Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / TreeNodeConverter.cs / 1305376 / TreeNodeConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Drawing; using System.Diagnostics; using System.Globalization; using System.Reflection; ////// /// TreeNodeConverter is a class that can be used to convert /// TreeNode objects from one data type to another. Access this /// class through the TypeDescriptor. /// public class TreeNodeConverter : TypeConverter { ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(InstanceDescriptor) && value is TreeNode) { TreeNode node = (TreeNode)value; MemberInfo info = null; object[] args = null; if (node.ImageIndex == -1 || node.SelectedImageIndex == -1) { if (node.Nodes.Count == 0) { info = typeof(TreeNode).GetConstructor(new Type[] {typeof(string)}); args = new object[] {node.Text}; } else { info = typeof(TreeNode).GetConstructor(new Type[] {typeof(string), typeof(TreeNode[])}); TreeNode[] nodesArray = new TreeNode[node.Nodes.Count]; node.Nodes.CopyTo(nodesArray, 0); args = new object[] {node.Text, nodesArray}; } } else { if (node.Nodes.Count == 0) { info = typeof(TreeNode).GetConstructor(new Type[] { typeof(string), typeof(int), typeof(int)}); args = new object[] { node.Text, node.ImageIndex, node.SelectedImageIndex}; } else { info = typeof(TreeNode).GetConstructor(new Type[] { typeof(string), typeof(int), typeof(int), typeof(TreeNode[])}); TreeNode[] nodesArray = new TreeNode[node.Nodes.Count]; node.Nodes.CopyTo(nodesArray, 0); args = new object[] { node.Text, node.ImageIndex, node.SelectedImageIndex, nodesArray}; } } if (info != null) { return new InstanceDescriptor(info, args, false); } } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Drawing; using System.Diagnostics; using System.Globalization; using System.Reflection; ////// /// TreeNodeConverter is a class that can be used to convert /// TreeNode objects from one data type to another. Access this /// class through the TypeDescriptor. /// public class TreeNodeConverter : TypeConverter { ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(InstanceDescriptor) && value is TreeNode) { TreeNode node = (TreeNode)value; MemberInfo info = null; object[] args = null; if (node.ImageIndex == -1 || node.SelectedImageIndex == -1) { if (node.Nodes.Count == 0) { info = typeof(TreeNode).GetConstructor(new Type[] {typeof(string)}); args = new object[] {node.Text}; } else { info = typeof(TreeNode).GetConstructor(new Type[] {typeof(string), typeof(TreeNode[])}); TreeNode[] nodesArray = new TreeNode[node.Nodes.Count]; node.Nodes.CopyTo(nodesArray, 0); args = new object[] {node.Text, nodesArray}; } } else { if (node.Nodes.Count == 0) { info = typeof(TreeNode).GetConstructor(new Type[] { typeof(string), typeof(int), typeof(int)}); args = new object[] { node.Text, node.ImageIndex, node.SelectedImageIndex}; } else { info = typeof(TreeNode).GetConstructor(new Type[] { typeof(string), typeof(int), typeof(int), typeof(TreeNode[])}); TreeNode[] nodesArray = new TreeNode[node.Nodes.Count]; node.Nodes.CopyTo(nodesArray, 0); args = new object[] { node.Text, node.ImageIndex, node.SelectedImageIndex, nodesArray}; } } if (info != null) { return new InstanceDescriptor(info, args, false); } } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
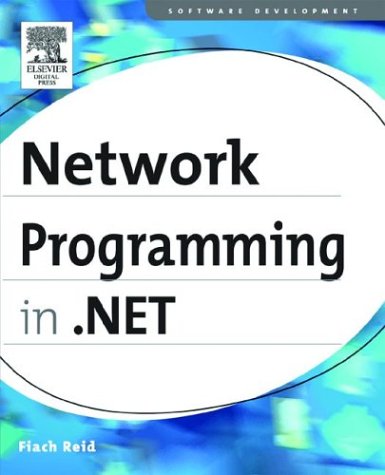
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ChannelTerminatedException.cs
- ExtentCqlBlock.cs
- RemoteHelper.cs
- ColorConvertedBitmap.cs
- DayRenderEvent.cs
- mansign.cs
- WmfPlaceableFileHeader.cs
- OptimizerPatterns.cs
- SubtreeProcessor.cs
- ProcessModuleDesigner.cs
- XhtmlBasicLiteralTextAdapter.cs
- PeerCollaboration.cs
- WinCategoryAttribute.cs
- InputManager.cs
- Point3DAnimationBase.cs
- WsatTransactionInfo.cs
- Number.cs
- CompositeCollectionView.cs
- PartialList.cs
- ProcessModelInfo.cs
- ObjectQueryExecutionPlan.cs
- SafeNativeMethods.cs
- Utilities.cs
- Visitors.cs
- PtsPage.cs
- ReferenceSchema.cs
- SimpleBitVector32.cs
- EntityDataSource.cs
- WebCategoryAttribute.cs
- CodeMemberProperty.cs
- SspiNegotiationTokenAuthenticatorState.cs
- localization.cs
- BooleanSwitch.cs
- Size.cs
- SHA512.cs
- AuthenticationModuleElementCollection.cs
- Bitmap.cs
- BidOverLoads.cs
- ServiceModelEnhancedConfigurationElementCollection.cs
- OracleColumn.cs
- Decimal.cs
- Activator.cs
- CanonicalFormWriter.cs
- StrongNameKeyPair.cs
- JoinQueryOperator.cs
- ColorConvertedBitmapExtension.cs
- FormsIdentity.cs
- HwndTarget.cs
- HashFinalRequest.cs
- WindowsGraphics.cs
- SharedStatics.cs
- PerspectiveCamera.cs
- GenericRootAutomationPeer.cs
- XmlCharacterData.cs
- DefaultTextStoreTextComposition.cs
- ExpiredSecurityTokenException.cs
- TransformPattern.cs
- StrongName.cs
- CollectionExtensions.cs
- GeneralTransform3DGroup.cs
- TableParaClient.cs
- _IPv4Address.cs
- QilBinary.cs
- InputLanguageProfileNotifySink.cs
- ScriptingSectionGroup.cs
- UrlAuthFailedErrorFormatter.cs
- PrePrepareMethodAttribute.cs
- ReferenceEqualityComparer.cs
- RewritingSimplifier.cs
- RSAOAEPKeyExchangeFormatter.cs
- DesignerToolboxInfo.cs
- CommonDialog.cs
- UseLicense.cs
- ReadOnlyCollectionBase.cs
- AppDomainFactory.cs
- _AutoWebProxyScriptHelper.cs
- CodeTypeDeclaration.cs
- PrtTicket_Public_Simple.cs
- RangeContentEnumerator.cs
- TextAdaptor.cs
- Rotation3DKeyFrameCollection.cs
- MimeMapping.cs
- ProxyDataContractResolver.cs
- WorkflowApplicationEventArgs.cs
- RecognitionEventArgs.cs
- PresentationAppDomainManager.cs
- WebPartDescriptionCollection.cs
- ColumnCollection.cs
- GridViewSelectEventArgs.cs
- ProgressBarAutomationPeer.cs
- OrthographicCamera.cs
- XmlEntity.cs
- HttpHandlersSection.cs
- WebPartConnectVerb.cs
- WhileDesigner.cs
- XmlSubtreeReader.cs
- CachedTypeface.cs
- WebPartEditorCancelVerb.cs
- SafeRightsManagementQueryHandle.cs
- Expression.cs