Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / InArgument.cs / 1305376 / InArgument.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities { using System; using System.Activities.Expressions; using System.Activities.Runtime; using System.Activities.XamlIntegration; using System.Collections.Generic; using System.ComponentModel; using System.Linq.Expressions; using System.Runtime; using System.Windows.Markup; using System.Diagnostics.CodeAnalysis; public abstract class InArgument : Argument { internal InArgument() : base() { this.Direction = ArgumentDirection.In; } [SuppressMessage(FxCop.Category.Design, FxCop.Rule.ConsiderPassingBaseTypesAsParameters, Justification = "Subclass needed to enforce rules about which directions can be referenced.")] public static InArgument CreateReference(InArgument argumentToReference, string referencedArgumentName) { if (argumentToReference == null) { throw FxTrace.Exception.ArgumentNull("argumentToReference"); } if (string.IsNullOrEmpty(referencedArgumentName)) { throw FxTrace.Exception.ArgumentNullOrEmpty("referencedArgumentName"); } return (InArgument)ActivityUtilities.CreateReferenceArgument(argumentToReference.ArgumentType, ArgumentDirection.In, referencedArgumentName); } [SuppressMessage(FxCop.Category.Design, FxCop.Rule.ConsiderPassingBaseTypesAsParameters, Justification = "Subclass needed to enforce rules about which directions can be referenced.")] public static InArgument CreateReference(InOutArgument argumentToReference, string referencedArgumentName) { if (argumentToReference == null) { throw FxTrace.Exception.ArgumentNull("argumentToReference"); } if (string.IsNullOrEmpty(referencedArgumentName)) { throw FxTrace.Exception.ArgumentNullOrEmpty("referencedArgumentName"); } // Note that we explicitly pass In since we want an InArgument created return (InArgument)ActivityUtilities.CreateReferenceArgument(argumentToReference.ArgumentType, ArgumentDirection.In, referencedArgumentName); } } [ContentProperty("Expression")] [TypeConverter(typeof(InArgumentConverter))] [ValueSerializer(typeof(ArgumentValueSerializer))] public sealed class InArgument: InArgument { public InArgument(Variable variable) : this() { if (variable != null) { this.Expression = new VariableValue { Variable = variable }; } } public InArgument(DelegateArgument delegateArgument) : this() { if (delegateArgument != null) { this.Expression = new DelegateArgumentValue { DelegateArgument = delegateArgument }; } } public InArgument(T constValue) : this() { this.Expression = new Literal { Value = constValue }; } public InArgument(Expression > expression) : this() { if (expression != null) { this.Expression = new LambdaValue (expression); } } public InArgument(Activity expression) : this() { this.Expression = expression; } public InArgument() : base() { this.ArgumentType = typeof(T); } [DefaultValue(null)] public new Activity Expression { get; set; } internal override ActivityWithResult ExpressionCore { get { return this.Expression; } set { if (value == null) { this.Expression = null; return; } if (value is Activity ) { this.Expression = (Activity )value; } else { // We do not verify compatibility here. We will do that // during CacheMetadata in Argument.Validate. this.Expression = new ActivityWithResultWrapper (value); } } } public static implicit operator InArgument (Variable variable) { return FromVariable(variable); } public static implicit operator InArgument (DelegateArgument delegateArgument) { return FromDelegateArgument(delegateArgument); } public static implicit operator InArgument (Activity expression) { return FromExpression(expression); } public static implicit operator InArgument (T constValue) { return FromValue(constValue); } public static InArgument FromVariable(Variable variable) { if (variable == null) { throw FxTrace.Exception.ArgumentNull("variable"); } return new InArgument (variable); } public static InArgument FromDelegateArgument(DelegateArgument delegateArgument) { if (delegateArgument == null) { throw FxTrace.Exception.ArgumentNull("delegateArgument"); } return new InArgument (delegateArgument); } public static InArgument FromExpression(Activity expression) { if (expression == null) { throw FxTrace.Exception.ArgumentNull("expression"); } return new InArgument (expression); } public static InArgument FromValue(T constValue) { return new InArgument { Expression = new Literal { Value = constValue } }; } // Soft-Link: This method is referenced through reflection by // ExpressionUtilities.TryRewriteLambdaExpression. Update that // file if the signature changes. public new T Get(ActivityContext context) { return Get (context); } public void Set(ActivityContext context, T value) { if (context == null) { throw FxTrace.Exception.ArgumentNull("context"); } context.SetValue(this, value); } internal override Location CreateDefaultLocation() { return Argument.CreateLocation (); } internal override bool TryPopulateValue(LocationEnvironment targetEnvironment, ActivityInstance activityInstance, ActivityContext resolutionContext) { Fx.Assert(this.Expression != null, "This should only be called for non-empty bindings"); Location location = Argument.CreateLocation (); targetEnvironment.Declare(this.RuntimeArgument, location, activityInstance); T argumentValue; if (this.Expression.TryGetValue(resolutionContext, out argumentValue)) { location.Value = argumentValue; return true; } else { return false; } } internal override void Declare(LocationEnvironment targetEnvironment, ActivityInstance targetActivityInstance) { targetEnvironment.Declare(this.RuntimeArgument, CreateDefaultLocation(), targetActivityInstance); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
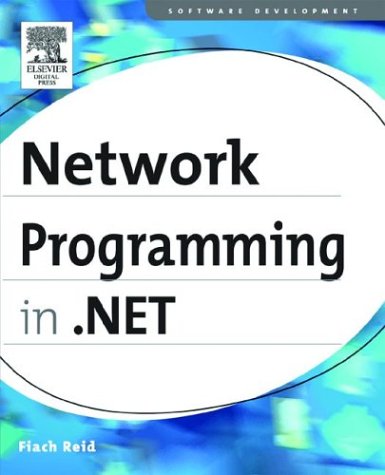
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Size.cs
- PreviewPrintController.cs
- MobileFormsAuthentication.cs
- QueryCoreOp.cs
- NameTable.cs
- RemoteWebConfigurationHostServer.cs
- XmlStreamStore.cs
- WebConfigurationManager.cs
- SqlXml.cs
- HtmlElement.cs
- ECDiffieHellmanCng.cs
- InvalidDataContractException.cs
- AssociatedControlConverter.cs
- WebMessageEncoderFactory.cs
- DocumentPage.cs
- BlobPersonalizationState.cs
- COM2FontConverter.cs
- ObjectDisposedException.cs
- ImportCatalogPart.cs
- Label.cs
- DataListItem.cs
- WebPartMovingEventArgs.cs
- TreeIterators.cs
- TreeNodeBindingDepthConverter.cs
- BufferedGraphicsManager.cs
- WebHeaderCollection.cs
- Viewport3DVisual.cs
- SqlDataSourceWizardForm.cs
- ScrollBarRenderer.cs
- KeyGestureConverter.cs
- WebPartVerb.cs
- DataGridViewCellEventArgs.cs
- AutomationPropertyInfo.cs
- BooleanSwitch.cs
- MenuBase.cs
- EventProvider.cs
- AnnotationHelper.cs
- __ComObject.cs
- MsmqChannelFactoryBase.cs
- TargetException.cs
- XmlNamespaceMapping.cs
- WorkflowDesigner.cs
- TraceHwndHost.cs
- SQLDateTime.cs
- HuffCodec.cs
- CLSCompliantAttribute.cs
- WorkflowServiceAttributes.cs
- XamlStyleSerializer.cs
- InheritanceContextChangedEventManager.cs
- XmlSchemaObject.cs
- safex509handles.cs
- ParsedAttributeCollection.cs
- TableCellAutomationPeer.cs
- ActionFrame.cs
- ScalarConstant.cs
- RenderingBiasValidation.cs
- Internal.cs
- login.cs
- MemberInitExpression.cs
- Msmq.cs
- CharacterBuffer.cs
- CodeLabeledStatement.cs
- SectionVisual.cs
- DragCompletedEventArgs.cs
- Tag.cs
- Vector3DCollectionValueSerializer.cs
- InvalidOperationException.cs
- DataControlFieldHeaderCell.cs
- BuildResult.cs
- SimpleNameService.cs
- FontWeights.cs
- UnionExpr.cs
- XmlObjectSerializerWriteContext.cs
- DSGeneratorProblem.cs
- ByteAnimationUsingKeyFrames.cs
- ProfileModule.cs
- FormParameter.cs
- ExceptionHandler.cs
- SafeNativeMethods.cs
- LabelExpression.cs
- BypassElement.cs
- XsltOutput.cs
- WsdlBuildProvider.cs
- CodeDelegateInvokeExpression.cs
- XmlQueryStaticData.cs
- ExceptionHelpers.cs
- SingleAnimationBase.cs
- AnnotationComponentManager.cs
- CategoryAttribute.cs
- EdmItemError.cs
- FrameworkElementFactory.cs
- Axis.cs
- TagPrefixAttribute.cs
- JsonCollectionDataContract.cs
- RoutedEventValueSerializer.cs
- JoinCqlBlock.cs
- DecoderNLS.cs
- SQLStringStorage.cs
- WeakHashtable.cs
- MULTI_QI.cs