Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Windows / Size.cs / 1305600 / Size.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001, 2002 // // File: Size.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Text; using System.Collections; using System.Globalization; using System.Windows; using System.Windows.Media; using System.Runtime.InteropServices; using MS.Internal.WindowsBase; namespace System.Windows { ////// Size - A value type which defined a size in terms of non-negative width and height /// public partial struct Size { #region Constructors ////// Constructor which sets the size's initial values. Width and Height must be non-negative /// /// double - The initial Width /// double - THe initial Height public Size(double width, double height) { if (width < 0 || height < 0) { throw new System.ArgumentException(SR.Get(SRID.Size_WidthAndHeightCannotBeNegative)); } _width = width; _height = height; } #endregion Constructors #region Statics ////// Empty - a static property which provides an Empty size. Width and Height are /// negative-infinity. This is the only situation /// where size can be negative. /// public static Size Empty { get { return s_empty; } } #endregion Statics #region Public Methods and Properties ////// IsEmpty - this returns true if this size is the Empty size. /// Note: If size is 0 this Size still contains a 0 or 1 dimensional set /// of points, so this method should not be used to check for 0 area. /// public bool IsEmpty { get { return _width < 0; } } ////// Width - Default is 0, must be non-negative /// public double Width { get { return _width; } set { if (IsEmpty) { throw new System.InvalidOperationException(SR.Get(SRID.Size_CannotModifyEmptySize)); } if (value < 0) { throw new System.ArgumentException(SR.Get(SRID.Size_WidthCannotBeNegative)); } _width = value; } } ////// Height - Default is 0, must be non-negative. /// public double Height { get { return _height; } set { if (IsEmpty) { throw new System.InvalidOperationException(SR.Get(SRID.Size_CannotModifyEmptySize)); } if (value < 0) { throw new System.ArgumentException(SR.Get(SRID.Size_HeightCannotBeNegative)); } _height = value; } } #endregion Public Methods #region Public Operators ////// Explicit conversion to Vector. /// ////// Vector - A Vector equal to this Size /// /// Size - the Size to convert to a Vector public static explicit operator Vector(Size size) { return new Vector(size._width, size._height); } ////// Explicit conversion to Point /// ////// Point - A Point equal to this Size /// /// Size - the Size to convert to a Point public static explicit operator Point(Size size) { return new Point(size._width, size._height); } #endregion Public Operators #region Private Methods static private Size CreateEmptySize() { Size size = new Size(); // We can't set these via the property setters because negatives widths // are rejected in those APIs. size._width = Double.NegativeInfinity; size._height = Double.NegativeInfinity; return size; } #endregion Private Methods #region Private Fields private readonly static Size s_empty = CreateEmptySize(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
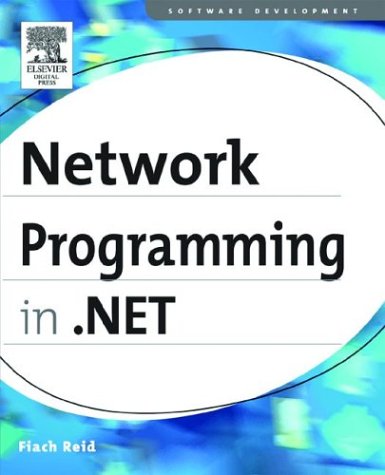
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SoapConverter.cs
- DataBoundControlParameterTarget.cs
- Brushes.cs
- ListBindableAttribute.cs
- Duration.cs
- DataErrorValidationRule.cs
- DemultiplexingClientMessageFormatter.cs
- DynamicScriptObject.cs
- MiniParameterInfo.cs
- FileSecurity.cs
- DeclaredTypeElementCollection.cs
- TreeBuilderBamlTranslator.cs
- EventLogPermissionHolder.cs
- IisTraceWebEventProvider.cs
- WindowsImpersonationContext.cs
- AccessDataSourceView.cs
- StyleSelector.cs
- HttpListenerContext.cs
- EllipseGeometry.cs
- ListViewGroupItemCollection.cs
- SingleResultAttribute.cs
- AsyncResult.cs
- PolicyUnit.cs
- SupportingTokenChannel.cs
- Vertex.cs
- XPathDocumentNavigator.cs
- Query.cs
- DSACryptoServiceProvider.cs
- UnsafeNativeMethods.cs
- SafeCryptoHandles.cs
- RoutedEventValueSerializer.cs
- AnimationStorage.cs
- EntityObject.cs
- XPathNodeIterator.cs
- MembershipValidatePasswordEventArgs.cs
- TextOptionsInternal.cs
- DataGridViewTopRowAccessibleObject.cs
- RowToFieldTransformer.cs
- Storyboard.cs
- EntityDataSourceConfigureObjectContext.cs
- SHA384Managed.cs
- AppDomainShutdownMonitor.cs
- DisplayNameAttribute.cs
- AggregatePushdown.cs
- XmlBinaryReader.cs
- CompilerGeneratedAttribute.cs
- PreloadedPackages.cs
- ContentElementAutomationPeer.cs
- CheckBoxAutomationPeer.cs
- LinearQuaternionKeyFrame.cs
- FileLogRecord.cs
- objectquery_tresulttype.cs
- NetworkAddressChange.cs
- CalendarAutoFormat.cs
- SessionStateSection.cs
- HMACRIPEMD160.cs
- XNodeNavigator.cs
- XPathDescendantIterator.cs
- ComponentChangingEvent.cs
- ActivityDesignerHelper.cs
- HMACSHA1.cs
- FilteredAttributeCollection.cs
- TextElement.cs
- XmlName.cs
- GetPageNumberCompletedEventArgs.cs
- Camera.cs
- DefaultTypeArgumentAttribute.cs
- Version.cs
- SchemaComplexType.cs
- ActivityExecutorOperation.cs
- OutArgumentConverter.cs
- TextElement.cs
- CollectionViewGroupInternal.cs
- BitHelper.cs
- DynamicPropertyReader.cs
- TextBlock.cs
- AuthenticationConfig.cs
- XmlName.cs
- DecimalKeyFrameCollection.cs
- base64Transforms.cs
- WebZoneDesigner.cs
- XmlSchemaSimpleTypeUnion.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- ElasticEase.cs
- SafeWaitHandle.cs
- CommentEmitter.cs
- TemplateBamlTreeBuilder.cs
- StyleXamlParser.cs
- ProfileService.cs
- ConfigXmlAttribute.cs
- ToolboxCategory.cs
- MemberInfoSerializationHolder.cs
- WindowsRichEditRange.cs
- XPathSelfQuery.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- ToolStripCodeDomSerializer.cs
- CardSpaceSelector.cs
- RemotingConfiguration.cs
- AlphabetConverter.cs
- DiagnosticsConfigurationHandler.cs