Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / UIAutomation / UIAutomationTypes / System / Windows / Automation / ScrollPatternIdentifiers.cs / 1 / ScrollPatternIdentifiers.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Automation Identifiers for Scroll Pattern // // History: // 04/15/2005 : MKarr Added // //--------------------------------------------------------------------------- using System; using MS.Internal.Automation; using System.Runtime.InteropServices; namespace System.Windows.Automation { ////// Used by ScrollPattern to indicate how much to scroll by /// [ComVisible(true)] [Guid("bd52d3c7-f990-4c52-9ae3-5c377e9eb772")] #if (NO_INTERNAL_COMPILE_BUG1080665) internal enum ScrollAmount #else public enum ScrollAmount #endif { ////// Scroll back by a large value typically the amount equal to PageUp /// or invoking a scrollbar between the up arrow and the thumb. /// If PageUp is not a relevant amount for the control and no scrollbar /// exists, LargeValue represents an amount equal to the /// current visible window. /// LargeDecrement, ////// Scroll back by a small value typically the amount equal to the /// Up or left arrow or invoking the arrow buttons on a scrollbar. /// SmallDecrement, ////// used to allow for no movement is a given direction. /// NoAmount, ////// Scroll forward by a large value typically the amount equal to PageDown /// or invoking a scrollbar between the down arrow and the thumb. /// If PageDown is not a relevant amount for the control and no scrollbar /// exists, LargeValue represents an amount equal to the /// current visible window. /// LargeIncrement, ////// Scroll forwards by a small value typically the amount equal to the /// Down or right arrow or invoking the arrow buttons on a scrollbar. /// SmallIncrement } ////// Represents UI elements that are expressing a value /// #if (INTERNAL_COMPILE) internal static class ScrollPatternIdentifiers #else public static class ScrollPatternIdentifiers #endif { //----------------------------------------------------- // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Value used by SetSCrollPercent to indicate that no scrolling should take place in the specified direction public const double NoScroll = -1.0; ///Scroll pattern public static readonly AutomationPattern Pattern = AutomationPattern.Register(AutomationIdentifierGuids.Scroll_Pattern, "ScrollPatternIdentifiers.Pattern"); ///Property ID: HorizontalScrollPercent - Current horizontal scroll position public static readonly AutomationProperty HorizontalScrollPercentProperty = AutomationProperty.Register(AutomationIdentifierGuids.Scroll_HorizontalScrollPercent_Property, "ScrollPatternIdentifiers.HorizontalScrollPercentProperty"); ///Property ID: HorizontalViewSize - Minimum possible horizontal scroll position public static readonly AutomationProperty HorizontalViewSizeProperty = AutomationProperty.Register(AutomationIdentifierGuids.Scroll_HorizontalViewSize_Property, "ScrollPatternIdentifiers.HorizontalViewSizeProperty"); ///Property ID: VerticalScrollPercent - Current vertical scroll position public static readonly AutomationProperty VerticalScrollPercentProperty = AutomationProperty.Register(AutomationIdentifierGuids.Scroll_VerticalScrollPercent_Property, "ScrollPatternIdentifiers.VerticalScrollPercentProperty"); ///Property ID: VerticalViewSize public static readonly AutomationProperty VerticalViewSizeProperty = AutomationProperty.Register(AutomationIdentifierGuids.Scroll_VerticalViewSize_Property, "ScrollPatternIdentifiers.VerticalViewSizeProperty"); ///Property ID: HorizontallyScrollable public static readonly AutomationProperty HorizontallyScrollableProperty = AutomationProperty.Register(AutomationIdentifierGuids.Scroll_HorizontallyScrollable_Property, "ScrollPatternIdentifiers.HorizontallyScrollableProperty"); ///Property ID: VerticallyScrollable public static readonly AutomationProperty VerticallyScrollableProperty = AutomationProperty.Register(AutomationIdentifierGuids.Scroll_VerticallyScrollable_Property, "ScrollPatternIdentifiers.VerticallyScrollableProperty"); #endregion Public Constants and Readonly Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Automation Identifiers for Scroll Pattern // // History: // 04/15/2005 : MKarr Added // //--------------------------------------------------------------------------- using System; using MS.Internal.Automation; using System.Runtime.InteropServices; namespace System.Windows.Automation { ////// Used by ScrollPattern to indicate how much to scroll by /// [ComVisible(true)] [Guid("bd52d3c7-f990-4c52-9ae3-5c377e9eb772")] #if (NO_INTERNAL_COMPILE_BUG1080665) internal enum ScrollAmount #else public enum ScrollAmount #endif { ////// Scroll back by a large value typically the amount equal to PageUp /// or invoking a scrollbar between the up arrow and the thumb. /// If PageUp is not a relevant amount for the control and no scrollbar /// exists, LargeValue represents an amount equal to the /// current visible window. /// LargeDecrement, ////// Scroll back by a small value typically the amount equal to the /// Up or left arrow or invoking the arrow buttons on a scrollbar. /// SmallDecrement, ////// used to allow for no movement is a given direction. /// NoAmount, ////// Scroll forward by a large value typically the amount equal to PageDown /// or invoking a scrollbar between the down arrow and the thumb. /// If PageDown is not a relevant amount for the control and no scrollbar /// exists, LargeValue represents an amount equal to the /// current visible window. /// LargeIncrement, ////// Scroll forwards by a small value typically the amount equal to the /// Down or right arrow or invoking the arrow buttons on a scrollbar. /// SmallIncrement } ////// Represents UI elements that are expressing a value /// #if (INTERNAL_COMPILE) internal static class ScrollPatternIdentifiers #else public static class ScrollPatternIdentifiers #endif { //----------------------------------------------------- // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Value used by SetSCrollPercent to indicate that no scrolling should take place in the specified direction public const double NoScroll = -1.0; ///Scroll pattern public static readonly AutomationPattern Pattern = AutomationPattern.Register(AutomationIdentifierGuids.Scroll_Pattern, "ScrollPatternIdentifiers.Pattern"); ///Property ID: HorizontalScrollPercent - Current horizontal scroll position public static readonly AutomationProperty HorizontalScrollPercentProperty = AutomationProperty.Register(AutomationIdentifierGuids.Scroll_HorizontalScrollPercent_Property, "ScrollPatternIdentifiers.HorizontalScrollPercentProperty"); ///Property ID: HorizontalViewSize - Minimum possible horizontal scroll position public static readonly AutomationProperty HorizontalViewSizeProperty = AutomationProperty.Register(AutomationIdentifierGuids.Scroll_HorizontalViewSize_Property, "ScrollPatternIdentifiers.HorizontalViewSizeProperty"); ///Property ID: VerticalScrollPercent - Current vertical scroll position public static readonly AutomationProperty VerticalScrollPercentProperty = AutomationProperty.Register(AutomationIdentifierGuids.Scroll_VerticalScrollPercent_Property, "ScrollPatternIdentifiers.VerticalScrollPercentProperty"); ///Property ID: VerticalViewSize public static readonly AutomationProperty VerticalViewSizeProperty = AutomationProperty.Register(AutomationIdentifierGuids.Scroll_VerticalViewSize_Property, "ScrollPatternIdentifiers.VerticalViewSizeProperty"); ///Property ID: HorizontallyScrollable public static readonly AutomationProperty HorizontallyScrollableProperty = AutomationProperty.Register(AutomationIdentifierGuids.Scroll_HorizontallyScrollable_Property, "ScrollPatternIdentifiers.HorizontallyScrollableProperty"); ///Property ID: VerticallyScrollable public static readonly AutomationProperty VerticallyScrollableProperty = AutomationProperty.Register(AutomationIdentifierGuids.Scroll_VerticallyScrollable_Property, "ScrollPatternIdentifiers.VerticallyScrollableProperty"); #endregion Public Constants and Readonly Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
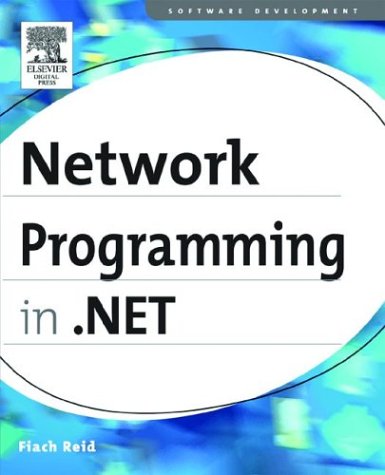
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ParseHttpDate.cs
- RadioButton.cs
- TextCompositionEventArgs.cs
- TableLayoutSettingsTypeConverter.cs
- RenderCapability.cs
- Stack.cs
- Events.cs
- TextTreeFixupNode.cs
- ImageAnimator.cs
- DesignerMetadata.cs
- SystemFonts.cs
- SafeFindHandle.cs
- TableCellAutomationPeer.cs
- EnumValAlphaComparer.cs
- MouseEventArgs.cs
- CurrentChangingEventManager.cs
- CustomValidator.cs
- InfoCardUIAgent.cs
- BaseDataList.cs
- SecurityToken.cs
- SQLInt64.cs
- ConfigXmlAttribute.cs
- ObjectHelper.cs
- TCPClient.cs
- WebPartConnectionsCancelVerb.cs
- TextContainer.cs
- DataContractSerializerMessageContractImporter.cs
- TrackBarRenderer.cs
- XmlILModule.cs
- Single.cs
- TableRow.cs
- EncryptedPackageFilter.cs
- QilReplaceVisitor.cs
- Color.cs
- DispatcherEventArgs.cs
- DecoderReplacementFallback.cs
- ScrollContentPresenter.cs
- TrackingDataItem.cs
- PointConverter.cs
- MessageQueueException.cs
- TraceUtils.cs
- SortQuery.cs
- PolicyVersion.cs
- SQLByteStorage.cs
- XmlDataLoader.cs
- DoWorkEventArgs.cs
- CollectionViewGroupInternal.cs
- BasicExpandProvider.cs
- Propagator.JoinPropagator.cs
- HttpFileCollection.cs
- TypeElement.cs
- DependencyProperty.cs
- StoreItemCollection.Loader.cs
- ipaddressinformationcollection.cs
- CodeTypeMemberCollection.cs
- ListViewItemEventArgs.cs
- DataStreamFromComStream.cs
- FacetValues.cs
- SafeCoTaskMem.cs
- NavigationWindow.cs
- Trace.cs
- RangeContentEnumerator.cs
- GB18030Encoding.cs
- EditingCommands.cs
- NameSpaceExtractor.cs
- ServiceModelConfigurationSectionGroup.cs
- DynamicMetaObjectBinder.cs
- EdmToObjectNamespaceMap.cs
- TileBrush.cs
- SQLBoolean.cs
- XmlSchemaCompilationSettings.cs
- DataServiceException.cs
- Dictionary.cs
- TypeDescriptor.cs
- x509store.cs
- PlatformCulture.cs
- SafeNativeMethods.cs
- QilTypeChecker.cs
- BoolExpression.cs
- ElementHostPropertyMap.cs
- SqlWriter.cs
- ClientSideQueueItem.cs
- CodeGen.cs
- ToolboxDataAttribute.cs
- SizeKeyFrameCollection.cs
- DataControlCommands.cs
- DecimalConstantAttribute.cs
- XsdDuration.cs
- HtmlForm.cs
- ValidatingPropertiesEventArgs.cs
- RegexFCD.cs
- SiblingIterators.cs
- SelectorAutomationPeer.cs
- CharUnicodeInfo.cs
- XmlSortKey.cs
- ZoneMembershipCondition.cs
- EmbeddedMailObjectsCollection.cs
- Rotation3DAnimation.cs
- IPHostEntry.cs
- SafeSecurityHelper.cs