Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Annotations / AnnotationResource.cs / 1 / AnnotationResource.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Resource represents a portion of content. It can refer to the content // or directly contain the content, or both. They are used to model anchors // and cargos of annotations. // // Spec: http://team/sites/ag/Specifications/Simplifying%20Store%20Cache%20Model.doc // // History: // 10/04/2002: rruiz: Added header comment to ObjectModel.cs // 07/03/2003: magedz: Renamed Link, LinkSequence to LocatorPart and Locator // respectively. // 05/31/2003: LGolding: Ported to WCP tree. // 07/15/2003: rruiz: Rewrote implementations to extend abstract classes // instead of implement interfaces; got rid of obsolete // classes; put each class in separate file. // 12/09/2003: ssimova: Added ResourceId // 6/30/2004: rruiz: Made the class concrete, updated the APIs to be more type // safe, made the class Xml serializable. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Annotations.Storage; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; using MS.Internal; using MS.Internal.Annotations; namespace System.Windows.Annotations { ////// Resource represents a portion of content. It can refer to the content /// or directly contain the content, or both. They are used to model anchors /// and cargos of annotations. /// [XmlRoot(Namespace = AnnotationXmlConstants.Namespaces.CoreSchemaNamespace, ElementName = AnnotationXmlConstants.Elements.Resource)] public sealed class AnnotationResource : IXmlSerializable, INotifyPropertyChanged2, IOwnedObject { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of Resource. /// public AnnotationResource() { _id = Guid.NewGuid(); } ////// Creates an instance of Resource with specified name. /// /// name used to distinguish this Resource /// from others in the same annotation; no validation is performed on the name ///name is null public AnnotationResource(string name) : this() { if (name == null) { throw new ArgumentNullException("name"); } _name = name; _id = Guid.NewGuid(); } ////// Creates an instance of Resource with the specified Guid as its Id. /// This constructor is for store implementations that use their own /// method of serialization and need a way to create a Resource with /// the correct read-only data. /// /// the new Resource's id ///id is equal to Guid.Empty public AnnotationResource(Guid id) { if (Guid.Empty.Equals(id)) throw new ArgumentException(SR.Get(SRID.InvalidGuid), "id"); // Guid is a struct and cannot be null _id = id; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods #region IXmlSerializable Implementation ////// Returns the null. The annotations schema can be found at /// http://schemas.microsoft.com/windows/annotations/2003/11/core. /// public XmlSchema GetSchema() { return null; } ////// Serializes this Resource to XML with the passed in XmlWriter. /// /// the writer to serialize the Resource to ///writer is null public void WriteXml(XmlWriter writer) { if (writer == null) { throw new ArgumentNullException("writer"); } if (String.IsNullOrEmpty(writer.LookupPrefix(AnnotationXmlConstants.Namespaces.CoreSchemaNamespace))) { writer.WriteAttributeString(AnnotationXmlConstants.Prefixes.XmlnsPrefix, AnnotationXmlConstants.Prefixes.CoreSchemaPrefix, null, AnnotationXmlConstants.Namespaces.CoreSchemaNamespace); } writer.WriteAttributeString(AnnotationXmlConstants.Attributes.Id, XmlConvert.ToString(_id)); if (_name != null) { writer.WriteAttributeString(AnnotationXmlConstants.Attributes.ResourceName, _name); } // Use the actual field here to avoid creating the collection for no reason if (_locators != null) { foreach (ContentLocatorBase locator in _locators) { if (locator != null) { if (locator is ContentLocatorGroup) { LocatorGroupSerializer.Serialize(writer, locator); } else { ListSerializer.Serialize(writer, locator); } } } } // Use the actual field here to avoid creating the collection for no reason if (_contents != null) { foreach (XmlElement content in _contents) { if (content != null) { content.WriteTo(writer); } } } } ////// Deserializes an Resource from the XmlReader passed in. /// /// reader to deserialize from ///reader is null public void ReadXml(XmlReader reader) { if (reader == null) { throw new ArgumentNullException("reader"); } XmlDocument doc = new XmlDocument(); ReadAttributes(reader); if (!reader.IsEmptyElement) { reader.Read(); // Reads the remainder of "Resource" start tag while (!(AnnotationXmlConstants.Elements.Resource == reader.LocalName && XmlNodeType.EndElement == reader.NodeType)) { if (AnnotationXmlConstants.Elements.ContentLocatorGroup == reader.LocalName) { ContentLocatorBase locator = (ContentLocatorBase)LocatorGroupSerializer.Deserialize(reader); InternalLocators.Add(locator); } else if (AnnotationXmlConstants.Elements.ContentLocator == reader.LocalName) { ContentLocatorBase locator = (ContentLocatorBase)ListSerializer.Deserialize(reader); InternalLocators.Add(locator); } else if (XmlNodeType.Element == reader.NodeType) { XmlElement element = doc.ReadNode(reader) as XmlElement; InternalContents.Add(element); } else { // The resource must contain a non-XmlElement child such as plain // text which is not part of the schema. throw new XmlException(SR.Get(SRID.InvalidXmlContent, AnnotationXmlConstants.Elements.Resource)); } } } reader.Read(); // Reads the end of the "Resource" element (or whole element if empty) } #endregion IXmlSerializable Implementation #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ #region Public Events ////// /// event PropertyChangedEventHandler INotifyPropertyChanged.PropertyChanged { add{ _propertyChanged += value; } remove{ _propertyChanged -= value; } } #endregion Public Events //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- #region Public Properties ////// A Resource is given a unique Guid when it is first instantiated. /// ///the unique id of this Resource; this property will return an /// invalid Guid if the Resource was instantied with the default constructor - /// which should not be used directly public Guid Id { get { return _id; } } ////// The name given this Resource to distinguish it from other anchors or /// cargos in an annotation. /// ///the name of this resource; can be null public string Name { get { return _name; } set { bool changed = false; if (_name == null) { if (value != null) { changed = true; } } else if (!_name.Equals(value)) { changed = true; } _name = value; if (changed) { FireResourceChanged("Name"); } } } ////// Collection of zero or more Locators in this Resource. /// ///collection of Locators; never returns null public CollectionContentLocators { get { return InternalLocators; } } /// /// Collection of zero or more XmlElements representing the /// contents of this Resource. /// ///collection of XmlElements which are the contents of /// this resource; never returns null public CollectionContents { get { return InternalContents; } } #endregion Public Properties //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties bool IOwnedObject.Owned { get { return _owned; } set { _owned = value; } } /// /// Returns serializer for ContentLocator objects. Lazily /// creates the serializer for cases where its not needed. /// The property is internal so that other classes (ContentLocatorGroup) /// has access to the same serializer. /// internal static Serializer ListSerializer { get { if (s_ListSerializer == null) { s_ListSerializer = new Serializer(typeof(ContentLocator)); } return s_ListSerializer; } } #endregion Internal Properties //----------------------------------------------------- // // Private Properties // //------------------------------------------------------ #region Private Properties ////// Returns the list of locators - used internally to /// lazily create the list when its needed. /// private AnnotationObservableCollectionInternalLocators { get { if (_locators == null) { _locators = new AnnotationObservableCollection (); _locators.CollectionChanged += OnLocatorsChanged; } return _locators; } } /// /// Returns the list of contents - used internally to /// lazily create the list when its needed. /// private XmlElementCollection InternalContents { get { if (_contents == null) { _contents = new XmlElementCollection(); _contents.CollectionChanged += OnContentsChanged; } return _contents; } } ////// Returns serializer for ContentLocatorGroup objects. Lazily creates /// the serializer for cases where its not needed. /// private static Serializer LocatorGroupSerializer { get { if (s_LocatorGroupSerializer == null) { s_LocatorGroupSerializer = new Serializer(typeof(ContentLocatorGroup)); } return s_LocatorGroupSerializer; } } #endregion Private Properties //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods ////// Reads all attributes for the "Resource" element and throws /// appropriate exceptions if any required attributes are missing /// or unexpected attributes are found /// private void ReadAttributes(XmlReader reader) { Invariant.Assert(reader != null, "No reader passed in."); // Use a temporary variable to determine if a Guid value // was actually provided. The member variable is set in // the default ctor and can't be relied on for this. Guid tempId = Guid.Empty; // Read all the attributes while (reader.MoveToNextAttribute()) { string value = reader.Value; // Skip null values - they will be treated the same as if // they weren't specified at all if (value == null) continue; switch (reader.LocalName) { case AnnotationXmlConstants.Attributes.Id: tempId = XmlConvert.ToGuid(value); break; case AnnotationXmlConstants.Attributes.ResourceName: _name = value; break; default: if (!Annotation.IsNamespaceDeclaration(reader)) throw new XmlException(SR.Get(SRID.UnexpectedAttribute, reader.LocalName, AnnotationXmlConstants.Elements.Resource)); break; } } if (Guid.Empty.Equals(tempId)) { throw new XmlException(SR.Get(SRID.RequiredAttributeMissing, AnnotationXmlConstants.Attributes.Id, AnnotationXmlConstants.Elements.Resource)); } _id = tempId; // Name attribute is optional, so no need to check it // Move back to the parent "Resource" element reader.MoveToContent(); } ////// Listens for change events from the list of locators. Fires a change event /// for this resource when an event is received. /// private void OnLocatorsChanged(object sender, NotifyCollectionChangedEventArgs e) { FireResourceChanged("Locators"); } ////// Listens for change events from the list of contents. Fires a change event /// for this resource when an event is received. /// private void OnContentsChanged(object sender, NotifyCollectionChangedEventArgs e) { FireResourceChanged("Contents"); } ////// Fires a change notification to the Annotation that contains /// this Resource. /// private void FireResourceChanged(string name) { if (_propertyChanged != null) { _propertyChanged(this, new System.ComponentModel.PropertyChangedEventArgs(name)); } } #endregion Private Methods //------------------------------------------------------ // // 2Private Fields // //----------------------------------------------------- #region Private Fields ////// Unique ID for this resource /// private Guid _id; ////// Name given this resource by the developer. Used to differentiate /// between multiple resources on an annotation. /// private string _name; ////// List of locators that refer to the data of this resource /// private AnnotationObservableCollection_locators; /// /// List of instances of the data for this resource /// private XmlElementCollection _contents; ////// Serializer we use for serializing and deserializing Locators. /// private static Serializer s_ListSerializer; ////// Serializer we use for serializing and deserializing LocatorGroups. /// private static Serializer s_LocatorGroupSerializer; ////// /// private bool _owned; ////// /// private PropertyChangedEventHandler _propertyChanged; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Resource represents a portion of content. It can refer to the content // or directly contain the content, or both. They are used to model anchors // and cargos of annotations. // // Spec: http://team/sites/ag/Specifications/Simplifying%20Store%20Cache%20Model.doc // // History: // 10/04/2002: rruiz: Added header comment to ObjectModel.cs // 07/03/2003: magedz: Renamed Link, LinkSequence to LocatorPart and Locator // respectively. // 05/31/2003: LGolding: Ported to WCP tree. // 07/15/2003: rruiz: Rewrote implementations to extend abstract classes // instead of implement interfaces; got rid of obsolete // classes; put each class in separate file. // 12/09/2003: ssimova: Added ResourceId // 6/30/2004: rruiz: Made the class concrete, updated the APIs to be more type // safe, made the class Xml serializable. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Annotations.Storage; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; using MS.Internal; using MS.Internal.Annotations; namespace System.Windows.Annotations { ////// Resource represents a portion of content. It can refer to the content /// or directly contain the content, or both. They are used to model anchors /// and cargos of annotations. /// [XmlRoot(Namespace = AnnotationXmlConstants.Namespaces.CoreSchemaNamespace, ElementName = AnnotationXmlConstants.Elements.Resource)] public sealed class AnnotationResource : IXmlSerializable, INotifyPropertyChanged2, IOwnedObject { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of Resource. /// public AnnotationResource() { _id = Guid.NewGuid(); } ////// Creates an instance of Resource with specified name. /// /// name used to distinguish this Resource /// from others in the same annotation; no validation is performed on the name ///name is null public AnnotationResource(string name) : this() { if (name == null) { throw new ArgumentNullException("name"); } _name = name; _id = Guid.NewGuid(); } ////// Creates an instance of Resource with the specified Guid as its Id. /// This constructor is for store implementations that use their own /// method of serialization and need a way to create a Resource with /// the correct read-only data. /// /// the new Resource's id ///id is equal to Guid.Empty public AnnotationResource(Guid id) { if (Guid.Empty.Equals(id)) throw new ArgumentException(SR.Get(SRID.InvalidGuid), "id"); // Guid is a struct and cannot be null _id = id; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods #region IXmlSerializable Implementation ////// Returns the null. The annotations schema can be found at /// http://schemas.microsoft.com/windows/annotations/2003/11/core. /// public XmlSchema GetSchema() { return null; } ////// Serializes this Resource to XML with the passed in XmlWriter. /// /// the writer to serialize the Resource to ///writer is null public void WriteXml(XmlWriter writer) { if (writer == null) { throw new ArgumentNullException("writer"); } if (String.IsNullOrEmpty(writer.LookupPrefix(AnnotationXmlConstants.Namespaces.CoreSchemaNamespace))) { writer.WriteAttributeString(AnnotationXmlConstants.Prefixes.XmlnsPrefix, AnnotationXmlConstants.Prefixes.CoreSchemaPrefix, null, AnnotationXmlConstants.Namespaces.CoreSchemaNamespace); } writer.WriteAttributeString(AnnotationXmlConstants.Attributes.Id, XmlConvert.ToString(_id)); if (_name != null) { writer.WriteAttributeString(AnnotationXmlConstants.Attributes.ResourceName, _name); } // Use the actual field here to avoid creating the collection for no reason if (_locators != null) { foreach (ContentLocatorBase locator in _locators) { if (locator != null) { if (locator is ContentLocatorGroup) { LocatorGroupSerializer.Serialize(writer, locator); } else { ListSerializer.Serialize(writer, locator); } } } } // Use the actual field here to avoid creating the collection for no reason if (_contents != null) { foreach (XmlElement content in _contents) { if (content != null) { content.WriteTo(writer); } } } } ////// Deserializes an Resource from the XmlReader passed in. /// /// reader to deserialize from ///reader is null public void ReadXml(XmlReader reader) { if (reader == null) { throw new ArgumentNullException("reader"); } XmlDocument doc = new XmlDocument(); ReadAttributes(reader); if (!reader.IsEmptyElement) { reader.Read(); // Reads the remainder of "Resource" start tag while (!(AnnotationXmlConstants.Elements.Resource == reader.LocalName && XmlNodeType.EndElement == reader.NodeType)) { if (AnnotationXmlConstants.Elements.ContentLocatorGroup == reader.LocalName) { ContentLocatorBase locator = (ContentLocatorBase)LocatorGroupSerializer.Deserialize(reader); InternalLocators.Add(locator); } else if (AnnotationXmlConstants.Elements.ContentLocator == reader.LocalName) { ContentLocatorBase locator = (ContentLocatorBase)ListSerializer.Deserialize(reader); InternalLocators.Add(locator); } else if (XmlNodeType.Element == reader.NodeType) { XmlElement element = doc.ReadNode(reader) as XmlElement; InternalContents.Add(element); } else { // The resource must contain a non-XmlElement child such as plain // text which is not part of the schema. throw new XmlException(SR.Get(SRID.InvalidXmlContent, AnnotationXmlConstants.Elements.Resource)); } } } reader.Read(); // Reads the end of the "Resource" element (or whole element if empty) } #endregion IXmlSerializable Implementation #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ #region Public Events ////// /// event PropertyChangedEventHandler INotifyPropertyChanged.PropertyChanged { add{ _propertyChanged += value; } remove{ _propertyChanged -= value; } } #endregion Public Events //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- #region Public Properties ////// A Resource is given a unique Guid when it is first instantiated. /// ///the unique id of this Resource; this property will return an /// invalid Guid if the Resource was instantied with the default constructor - /// which should not be used directly public Guid Id { get { return _id; } } ////// The name given this Resource to distinguish it from other anchors or /// cargos in an annotation. /// ///the name of this resource; can be null public string Name { get { return _name; } set { bool changed = false; if (_name == null) { if (value != null) { changed = true; } } else if (!_name.Equals(value)) { changed = true; } _name = value; if (changed) { FireResourceChanged("Name"); } } } ////// Collection of zero or more Locators in this Resource. /// ///collection of Locators; never returns null public CollectionContentLocators { get { return InternalLocators; } } /// /// Collection of zero or more XmlElements representing the /// contents of this Resource. /// ///collection of XmlElements which are the contents of /// this resource; never returns null public CollectionContents { get { return InternalContents; } } #endregion Public Properties //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties bool IOwnedObject.Owned { get { return _owned; } set { _owned = value; } } /// /// Returns serializer for ContentLocator objects. Lazily /// creates the serializer for cases where its not needed. /// The property is internal so that other classes (ContentLocatorGroup) /// has access to the same serializer. /// internal static Serializer ListSerializer { get { if (s_ListSerializer == null) { s_ListSerializer = new Serializer(typeof(ContentLocator)); } return s_ListSerializer; } } #endregion Internal Properties //----------------------------------------------------- // // Private Properties // //------------------------------------------------------ #region Private Properties ////// Returns the list of locators - used internally to /// lazily create the list when its needed. /// private AnnotationObservableCollectionInternalLocators { get { if (_locators == null) { _locators = new AnnotationObservableCollection (); _locators.CollectionChanged += OnLocatorsChanged; } return _locators; } } /// /// Returns the list of contents - used internally to /// lazily create the list when its needed. /// private XmlElementCollection InternalContents { get { if (_contents == null) { _contents = new XmlElementCollection(); _contents.CollectionChanged += OnContentsChanged; } return _contents; } } ////// Returns serializer for ContentLocatorGroup objects. Lazily creates /// the serializer for cases where its not needed. /// private static Serializer LocatorGroupSerializer { get { if (s_LocatorGroupSerializer == null) { s_LocatorGroupSerializer = new Serializer(typeof(ContentLocatorGroup)); } return s_LocatorGroupSerializer; } } #endregion Private Properties //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods ////// Reads all attributes for the "Resource" element and throws /// appropriate exceptions if any required attributes are missing /// or unexpected attributes are found /// private void ReadAttributes(XmlReader reader) { Invariant.Assert(reader != null, "No reader passed in."); // Use a temporary variable to determine if a Guid value // was actually provided. The member variable is set in // the default ctor and can't be relied on for this. Guid tempId = Guid.Empty; // Read all the attributes while (reader.MoveToNextAttribute()) { string value = reader.Value; // Skip null values - they will be treated the same as if // they weren't specified at all if (value == null) continue; switch (reader.LocalName) { case AnnotationXmlConstants.Attributes.Id: tempId = XmlConvert.ToGuid(value); break; case AnnotationXmlConstants.Attributes.ResourceName: _name = value; break; default: if (!Annotation.IsNamespaceDeclaration(reader)) throw new XmlException(SR.Get(SRID.UnexpectedAttribute, reader.LocalName, AnnotationXmlConstants.Elements.Resource)); break; } } if (Guid.Empty.Equals(tempId)) { throw new XmlException(SR.Get(SRID.RequiredAttributeMissing, AnnotationXmlConstants.Attributes.Id, AnnotationXmlConstants.Elements.Resource)); } _id = tempId; // Name attribute is optional, so no need to check it // Move back to the parent "Resource" element reader.MoveToContent(); } ////// Listens for change events from the list of locators. Fires a change event /// for this resource when an event is received. /// private void OnLocatorsChanged(object sender, NotifyCollectionChangedEventArgs e) { FireResourceChanged("Locators"); } ////// Listens for change events from the list of contents. Fires a change event /// for this resource when an event is received. /// private void OnContentsChanged(object sender, NotifyCollectionChangedEventArgs e) { FireResourceChanged("Contents"); } ////// Fires a change notification to the Annotation that contains /// this Resource. /// private void FireResourceChanged(string name) { if (_propertyChanged != null) { _propertyChanged(this, new System.ComponentModel.PropertyChangedEventArgs(name)); } } #endregion Private Methods //------------------------------------------------------ // // 2Private Fields // //----------------------------------------------------- #region Private Fields ////// Unique ID for this resource /// private Guid _id; ////// Name given this resource by the developer. Used to differentiate /// between multiple resources on an annotation. /// private string _name; ////// List of locators that refer to the data of this resource /// private AnnotationObservableCollection_locators; /// /// List of instances of the data for this resource /// private XmlElementCollection _contents; ////// Serializer we use for serializing and deserializing Locators. /// private static Serializer s_ListSerializer; ////// Serializer we use for serializing and deserializing LocatorGroups. /// private static Serializer s_LocatorGroupSerializer; ////// /// private bool _owned; ////// /// private PropertyChangedEventHandler _propertyChanged; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
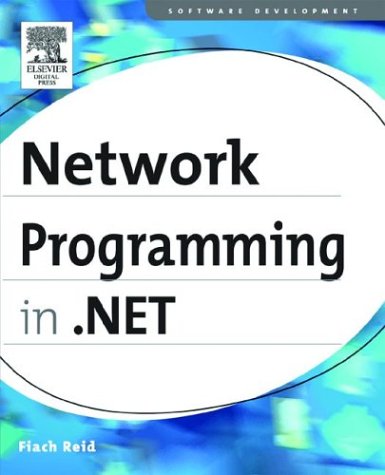
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- CfgRule.cs
- WebPartZone.cs
- WebConfigurationManager.cs
- Timer.cs
- UInt16Storage.cs
- ThicknessAnimationBase.cs
- HttpListenerPrefixCollection.cs
- DateTimePicker.cs
- ToolStripDropTargetManager.cs
- ZipPackage.cs
- OdbcHandle.cs
- EncoderBestFitFallback.cs
- Addressing.cs
- MultiBindingExpression.cs
- WebPartMenu.cs
- _SpnDictionary.cs
- CodeMemberMethod.cs
- ImageSourceValueSerializer.cs
- ContentType.cs
- ManipulationVelocities.cs
- TcpStreams.cs
- SpecularMaterial.cs
- DateTimeFormat.cs
- TextCompositionManager.cs
- XmlC14NWriter.cs
- ResourceManager.cs
- LinkedDataMemberFieldEditor.cs
- SqlConnectionHelper.cs
- X509ChainPolicy.cs
- XPathAxisIterator.cs
- SingleBodyParameterMessageFormatter.cs
- SymmetricKeyWrap.cs
- RangeBase.cs
- WsatConfiguration.cs
- OracleEncoding.cs
- StackSpiller.cs
- Lock.cs
- TraceSection.cs
- ProgramNode.cs
- RemotingAttributes.cs
- HeaderFilter.cs
- translator.cs
- FixedBufferAttribute.cs
- ConfigurationProperty.cs
- SelectManyQueryOperator.cs
- CqlQuery.cs
- GeometryHitTestResult.cs
- FileCodeGroup.cs
- EntityWithChangeTrackerStrategy.cs
- CellQuery.cs
- WebControlParameterProxy.cs
- XmlAttribute.cs
- EntityCollection.cs
- HijriCalendar.cs
- StructuredProperty.cs
- TraceHandlerErrorFormatter.cs
- XPathNavigatorReader.cs
- EncodingDataItem.cs
- input.cs
- HttpSessionStateWrapper.cs
- InputScopeManager.cs
- MinMaxParagraphWidth.cs
- ConfigurationManagerHelper.cs
- CustomValidator.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- MexTcpBindingCollectionElement.cs
- TableCell.cs
- PatternMatcher.cs
- Triplet.cs
- List.cs
- PartitionResolver.cs
- OracleInfoMessageEventArgs.cs
- SynchronizationContext.cs
- GeneratedCodeAttribute.cs
- ImpersonateTokenRef.cs
- HtmlInputCheckBox.cs
- FixedElement.cs
- DynamicHyperLink.cs
- BasicExpressionVisitor.cs
- TraceEventCache.cs
- OdbcErrorCollection.cs
- OpenFileDialog.cs
- MediaPlayerState.cs
- MatrixCamera.cs
- NamespaceExpr.cs
- EventToken.cs
- ClientUtils.cs
- Compilation.cs
- Win32Exception.cs
- WCFModelStrings.Designer.cs
- Rule.cs
- ContainerUIElement3D.cs
- Assembly.cs
- BitmapSource.cs
- oledbmetadatacolumnnames.cs
- InkCanvasAutomationPeer.cs
- WebHttpSecurity.cs
- _LocalDataStoreMgr.cs
- KnownBoxes.cs