Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Reflection / Emit / Opcode.cs / 1305376 / Opcode.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] namespace System.Reflection.Emit { using System; using System.Security.Permissions; using System.Diagnostics.Contracts; [System.Runtime.InteropServices.ComVisible(true)] public struct OpCode { internal String m_stringname; internal StackBehaviour m_pop; internal StackBehaviour m_push; internal OperandType m_operand; internal OpCodeType m_type; internal int m_size; internal byte m_s1; internal byte m_s2; internal FlowControl m_ctrl; // Specifies whether the current instructions causes the control flow to // change unconditionally. internal bool m_endsUncondJmpBlk; // Specifies the stack change that the current instruction causes not // taking into account the operand dependant stack changes. internal int m_stackChange; internal OpCode(String stringname, StackBehaviour pop, StackBehaviour push, OperandType operand, OpCodeType type, int size, byte s1, byte s2, FlowControl ctrl, bool endsjmpblk, int stack) { m_stringname = stringname; m_pop = pop; m_push = push; m_operand = operand; m_type = type; m_size = size; m_s1 = s1; m_s2 = s2; m_ctrl = ctrl; m_endsUncondJmpBlk = endsjmpblk; m_stackChange = stack; } internal bool EndsUncondJmpBlk() { return m_endsUncondJmpBlk; } internal int StackChange() { return m_stackChange; } public OperandType OperandType { get { return (m_operand); } } public FlowControl FlowControl { get { return (m_ctrl); } } public OpCodeType OpCodeType { get { return (m_type); } } public StackBehaviour StackBehaviourPop { get { return (m_pop); } } public StackBehaviour StackBehaviourPush { get { return (m_push); } } public int Size { get { return (m_size); } } public short Value { get { if (m_size == 2) return (short) (m_s1 << 8 | m_s2); return (short) m_s2; } } public String Name { get { return m_stringname; } } [Pure] public override bool Equals(Object obj) { if (obj is OpCode) return Equals((OpCode)obj); else return false; } [Pure] public bool Equals(OpCode obj) { return obj.m_s1 == m_s1 && obj.m_s2 == m_s2; } [Pure] public static bool operator ==(OpCode a, OpCode b) { return a.Equals(b); } [Pure] public static bool operator !=(OpCode a, OpCode b) { return !(a == b); } public override int GetHashCode() { return this.m_stringname.GetHashCode(); } public override String ToString() { return m_stringname; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] namespace System.Reflection.Emit { using System; using System.Security.Permissions; using System.Diagnostics.Contracts; [System.Runtime.InteropServices.ComVisible(true)] public struct OpCode { internal String m_stringname; internal StackBehaviour m_pop; internal StackBehaviour m_push; internal OperandType m_operand; internal OpCodeType m_type; internal int m_size; internal byte m_s1; internal byte m_s2; internal FlowControl m_ctrl; // Specifies whether the current instructions causes the control flow to // change unconditionally. internal bool m_endsUncondJmpBlk; // Specifies the stack change that the current instruction causes not // taking into account the operand dependant stack changes. internal int m_stackChange; internal OpCode(String stringname, StackBehaviour pop, StackBehaviour push, OperandType operand, OpCodeType type, int size, byte s1, byte s2, FlowControl ctrl, bool endsjmpblk, int stack) { m_stringname = stringname; m_pop = pop; m_push = push; m_operand = operand; m_type = type; m_size = size; m_s1 = s1; m_s2 = s2; m_ctrl = ctrl; m_endsUncondJmpBlk = endsjmpblk; m_stackChange = stack; } internal bool EndsUncondJmpBlk() { return m_endsUncondJmpBlk; } internal int StackChange() { return m_stackChange; } public OperandType OperandType { get { return (m_operand); } } public FlowControl FlowControl { get { return (m_ctrl); } } public OpCodeType OpCodeType { get { return (m_type); } } public StackBehaviour StackBehaviourPop { get { return (m_pop); } } public StackBehaviour StackBehaviourPush { get { return (m_push); } } public int Size { get { return (m_size); } } public short Value { get { if (m_size == 2) return (short) (m_s1 << 8 | m_s2); return (short) m_s2; } } public String Name { get { return m_stringname; } } [Pure] public override bool Equals(Object obj) { if (obj is OpCode) return Equals((OpCode)obj); else return false; } [Pure] public bool Equals(OpCode obj) { return obj.m_s1 == m_s1 && obj.m_s2 == m_s2; } [Pure] public static bool operator ==(OpCode a, OpCode b) { return a.Equals(b); } [Pure] public static bool operator !=(OpCode a, OpCode b) { return !(a == b); } public override int GetHashCode() { return this.m_stringname.GetHashCode(); } public override String ToString() { return m_stringname; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
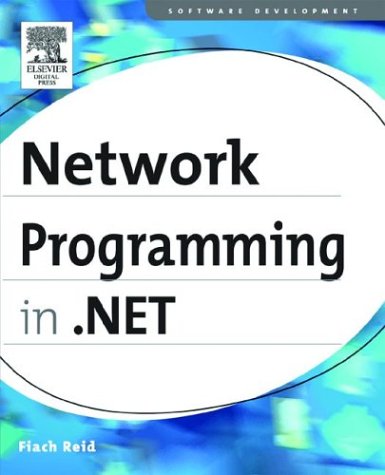
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataServiceQueryProvider.cs
- mda.cs
- TypeForwardedToAttribute.cs
- XmlCharCheckingWriter.cs
- UnsafeNativeMethods.cs
- PartitionerStatic.cs
- DateTimeUtil.cs
- SymbolDocumentGenerator.cs
- _Events.cs
- GradientSpreadMethodValidation.cs
- EllipseGeometry.cs
- AppSettingsExpressionBuilder.cs
- CompoundFileDeflateTransform.cs
- DesignerHost.cs
- SHA384Managed.cs
- sitestring.cs
- ProcessThread.cs
- ListViewCommandEventArgs.cs
- TTSEngineTypes.cs
- EmbeddedMailObjectsCollection.cs
- PassportAuthentication.cs
- ModuleBuilder.cs
- HTMLTextWriter.cs
- XmlSchemaExternal.cs
- DataGridColumnCollection.cs
- AbandonedMutexException.cs
- WebServiceReceiveDesigner.cs
- TypeInfo.cs
- HtmlEmptyTagControlBuilder.cs
- QueryCursorEventArgs.cs
- AlignmentXValidation.cs
- ConfigurationManagerInternal.cs
- WebPartActionVerb.cs
- TraceHelpers.cs
- DataMember.cs
- dsa.cs
- SqlDataSourceStatusEventArgs.cs
- FirewallWrapper.cs
- Parallel.cs
- TextParaClient.cs
- Figure.cs
- Span.cs
- MouseGesture.cs
- Point.cs
- DateTimeValueSerializer.cs
- RowsCopiedEventArgs.cs
- XDeferredAxisSource.cs
- SimpleWebHandlerParser.cs
- BasicDesignerLoader.cs
- CounterSetInstanceCounterDataSet.cs
- ScriptReferenceBase.cs
- HostedNamedPipeTransportManager.cs
- OrthographicCamera.cs
- PropertyGridView.cs
- FilteredXmlReader.cs
- ZoomPercentageConverter.cs
- EventBuilder.cs
- PrintController.cs
- CacheHelper.cs
- counter.cs
- DeferredRunTextReference.cs
- ThaiBuddhistCalendar.cs
- MSHTMLHost.cs
- XPathExpr.cs
- ColumnResizeAdorner.cs
- ModifierKeysValueSerializer.cs
- EncoderReplacementFallback.cs
- XsltException.cs
- RootDesignerSerializerAttribute.cs
- QueueProcessor.cs
- ScaleTransform.cs
- FieldAccessException.cs
- TextBox.cs
- SqlInternalConnectionSmi.cs
- ClientTargetSection.cs
- SafeFindHandle.cs
- Claim.cs
- VectorAnimationUsingKeyFrames.cs
- Visual3D.cs
- ToolboxService.cs
- Normalizer.cs
- CurrentChangingEventManager.cs
- JsonClassDataContract.cs
- CollectionsUtil.cs
- GorillaCodec.cs
- SqlConnectionHelper.cs
- DispatcherSynchronizationContext.cs
- UserPreferenceChangedEventArgs.cs
- cookieexception.cs
- ISAPIApplicationHost.cs
- Point.cs
- SqlClientWrapperSmiStreamChars.cs
- FontStretches.cs
- DisplayNameAttribute.cs
- WebBrowserContainer.cs
- XmlNamedNodeMap.cs
- SelectionUIService.cs
- BitmapEffectState.cs
- ListViewItemMouseHoverEvent.cs
- JsonFormatGeneratorStatics.cs