Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / EntitySet.cs / 1305376 / EntitySet.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Threading; using System.Data.Common.Utils; using System.Collections.ObjectModel; namespace System.Data.Metadata.Edm { ////// concrete Class for representing a entity set /// public class EntitySet : EntitySetBase { #region Constructors ////// The constructor for constructing the EntitySet with a given name and an entity type /// /// The name of the EntitySet /// The db schema /// The db table /// The provider specific query that should be used to retrieve the EntitySet /// The entity type of the entities that this entity set type contains ///Thrown if the argument name or entityType is null internal EntitySet(string name, string schema, string table, string definingQuery, EntityType entityType) : base(name, schema, table, definingQuery, entityType) { } #endregion #region Fields private ReadOnlyCollection> _foreignKeyDependents; private ReadOnlyCollection > _foreignKeyPrincipals; private volatile bool _hasForeignKeyRelationships; private volatile bool _hasIndependentRelationships; #endregion #region Properties /// /// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.EntitySet; } } ////// Gets/Sets the entity type of this entity set /// public new EntityType ElementType { get { return (EntityType)base.ElementType; } } ////// Returns the associations and constraints where "this" EntitySet particpates as the Principal end. /// From the results of this list, you can retrieve the Dependent IRelatedEnds /// internal ReadOnlyCollection> ForeignKeyDependents { get { if (_foreignKeyDependents == null) { InitializeForeignKeyLists(); } return _foreignKeyDependents; } } /// /// Returns the associations and constraints where "this" EntitySet particpates as the Dependent end. /// From the results of this list, you can retrieve the Principal IRelatedEnds /// internal ReadOnlyCollection> ForeignKeyPrincipals { get { if (_foreignKeyPrincipals == null) { InitializeForeignKeyLists(); } return _foreignKeyPrincipals; } } /// /// True if this entity set participates in any foreign key relationships, otherwise false. /// internal bool HasForeignKeyRelationships { get { if (_foreignKeyPrincipals == null) { InitializeForeignKeyLists(); } return _hasForeignKeyRelationships; } } ////// True if this entity set participates in any independent relationships, otherwise false. /// internal bool HasIndependentRelationships { get { if (_foreignKeyPrincipals == null) { InitializeForeignKeyLists(); } return _hasIndependentRelationships; } } #endregion #region Methods private void InitializeForeignKeyLists() { var dependents = new List>(); var principals = new List >(); bool foundFkRelationship = false; bool foundIndependentRelationship = false; foreach (AssociationSet associationSet in MetadataHelper.GetAssociationsForEntitySet(this)) { if (associationSet.ElementType.IsForeignKey) { foundFkRelationship = true; Debug.Assert(associationSet.ElementType.ReferentialConstraints.Count == 1, "Expected exactly one constraint for FK"); ReferentialConstraint constraint = associationSet.ElementType.ReferentialConstraints[0]; if (constraint.ToRole.GetEntityType().IsAssignableFrom(this.ElementType) || this.ElementType.IsAssignableFrom(constraint.ToRole.GetEntityType())) { // Dependents dependents.Add(new Tuple (associationSet, constraint)); } if (constraint.FromRole.GetEntityType().IsAssignableFrom(this.ElementType) || this.ElementType.IsAssignableFrom(constraint.FromRole.GetEntityType())) { // Principals principals.Add(new Tuple (associationSet, constraint)); } } else { foundIndependentRelationship = true; } } _hasForeignKeyRelationships = foundFkRelationship; _hasIndependentRelationships = foundIndependentRelationship; var readOnlyDependents = dependents.AsReadOnly(); var readOnlyPrincipals = principals.AsReadOnly(); Interlocked.CompareExchange(ref _foreignKeyDependents, readOnlyDependents, null); Interlocked.CompareExchange(ref _foreignKeyPrincipals, readOnlyPrincipals, null); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Threading; using System.Data.Common.Utils; using System.Collections.ObjectModel; namespace System.Data.Metadata.Edm { ////// concrete Class for representing a entity set /// public class EntitySet : EntitySetBase { #region Constructors ////// The constructor for constructing the EntitySet with a given name and an entity type /// /// The name of the EntitySet /// The db schema /// The db table /// The provider specific query that should be used to retrieve the EntitySet /// The entity type of the entities that this entity set type contains ///Thrown if the argument name or entityType is null internal EntitySet(string name, string schema, string table, string definingQuery, EntityType entityType) : base(name, schema, table, definingQuery, entityType) { } #endregion #region Fields private ReadOnlyCollection> _foreignKeyDependents; private ReadOnlyCollection > _foreignKeyPrincipals; private volatile bool _hasForeignKeyRelationships; private volatile bool _hasIndependentRelationships; #endregion #region Properties /// /// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.EntitySet; } } ////// Gets/Sets the entity type of this entity set /// public new EntityType ElementType { get { return (EntityType)base.ElementType; } } ////// Returns the associations and constraints where "this" EntitySet particpates as the Principal end. /// From the results of this list, you can retrieve the Dependent IRelatedEnds /// internal ReadOnlyCollection> ForeignKeyDependents { get { if (_foreignKeyDependents == null) { InitializeForeignKeyLists(); } return _foreignKeyDependents; } } /// /// Returns the associations and constraints where "this" EntitySet particpates as the Dependent end. /// From the results of this list, you can retrieve the Principal IRelatedEnds /// internal ReadOnlyCollection> ForeignKeyPrincipals { get { if (_foreignKeyPrincipals == null) { InitializeForeignKeyLists(); } return _foreignKeyPrincipals; } } /// /// True if this entity set participates in any foreign key relationships, otherwise false. /// internal bool HasForeignKeyRelationships { get { if (_foreignKeyPrincipals == null) { InitializeForeignKeyLists(); } return _hasForeignKeyRelationships; } } ////// True if this entity set participates in any independent relationships, otherwise false. /// internal bool HasIndependentRelationships { get { if (_foreignKeyPrincipals == null) { InitializeForeignKeyLists(); } return _hasIndependentRelationships; } } #endregion #region Methods private void InitializeForeignKeyLists() { var dependents = new List>(); var principals = new List >(); bool foundFkRelationship = false; bool foundIndependentRelationship = false; foreach (AssociationSet associationSet in MetadataHelper.GetAssociationsForEntitySet(this)) { if (associationSet.ElementType.IsForeignKey) { foundFkRelationship = true; Debug.Assert(associationSet.ElementType.ReferentialConstraints.Count == 1, "Expected exactly one constraint for FK"); ReferentialConstraint constraint = associationSet.ElementType.ReferentialConstraints[0]; if (constraint.ToRole.GetEntityType().IsAssignableFrom(this.ElementType) || this.ElementType.IsAssignableFrom(constraint.ToRole.GetEntityType())) { // Dependents dependents.Add(new Tuple (associationSet, constraint)); } if (constraint.FromRole.GetEntityType().IsAssignableFrom(this.ElementType) || this.ElementType.IsAssignableFrom(constraint.FromRole.GetEntityType())) { // Principals principals.Add(new Tuple (associationSet, constraint)); } } else { foundIndependentRelationship = true; } } _hasForeignKeyRelationships = foundFkRelationship; _hasIndependentRelationships = foundIndependentRelationship; var readOnlyDependents = dependents.AsReadOnly(); var readOnlyPrincipals = principals.AsReadOnly(); Interlocked.CompareExchange(ref _foreignKeyDependents, readOnlyDependents, null); Interlocked.CompareExchange(ref _foreignKeyPrincipals, readOnlyPrincipals, null); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
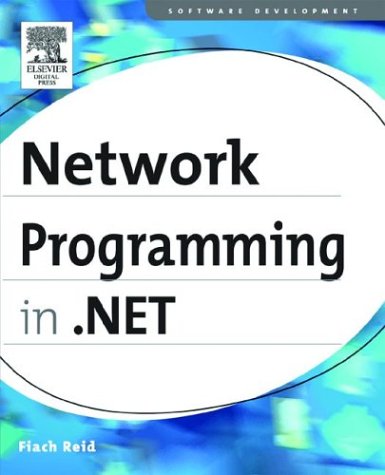
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SmtpNetworkElement.cs
- ZoneLinkButton.cs
- SystemColorTracker.cs
- TreeViewAutomationPeer.cs
- WebControlParameterProxy.cs
- ChangeBlockUndoRecord.cs
- SqlProcedureAttribute.cs
- XamlSerializer.cs
- PopupRootAutomationPeer.cs
- ScaleTransform3D.cs
- FloatUtil.cs
- GenericTypeParameterConverter.cs
- StructuralType.cs
- FloatUtil.cs
- VisualStyleRenderer.cs
- DynamicDiscoveryDocument.cs
- MsiStyleLogWriter.cs
- ImmComposition.cs
- DrawingAttributeSerializer.cs
- SiteIdentityPermission.cs
- SamlConstants.cs
- SecurityRuntime.cs
- ErasingStroke.cs
- PeerNameRegistration.cs
- PersianCalendar.cs
- CallbackHandler.cs
- EventLogEntry.cs
- LogArchiveSnapshot.cs
- SvcMapFile.cs
- OracleBFile.cs
- ThemeableAttribute.cs
- HttpListenerPrefixCollection.cs
- Polyline.cs
- AnimationClockResource.cs
- SelectionRangeConverter.cs
- CacheDependency.cs
- HMACMD5.cs
- MatrixTransform.cs
- RequestNavigateEventArgs.cs
- Empty.cs
- PrePrepareMethodAttribute.cs
- HtmlGenericControl.cs
- CreateUserWizard.cs
- MissingSatelliteAssemblyException.cs
- Frame.cs
- NamespaceQuery.cs
- PasswordBox.cs
- ResourceDictionaryCollection.cs
- DbConnectionInternal.cs
- RunInstallerAttribute.cs
- DesignTimeData.cs
- FormsAuthenticationModule.cs
- MenuItem.cs
- PointIndependentAnimationStorage.cs
- xmlformatgeneratorstatics.cs
- FixedSOMLineCollection.cs
- TextPatternIdentifiers.cs
- ObjectContext.cs
- Window.cs
- ButtonFieldBase.cs
- PermissionToken.cs
- NativeMethods.cs
- TextBoxView.cs
- AutoResizedEvent.cs
- ProtocolsSection.cs
- ManualResetEvent.cs
- FixedTextSelectionProcessor.cs
- XmlParserContext.cs
- ParallelLoopState.cs
- DataListItemCollection.cs
- PixelFormat.cs
- SchemaNamespaceManager.cs
- ButtonPopupAdapter.cs
- DataGridViewButtonColumn.cs
- ProvideValueServiceProvider.cs
- PeerToPeerException.cs
- CanExecuteRoutedEventArgs.cs
- SQLConvert.cs
- CodeEventReferenceExpression.cs
- BamlLocalizableResourceKey.cs
- SmtpMail.cs
- CompensatableTransactionScopeActivityDesigner.cs
- DbDataReader.cs
- TemplateBindingExpression.cs
- SizeIndependentAnimationStorage.cs
- SafeNativeMethods.cs
- AppSettingsExpressionBuilder.cs
- ContourSegment.cs
- SharedPerformanceCounter.cs
- CryptoKeySecurity.cs
- EllipticalNodeOperations.cs
- DataGridViewCellEventArgs.cs
- XpsFont.cs
- Hashtable.cs
- ParsedAttributeCollection.cs
- CacheManager.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- COM2PropertyPageUITypeConverter.cs
- SqlParameter.cs
- TemplateControl.cs