Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / XPath / Internal / NamespaceQuery.cs / 1305376 / NamespaceQuery.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Globalization; internal sealed class NamespaceQuery : BaseAxisQuery { private bool onNamespace; public NamespaceQuery(Query qyParent, string Name, string Prefix, XPathNodeType Type) : base(qyParent, Name, Prefix, Type) {} private NamespaceQuery(NamespaceQuery other) : base(other) { this.onNamespace = other.onNamespace; } public override void Reset() { onNamespace = false; base.Reset(); } public override XPathNavigator Advance() { while (true) { if (!onNamespace) { currentNode = qyInput.Advance(); if (currentNode == null) { return null; } position = 0; currentNode = currentNode.Clone(); onNamespace = currentNode.MoveToFirstNamespace(); } else { onNamespace = currentNode.MoveToNextNamespace(); } if (onNamespace) { if (matches(currentNode)) { position++; return currentNode; } } } // while } // Advance public override bool matches(XPathNavigator e) { Debug.Assert(e.NodeType == XPathNodeType.Namespace); if (e.Value.Length == 0) { Debug.Assert(e.LocalName.Length == 0, "Only xmlns='' can have empty string as a value"); // Namespace axes never returns xmlns='', // because it's not a NS declaration but rather undeclaration. return false; } if (NameTest) { return Name.Equals(e.LocalName); } else { return true; } } public override XPathNodeIterator Clone() { return new NamespaceQuery(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Globalization; internal sealed class NamespaceQuery : BaseAxisQuery { private bool onNamespace; public NamespaceQuery(Query qyParent, string Name, string Prefix, XPathNodeType Type) : base(qyParent, Name, Prefix, Type) {} private NamespaceQuery(NamespaceQuery other) : base(other) { this.onNamespace = other.onNamespace; } public override void Reset() { onNamespace = false; base.Reset(); } public override XPathNavigator Advance() { while (true) { if (!onNamespace) { currentNode = qyInput.Advance(); if (currentNode == null) { return null; } position = 0; currentNode = currentNode.Clone(); onNamespace = currentNode.MoveToFirstNamespace(); } else { onNamespace = currentNode.MoveToNextNamespace(); } if (onNamespace) { if (matches(currentNode)) { position++; return currentNode; } } } // while } // Advance public override bool matches(XPathNavigator e) { Debug.Assert(e.NodeType == XPathNodeType.Namespace); if (e.Value.Length == 0) { Debug.Assert(e.LocalName.Length == 0, "Only xmlns='' can have empty string as a value"); // Namespace axes never returns xmlns='', // because it's not a NS declaration but rather undeclaration. return false; } if (NameTest) { return Name.Equals(e.LocalName); } else { return true; } } public override XPathNodeIterator Clone() { return new NamespaceQuery(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
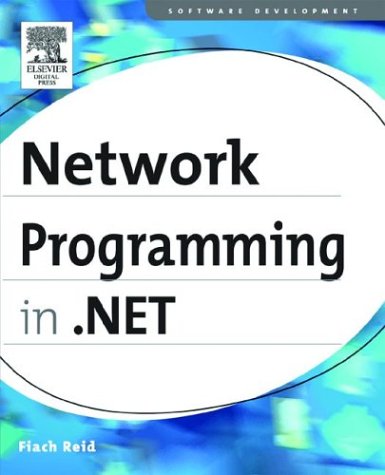
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetadataSource.cs
- NameValueFileSectionHandler.cs
- IncomingWebResponseContext.cs
- URLEditor.cs
- PrincipalPermission.cs
- Fx.cs
- WebPartsPersonalization.cs
- RtfToXamlReader.cs
- TextAutomationPeer.cs
- RuntimeVariablesExpression.cs
- GlobalizationSection.cs
- HotSpotCollection.cs
- XmlJsonReader.cs
- DataRecordInternal.cs
- AnnotationHelper.cs
- CompiledQuery.cs
- itemelement.cs
- InheritablePropertyChangeInfo.cs
- WebControl.cs
- WorkflowOperationBehavior.cs
- ListViewCancelEventArgs.cs
- InstanceDataCollectionCollection.cs
- TreeNodeBindingCollection.cs
- PolyBezierSegmentFigureLogic.cs
- ParserExtension.cs
- ItemCollection.cs
- PolicyManager.cs
- XmlNamespaceDeclarationsAttribute.cs
- FontInfo.cs
- SoapFormatExtensions.cs
- ViewGenerator.cs
- _NativeSSPI.cs
- XmlDeclaration.cs
- QueryOperationResponseOfT.cs
- ProvidersHelper.cs
- OutgoingWebResponseContext.cs
- MimeMapping.cs
- StateMachine.cs
- GetMemberBinder.cs
- OdbcDataReader.cs
- CorrelationManager.cs
- XamlBrushSerializer.cs
- PropertyCondition.cs
- RIPEMD160Managed.cs
- ObjectAnimationBase.cs
- NominalTypeEliminator.cs
- TypeToken.cs
- WindowsTab.cs
- SqlDependencyListener.cs
- HtmlInputText.cs
- OdbcConnection.cs
- ImportedNamespaceContextItem.cs
- ToolStripSplitStackLayout.cs
- FileDialog.cs
- RowType.cs
- TextServicesCompartmentEventSink.cs
- ComboBox.cs
- thaishape.cs
- EntityDataSourceDataSelection.cs
- Frame.cs
- ErasingStroke.cs
- ConsumerConnectionPointCollection.cs
- TileModeValidation.cs
- SolidBrush.cs
- TraceSection.cs
- ToolStripContentPanel.cs
- DataTemplate.cs
- ByteAnimation.cs
- StateMachine.cs
- contentDescriptor.cs
- SmiRecordBuffer.cs
- SqlCaseSimplifier.cs
- BStrWrapper.cs
- DataGridClipboardHelper.cs
- WebSysDisplayNameAttribute.cs
- MonitoringDescriptionAttribute.cs
- TypeExtensions.cs
- Int32CAMarshaler.cs
- ExcCanonicalXml.cs
- LambdaCompiler.Lambda.cs
- ProxyHelper.cs
- XslCompiledTransform.cs
- SHA512.cs
- FormClosingEvent.cs
- OleDbException.cs
- TypeFieldSchema.cs
- EventLogPermissionAttribute.cs
- CmsInterop.cs
- MemberMaps.cs
- SecurityElement.cs
- PaintValueEventArgs.cs
- ToolStripRendererSwitcher.cs
- SpanIndex.cs
- _SslState.cs
- NotificationContext.cs
- EnumBuilder.cs
- ConnectionConsumerAttribute.cs
- NoPersistHandle.cs
- ColumnClickEvent.cs
- ToolStripSeparator.cs