Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / InfoCardListRequest.cs / 1 / InfoCardListRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using System.IO; class InfoCardListRequest : UIAgentRequest { InfoCardPolicy m_policy; IListm_allCards; public InfoCardListRequest( IntPtr rpcHandle, Stream inArgs, Stream outArgs, ClientUIRequest parent ) : base( rpcHandle, inArgs, outArgs, parent ) { } protected override void OnInitializeAsSystem() { base.OnInitializeAsSystem(); m_policy = GetPolicy(); } protected override void OnMarshalInArgs() { // // Nothing to marshall // } protected override void OnProcess() { // // Get the full list of all infocards. // IList rows = ( IList ) GetAllCards(); if ( null != rows && 0 != rows.Count ) { IList cards = new List ( rows.Count ); for ( int i = 0; i < rows.Count; i++ ) { byte[ ] rawForm = rows[ i ].GetDataField(); try { using ( MemoryStream stream = new MemoryStream( rawForm ) ) { cards.Add( new InfoCard( stream ) ); } } finally { Array.Clear( rawForm, 0, rawForm.Length ); } } m_allCards = cards; } } protected override void OnMarshalOutArgs() { Stream stream = OutArgs; BinaryWriter writer = new BinaryWriter( stream ); if ( null != m_allCards && 0 != m_allCards.Count ) { StoreConnection connection = StoreConnection.GetConnection(); try { // // write thte count of the array. // writer.Write( m_allCards.Count ); for ( int i = 0; i < m_allCards.Count; i++ ) { // // Flush the writer to ensure that all data // is committed to the base stream before // we pass the base stream into the card. // writer.Flush(); m_allCards[ i ].AgentSerialize( stream, ( ParentRequest is GetTokenRequest ), m_policy, connection, new System.Globalization.CultureInfo( ParentRequest.UserLanguage ) ); } writer.Flush(); } finally { if ( null != m_allCards ) { foreach ( InfoCard icard in m_allCards ) { icard.ClearSensitiveData(); } } connection.Close(); } } else { // // write the count of the array as 0. // writer.Write( 0 ); } } IList GetAllCards() { StoreConnection connection = StoreConnection.GetConnection(); try { return ( IList ) connection.Query( QueryDetails.FullRow, connection.LocalDataSource, new QueryParameter( SecondaryIndexDefinition.ObjectTypeIndex, (Int32) StorableObjectType.InfoCard ) ); } finally { connection.Close(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
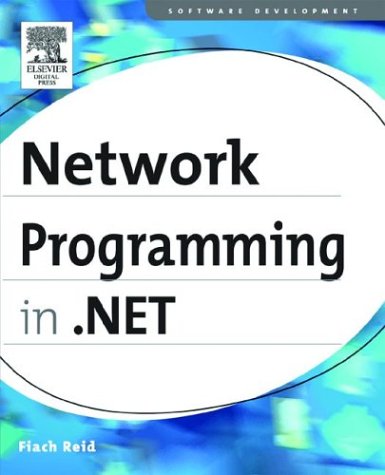
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PageSettings.cs
- SelectionGlyph.cs
- HtmlInputSubmit.cs
- ListItemCollection.cs
- SafePEFileHandle.cs
- SmiSettersStream.cs
- InvokeBinder.cs
- QueryCursorEventArgs.cs
- XmlQuerySequence.cs
- MediaPlayerState.cs
- NavigatorInvalidBodyAccessException.cs
- StylusButton.cs
- AddressHeaderCollectionElement.cs
- compensatingcollection.cs
- HttpRuntime.cs
- ImageAttributes.cs
- ProgressBarRenderer.cs
- ProcessHostMapPath.cs
- BitmapMetadataBlob.cs
- DetailsView.cs
- DesignerDataParameter.cs
- CacheModeValueSerializer.cs
- CustomCategoryAttribute.cs
- DefaultMemberAttribute.cs
- ObjectNotFoundException.cs
- SiteMapNodeItem.cs
- BufferModesCollection.cs
- AsymmetricAlgorithm.cs
- DataGridRelationshipRow.cs
- TableLayoutPanelResizeGlyph.cs
- RoutingConfiguration.cs
- XamlToRtfWriter.cs
- DeviceSpecificChoiceCollection.cs
- AnimationTimeline.cs
- DbModificationCommandTree.cs
- XmlNamedNodeMap.cs
- QueryExtender.cs
- TileBrush.cs
- MulticastNotSupportedException.cs
- ToolStripDropDownItemDesigner.cs
- SQLInt32.cs
- PropertyPath.cs
- ToolboxItemFilterAttribute.cs
- AuthenticatedStream.cs
- PenContexts.cs
- BamlResourceDeserializer.cs
- KeyEvent.cs
- datacache.cs
- CodeRegionDirective.cs
- FormViewUpdateEventArgs.cs
- X509Certificate2Collection.cs
- ContentPosition.cs
- XmlIncludeAttribute.cs
- KeyboardDevice.cs
- PropertyItemInternal.cs
- FileFormatException.cs
- WebControl.cs
- Rotation3DAnimation.cs
- ProfileGroupSettingsCollection.cs
- DataGridViewAdvancedBorderStyle.cs
- DateTimeFormatInfoScanner.cs
- ComplexPropertyEntry.cs
- DataSourceControlBuilder.cs
- KnownTypesHelper.cs
- SmtpNtlmAuthenticationModule.cs
- ProcessHostServerConfig.cs
- StatusCommandUI.cs
- ScaleTransform3D.cs
- ReferencedType.cs
- _NegotiateClient.cs
- BamlRecordHelper.cs
- PseudoWebRequest.cs
- DataStreamFromComStream.cs
- EntryPointNotFoundException.cs
- SerializationInfoEnumerator.cs
- XmlChoiceIdentifierAttribute.cs
- PropertyGeneratedEventArgs.cs
- ThreadAbortException.cs
- PrintDialog.cs
- BaseTreeIterator.cs
- EndpointNotFoundException.cs
- ConstraintStruct.cs
- WebPartTransformerAttribute.cs
- TextPenaltyModule.cs
- PageHandlerFactory.cs
- SoapIgnoreAttribute.cs
- SrgsElementList.cs
- ETagAttribute.cs
- CallbackValidatorAttribute.cs
- ResourceDescriptionAttribute.cs
- StringWriter.cs
- DropSource.cs
- NavigatorInput.cs
- JavaScriptSerializer.cs
- ResourceExpressionBuilder.cs
- SwitchAttribute.cs
- MissingFieldException.cs
- OneOfScalarConst.cs
- StringBlob.cs
- SqlAggregateChecker.cs