Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / PixelFormats.cs / 1 / PixelFormats.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: PixelFormats.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; namespace System.Windows.Media { #region PixelFormats ////// PixelFormats - The collection of supported Pixel Formats /// public static class PixelFormats { ////// Default: for situations when the pixel format may not be important /// public static PixelFormat Default { get { return new PixelFormat(PixelFormatEnum.Default); } } ////// Indexed1: Paletted image with 2 colors. /// public static PixelFormat Indexed1 { get { return new PixelFormat(PixelFormatEnum.Indexed1); } } ////// Indexed2: Paletted image with 4 colors. /// public static PixelFormat Indexed2 { get { return new PixelFormat(PixelFormatEnum.Indexed2); } } ////// Indexed4: Paletted image with 16 colors. /// public static PixelFormat Indexed4 { get { return new PixelFormat(PixelFormatEnum.Indexed4); } } ////// Indexed8: Paletted image with 256 colors. /// public static PixelFormat Indexed8 { get { return new PixelFormat(PixelFormatEnum.Indexed8); } } ////// BlackWhite: Monochrome, 2-color image, black and white only. /// public static PixelFormat BlackWhite { get { return new PixelFormat(PixelFormatEnum.BlackWhite); } } ////// Gray2: Image with 4 shades of gray /// public static PixelFormat Gray2 { get { return new PixelFormat(PixelFormatEnum.Gray2); } } ////// Gray4: Image with 16 shades of gray /// public static PixelFormat Gray4 { get { return new PixelFormat(PixelFormatEnum.Gray4); } } ////// Gray8: Image with 256 shades of gray /// public static PixelFormat Gray8 { get { return new PixelFormat(PixelFormatEnum.Gray8); } } ////// Bgr555: 16 bpp SRGB format /// public static PixelFormat Bgr555 { get { return new PixelFormat(PixelFormatEnum.Bgr555); } } ////// Bgr565: 16 bpp SRGB format /// public static PixelFormat Bgr565 { get { return new PixelFormat(PixelFormatEnum.Bgr565); } } ////// Rgb128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgb128Float { get { return new PixelFormat(PixelFormatEnum.Rgb128Float); } } ////// Bgr24: 24 bpp SRGB format /// public static PixelFormat Bgr24 { get { return new PixelFormat(PixelFormatEnum.Bgr24); } } ////// Rgb24: 24 bpp SRGB format /// public static PixelFormat Rgb24 { get { return new PixelFormat(PixelFormatEnum.Rgb24); } } ////// Bgr101010: 32 bpp SRGB format /// public static PixelFormat Bgr101010 { get { return new PixelFormat(PixelFormatEnum.Bgr101010); } } ////// Bgr32: 32 bpp SRGB format /// public static PixelFormat Bgr32 { get { return new PixelFormat(PixelFormatEnum.Bgr32); } } ////// Bgra32: 32 bpp SRGB format /// public static PixelFormat Bgra32 { get { return new PixelFormat(PixelFormatEnum.Bgra32); } } ////// Pbgra32: 32 bpp SRGB format /// public static PixelFormat Pbgra32 { get { return new PixelFormat(PixelFormatEnum.Pbgra32); } } ////// Rgb48: 48 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgb48 { get { return new PixelFormat(PixelFormatEnum.Rgb48); } } ////// Rgba64: 64 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgba64 { get { return new PixelFormat(PixelFormatEnum.Rgba64); } } ////// Prgba64: 64 bpp extended format; Gamma is 1.0 /// public static PixelFormat Prgba64 { get { return new PixelFormat(PixelFormatEnum.Prgba64); } } ////// Gray16: 16 bpp Gray-scale format; Gamma is 1.0 /// public static PixelFormat Gray16 { get { return new PixelFormat(PixelFormatEnum.Gray16); } } ////// Gray32Float: 32 bpp Gray-scale format; Gamma is 1.0 /// public static PixelFormat Gray32Float { get { return new PixelFormat(PixelFormatEnum.Gray32Float); } } ////// Rgba128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgba128Float { get { return new PixelFormat(PixelFormatEnum.Rgba128Float); } } ////// Prgba128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Prgba128Float { get { return new PixelFormat(PixelFormatEnum.Prgba128Float); } } ////// Cmyk32: 32 bpp format /// public static PixelFormat Cmyk32 { get { return new PixelFormat(PixelFormatEnum.Cmyk32); } } } #endregion // PixelFormats } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: PixelFormats.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; namespace System.Windows.Media { #region PixelFormats ////// PixelFormats - The collection of supported Pixel Formats /// public static class PixelFormats { ////// Default: for situations when the pixel format may not be important /// public static PixelFormat Default { get { return new PixelFormat(PixelFormatEnum.Default); } } ////// Indexed1: Paletted image with 2 colors. /// public static PixelFormat Indexed1 { get { return new PixelFormat(PixelFormatEnum.Indexed1); } } ////// Indexed2: Paletted image with 4 colors. /// public static PixelFormat Indexed2 { get { return new PixelFormat(PixelFormatEnum.Indexed2); } } ////// Indexed4: Paletted image with 16 colors. /// public static PixelFormat Indexed4 { get { return new PixelFormat(PixelFormatEnum.Indexed4); } } ////// Indexed8: Paletted image with 256 colors. /// public static PixelFormat Indexed8 { get { return new PixelFormat(PixelFormatEnum.Indexed8); } } ////// BlackWhite: Monochrome, 2-color image, black and white only. /// public static PixelFormat BlackWhite { get { return new PixelFormat(PixelFormatEnum.BlackWhite); } } ////// Gray2: Image with 4 shades of gray /// public static PixelFormat Gray2 { get { return new PixelFormat(PixelFormatEnum.Gray2); } } ////// Gray4: Image with 16 shades of gray /// public static PixelFormat Gray4 { get { return new PixelFormat(PixelFormatEnum.Gray4); } } ////// Gray8: Image with 256 shades of gray /// public static PixelFormat Gray8 { get { return new PixelFormat(PixelFormatEnum.Gray8); } } ////// Bgr555: 16 bpp SRGB format /// public static PixelFormat Bgr555 { get { return new PixelFormat(PixelFormatEnum.Bgr555); } } ////// Bgr565: 16 bpp SRGB format /// public static PixelFormat Bgr565 { get { return new PixelFormat(PixelFormatEnum.Bgr565); } } ////// Rgb128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgb128Float { get { return new PixelFormat(PixelFormatEnum.Rgb128Float); } } ////// Bgr24: 24 bpp SRGB format /// public static PixelFormat Bgr24 { get { return new PixelFormat(PixelFormatEnum.Bgr24); } } ////// Rgb24: 24 bpp SRGB format /// public static PixelFormat Rgb24 { get { return new PixelFormat(PixelFormatEnum.Rgb24); } } ////// Bgr101010: 32 bpp SRGB format /// public static PixelFormat Bgr101010 { get { return new PixelFormat(PixelFormatEnum.Bgr101010); } } ////// Bgr32: 32 bpp SRGB format /// public static PixelFormat Bgr32 { get { return new PixelFormat(PixelFormatEnum.Bgr32); } } ////// Bgra32: 32 bpp SRGB format /// public static PixelFormat Bgra32 { get { return new PixelFormat(PixelFormatEnum.Bgra32); } } ////// Pbgra32: 32 bpp SRGB format /// public static PixelFormat Pbgra32 { get { return new PixelFormat(PixelFormatEnum.Pbgra32); } } ////// Rgb48: 48 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgb48 { get { return new PixelFormat(PixelFormatEnum.Rgb48); } } ////// Rgba64: 64 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgba64 { get { return new PixelFormat(PixelFormatEnum.Rgba64); } } ////// Prgba64: 64 bpp extended format; Gamma is 1.0 /// public static PixelFormat Prgba64 { get { return new PixelFormat(PixelFormatEnum.Prgba64); } } ////// Gray16: 16 bpp Gray-scale format; Gamma is 1.0 /// public static PixelFormat Gray16 { get { return new PixelFormat(PixelFormatEnum.Gray16); } } ////// Gray32Float: 32 bpp Gray-scale format; Gamma is 1.0 /// public static PixelFormat Gray32Float { get { return new PixelFormat(PixelFormatEnum.Gray32Float); } } ////// Rgba128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgba128Float { get { return new PixelFormat(PixelFormatEnum.Rgba128Float); } } ////// Prgba128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Prgba128Float { get { return new PixelFormat(PixelFormatEnum.Prgba128Float); } } ////// Cmyk32: 32 bpp format /// public static PixelFormat Cmyk32 { get { return new PixelFormat(PixelFormatEnum.Cmyk32); } } } #endregion // PixelFormats } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
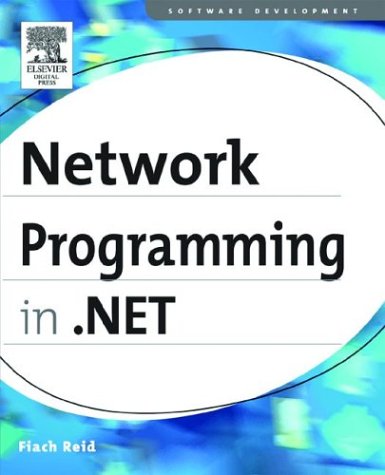
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OleDbTransaction.cs
- CreateUserWizardAutoFormat.cs
- DataGridView.cs
- ThreadAbortException.cs
- WorkerRequest.cs
- HitTestWithPointDrawingContextWalker.cs
- SqlExpressionNullability.cs
- KnownTypesProvider.cs
- AsyncCompletedEventArgs.cs
- AddIn.cs
- ToolboxDataAttribute.cs
- XmlILConstructAnalyzer.cs
- WebHostScriptMappingsInstallComponent.cs
- NavigationCommands.cs
- JsonDeserializer.cs
- PreviewPageInfo.cs
- SafeRightsManagementSessionHandle.cs
- DrawingCollection.cs
- GZipDecoder.cs
- StorageTypeMapping.cs
- ElementAction.cs
- FirewallWrapper.cs
- WebBrowserEvent.cs
- ProbeMatchesMessageCD1.cs
- PowerStatus.cs
- XmlWriterTraceListener.cs
- WebReferencesBuildProvider.cs
- ColorContextHelper.cs
- WebPartMovingEventArgs.cs
- WebPartDesigner.cs
- XamlFrame.cs
- MimePart.cs
- AnonymousIdentificationSection.cs
- DataGridViewCellValidatingEventArgs.cs
- SQLStringStorage.cs
- MenuRendererStandards.cs
- TeredoHelper.cs
- OperationPerformanceCounters.cs
- ActivityStatusChangeEventArgs.cs
- WsdlExporter.cs
- DecoderNLS.cs
- StoreItemCollection.Loader.cs
- DecoratedNameAttribute.cs
- ObjectDataSourceSelectingEventArgs.cs
- Documentation.cs
- UIElementCollection.cs
- Crc32.cs
- AspCompat.cs
- BuildProviderAppliesToAttribute.cs
- BasicSecurityProfileVersion.cs
- WebServiceClientProxyGenerator.cs
- SafeNativeHandle.cs
- PropertyItem.cs
- BamlRecordHelper.cs
- CustomTypeDescriptor.cs
- ProvideValueServiceProvider.cs
- MaskedTextBoxDesigner.cs
- CacheHelper.cs
- ImpersonateTokenRef.cs
- SQLBytesStorage.cs
- ELinqQueryState.cs
- EditorPart.cs
- ParentQuery.cs
- SqlMethodCallConverter.cs
- DataControlLinkButton.cs
- Translator.cs
- RetrieveVirtualItemEventArgs.cs
- UICuesEvent.cs
- cryptoapiTransform.cs
- TransactionTraceIdentifier.cs
- DrawingContextWalker.cs
- SoapReflectionImporter.cs
- BindToObject.cs
- KeyPullup.cs
- ExtendedPropertiesHandler.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- CacheForPrimitiveTypes.cs
- ProfileProvider.cs
- URLMembershipCondition.cs
- RelationshipManager.cs
- RegistryKey.cs
- Win32MouseDevice.cs
- GroupBox.cs
- XmlSchemaGroup.cs
- Dynamic.cs
- FixedSOMElement.cs
- ZipArchive.cs
- AppDomainResourcePerfCounters.cs
- DictionaryMarkupSerializer.cs
- PrivilegeNotHeldException.cs
- MonitorWrapper.cs
- AssemblyAssociatedContentFileAttribute.cs
- StringConverter.cs
- WebBrowserPermission.cs
- ProvideValueServiceProvider.cs
- BaseServiceProvider.cs
- FreezableCollection.cs
- LoadedOrUnloadedOperation.cs
- EdmType.cs
- FormViewPagerRow.cs