Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / XmlUtil.cs / 1305376 / XmlUtil.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel { using System.Xml; using System.Diagnostics; static class XmlUtil { public const string XmlNs = "http://www.w3.org/XML/1998/namespace"; public const string XmlNsNs = "http://www.w3.org/2000/xmlns/"; //public static string GetXmlLangAttribute(XmlReader reader) //{ // string xmlLang = null; // if (reader.MoveToAttribute("lang", XmlNs)) // { // xmlLang = reader.Value; // reader.MoveToElement(); // } // if (xmlLang == null) // throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR.GetString(SR.XmlLangAttributeMissing))); // return xmlLang; //} //public static void ReadContentAsQName(XmlReader reader, out string localName, out string ns) //{ // ParseQName(reader, reader.ReadContentAsString(), out localName, out ns); //} public static bool IsWhitespace(char ch) { return (ch == ' ' || ch == '\t' || ch == '\r' || ch == '\n'); } public static string TrimEnd(string s) { int i; for (i = s.Length; i > 0 && IsWhitespace(s[i - 1]); i--) ; if (i != s.Length) { return s.Substring(0, i); } return s; } public static string TrimStart(string s) { int i; for (i = 0; i < s.Length && IsWhitespace(s[i]); i++) ; if (i != 0) { return s.Substring(i); } return s; } public static string Trim(string s) { int i; for (i = 0; i < s.Length && IsWhitespace(s[i]); i++); if (i >= s.Length) { return string.Empty; } int j; for (j = s.Length; j > 0 && IsWhitespace(s[j - 1]); j--); DiagnosticUtility.DebugAssert(j > i, "Logic error in XmlUtil.Trim()."); if (i != 0 || j != s.Length) { return s.Substring(i, j - i); } return s; } //public static void ParseQName(XmlReader reader, string qname, out string localName, out string ns) //{ // int index = qname.IndexOf(':'); // string prefix; // if (index < 0) // { // prefix = ""; // localName = TrimStart(TrimEnd(qname)); // } // else // { // if (index == qname.Length - 1) // throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR.GetString(SR.InvalidXmlQualifiedName, qname))); // prefix = TrimStart(qname.Substring(0, index)); // localName = TrimEnd(qname.Substring(index + 1)); // } // ns = reader.LookupNamespace(prefix); // if (ns == null) // throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR.GetString(SR.UnboundPrefixInQName, qname))); //} } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel { using System.Xml; using System.Diagnostics; static class XmlUtil { public const string XmlNs = "http://www.w3.org/XML/1998/namespace"; public const string XmlNsNs = "http://www.w3.org/2000/xmlns/"; //public static string GetXmlLangAttribute(XmlReader reader) //{ // string xmlLang = null; // if (reader.MoveToAttribute("lang", XmlNs)) // { // xmlLang = reader.Value; // reader.MoveToElement(); // } // if (xmlLang == null) // throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR.GetString(SR.XmlLangAttributeMissing))); // return xmlLang; //} //public static void ReadContentAsQName(XmlReader reader, out string localName, out string ns) //{ // ParseQName(reader, reader.ReadContentAsString(), out localName, out ns); //} public static bool IsWhitespace(char ch) { return (ch == ' ' || ch == '\t' || ch == '\r' || ch == '\n'); } public static string TrimEnd(string s) { int i; for (i = s.Length; i > 0 && IsWhitespace(s[i - 1]); i--) ; if (i != s.Length) { return s.Substring(0, i); } return s; } public static string TrimStart(string s) { int i; for (i = 0; i < s.Length && IsWhitespace(s[i]); i++) ; if (i != 0) { return s.Substring(i); } return s; } public static string Trim(string s) { int i; for (i = 0; i < s.Length && IsWhitespace(s[i]); i++); if (i >= s.Length) { return string.Empty; } int j; for (j = s.Length; j > 0 && IsWhitespace(s[j - 1]); j--); DiagnosticUtility.DebugAssert(j > i, "Logic error in XmlUtil.Trim()."); if (i != 0 || j != s.Length) { return s.Substring(i, j - i); } return s; } //public static void ParseQName(XmlReader reader, string qname, out string localName, out string ns) //{ // int index = qname.IndexOf(':'); // string prefix; // if (index < 0) // { // prefix = ""; // localName = TrimStart(TrimEnd(qname)); // } // else // { // if (index == qname.Length - 1) // throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR.GetString(SR.InvalidXmlQualifiedName, qname))); // prefix = TrimStart(qname.Substring(0, index)); // localName = TrimEnd(qname.Substring(index + 1)); // } // ns = reader.LookupNamespace(prefix); // if (ns == null) // throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR.GetString(SR.UnboundPrefixInQName, qname))); //} } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
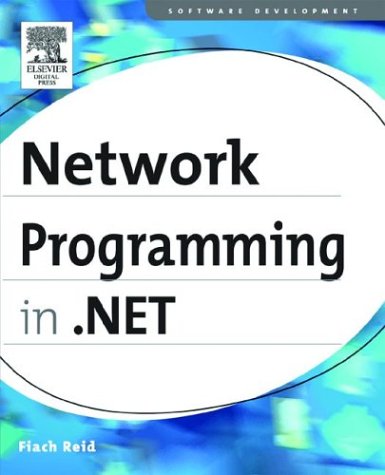
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WorkflowItemsPresenter.cs
- UniqueEventHelper.cs
- UserMapPath.cs
- _NetworkingPerfCounters.cs
- MissingMethodException.cs
- RuleAttributes.cs
- RuntimeEnvironment.cs
- uribuilder.cs
- TimerExtension.cs
- DataViewManager.cs
- LocationUpdates.cs
- BamlRecords.cs
- RadioButtonFlatAdapter.cs
- PassportAuthenticationEventArgs.cs
- ScrollChrome.cs
- ObjectSecurity.cs
- XmlHierarchicalEnumerable.cs
- DataGridCell.cs
- CookielessHelper.cs
- ProjectionPathBuilder.cs
- DoubleLinkListEnumerator.cs
- CompensateDesigner.cs
- TypedColumnHandler.cs
- FormViewDesigner.cs
- HighContrastHelper.cs
- UIAgentAsyncParams.cs
- SafeRightsManagementPubHandle.cs
- CompensatableTransactionScopeActivityDesigner.cs
- BodyGlyph.cs
- HandlerBase.cs
- DataGridBoolColumn.cs
- AccessText.cs
- GenericWebPart.cs
- CqlQuery.cs
- SqlConnectionString.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- ListViewCancelEventArgs.cs
- SelectedDatesCollection.cs
- ExpressionPrinter.cs
- RegexNode.cs
- EntitySet.cs
- XPathEmptyIterator.cs
- SkinBuilder.cs
- FullTextState.cs
- Themes.cs
- PlainXmlSerializer.cs
- CleanUpVirtualizedItemEventArgs.cs
- WindowsGrip.cs
- BatchStream.cs
- MdImport.cs
- RegistryPermission.cs
- SimpleExpression.cs
- TextElementEditingBehaviorAttribute.cs
- XmlSortKey.cs
- DtdParser.cs
- HttpContext.cs
- ToolStripItemEventArgs.cs
- ListViewItemEventArgs.cs
- CacheRequest.cs
- ScrollItemPattern.cs
- DataControlImageButton.cs
- TimeSpanStorage.cs
- ExitEventArgs.cs
- ComponentEvent.cs
- KeyMatchBuilder.cs
- MemoryFailPoint.cs
- Tag.cs
- RewritingValidator.cs
- CultureSpecificStringDictionary.cs
- OciEnlistContext.cs
- BezierSegment.cs
- TextProperties.cs
- _NtlmClient.cs
- TargetException.cs
- InfoCardMasterKey.cs
- GridProviderWrapper.cs
- Int32.cs
- XmlSchemaSimpleContentRestriction.cs
- NativeMethods.cs
- PerformanceCounterCategory.cs
- ZipIOCentralDirectoryBlock.cs
- BinaryUtilClasses.cs
- AsyncCompletedEventArgs.cs
- TextAnchor.cs
- AppDomainAttributes.cs
- DataService.cs
- Encoder.cs
- DragEvent.cs
- SystemGatewayIPAddressInformation.cs
- EnglishPluralizationService.cs
- WebPartZoneBase.cs
- LogicalTreeHelper.cs
- NodeFunctions.cs
- SinglePhaseEnlistment.cs
- XmlNamedNodeMap.cs
- PagePropertiesChangingEventArgs.cs
- PermissionListSet.cs
- CustomErrorCollection.cs
- XPathScanner.cs
- CapacityStreamGeometryContext.cs