Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / clr / src / BCL / System / Reflection / StrongNameKeyPair.cs / 1 / StrongNameKeyPair.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** File: StrongNameKeyPair.cs ** ** ** Purpose: Encapsulate access to a public/private key pair ** used to sign strong name assemblies. ** ** ===========================================================*/ namespace System.Reflection { using System; using System.IO; using System.Runtime.CompilerServices; using System.Runtime.Serialization; using System.Security.Permissions; [Serializable()] [System.Runtime.InteropServices.ComVisible(true)] public class StrongNameKeyPair : IDeserializationCallback, ISerializable { private bool _keyPairExported; private byte[] _keyPairArray; private String _keyPairContainer; private byte[] _publicKey; // Build key pair from file. [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.UnmanagedCode)] public StrongNameKeyPair(FileStream keyPairFile) { if (keyPairFile == null) throw new ArgumentNullException("keyPairFile"); int length = (int)keyPairFile.Length; _keyPairArray = new byte[length]; keyPairFile.Read(_keyPairArray, 0, length); _keyPairExported = true; } // Build key pair from byte array in memory. [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.UnmanagedCode)] public StrongNameKeyPair(byte[] keyPairArray) { if (keyPairArray == null) throw new ArgumentNullException("keyPairArray"); _keyPairArray = new byte[keyPairArray.Length]; Array.Copy(keyPairArray, _keyPairArray, keyPairArray.Length); _keyPairExported = true; } // Reference key pair in named key container. [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.UnmanagedCode)] public StrongNameKeyPair(String keyPairContainer) { if (keyPairContainer == null) throw new ArgumentNullException("keyPairContainer"); _keyPairContainer = keyPairContainer; _keyPairExported = false; } [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.UnmanagedCode)] protected StrongNameKeyPair (SerializationInfo info, StreamingContext context) { _keyPairExported = (bool) info.GetValue("_keyPairExported", typeof(bool)); _keyPairArray = (byte[]) info.GetValue("_keyPairArray", typeof(byte[])); _keyPairContainer = (string) info.GetValue("_keyPairContainer", typeof(string)); _publicKey = (byte[]) info.GetValue("_publicKey", typeof(byte[])); } // Get the public portion of the key pair. public byte[] PublicKey { get { if (_publicKey == null) { _publicKey = nGetPublicKey(_keyPairExported, _keyPairArray, _keyPairContainer); } byte[] publicKey = new byte[_publicKey.Length]; Array.Copy(_publicKey, publicKey, _publicKey.Length); return publicKey; } } ///void ISerializable.GetObjectData (SerializationInfo info, StreamingContext context) { info.AddValue("_keyPairExported", _keyPairExported); info.AddValue("_keyPairArray", _keyPairArray); info.AddValue("_keyPairContainer", _keyPairContainer); info.AddValue("_publicKey", _publicKey); } /// void IDeserializationCallback.OnDeserialization (Object sender) {} // Internal routine used to retrieve key pair info from unmanaged code. private bool GetKeyPair(out Object arrayOrContainer) { arrayOrContainer = _keyPairExported ? (Object)_keyPairArray : (Object)_keyPairContainer; return _keyPairExported; } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern byte[] nGetPublicKey(bool exported, byte[] array, String container); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
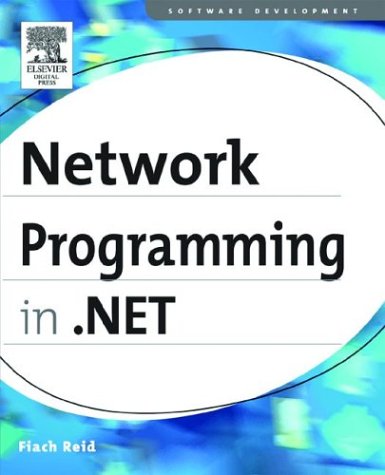
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlDesigner.cs
- ApplicationContext.cs
- MasterPageParser.cs
- ImageList.cs
- DeviceContexts.cs
- HttpCacheVaryByContentEncodings.cs
- ForwardPositionQuery.cs
- ResourceWriter.cs
- DrawItemEvent.cs
- IRCollection.cs
- RightsManagementEncryptionTransform.cs
- QilReference.cs
- SectionRecord.cs
- VectorCollectionConverter.cs
- VectorValueSerializer.cs
- VisualStyleRenderer.cs
- BatchParser.cs
- PrefixQName.cs
- TypeBrowserDialog.cs
- TextProperties.cs
- AspNetCompatibilityRequirementsAttribute.cs
- ContentType.cs
- ZipIOLocalFileDataDescriptor.cs
- ResourceDisplayNameAttribute.cs
- ReflectionTypeLoadException.cs
- Brush.cs
- Form.cs
- FilteredReadOnlyMetadataCollection.cs
- ErrorsHelper.cs
- StretchValidation.cs
- basecomparevalidator.cs
- AdapterDictionary.cs
- PackageDigitalSignature.cs
- DataGridHeaderBorder.cs
- Stack.cs
- CustomAssemblyResolver.cs
- HtmlTable.cs
- FlowNode.cs
- PrimaryKeyTypeConverter.cs
- CurrentTimeZone.cs
- SystemBrushes.cs
- indexingfiltermarshaler.cs
- CodeEntryPointMethod.cs
- CodeVariableDeclarationStatement.cs
- ProfileProvider.cs
- CodeTypeReferenceCollection.cs
- TextRunTypographyProperties.cs
- SelectionProviderWrapper.cs
- PassportPrincipal.cs
- ChildDocumentBlock.cs
- NavigatorOutput.cs
- ModuleConfigurationInfo.cs
- FtpWebResponse.cs
- TextDecorations.cs
- HebrewNumber.cs
- FileDialog_Vista_Interop.cs
- hwndwrapper.cs
- BufferedGraphicsManager.cs
- Events.cs
- Semaphore.cs
- StringFormat.cs
- SchemaInfo.cs
- XmlSchemaAnnotation.cs
- GraphicsState.cs
- FilteredSchemaElementLookUpTable.cs
- StreamInfo.cs
- EntitySqlQueryCacheKey.cs
- ReturnValue.cs
- Helper.cs
- Timer.cs
- NullRuntimeConfig.cs
- ScrollItemPattern.cs
- basevalidator.cs
- EventLog.cs
- CodeAttributeArgument.cs
- FacetValueContainer.cs
- WaitForChangedResult.cs
- DataGridPagerStyle.cs
- PolicyException.cs
- TemplatePropertyEntry.cs
- Rotation3DAnimationUsingKeyFrames.cs
- OdbcFactory.cs
- StateMachineSubscription.cs
- IntegerValidator.cs
- StorageRoot.cs
- FontNameEditor.cs
- DataBoundLiteralControl.cs
- QilParameter.cs
- PreservationFileReader.cs
- ToolStripPanel.cs
- ExeContext.cs
- CompositeActivityTypeDescriptor.cs
- SharedPersonalizationStateInfo.cs
- XmlQualifiedName.cs
- HitTestResult.cs
- COM2PropertyDescriptor.cs
- RenderTargetBitmap.cs
- CommittableTransaction.cs
- CopyAction.cs
- StylusOverProperty.cs