Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / XamlBuildTask / Microsoft / Build / Tasks / Xaml / CompilationPass2Task.cs / 1305376 / CompilationPass2Task.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace Microsoft.Build.Tasks.Xaml { using System; using System.Collections.Generic; using Microsoft.Build.Framework; using Microsoft.Build.Utilities; using System.Reflection; using System.Runtime; [Fx.Tag.XamlVisible(true)] public class CompilationPass2Task : Task { public CompilationPass2Task() { } [Fx.Tag.KnownXamlExternal] public ITaskItem[] ApplicationMarkup { get; set; } public string AssemblyName { get; set; } [Fx.Tag.KnownXamlExternal] public ITaskItem[] References { get; set; } public string LocalAssemblyReference { get; set; } public string RootNamespace { get; set; } public string BuildTaskPath { get; set; } public override bool Execute() { AppDomain appDomain = null; try { appDomain = XamlBuildTaskServices.CreateAppDomain("CompilationPass2AppDomain_" + Guid.NewGuid(), BuildTaskPath); CompilationPass2TaskInternal wrapper = (CompilationPass2TaskInternal)appDomain.CreateInstanceAndUnwrap( Assembly.GetExecutingAssembly().FullName, typeof(CompilationPass2TaskInternal).FullName); PopulateBuildArtifacts(wrapper); bool ret = wrapper.Execute(); if (!ret) { foreach (LogData logData in wrapper.LogData) { XamlBuildTaskServices.LogException( this, logData.Message, logData.FileName, logData.LineNumber, logData.LinePosition); } } return ret; } catch (Exception e) { if (Fx.IsFatal(e)) { throw; } XamlBuildTaskServices.LogException(this, e.Message); return false; } finally { if (appDomain != null) { AppDomain.Unload(appDomain); } } } void PopulateBuildArtifacts(CompilationPass2TaskInternal wrapper) { IListapplicationMarkup = new List (this.ApplicationMarkup.Length); foreach (ITaskItem taskItem in this.ApplicationMarkup) { applicationMarkup.Add(taskItem.ItemSpec); } wrapper.ApplicationMarkup = applicationMarkup; IList references = new List (this.References.Length); foreach (ITaskItem reference in this.References) { references.Add(reference.ItemSpec); } wrapper.References = references; wrapper.LocalAssemblyReference = this.LocalAssemblyReference; wrapper.AssemblyName = this.AssemblyName; wrapper.RootNamespace = this.RootNamespace; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace Microsoft.Build.Tasks.Xaml { using System; using System.Collections.Generic; using Microsoft.Build.Framework; using Microsoft.Build.Utilities; using System.Reflection; using System.Runtime; [Fx.Tag.XamlVisible(true)] public class CompilationPass2Task : Task { public CompilationPass2Task() { } [Fx.Tag.KnownXamlExternal] public ITaskItem[] ApplicationMarkup { get; set; } public string AssemblyName { get; set; } [Fx.Tag.KnownXamlExternal] public ITaskItem[] References { get; set; } public string LocalAssemblyReference { get; set; } public string RootNamespace { get; set; } public string BuildTaskPath { get; set; } public override bool Execute() { AppDomain appDomain = null; try { appDomain = XamlBuildTaskServices.CreateAppDomain("CompilationPass2AppDomain_" + Guid.NewGuid(), BuildTaskPath); CompilationPass2TaskInternal wrapper = (CompilationPass2TaskInternal)appDomain.CreateInstanceAndUnwrap( Assembly.GetExecutingAssembly().FullName, typeof(CompilationPass2TaskInternal).FullName); PopulateBuildArtifacts(wrapper); bool ret = wrapper.Execute(); if (!ret) { foreach (LogData logData in wrapper.LogData) { XamlBuildTaskServices.LogException( this, logData.Message, logData.FileName, logData.LineNumber, logData.LinePosition); } } return ret; } catch (Exception e) { if (Fx.IsFatal(e)) { throw; } XamlBuildTaskServices.LogException(this, e.Message); return false; } finally { if (appDomain != null) { AppDomain.Unload(appDomain); } } } void PopulateBuildArtifacts(CompilationPass2TaskInternal wrapper) { IList applicationMarkup = new List (this.ApplicationMarkup.Length); foreach (ITaskItem taskItem in this.ApplicationMarkup) { applicationMarkup.Add(taskItem.ItemSpec); } wrapper.ApplicationMarkup = applicationMarkup; IList references = new List (this.References.Length); foreach (ITaskItem reference in this.References) { references.Add(reference.ItemSpec); } wrapper.References = references; wrapper.LocalAssemblyReference = this.LocalAssemblyReference; wrapper.AssemblyName = this.AssemblyName; wrapper.RootNamespace = this.RootNamespace; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
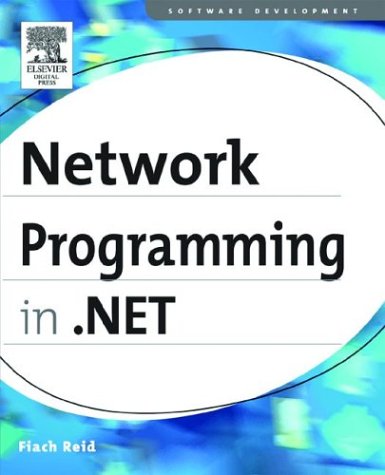
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClientFormsAuthenticationCredentials.cs
- Parser.cs
- GenericWebPart.cs
- TemplateContentLoader.cs
- Control.cs
- XmlUtil.cs
- BigInt.cs
- InfiniteIntConverter.cs
- TableDesigner.cs
- ContainerParaClient.cs
- DatatypeImplementation.cs
- SymLanguageType.cs
- DataObject.cs
- PointLight.cs
- BufferedStream.cs
- IIS7WorkerRequest.cs
- TextBoxBase.cs
- RegisteredScript.cs
- OperatingSystem.cs
- InfoCardListRequest.cs
- WebConfigurationHost.cs
- RemoteWebConfigurationHost.cs
- EndOfStreamException.cs
- PropertyTab.cs
- QuaternionConverter.cs
- MiniModule.cs
- RequestUriProcessor.cs
- HierarchicalDataBoundControlAdapter.cs
- BuildProviderAppliesToAttribute.cs
- ObjectDataSourceStatusEventArgs.cs
- OdbcConnection.cs
- ProfilePropertyNameValidator.cs
- WebBrowserSiteBase.cs
- BindValidator.cs
- ClassicBorderDecorator.cs
- InvalidOleVariantTypeException.cs
- ContainerControl.cs
- BooleanKeyFrameCollection.cs
- FilteredReadOnlyMetadataCollection.cs
- NavigationPropertyAccessor.cs
- MenuStrip.cs
- ButtonFieldBase.cs
- Predicate.cs
- DrawingContextWalker.cs
- Converter.cs
- WebPartDesigner.cs
- FilterEventArgs.cs
- UIElement3D.cs
- Pkcs9Attribute.cs
- StorageComplexTypeMapping.cs
- InvokeGenerator.cs
- Int16AnimationUsingKeyFrames.cs
- ActivityExecutionWorkItem.cs
- InternalRelationshipCollection.cs
- Positioning.cs
- StringComparer.cs
- WebPartMinimizeVerb.cs
- GifBitmapEncoder.cs
- DynamicDocumentPaginator.cs
- FixedSOMContainer.cs
- PerspectiveCamera.cs
- ValidatorAttribute.cs
- SSmlParser.cs
- WindowsTokenRoleProvider.cs
- UserValidatedEventArgs.cs
- CodeNamespaceImportCollection.cs
- FSWPathEditor.cs
- ZipPackagePart.cs
- SQLInt32.cs
- HashMembershipCondition.cs
- OperationFormatUse.cs
- FontWeights.cs
- TailCallAnalyzer.cs
- HttpContextWrapper.cs
- KnownColorTable.cs
- FileUpload.cs
- WCFBuildProvider.cs
- SqlDependency.cs
- filewebrequest.cs
- HMACSHA1.cs
- XmlWriterTraceListener.cs
- ByteStack.cs
- ProvideValueServiceProvider.cs
- HostingEnvironmentException.cs
- SplineQuaternionKeyFrame.cs
- HandlerBase.cs
- datacache.cs
- SmiTypedGetterSetter.cs
- KeyConverter.cs
- TextBox.cs
- ValueConversionAttribute.cs
- IconConverter.cs
- SoapObjectReader.cs
- DataSourceControlBuilder.cs
- TextClipboardData.cs
- SvcMapFileSerializer.cs
- PageVisual.cs
- DockProviderWrapper.cs
- ViewStateException.cs
- XmlSchemaImport.cs