Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Metadata / ObjectHelper.cs / 2 / ObjectHelper.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Data.Mapping; using System.Diagnostics; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Common.Utils; using System.IO; namespace System.Data.Metadata.Edm { ////// Helper Class for EDM Metadata - this class contains all the helper methods /// which needs access to internal methods. The other partial class contains all /// helper methods which just uses public methods/properties. The reason why we /// did this for allowing view gen to happen at compile time - all the helper /// methods that view gen or mapping uses are in the other helper class. Rest of the /// methods are in this class /// internal static partial class Helper { #region Fields // List of all the static empty list used all over the code internal static readonly ReadOnlyCollection> EmptyKeyValueStringObjectList = new ReadOnlyCollection >(new KeyValuePair [0]); internal static readonly ReadOnlyCollection EmptyStringList = new ReadOnlyCollection (new string[0]); internal static readonly ReadOnlyCollection EmptyFacetDescriptionEnumerable = new ReadOnlyCollection (new FacetDescription[0]); internal static readonly ReadOnlyCollection EmptyEdmFunctionReadOnlyCollection = new ReadOnlyCollection (new EdmFunction[0]); internal static readonly ReadOnlyCollection EmptyPrimitiveTypeReadOnlyCollection = new ReadOnlyCollection (new PrimitiveType[0]); internal static readonly KeyValuePair [] EmptyKeyValueStringObjectArray = new KeyValuePair [0]; internal const char PeriodSymbol = '.'; internal const char CommaSymbol = ','; #endregion #region Methods /// /// Returns the single error message from the list of errors /// /// ///static internal string CombineErrorMessage(IEnumerable errors) { Debug.Assert(errors != null); StringBuilder sb = new StringBuilder(System.Environment.NewLine); int count = 0; foreach (System.Data.Metadata.Edm.EdmSchemaError error in errors) { //Don't append a new line at the beginning of the messages if ((count++) != 0) { sb.Append(System.Environment.NewLine); } sb.Append(error.ToString()); } Debug.Assert(count!=0, "Empty Error List"); return sb.ToString(); } /// /// Returns the single error message from the list of errors /// /// ///static internal string CombineErrorMessage(IEnumerable errors) { StringBuilder sb = new StringBuilder(System.Environment.NewLine); int count = 0; foreach (EdmItemError error in errors) { // Only add the new line if this is not the first error if ((count++) != 0) { sb.Append(System.Environment.NewLine); } sb.Append(error.Message); } return sb.ToString(); } // requires: enumerations must have the same number of members // effects: returns paired enumeration values internal static IEnumerable > PairEnumerations (IBaseList left, IEnumerable right) { IEnumerator leftEnumerator = left.GetEnumerator(); IEnumeratorrightEnumerator = right.GetEnumerator(); while (leftEnumerator.MoveNext() && rightEnumerator.MoveNext()) { yield return new KeyValuePair((T)leftEnumerator.Current, rightEnumerator.Current); } yield break; } /// /// Returns a model (C-Space) typeusage for the given typeusage. if the type is already in c-space, it returns /// the given typeusage. The typeUsage returned is created by invoking the provider service to map from provider /// specific type to model type. /// /// typeusage ///the respective Model (C-Space) typeusage static internal TypeUsage GetModelTypeUsage(TypeUsage typeUsage) { return typeUsage.GetModelTypeUsage(); } ////// Returns a model (C-Space) typeusage for the given member typeusage. if the type is already in c-space, it returns /// the given typeusage. The typeUsage returned is created by invoking the provider service to map from provider /// specific type to model type. /// /// EdmMember ///the respective Model (C-Space) typeusage static internal TypeUsage GetModelTypeUsage(EdmMember member) { return GetModelTypeUsage(member.TypeUsage); } ////// Checks if the edm type in the cspace type usage maps to some sspace type (called it S1). If S1 is equivalent or /// promotable to the store type in sspace type usage, then it creates a new type usage with S1 and copies all facets /// if necessary /// /// Edm property containing the cspace member type information /// edm property containing the sspace member type information /// name of the mapping file from which this information was loaded from /// lineInfo containing the line information about the cspace and sspace property mapping /// List of parsing errors - we need to add any new error to this list /// store item collection ///internal static TypeUsage ValidateAndConvertTypeUsage(EdmProperty edmProperty, EdmProperty columnProperty, Xml.IXmlLineInfo lineInfo, string sourceLocation, List parsingErrors, StoreItemCollection storeItemCollection) { Debug.Assert(edmProperty.TypeUsage.EdmType.DataSpace == DataSpace.CSpace, "cspace property must have a cspace type"); Debug.Assert(columnProperty.TypeUsage.EdmType.DataSpace == DataSpace.SSpace, "sspace type usage must have a sspace type"); Debug.Assert(Helper.IsPrimitiveType(edmProperty.TypeUsage.EdmType), "cspace property must contain a primitive type"); Debug.Assert(Helper.IsPrimitiveType(columnProperty.TypeUsage.EdmType), "sspace property must contain a primitive type"); TypeUsage mappedStoreType = ValidateAndConvertTypeUsage(edmProperty, lineInfo, sourceLocation, edmProperty.TypeUsage, columnProperty.TypeUsage, parsingErrors, storeItemCollection); return mappedStoreType; } internal static TypeUsage ValidateAndConvertTypeUsage(EdmMember edmMember, Xml.IXmlLineInfo lineInfo, string sourceLocation, TypeUsage cspaceType, TypeUsage sspaceType, List parsingErrors, StoreItemCollection storeItemCollection) { // if we are already C-Space, dont call the provider. this can happen for functions. TypeUsage modelEquivalentSspace = sspaceType; if (sspaceType.EdmType.DataSpace == DataSpace.SSpace) { modelEquivalentSspace = sspaceType.GetModelTypeUsage(); } // check that cspace type is subtype of c-space equivalent type from the ssdl definition if (TypeSemantics.IsSubTypeOf(cspaceType, modelEquivalentSspace)) { return modelEquivalentSspace; } return null; } /// /// Checks whether the given primitive type is present in the list of promotion types /// /// /// ///internal static int IsPromotableTo(IList promotionTypes, PrimitiveType primitiveType) { for (int i = 0; i < promotionTypes.Count; i++) { if (Object.ReferenceEquals(promotionTypes[i], primitiveType)) { return i; } } return -1; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Data.Mapping; using System.Diagnostics; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Common.Utils; using System.IO; namespace System.Data.Metadata.Edm { ////// Helper Class for EDM Metadata - this class contains all the helper methods /// which needs access to internal methods. The other partial class contains all /// helper methods which just uses public methods/properties. The reason why we /// did this for allowing view gen to happen at compile time - all the helper /// methods that view gen or mapping uses are in the other helper class. Rest of the /// methods are in this class /// internal static partial class Helper { #region Fields // List of all the static empty list used all over the code internal static readonly ReadOnlyCollection> EmptyKeyValueStringObjectList = new ReadOnlyCollection >(new KeyValuePair [0]); internal static readonly ReadOnlyCollection EmptyStringList = new ReadOnlyCollection (new string[0]); internal static readonly ReadOnlyCollection EmptyFacetDescriptionEnumerable = new ReadOnlyCollection (new FacetDescription[0]); internal static readonly ReadOnlyCollection EmptyEdmFunctionReadOnlyCollection = new ReadOnlyCollection (new EdmFunction[0]); internal static readonly ReadOnlyCollection EmptyPrimitiveTypeReadOnlyCollection = new ReadOnlyCollection (new PrimitiveType[0]); internal static readonly KeyValuePair [] EmptyKeyValueStringObjectArray = new KeyValuePair [0]; internal const char PeriodSymbol = '.'; internal const char CommaSymbol = ','; #endregion #region Methods /// /// Returns the single error message from the list of errors /// /// ///static internal string CombineErrorMessage(IEnumerable errors) { Debug.Assert(errors != null); StringBuilder sb = new StringBuilder(System.Environment.NewLine); int count = 0; foreach (System.Data.Metadata.Edm.EdmSchemaError error in errors) { //Don't append a new line at the beginning of the messages if ((count++) != 0) { sb.Append(System.Environment.NewLine); } sb.Append(error.ToString()); } Debug.Assert(count!=0, "Empty Error List"); return sb.ToString(); } /// /// Returns the single error message from the list of errors /// /// ///static internal string CombineErrorMessage(IEnumerable errors) { StringBuilder sb = new StringBuilder(System.Environment.NewLine); int count = 0; foreach (EdmItemError error in errors) { // Only add the new line if this is not the first error if ((count++) != 0) { sb.Append(System.Environment.NewLine); } sb.Append(error.Message); } return sb.ToString(); } // requires: enumerations must have the same number of members // effects: returns paired enumeration values internal static IEnumerable > PairEnumerations (IBaseList left, IEnumerable right) { IEnumerator leftEnumerator = left.GetEnumerator(); IEnumeratorrightEnumerator = right.GetEnumerator(); while (leftEnumerator.MoveNext() && rightEnumerator.MoveNext()) { yield return new KeyValuePair((T)leftEnumerator.Current, rightEnumerator.Current); } yield break; } /// /// Returns a model (C-Space) typeusage for the given typeusage. if the type is already in c-space, it returns /// the given typeusage. The typeUsage returned is created by invoking the provider service to map from provider /// specific type to model type. /// /// typeusage ///the respective Model (C-Space) typeusage static internal TypeUsage GetModelTypeUsage(TypeUsage typeUsage) { return typeUsage.GetModelTypeUsage(); } ////// Returns a model (C-Space) typeusage for the given member typeusage. if the type is already in c-space, it returns /// the given typeusage. The typeUsage returned is created by invoking the provider service to map from provider /// specific type to model type. /// /// EdmMember ///the respective Model (C-Space) typeusage static internal TypeUsage GetModelTypeUsage(EdmMember member) { return GetModelTypeUsage(member.TypeUsage); } ////// Checks if the edm type in the cspace type usage maps to some sspace type (called it S1). If S1 is equivalent or /// promotable to the store type in sspace type usage, then it creates a new type usage with S1 and copies all facets /// if necessary /// /// Edm property containing the cspace member type information /// edm property containing the sspace member type information /// name of the mapping file from which this information was loaded from /// lineInfo containing the line information about the cspace and sspace property mapping /// List of parsing errors - we need to add any new error to this list /// store item collection ///internal static TypeUsage ValidateAndConvertTypeUsage(EdmProperty edmProperty, EdmProperty columnProperty, Xml.IXmlLineInfo lineInfo, string sourceLocation, List parsingErrors, StoreItemCollection storeItemCollection) { Debug.Assert(edmProperty.TypeUsage.EdmType.DataSpace == DataSpace.CSpace, "cspace property must have a cspace type"); Debug.Assert(columnProperty.TypeUsage.EdmType.DataSpace == DataSpace.SSpace, "sspace type usage must have a sspace type"); Debug.Assert(Helper.IsPrimitiveType(edmProperty.TypeUsage.EdmType), "cspace property must contain a primitive type"); Debug.Assert(Helper.IsPrimitiveType(columnProperty.TypeUsage.EdmType), "sspace property must contain a primitive type"); TypeUsage mappedStoreType = ValidateAndConvertTypeUsage(edmProperty, lineInfo, sourceLocation, edmProperty.TypeUsage, columnProperty.TypeUsage, parsingErrors, storeItemCollection); return mappedStoreType; } internal static TypeUsage ValidateAndConvertTypeUsage(EdmMember edmMember, Xml.IXmlLineInfo lineInfo, string sourceLocation, TypeUsage cspaceType, TypeUsage sspaceType, List parsingErrors, StoreItemCollection storeItemCollection) { // if we are already C-Space, dont call the provider. this can happen for functions. TypeUsage modelEquivalentSspace = sspaceType; if (sspaceType.EdmType.DataSpace == DataSpace.SSpace) { modelEquivalentSspace = sspaceType.GetModelTypeUsage(); } // check that cspace type is subtype of c-space equivalent type from the ssdl definition if (TypeSemantics.IsSubTypeOf(cspaceType, modelEquivalentSspace)) { return modelEquivalentSspace; } return null; } /// /// Checks whether the given primitive type is present in the list of promotion types /// /// /// ///internal static int IsPromotableTo(IList promotionTypes, PrimitiveType primitiveType) { for (int i = 0; i < promotionTypes.Count; i++) { if (Object.ReferenceEquals(promotionTypes[i], primitiveType)) { return i; } } return -1; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
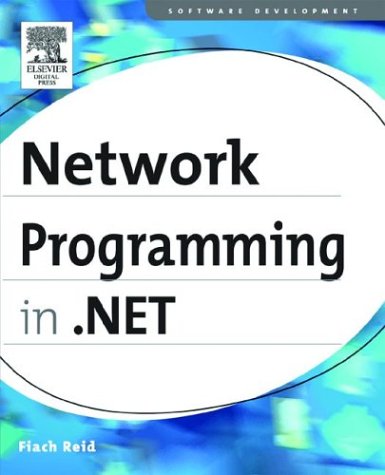
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- KernelTypeValidation.cs
- ImageKeyConverter.cs
- MatrixValueSerializer.cs
- PrinterUnitConvert.cs
- AssociatedControlConverter.cs
- DoubleLinkListEnumerator.cs
- SqlClientMetaDataCollectionNames.cs
- HttpRuntimeSection.cs
- Maps.cs
- SrgsElement.cs
- PrimitiveSchema.cs
- SystemUdpStatistics.cs
- COM2FontConverter.cs
- DiscoveryServerProtocol.cs
- NotifyCollectionChangedEventArgs.cs
- InstallerTypeAttribute.cs
- GridErrorDlg.cs
- ConfigXmlText.cs
- HashCryptoHandle.cs
- BeginEvent.cs
- DesignerView.Commands.cs
- EqualityComparer.cs
- ScaleTransform3D.cs
- XmlArrayItemAttribute.cs
- TableRowsCollectionEditor.cs
- Invariant.cs
- Listbox.cs
- ReversePositionQuery.cs
- SwitchLevelAttribute.cs
- WebPartZoneCollection.cs
- MenuCommandsChangedEventArgs.cs
- SchemaElementDecl.cs
- DrawingCollection.cs
- FloaterParaClient.cs
- PerfCounters.cs
- CurrencyWrapper.cs
- CultureSpecificStringDictionary.cs
- EncodingNLS.cs
- TreeView.cs
- ProcessModuleDesigner.cs
- ScriptManagerProxy.cs
- _ListenerResponseStream.cs
- _SSPIWrapper.cs
- View.cs
- SafeCryptoKeyHandle.cs
- DataGridHyperlinkColumn.cs
- CodeNamespace.cs
- TableLayoutColumnStyleCollection.cs
- MouseDevice.cs
- SpecularMaterial.cs
- Mutex.cs
- HttpDictionary.cs
- COSERVERINFO.cs
- XmlDataCollection.cs
- ISO2022Encoding.cs
- ComponentEditorPage.cs
- SqlRemoveConstantOrderBy.cs
- BrushValueSerializer.cs
- InputScope.cs
- XmlWriterSettings.cs
- DeviceOverridableAttribute.cs
- BitmapImage.cs
- DataObject.cs
- AutomationEventArgs.cs
- CqlLexerHelpers.cs
- _NestedSingleAsyncResult.cs
- IdentityHolder.cs
- SqlXml.cs
- DataObjectEventArgs.cs
- SoapSchemaImporter.cs
- CommandDevice.cs
- WebEncodingValidatorAttribute.cs
- CFGGrammar.cs
- SimpleFileLog.cs
- UnknownBitmapEncoder.cs
- AudioFormatConverter.cs
- WindowsToolbarAsMenu.cs
- UserInitiatedNavigationPermission.cs
- ListViewGroup.cs
- RuleSetDialog.Designer.cs
- SubpageParaClient.cs
- OutputCacheModule.cs
- QueueNameHelper.cs
- Dispatcher.cs
- CodeSubDirectoriesCollection.cs
- DataGridViewRow.cs
- SiteMapNodeCollection.cs
- RegionInfo.cs
- DesignerToolStripControlHost.cs
- EndpointConfigContainer.cs
- SoapMessage.cs
- JsonDataContract.cs
- CommandField.cs
- SymLanguageVendor.cs
- Floater.cs
- IteratorFilter.cs
- MonitoringDescriptionAttribute.cs
- BamlResourceContent.cs
- Drawing.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs