Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Query / PlanCompiler / ProviderCommandInfoUtils.cs / 1305376 / ProviderCommandInfoUtils.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; //using System.Diagnostics; // Please use PlanCompiler.Assert instead of Debug.Assert in this class... using System.Data.Common.CommandTrees; using System.Data.Common; using md = System.Data.Metadata.Edm; using System.Data.Query.InternalTrees; using System.Data.Query.PlanCompiler; namespace System.Data.Query.PlanCompiler { ////// Helper class for creating a ProviderCommandInfo given an Iqt Node. /// internal static class ProviderCommandInfoUtils { #region Public Methods ////// Creates a ProviderCommandInfo for the given node. /// This method should be called when the keys, foreign keys and sort keys are known ahead of time. /// Typically it is used when the original command is factored into multiple commands. /// /// The owning command, used for creating VarVecs, etc /// The root of the sub-command for which a ProviderCommandInfo should be generated /// A list of ProviderCommandInfos that were created for the child sub-commands. ///The resulting ProviderCommandInfo internal static ProviderCommandInfo Create( Command command, Node node, Listchildren) { PhysicalProjectOp projectOp = node.Op as PhysicalProjectOp; PlanCompiler.Assert(projectOp != null, "Expected root Op to be a physical Project"); // build up the CQT DbCommandTree ctree = CTreeGenerator.Generate(command, node); DbQueryCommandTree cqtree = ctree as DbQueryCommandTree; PlanCompiler.Assert(cqtree != null, "null query command tree"); // Get the rowtype for the result cqt md.CollectionType collType = TypeHelpers.GetEdmType (cqtree.Query.ResultType); PlanCompiler.Assert(md.TypeSemantics.IsRowType(collType.TypeUsage), "command rowtype is not a record"); // Build up a mapping from Vars to the corresponding output property/column Dictionary outputVarMap = BuildOutputVarMap(projectOp, collType.TypeUsage); return new ProviderCommandInfo(ctree, children); } /// /// Creates a ProviderCommandInfo for the given node. /// This method should be called when the keys and the sort keys are not known ahead of time. /// Typically it is used when there is only one command, that is no query factoring is done. /// This method also has the option of pulling up keys and sort information. /// /// The owning command, used for creating VarVecs, etc /// The root of the sub-command for which a ProviderCommandInfo should be generated ///The resulting ProviderCommandInfo internal static ProviderCommandInfo Create( Command command, Node node) { return Create( command, node, new List() //children ); } #endregion #region Private Methods /// /// Build up a mapping from Vars to the corresponding property of the output row type /// /// the physical projectOp /// output type ///a map from Vars to the output type member private static Dictionary BuildOutputVarMap(PhysicalProjectOp projectOp, md.TypeUsage outputType) { Dictionary outputVarMap = new Dictionary(); PlanCompiler.Assert(md.TypeSemantics.IsRowType(outputType), "PhysicalProjectOp result type is not a RowType?"); IEnumeratorpropertyEnumerator = TypeHelpers.GetEdmType (outputType).Properties.GetEnumerator(); IEnumerator varEnumerator = projectOp.Outputs.GetEnumerator(); while (true) { bool foundProp = propertyEnumerator.MoveNext(); bool foundVar = varEnumerator.MoveNext(); if (foundProp != foundVar) { throw EntityUtil.InternalError(EntityUtil.InternalErrorCode.ColumnCountMismatch, 1); } if (!foundProp) { break; } outputVarMap[varEnumerator.Current] = propertyEnumerator.Current; } return outputVarMap; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
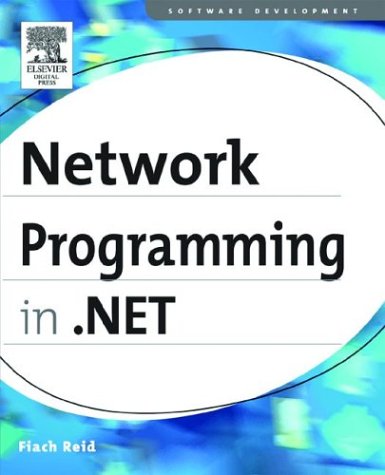
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeView.cs
- HostingEnvironmentException.cs
- AssemblyHash.cs
- ClientTarget.cs
- MessageQueueConverter.cs
- DecoderExceptionFallback.cs
- TextEndOfLine.cs
- SrgsGrammar.cs
- JoinTreeNode.cs
- TextEffectResolver.cs
- TraceRecord.cs
- EmptyControlCollection.cs
- CacheChildrenQuery.cs
- SqlBuilder.cs
- NonDualMessageSecurityOverHttpElement.cs
- DataGridViewRowCollection.cs
- AttributeCollection.cs
- RpcAsyncResult.cs
- AxisAngleRotation3D.cs
- BooleanAnimationBase.cs
- OutputBuffer.cs
- Binding.cs
- ImageKeyConverter.cs
- DataGridViewColumnCollection.cs
- CryptoApi.cs
- EncryptedReference.cs
- MessageQueueAccessControlEntry.cs
- GridViewDeleteEventArgs.cs
- PeerResolverBindingElement.cs
- DataList.cs
- DesignBindingPropertyDescriptor.cs
- SpecialFolderEnumConverter.cs
- Calendar.cs
- DataSourceControlBuilder.cs
- ArgIterator.cs
- SystemInfo.cs
- TypedColumnHandler.cs
- GroupQuery.cs
- CompoundFileStorageReference.cs
- ClientTarget.cs
- HandleCollector.cs
- ConnectionConsumerAttribute.cs
- SafePEFileHandle.cs
- ObjRef.cs
- DataGridCellAutomationPeer.cs
- NavigationHelper.cs
- ActiveXSerializer.cs
- PartialTrustVisibleAssemblyCollection.cs
- PixelFormats.cs
- ServiceReference.cs
- SynchronizationScope.cs
- Selection.cs
- SchemaTypeEmitter.cs
- StartUpEventArgs.cs
- ClientBuildManagerCallback.cs
- Overlapped.cs
- wmiprovider.cs
- TypeSystemProvider.cs
- RealProxy.cs
- PrintPreviewControl.cs
- AttributeProviderAttribute.cs
- XamlWriterExtensions.cs
- SessionPageStateSection.cs
- EntityConnectionStringBuilderItem.cs
- elementinformation.cs
- ReturnValue.cs
- GeneratedCodeAttribute.cs
- CustomCredentialPolicy.cs
- AdRotator.cs
- AspNetSynchronizationContext.cs
- TableLayoutSettingsTypeConverter.cs
- ContentElementAutomationPeer.cs
- RegexCompilationInfo.cs
- XslAstAnalyzer.cs
- ImageCodecInfo.cs
- TranslateTransform3D.cs
- TextTreeTextBlock.cs
- ThreadStartException.cs
- ToolStripDesignerAvailabilityAttribute.cs
- SqlClientPermission.cs
- Canvas.cs
- DataSourceListEditor.cs
- AuthenticationModulesSection.cs
- DataGridViewCell.cs
- OperatingSystem.cs
- Triplet.cs
- RsaSecurityTokenParameters.cs
- MultiBindingExpression.cs
- DataTableClearEvent.cs
- HttpWebRequestElement.cs
- GridViewItemAutomationPeer.cs
- VisualTreeUtils.cs
- InstanceKeyCollisionException.cs
- TextPenaltyModule.cs
- RoutedPropertyChangedEventArgs.cs
- XamlInt32CollectionSerializer.cs
- SafeFindHandle.cs
- MemoryPressure.cs
- SafeNativeMethods.cs
- DefaultAssemblyResolver.cs