTimeConstantAttribute.cs
- DataGridCaption.cs
- StrongNameUtility.cs
- ReferencedAssembly.cs
- DataGridParentRows.cs
- OdbcConnectionPoolProviderInfo.cs
- BitmapEffectInputData.cs
- ConfigXmlText.cs
- InvalidFilterCriteriaException.cs
- UserControlFileEditor.cs
- FilterRepeater.cs
- Update.cs
- ListSourceHelper.cs
- ServiceOperation.cs
- ProtocolsConfiguration.cs
- HttpListenerResponse.cs
- SchemaComplexType.cs
- CatalogPart.cs
- MarginsConverter.cs
- EventProvider.cs
- WebEvents.cs
- QueryReaderSettings.cs
- ErrorHandler.cs
- EncryptedReference.cs
- ListViewGroup.cs
- RC2.cs
- SQLDecimalStorage.cs
- FlagsAttribute.cs
- HexParser.cs
- ChangeToolStripParentVerb.cs
- XPathNodePointer.cs
- graph.cs
- BuiltInExpr.cs
- DriveNotFoundException.cs
- IsolatedStorageFile.cs
- TypeReference.cs
- GiveFeedbackEvent.cs
- ResourceType.cs
- Int32AnimationUsingKeyFrames.cs
- ChangeProcessor.cs
- ItemCheckEvent.cs
- SiteMapNode.cs
- ExecutionContext.cs
- MultilineStringEditor.cs
- FormatterServices.cs
- WrappingXamlSchemaContext.cs
- DesignRelationCollection.cs
- SqlRowUpdatedEvent.cs