Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / ApplicationContext.cs / 1 / ApplicationContext.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.ComponentModel; ////// /// ApplicationContext provides contextual information about an application /// thread. Specifically this allows an application author to redifine what /// circurmstances cause a message loop to exit. By default the application /// context listens to the close event on the mainForm, then exits the /// thread's message loop. /// public class ApplicationContext : IDisposable { Form mainForm; object userData; ////// /// Creates a new ApplicationContext with no mainForm. /// public ApplicationContext() : this(null) { } ////// /// Creates a new ApplicationContext with the specified mainForm. /// If OnMainFormClosed is not overriden, the thread's message /// loop will be terminated when mainForm is closed. /// public ApplicationContext(Form mainForm) { this.MainForm = mainForm; } ///~ApplicationContext() { Dispose(false); } /// /// /// Determines the mainForm for this context. This may be changed /// at anytime. /// If OnMainFormClosed is not overriden, the thread's message /// loop will be terminated when mainForm is closed. /// public Form MainForm { get { return mainForm; } set { EventHandler onClose = new EventHandler(OnMainFormDestroy); if (mainForm != null) { mainForm.HandleDestroyed -= onClose; } mainForm = value; if (mainForm != null) { mainForm.HandleDestroyed += onClose; } } } ///[ SRCategory(SR.CatData), Localizable(false), Bindable(true), SRDescription(SR.ControlTagDescr), DefaultValue(null), TypeConverter(typeof(StringConverter)), ] public object Tag { get { return userData; } set { userData = value; } } /// /// /// Is raised when the thread's message loop should be terminated. /// This is raised by calling ExitThread. /// public event EventHandler ThreadExit; ////// /// Disposes the context. This should dispose the mainForm. This is /// called immediately after the thread's message loop is terminated. /// Application will dispose all forms on this thread by default. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ///protected virtual void Dispose(bool disposing) { if (disposing) { if (mainForm != null) { if (!mainForm.IsDisposed) { mainForm.Dispose(); } mainForm = null; } } } /// /// /// Causes the thread's message loop to be terminated. This /// will call ExitThreadCore. /// public void ExitThread() { ExitThreadCore(); } ////// /// Causes the thread's message loop to be terminated. /// protected virtual void ExitThreadCore() { if (ThreadExit != null) { ThreadExit(this, EventArgs.Empty); } } ////// /// Called when the mainForm is closed. The default implementation /// of this will call ExitThreadCore. /// protected virtual void OnMainFormClosed(object sender, EventArgs e) { ExitThreadCore(); } ////// Called when the mainForm is closed. The default implementation /// of this will call ExitThreadCore. /// private void OnMainFormDestroy(object sender, EventArgs e) { Form form = (Form)sender; if (!form.RecreatingHandle) { form.HandleDestroyed -= new EventHandler(OnMainFormDestroy); OnMainFormClosed(sender, e); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.ComponentModel; ////// /// ApplicationContext provides contextual information about an application /// thread. Specifically this allows an application author to redifine what /// circurmstances cause a message loop to exit. By default the application /// context listens to the close event on the mainForm, then exits the /// thread's message loop. /// public class ApplicationContext : IDisposable { Form mainForm; object userData; ////// /// Creates a new ApplicationContext with no mainForm. /// public ApplicationContext() : this(null) { } ////// /// Creates a new ApplicationContext with the specified mainForm. /// If OnMainFormClosed is not overriden, the thread's message /// loop will be terminated when mainForm is closed. /// public ApplicationContext(Form mainForm) { this.MainForm = mainForm; } ///~ApplicationContext() { Dispose(false); } /// /// /// Determines the mainForm for this context. This may be changed /// at anytime. /// If OnMainFormClosed is not overriden, the thread's message /// loop will be terminated when mainForm is closed. /// public Form MainForm { get { return mainForm; } set { EventHandler onClose = new EventHandler(OnMainFormDestroy); if (mainForm != null) { mainForm.HandleDestroyed -= onClose; } mainForm = value; if (mainForm != null) { mainForm.HandleDestroyed += onClose; } } } ///[ SRCategory(SR.CatData), Localizable(false), Bindable(true), SRDescription(SR.ControlTagDescr), DefaultValue(null), TypeConverter(typeof(StringConverter)), ] public object Tag { get { return userData; } set { userData = value; } } /// /// /// Is raised when the thread's message loop should be terminated. /// This is raised by calling ExitThread. /// public event EventHandler ThreadExit; ////// /// Disposes the context. This should dispose the mainForm. This is /// called immediately after the thread's message loop is terminated. /// Application will dispose all forms on this thread by default. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ///protected virtual void Dispose(bool disposing) { if (disposing) { if (mainForm != null) { if (!mainForm.IsDisposed) { mainForm.Dispose(); } mainForm = null; } } } /// /// /// Causes the thread's message loop to be terminated. This /// will call ExitThreadCore. /// public void ExitThread() { ExitThreadCore(); } ////// /// Causes the thread's message loop to be terminated. /// protected virtual void ExitThreadCore() { if (ThreadExit != null) { ThreadExit(this, EventArgs.Empty); } } ////// /// Called when the mainForm is closed. The default implementation /// of this will call ExitThreadCore. /// protected virtual void OnMainFormClosed(object sender, EventArgs e) { ExitThreadCore(); } ////// Called when the mainForm is closed. The default implementation /// of this will call ExitThreadCore. /// private void OnMainFormDestroy(object sender, EventArgs e) { Form form = (Form)sender; if (!form.RecreatingHandle) { form.HandleDestroyed -= new EventHandler(OnMainFormDestroy); OnMainFormClosed(sender, e); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
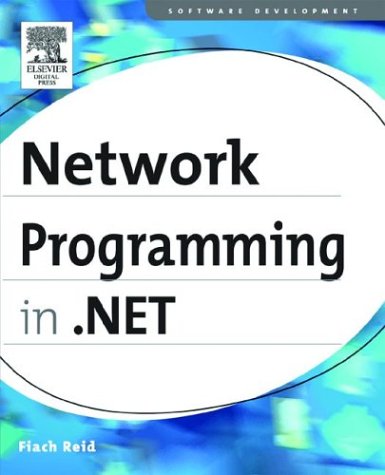
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DetailsViewUpdateEventArgs.cs
- ElementHostPropertyMap.cs
- ReadOnlyHierarchicalDataSourceView.cs
- ContextQuery.cs
- DependencyProperty.cs
- XsdValidatingReader.cs
- Matrix3DStack.cs
- DependencyObjectPropertyDescriptor.cs
- IsolationInterop.cs
- LassoHelper.cs
- ClientConfigurationSystem.cs
- RemotingHelper.cs
- BlobPersonalizationState.cs
- XmlElementList.cs
- HostingEnvironmentSection.cs
- XmlQueryStaticData.cs
- ScrollProperties.cs
- CodeDefaultValueExpression.cs
- XmlNamespaceDeclarationsAttribute.cs
- DataSvcMapFileSerializer.cs
- MobileUserControl.cs
- MessageHeaderAttribute.cs
- RecognizerInfo.cs
- TemplateBindingExtensionConverter.cs
- SchemaInfo.cs
- WebResponse.cs
- KnownTypes.cs
- ObjectStateManagerMetadata.cs
- MetadataPropertyvalue.cs
- Message.cs
- ApplicationFileParser.cs
- ImageSourceValueSerializer.cs
- TextBlockAutomationPeer.cs
- ScaleTransform.cs
- ParserOptions.cs
- BaseTreeIterator.cs
- WebServiceParameterData.cs
- UserControlFileEditor.cs
- XmlSchemaExporter.cs
- ConfigurationFileMap.cs
- CompareValidator.cs
- UInt64Converter.cs
- WebBrowserBase.cs
- ExtendedProperty.cs
- ImageKeyConverter.cs
- PrintingPermission.cs
- TextRangeBase.cs
- Constraint.cs
- OutputScopeManager.cs
- ResourceWriter.cs
- CngProperty.cs
- MethodToken.cs
- WebPartConnectionsCloseVerb.cs
- TextEvent.cs
- CalculatedColumn.cs
- DataGridTable.cs
- Opcode.cs
- ContractBase.cs
- TopClause.cs
- ConnectionManager.cs
- StreamReader.cs
- SHA1.cs
- SchemaTableColumn.cs
- SqlFactory.cs
- FrameworkElement.cs
- FileDialog.cs
- SqlRecordBuffer.cs
- Material.cs
- XmlEncoding.cs
- HtmlSelect.cs
- FileUtil.cs
- LoadedOrUnloadedOperation.cs
- UpdatePanel.cs
- TableLayoutRowStyleCollection.cs
- AspNetSynchronizationContext.cs
- ServiceManager.cs
- SmtpSection.cs
- ProjectionNode.cs
- DecoderFallbackWithFailureFlag.cs
- TreeViewImageGenerator.cs
- RadioButton.cs
- serverconfig.cs
- Point3D.cs
- Size.cs
- documentsequencetextcontainer.cs
- ExpressionPrefixAttribute.cs
- RealizationContext.cs
- PersonalizationAdministration.cs
- CodeExporter.cs
- NativeMethods.cs
- ComboBox.cs
- EventDescriptorCollection.cs
- ConstructorExpr.cs
- LocalBuilder.cs
- ProfileService.cs
- MinMaxParagraphWidth.cs
- UpdateRecord.cs
- ColumnResizeUndoUnit.cs
- TextBoxAutoCompleteSourceConverter.cs
- SqlDependencyUtils.cs