Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / BoundPropertyEntry.cs / 1305376 / BoundPropertyEntry.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Reflection; using System.Web.Util; using System.Web.Compilation; using System.ComponentModel.Design; using System.Security.Permissions; ////// PropertyEntry for any bound properties /// public class BoundPropertyEntry : PropertyEntry { private string _expression; private ExpressionBuilder _expressionBuilder; private string _expressionPrefix; private bool _useSetAttribute; private object _parsedExpressionData; private bool _generated; private string _fieldName; private string _formatString; private string _controlID; private Type _controlType; private bool _readOnlyProperty; private bool _twoWayBound; internal BoundPropertyEntry() { } ////// The id of the control that contains this binding. /// public string ControlID { get { return _controlID; } set { _controlID = value; } } ////// The type of the control which is being bound to a runtime value. /// public Type ControlType { get { return _controlType; } set { _controlType = value; } } ////// public string Expression { get { return _expression; } set { _expression = value; } } public ExpressionBuilder ExpressionBuilder { get { return _expressionBuilder; } set { _expressionBuilder = value; } } public string ExpressionPrefix { get { return _expressionPrefix; } set { _expressionPrefix = value; } } ////// The name of the data field that is being bound to. /// public string FieldName { get { return _fieldName; } set { _fieldName = value; } } ////// The format string applied to the field for display. /// public string FormatString { get { return _formatString; } set { _formatString = value; } } internal bool IsDataBindingEntry { // Empty prefix means it's a databinding expression (i.e. <%# ... %>) get { return String.IsNullOrEmpty(ExpressionPrefix); } } public bool Generated { get { return _generated; } set { _generated = value; } } public object ParsedExpressionData { get { return _parsedExpressionData; } set { _parsedExpressionData = value; } } ////// Indicates whether the two way statement is set and get, or just get but not set. /// public bool ReadOnlyProperty { get { return _readOnlyProperty; } set { _readOnlyProperty = value; } } public bool TwoWayBound { get { return _twoWayBound; } set { _twoWayBound = value; } } ////// public bool UseSetAttribute { get { return _useSetAttribute; } set { _useSetAttribute = value; } } // Parse the expression, and store the resulting object internal void ParseExpression(ExpressionBuilderContext context) { if (Expression == null || ExpressionPrefix == null || ExpressionBuilder == null) return; _parsedExpressionData = ExpressionBuilder.ParseExpression(Expression, Type, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
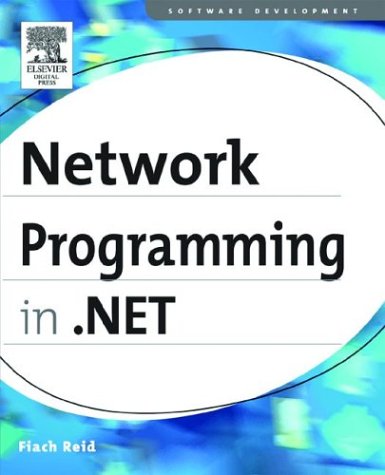
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReferencedAssembly.cs
- SendKeys.cs
- UIAgentAsyncBeginRequest.cs
- CreateSequenceResponse.cs
- ProcessStartInfo.cs
- PrePostDescendentsWalker.cs
- AmbientLight.cs
- FixedTextView.cs
- XmlSchemaExternal.cs
- Pkcs7Recipient.cs
- TextTreeUndo.cs
- HttpPostClientProtocol.cs
- DmlSqlGenerator.cs
- StorageMappingItemCollection.cs
- ColumnProvider.cs
- UrlAuthFailedErrorFormatter.cs
- TreeNodeConverter.cs
- _HeaderInfo.cs
- FileSystemInfo.cs
- DataListDesigner.cs
- TextRangeEditTables.cs
- SolidColorBrush.cs
- SelectionList.cs
- XmlSerializer.cs
- HyperLinkField.cs
- VirtualDirectoryMapping.cs
- DataGridPageChangedEventArgs.cs
- FormatConvertedBitmap.cs
- PointValueSerializer.cs
- ReachSerializationCacheItems.cs
- WmlObjectListAdapter.cs
- XmlSchemaAppInfo.cs
- ParameterElementCollection.cs
- TextRangeSerialization.cs
- DataSpaceManager.cs
- DocumentXPathNavigator.cs
- HandleCollector.cs
- ContractAdapter.cs
- CellTreeNodeVisitors.cs
- InternalSafeNativeMethods.cs
- WpfXamlMember.cs
- DeploymentSection.cs
- CLSCompliantAttribute.cs
- CodeTryCatchFinallyStatement.cs
- HotCommands.cs
- BitmapCodecInfoInternal.cs
- InvalidFilterCriteriaException.cs
- TabletCollection.cs
- ByteStack.cs
- ISFTagAndGuidCache.cs
- UrlAuthFailedErrorFormatter.cs
- XmlSchemaComplexContentRestriction.cs
- DiscriminatorMap.cs
- Trigger.cs
- ProfileGroupSettings.cs
- AnimatedTypeHelpers.cs
- FixedSOMElement.cs
- BackgroundWorker.cs
- XmlEnumAttribute.cs
- SoapSchemaImporter.cs
- HtmlTitle.cs
- TreeViewDataItemAutomationPeer.cs
- TypeDelegator.cs
- userdatakeys.cs
- OracleInfoMessageEventArgs.cs
- SignatureToken.cs
- ObjectListCommandEventArgs.cs
- VectorAnimationUsingKeyFrames.cs
- HwndMouseInputProvider.cs
- ComponentConverter.cs
- ProcessManager.cs
- Facet.cs
- SqlCommandAsyncResult.cs
- RecognitionResult.cs
- TypedAsyncResult.cs
- ActivityInstanceMap.cs
- TextSchema.cs
- VisualBrush.cs
- ItemCollection.cs
- DetailsViewDeleteEventArgs.cs
- ConditionChanges.cs
- Classification.cs
- TableAutomationPeer.cs
- InputBinder.cs
- InputElement.cs
- ContentHostHelper.cs
- DockProviderWrapper.cs
- BuildProvider.cs
- columnmapfactory.cs
- PersonalizableAttribute.cs
- CodeIndexerExpression.cs
- XmlSortKeyAccumulator.cs
- UnknownWrapper.cs
- NotifyInputEventArgs.cs
- XmlDictionaryReaderQuotas.cs
- FileRegion.cs
- OptionUsage.cs
- LayoutTable.cs
- VirtualPathProvider.cs
- StandardMenuStripVerb.cs