Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / Compilation / WCFModel / ExternalFile.cs / 1305376 / ExternalFile.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- // // This code is shared between ndp\fx\src\xsp\System\Web\Extensions\Compilation\WCFModel // and wizard\vsdesigner\designer\microsoft\vsdesigner\WCFModel. // Please make sure the code files between those two directories are alway in [....] when you make any changes to this code. // And always test these code in both places before check in. // The code under ndp\fx\src\xsp\System\Web\Extensions\Compilation\XmlSerializer might have to be regerenated when // the format of the svcmap file is changed, or class structure has been changed in this directory. Please follow the HowTo file // under Compilation directory to see how to regerenate that code. // using System; using System.Collections; using System.Diagnostics; using System.Globalization; using System.IO; #if WEB_EXTENSIONS_CODE using System.Web.Resources; #else using Microsoft.VSDesigner.Resources.Microsoft.VSDesigner; #endif using XmlSerialization = System.Xml.Serialization; #if WEB_EXTENSIONS_CODE namespace System.Web.Compilation.WCFModel #else namespace Microsoft.VSDesigner.WCFModel #endif { ////// This class presents a single file referenced by a svcmap file /// ///#if WEB_EXTENSIONS_CODE internal class ExternalFile #else [CLSCompliant(true)] public class ExternalFile #endif { // File Name private string m_FileName; // Is the MeatadataFile loaded from the file? If it is false, we need create a new file when we save to the disket private bool m_IsExistingFile; // error happens when the file is loaded private Exception m_ErrorInLoading; /// /// Constructor /// ///Must support a default construct for XmlSerializer public ExternalFile() { m_FileName = String.Empty; } ////// Constructor /// /// File Name public ExternalFile(string fileName) { this.FileName = fileName; } ////// Error happens when the file is loaded /// ////// [XmlSerialization.XmlIgnore()] public Exception ErrorInLoading { get { return m_ErrorInLoading; } set { m_ErrorInLoading = value; } } /// /// FileName in the storage /// ////// [XmlSerialization.XmlAttribute()] public string FileName { get { return m_FileName; } set { if (value == null) { throw new ArgumentNullException("value"); } if (!IsLocalFileName(value)) { throw new NotSupportedException(String.Format(CultureInfo.CurrentCulture, WCFModelStrings.ReferenceGroup_InvalidFileName, value)); } m_FileName = value; } } /// /// Is the item loaded from the file? If it is false, we need create a new file when we save to the disket /// ////// [XmlSerialization.XmlIgnore()] public bool IsExistingFile { get { return m_IsExistingFile; } set { m_IsExistingFile = value; } } /// /// Check the file name is a real file name but not a path /// /// ///public static bool IsLocalFileName(string fileName) { if (fileName == null) { throw new ArgumentNullException("fileName"); } if (fileName.IndexOfAny(Path.GetInvalidFileNameChars()) >= 0 || fileName.IndexOfAny(new Char[] { Path.DirectorySeparatorChar, Path.AltDirectorySeparatorChar, Path.VolumeSeparatorChar }) >= 0) { return false; } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
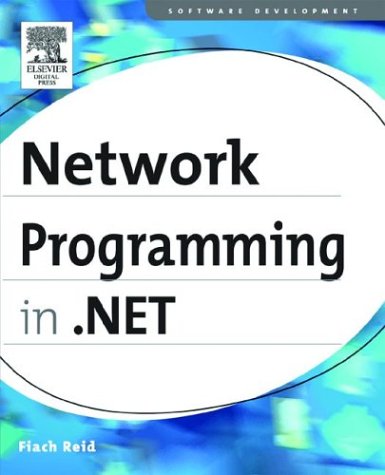
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplatePropertyEntry.cs
- MULTI_QI.cs
- CheckoutException.cs
- SizeValueSerializer.cs
- WindowsEditBoxRange.cs
- X509Certificate2Collection.cs
- ObjectReaderCompiler.cs
- NamespaceEmitter.cs
- RandomNumberGenerator.cs
- NonBatchDirectoryCompiler.cs
- XmlChoiceIdentifierAttribute.cs
- MissingFieldException.cs
- ArrayConverter.cs
- EndpointDispatcherTable.cs
- PipelineModuleStepContainer.cs
- SafeRsaProviderHandle.cs
- Duration.cs
- CacheAxisQuery.cs
- Size.cs
- RoleManagerSection.cs
- FormClosedEvent.cs
- CookieHandler.cs
- TableColumnCollection.cs
- _CookieModule.cs
- CollectionViewProxy.cs
- CodeNamespaceCollection.cs
- StringCollection.cs
- IdnElement.cs
- InternalsVisibleToAttribute.cs
- BaseProcessor.cs
- URLIdentityPermission.cs
- HtmlEncodedRawTextWriter.cs
- CheckoutException.cs
- DataGridViewAddColumnDialog.cs
- CodeNamespaceImport.cs
- PageAsyncTask.cs
- TimeSpanConverter.cs
- DataPager.cs
- ProxyWebPartManager.cs
- ContainerFilterService.cs
- BrowserCapabilitiesCodeGenerator.cs
- EditorAttributeInfo.cs
- DesignerValidatorAdapter.cs
- FramingFormat.cs
- SymbolDocumentInfo.cs
- SoapSchemaImporter.cs
- ColumnResizeAdorner.cs
- XmlWhitespace.cs
- UnknownBitmapDecoder.cs
- OpCodes.cs
- coordinatorscratchpad.cs
- OdbcInfoMessageEvent.cs
- RegistryPermission.cs
- DragStartedEventArgs.cs
- XamlFigureLengthSerializer.cs
- InputLanguage.cs
- ContentValidator.cs
- XmlText.cs
- Int16KeyFrameCollection.cs
- StringInfo.cs
- RegularExpressionValidator.cs
- PropertyItem.cs
- GB18030Encoding.cs
- HttpRequestCacheValidator.cs
- StrongNameMembershipCondition.cs
- KerberosReceiverSecurityToken.cs
- TextMarkerSource.cs
- RawStylusSystemGestureInputReport.cs
- DataObjectMethodAttribute.cs
- Size3D.cs
- FileSystemEnumerable.cs
- NetMsmqSecurity.cs
- SQLMembershipProvider.cs
- ReferenceSchema.cs
- DataGridViewHeaderCell.cs
- SHA1.cs
- ClipboardProcessor.cs
- BaseUriHelper.cs
- MatchingStyle.cs
- RestHandlerFactory.cs
- Package.cs
- sqlstateclientmanager.cs
- Scheduler.cs
- Pair.cs
- Application.cs
- XpsResource.cs
- AttachedAnnotation.cs
- BatchServiceHost.cs
- WindowInteropHelper.cs
- ColumnHeaderConverter.cs
- BufferedConnection.cs
- RadioButtonRenderer.cs
- AuthorizationRuleCollection.cs
- EncryptedReference.cs
- BufferedGraphicsContext.cs
- UserNameSecurityTokenAuthenticator.cs
- DelegatedStream.cs
- ListViewTableRow.cs
- DesignerOptionService.cs
- AspNetHostingPermission.cs