Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / DesignerToolStripControlHost.cs / 1 / DesignerToolStripControlHost.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.Design; using Accessibility; using System.ComponentModel; using System.Diagnostics; using System; using System.Security; using System.Security.Permissions; using System.ComponentModel.Design; using System.Windows.Forms; using System.Drawing; using System.Drawing.Design; using System.Windows.Forms.Design.Behavior; ////// /// This internal class is used by the new ToolStripDesigner to add a dummy /// node to the end. This class inherits from WinBarControlHost and overrides the /// CanSelect property so that the dummy Node when shown in the designer doesnt show /// selection on Mouse movements. /// The image is set to theDummyNodeImage embedded into the resources. /// ///internal class DesignerToolStripControlHost : ToolStripControlHost, IComponent { private BehaviorService b; internal ToolStrip parent=null; // // Constructor // /// public DesignerToolStripControlHost(Control c) : base(c) { // this ToolStripItem should not have defaultPadding. this.Margin = Padding.Empty; } /// /// /// We need to return Default size for Editor ToolStrip (92, 22). /// protected override Size DefaultSize { get { return new Size(92, 22); } } internal GlyphCollection GetGlyphs(ToolStrip parent, GlyphCollection glyphs, System.Windows.Forms.Design.Behavior.Behavior standardBehavior) { if (b == null) { b = (BehaviorService)parent.Site.GetService(typeof(BehaviorService)); } Point loc = b.ControlToAdornerWindow(this.Parent); Rectangle r = this.Bounds; r.Offset(loc); r.Inflate (-2 , -2); glyphs.Add(new MiniLockedBorderGlyph(r, SelectionBorderGlyphType.Top, standardBehavior, true)); glyphs.Add(new MiniLockedBorderGlyph(r, SelectionBorderGlyphType.Bottom, standardBehavior, true)); glyphs.Add(new MiniLockedBorderGlyph(r, SelectionBorderGlyphType.Left, standardBehavior, true)); glyphs.Add(new MiniLockedBorderGlyph(r, SelectionBorderGlyphType.Right, standardBehavior, true)); return glyphs; } internal void RefreshSelectionGlyph() { ToolStrip miniToolStrip = this.Control as ToolStrip; if (miniToolStrip != null) { ToolStripTemplateNode.MiniToolStripRenderer renderer = miniToolStrip.Renderer as ToolStripTemplateNode.MiniToolStripRenderer; if (renderer != null) { renderer.State = (int)TemplateNodeSelectionState.None; miniToolStrip.Invalidate(); } } } internal void SelectControl() { ToolStrip miniToolStrip = this.Control as ToolStrip; if (miniToolStrip != null) { ToolStripTemplateNode.MiniToolStripRenderer renderer = miniToolStrip.Renderer as ToolStripTemplateNode.MiniToolStripRenderer; if (renderer != null) { renderer.State = (int)TemplateNodeSelectionState.TemplateNodeSelected; miniToolStrip.Invalidate(); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
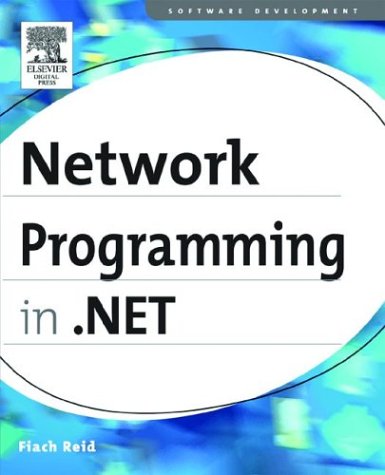
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlBinaryReader.cs
- ToolStripDesigner.cs
- HttpCachePolicyElement.cs
- SecurityContextTokenCache.cs
- JsonXmlDataContract.cs
- CallbackValidatorAttribute.cs
- HttpRawResponse.cs
- Environment.cs
- EventlogProvider.cs
- RegistryPermission.cs
- UrlPath.cs
- ChangePassword.cs
- SettingsPropertyValue.cs
- x509utils.cs
- SafeFileMapViewHandle.cs
- DeadCharTextComposition.cs
- SafeWaitHandle.cs
- CapabilitiesState.cs
- HttpModuleActionCollection.cs
- MutexSecurity.cs
- StylusPointPropertyId.cs
- TemplateNameScope.cs
- ExtendedPropertyDescriptor.cs
- WorkerRequest.cs
- DockAndAnchorLayout.cs
- RtfToken.cs
- LineInfo.cs
- PanelContainerDesigner.cs
- InkCanvasSelection.cs
- TableRowsCollectionEditor.cs
- EasingKeyFrames.cs
- RoutedEvent.cs
- EntityDataSourceWrapper.cs
- RegexCompilationInfo.cs
- CallbackHandler.cs
- ColorTransformHelper.cs
- ToolboxItem.cs
- PropertyPathConverter.cs
- TextTreeUndoUnit.cs
- SignedXml.cs
- RenderOptions.cs
- CatalogPart.cs
- BitmapEffectInputConnector.cs
- ExceptionUtility.cs
- DecoderReplacementFallback.cs
- TraceHandler.cs
- LinqDataSourceView.cs
- ArraySubsetEnumerator.cs
- VectorCollectionConverter.cs
- X509ChainPolicy.cs
- PassportAuthentication.cs
- OLEDB_Enum.cs
- XmlSchemaAnyAttribute.cs
- IndexedSelectQueryOperator.cs
- FileDataSourceCache.cs
- SpotLight.cs
- CustomBindingCollectionElement.cs
- DataSourceControl.cs
- RawStylusInputCustomDataList.cs
- XPathChildIterator.cs
- PseudoWebRequest.cs
- HttpApplicationStateBase.cs
- PersistenceProviderBehavior.cs
- TemplateBindingExtension.cs
- _UncName.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- GeneralTransform3D.cs
- XmlAttributeAttribute.cs
- StringAnimationBase.cs
- HostingMessageProperty.cs
- ExpressionParser.cs
- PropertyNames.cs
- CultureInfo.cs
- CompilationRelaxations.cs
- HtmlInputButton.cs
- PerfCounters.cs
- SmtpDigestAuthenticationModule.cs
- TreeViewAutomationPeer.cs
- LinearGradientBrush.cs
- Stylesheet.cs
- CoreSwitches.cs
- TreeBuilder.cs
- ComplexObject.cs
- MulticastDelegate.cs
- XmlSchemaAttributeGroupRef.cs
- MergeFailedEvent.cs
- Converter.cs
- CodeCompileUnit.cs
- AddInBase.cs
- XmlUtil.cs
- GenericWebPart.cs
- XmlILStorageConverter.cs
- LateBoundBitmapDecoder.cs
- BindingBase.cs
- InputMethod.cs
- SqlDataSourceEnumerator.cs
- Operator.cs
- CompareValidator.cs
- MetadataPropertyvalue.cs
- Interop.cs