Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / xsp / System / Web / Extensions / Compilation / WCFModel / MetadataSource.cs / 1 / MetadataSource.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- // // This code is shared between ndp\fx\src\xsp\System\Web\Extensions\Compilation\WCFModel // and wizard\vsdesigner\designer\microsoft\vsdesigner\WCFModel. // Please make sure the code files between those two directories are alway in [....] when you make any changes to this code. // And always test these code in both places before check in. // The code under ndp\fx\src\xsp\System\Web\Extensions\Compilation\XmlSerializer might have to be regerenated when // the format of the svcmap file is changed, or class structure has been changed in this directory. Please follow the HowTo file // under Compilation directory to see how to regerenate that code. // using System; using System.Diagnostics; using System.Globalization; #if WEB_EXTENSIONS_CODE using System.Web.Resources; #else using Microsoft.VSDesigner.Resources.Microsoft.VSDesigner; #endif using XmlSerialization = System.Xml.Serialization; #if WEB_EXTENSIONS_CODE namespace System.Web.Compilation.WCFModel #else namespace Microsoft.VSDesigner.WCFModel #endif { ////// This class implements a MetadataSource item in the svcmap file /// ///#if WEB_EXTENSIONS_CODE internal class MetadataSource #else [CLSCompliant(true)] public class MetadataSource #endif { // URL of metadata source private string m_Address; // protocol to download it private string m_Protocol; // ID of this source private int m_SourceId; /// /// Constructor /// ///Must support a default construct for XmlSerializer public MetadataSource() { m_Address = String.Empty; m_Protocol = String.Empty; } ////// Constructor /// /// /// /// public MetadataSource(string protocol, string address, int sourceId) { if (protocol == null) { throw new ArgumentNullException("protocol"); } if (address == null) { throw new ArgumentNullException("address"); } if (protocol.Length == 0) { throw new ArgumentException(WCFModelStrings.ReferenceGroup_EmptyProtocol); } if (address == null) { throw new ArgumentException(WCFModelStrings.ReferenceGroup_EmptyAddress); } m_Protocol = protocol; m_Address = address; if (sourceId < 0) { Debug.Fail("Source ID shouldn't be a nagtive number"); throw new ArgumentException(WCFModelStrings.ReferenceGroup_InvalidSourceId); } m_SourceId = sourceId; } ////// URL address to download metadata /// ////// [XmlSerialization.XmlAttribute()] public string Address { get { return m_Address; } set { if (value == null) { throw new ArgumentNullException("value"); } m_Address = value; } } /// /// protocol used to download metadata /// ////// [XmlSerialization.XmlAttribute()] public string Protocol { get { return m_Protocol; } set { if (value == null) { throw new ArgumentNullException("value"); } m_Protocol = value; } } /// /// generated ID for this metadata source /// ////// [XmlSerialization.XmlAttribute()] public int SourceId { get { return m_SourceId; } set { if (value < 0) { Debug.Fail("Source ID shouldn't be a nagtive number"); throw new ArgumentException(WCFModelStrings.ReferenceGroup_InvalidSourceId); } m_SourceId = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- // // This code is shared between ndp\fx\src\xsp\System\Web\Extensions\Compilation\WCFModel // and wizard\vsdesigner\designer\microsoft\vsdesigner\WCFModel. // Please make sure the code files between those two directories are alway in [....] when you make any changes to this code. // And always test these code in both places before check in. // The code under ndp\fx\src\xsp\System\Web\Extensions\Compilation\XmlSerializer might have to be regerenated when // the format of the svcmap file is changed, or class structure has been changed in this directory. Please follow the HowTo file // under Compilation directory to see how to regerenate that code. // using System; using System.Diagnostics; using System.Globalization; #if WEB_EXTENSIONS_CODE using System.Web.Resources; #else using Microsoft.VSDesigner.Resources.Microsoft.VSDesigner; #endif using XmlSerialization = System.Xml.Serialization; #if WEB_EXTENSIONS_CODE namespace System.Web.Compilation.WCFModel #else namespace Microsoft.VSDesigner.WCFModel #endif { ////// This class implements a MetadataSource item in the svcmap file /// ///#if WEB_EXTENSIONS_CODE internal class MetadataSource #else [CLSCompliant(true)] public class MetadataSource #endif { // URL of metadata source private string m_Address; // protocol to download it private string m_Protocol; // ID of this source private int m_SourceId; /// /// Constructor /// ///Must support a default construct for XmlSerializer public MetadataSource() { m_Address = String.Empty; m_Protocol = String.Empty; } ////// Constructor /// /// /// /// public MetadataSource(string protocol, string address, int sourceId) { if (protocol == null) { throw new ArgumentNullException("protocol"); } if (address == null) { throw new ArgumentNullException("address"); } if (protocol.Length == 0) { throw new ArgumentException(WCFModelStrings.ReferenceGroup_EmptyProtocol); } if (address == null) { throw new ArgumentException(WCFModelStrings.ReferenceGroup_EmptyAddress); } m_Protocol = protocol; m_Address = address; if (sourceId < 0) { Debug.Fail("Source ID shouldn't be a nagtive number"); throw new ArgumentException(WCFModelStrings.ReferenceGroup_InvalidSourceId); } m_SourceId = sourceId; } ////// URL address to download metadata /// ////// [XmlSerialization.XmlAttribute()] public string Address { get { return m_Address; } set { if (value == null) { throw new ArgumentNullException("value"); } m_Address = value; } } /// /// protocol used to download metadata /// ////// [XmlSerialization.XmlAttribute()] public string Protocol { get { return m_Protocol; } set { if (value == null) { throw new ArgumentNullException("value"); } m_Protocol = value; } } /// /// generated ID for this metadata source /// ////// [XmlSerialization.XmlAttribute()] public int SourceId { get { return m_SourceId; } set { if (value < 0) { Debug.Fail("Source ID shouldn't be a nagtive number"); throw new ArgumentException(WCFModelStrings.ReferenceGroup_InvalidSourceId); } m_SourceId = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
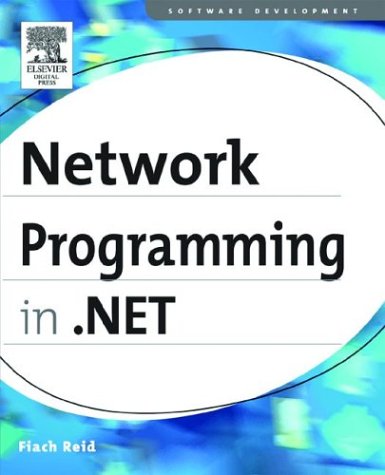
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WinInetCache.cs
- SystemGatewayIPAddressInformation.cs
- WSSecurityOneDotOneSendSecurityHeader.cs
- TextDecorationUnitValidation.cs
- CharacterMetricsDictionary.cs
- AdapterDictionary.cs
- ListItemCollection.cs
- DisposableCollectionWrapper.cs
- QuotaExceededException.cs
- XmlCompatibilityReader.cs
- HtmlInputImage.cs
- ConvertEvent.cs
- WSFederationHttpSecurityElement.cs
- RegexWriter.cs
- OSFeature.cs
- TextWriterTraceListener.cs
- TypeReference.cs
- AlternationConverter.cs
- XhtmlBasicLabelAdapter.cs
- MethodAccessException.cs
- ContainerAction.cs
- StrokeIntersection.cs
- TransformCryptoHandle.cs
- UnsafeNativeMethods.cs
- UserControlParser.cs
- CultureInfo.cs
- NetDispatcherFaultException.cs
- HuffModule.cs
- RegistrationContext.cs
- SettingsProviderCollection.cs
- WebPartEditVerb.cs
- CatalogZone.cs
- ComplexTypeEmitter.cs
- SpotLight.cs
- NamedPermissionSet.cs
- ColumnWidthChangedEvent.cs
- Preprocessor.cs
- MaterialCollection.cs
- WmlObjectListAdapter.cs
- MarkedHighlightComponent.cs
- DocumentEventArgs.cs
- CheckPair.cs
- XmlSignatureProperties.cs
- FixedNode.cs
- ObjectStateManagerMetadata.cs
- DataGridPageChangedEventArgs.cs
- CaseInsensitiveHashCodeProvider.cs
- ObjectStateManager.cs
- XmlEncodedRawTextWriter.cs
- EDesignUtil.cs
- RelationshipConstraintValidator.cs
- AttachmentCollection.cs
- Collection.cs
- SelectionRangeConverter.cs
- XPathScanner.cs
- SiteOfOriginPart.cs
- PropertyGroupDescription.cs
- JsonWriterDelegator.cs
- ObjectDataSource.cs
- HttpClientProtocol.cs
- CheckableControlBaseAdapter.cs
- ConfigXmlDocument.cs
- QueuePropertyVariants.cs
- PowerStatus.cs
- EntityConnection.cs
- HttpModuleActionCollection.cs
- DocumentAutomationPeer.cs
- Effect.cs
- SettingsBase.cs
- PropertyReference.cs
- CommonXSendMessage.cs
- TypeValidationEventArgs.cs
- TypeLibConverter.cs
- BamlMapTable.cs
- SynchronizingStream.cs
- ReflectionUtil.cs
- DecoderFallbackWithFailureFlag.cs
- NameValueFileSectionHandler.cs
- Inline.cs
- WebPartTransformer.cs
- HighlightComponent.cs
- ChangeInterceptorAttribute.cs
- InertiaRotationBehavior.cs
- XhtmlBasicPageAdapter.cs
- BufferBuilder.cs
- SessionEndingEventArgs.cs
- EntityDataSourceConfigureObjectContext.cs
- WebPartConnection.cs
- SrgsGrammarCompiler.cs
- SiteMapNodeItem.cs
- SelectionChangedEventArgs.cs
- DbExpressionVisitor.cs
- Permission.cs
- ISAPIWorkerRequest.cs
- MemberMaps.cs
- ImageBrush.cs
- GenericTypeParameterBuilder.cs
- HtmlImage.cs
- CatalogPartDesigner.cs
- Graphics.cs