Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / IO / StringWriter.cs / 1 / StringWriter.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: StringWriter ** ** Purpose: For writing text to a string ** ** ===========================================================*/ using System; using System.Text; using System.Globalization; namespace System.IO { // This class implements a text writer that writes to a string buffer and allows // the resulting sequence of characters to be presented as a string. // [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public class StringWriter : TextWriter { private static UnicodeEncoding m_encoding=null; private StringBuilder _sb; private bool _isOpen; // Constructs a new StringWriter. A new StringBuilder is automatically // created and associated with the new StringWriter. public StringWriter() : this(new StringBuilder(), CultureInfo.CurrentCulture) { } public StringWriter(IFormatProvider formatProvider) : this(new StringBuilder(), formatProvider) { } // Constructs a new StringWriter that writes to the given StringBuilder. // public StringWriter(StringBuilder sb) : this(sb, CultureInfo.CurrentCulture) { } public StringWriter(StringBuilder sb, IFormatProvider formatProvider) : base(formatProvider) { if (sb==null) throw new ArgumentNullException("sb", Environment.GetResourceString("ArgumentNull_Buffer")); _sb = sb; _isOpen = true; } public override void Close() { Dispose(true); } protected override void Dispose(bool disposing) { // Do not destroy _sb, so that we can extract this after we are // done writing (similar to MemoryStream's GetBuffer & ToArray methods) _isOpen = false; base.Dispose(disposing); } public override Encoding Encoding { get { if (m_encoding==null) { m_encoding = new UnicodeEncoding(false, false); } return m_encoding; } } // Returns the underlying StringBuilder. This is either the StringBuilder // that was passed to the constructor, or the StringBuilder that was // automatically created. // public virtual StringBuilder GetStringBuilder() { return _sb; } // Writes a character to the underlying string buffer. // public override void Write(char value) { if (!_isOpen) __Error.WriterClosed(); _sb.Append(value); } // Writes a range of a character array to the underlying string buffer. // This method will write count characters of data into this // StringWriter from the buffer character array starting at position // index. // public override void Write(char[] buffer, int index, int count) { if (!_isOpen) __Error.WriterClosed(); if (buffer==null) throw new ArgumentNullException("buffer", Environment.GetResourceString("ArgumentNull_Buffer")); if (index < 0) throw new ArgumentOutOfRangeException("index", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (count < 0) throw new ArgumentOutOfRangeException("count", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (buffer.Length - index < count) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidOffLen")); _sb.Append(buffer, index, count); } // Writes a string to the underlying string buffer. If the given string is // null, nothing is written. // public override void Write(String value) { if (!_isOpen) __Error.WriterClosed(); if (value != null) _sb.Append(value); } // Returns a string containing the characters written to this TextWriter // so far. // public override String ToString() { return _sb.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: StringWriter ** ** Purpose: For writing text to a string ** ** ===========================================================*/ using System; using System.Text; using System.Globalization; namespace System.IO { // This class implements a text writer that writes to a string buffer and allows // the resulting sequence of characters to be presented as a string. // [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public class StringWriter : TextWriter { private static UnicodeEncoding m_encoding=null; private StringBuilder _sb; private bool _isOpen; // Constructs a new StringWriter. A new StringBuilder is automatically // created and associated with the new StringWriter. public StringWriter() : this(new StringBuilder(), CultureInfo.CurrentCulture) { } public StringWriter(IFormatProvider formatProvider) : this(new StringBuilder(), formatProvider) { } // Constructs a new StringWriter that writes to the given StringBuilder. // public StringWriter(StringBuilder sb) : this(sb, CultureInfo.CurrentCulture) { } public StringWriter(StringBuilder sb, IFormatProvider formatProvider) : base(formatProvider) { if (sb==null) throw new ArgumentNullException("sb", Environment.GetResourceString("ArgumentNull_Buffer")); _sb = sb; _isOpen = true; } public override void Close() { Dispose(true); } protected override void Dispose(bool disposing) { // Do not destroy _sb, so that we can extract this after we are // done writing (similar to MemoryStream's GetBuffer & ToArray methods) _isOpen = false; base.Dispose(disposing); } public override Encoding Encoding { get { if (m_encoding==null) { m_encoding = new UnicodeEncoding(false, false); } return m_encoding; } } // Returns the underlying StringBuilder. This is either the StringBuilder // that was passed to the constructor, or the StringBuilder that was // automatically created. // public virtual StringBuilder GetStringBuilder() { return _sb; } // Writes a character to the underlying string buffer. // public override void Write(char value) { if (!_isOpen) __Error.WriterClosed(); _sb.Append(value); } // Writes a range of a character array to the underlying string buffer. // This method will write count characters of data into this // StringWriter from the buffer character array starting at position // index. // public override void Write(char[] buffer, int index, int count) { if (!_isOpen) __Error.WriterClosed(); if (buffer==null) throw new ArgumentNullException("buffer", Environment.GetResourceString("ArgumentNull_Buffer")); if (index < 0) throw new ArgumentOutOfRangeException("index", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (count < 0) throw new ArgumentOutOfRangeException("count", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (buffer.Length - index < count) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidOffLen")); _sb.Append(buffer, index, count); } // Writes a string to the underlying string buffer. If the given string is // null, nothing is written. // public override void Write(String value) { if (!_isOpen) __Error.WriterClosed(); if (value != null) _sb.Append(value); } // Returns a string containing the characters written to this TextWriter // so far. // public override String ToString() { return _sb.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
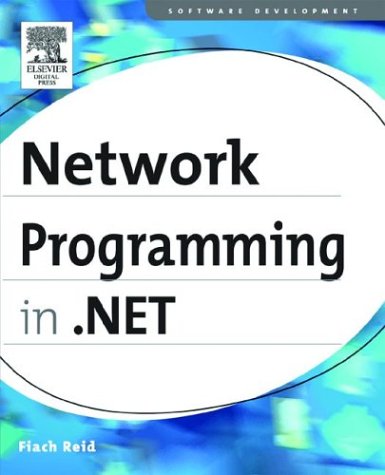
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewCellStyleChangedEventArgs.cs
- ProviderConnectionPoint.cs
- NotConverter.cs
- HtmlTextBoxAdapter.cs
- TransportContext.cs
- MetadataItemSerializer.cs
- LifetimeServices.cs
- WebServiceReceiveDesigner.cs
- SourceFileBuildProvider.cs
- ViewManagerAttribute.cs
- DispatcherEventArgs.cs
- DataColumnMappingCollection.cs
- ScrollEvent.cs
- CalendarSelectionChangedEventArgs.cs
- AlternationConverter.cs
- ScrollItemPatternIdentifiers.cs
- ScrollBar.cs
- SessionPageStatePersister.cs
- CheckBoxBaseAdapter.cs
- AttributeData.cs
- filewebrequest.cs
- LineProperties.cs
- Optimizer.cs
- ReadOnlyDataSource.cs
- PipeStream.cs
- DesignerVerb.cs
- DataConnectionHelper.cs
- CharEnumerator.cs
- IPPacketInformation.cs
- CodeLabeledStatement.cs
- ThousandthOfEmRealPoints.cs
- ConfigurationLocation.cs
- EntityCodeGenerator.cs
- CopyOnWriteList.cs
- DecoratedNameAttribute.cs
- EventMappingSettings.cs
- MarkupExtensionReturnTypeAttribute.cs
- HtmlTable.cs
- Label.cs
- DataGridView.cs
- DbConnectionClosed.cs
- CacheHelper.cs
- GradientStop.cs
- ComplexType.cs
- XmlCharCheckingReader.cs
- ZoomingMessageFilter.cs
- FixedTextSelectionProcessor.cs
- ComponentEvent.cs
- MarkedHighlightComponent.cs
- XmlDataCollection.cs
- TypedDatasetGenerator.cs
- TdsEnums.cs
- OletxResourceManager.cs
- WeakReferenceList.cs
- GroupDescription.cs
- _NativeSSPI.cs
- GridLength.cs
- ComponentDispatcher.cs
- X509CertificateValidator.cs
- ListViewTableRow.cs
- CustomCategoryAttribute.cs
- Highlights.cs
- ThreadExceptionDialog.cs
- ErrorEventArgs.cs
- BaseDataBoundControl.cs
- TemplateInstanceAttribute.cs
- NamedElement.cs
- HttpDebugHandler.cs
- AttributeSetAction.cs
- Registry.cs
- ListViewItemCollectionEditor.cs
- IteratorDescriptor.cs
- ParentUndoUnit.cs
- SafeFileMapViewHandle.cs
- PrimitiveType.cs
- ShaderEffect.cs
- ListViewAutomationPeer.cs
- IISUnsafeMethods.cs
- ProxyHwnd.cs
- AccessDataSourceView.cs
- X509SecurityTokenProvider.cs
- ShaderRenderModeValidation.cs
- __FastResourceComparer.cs
- NativeMethods.cs
- AccessDataSource.cs
- TextElementEnumerator.cs
- DesigntimeLicenseContext.cs
- StrokeCollectionDefaultValueFactory.cs
- MonthChangedEventArgs.cs
- TraceContextRecord.cs
- OptimalBreakSession.cs
- SerializerDescriptor.cs
- Configuration.cs
- SecuritySessionSecurityTokenProvider.cs
- LineGeometry.cs
- GridViewRowEventArgs.cs
- XmlnsCache.cs
- ScrollPatternIdentifiers.cs
- EntityDataSourceDesigner.cs
- BamlRecordHelper.cs