Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / MS / Internal / Ink / InkSerializedFormat / HuffModule.cs / 1 / HuffModule.cs
using MS.Utility; using System; using System.Runtime.InteropServices; using System.Security; using System.Globalization; using System.Windows; using System.Windows.Input; using System.Windows.Ink; using MS.Internal.Ink.InkSerializedFormat; using System.Collections.Generic; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink.InkSerializedFormat { ////// HuffModule /// internal class HuffModule { ////// Ctor /// internal HuffModule() { } ////// GetDefCodec /// internal HuffCodec GetDefCodec(uint index) { HuffCodec huffCodec = null; if (AlgoModule.DefaultBAACount > index) { huffCodec = _defaultHuffCodecs[index]; if (huffCodec == null) { huffCodec = new HuffCodec(index); _defaultHuffCodecs[index] = huffCodec; } } else { throw new ArgumentOutOfRangeException("index"); } return huffCodec; } ////// FindCodec /// /// internal HuffCodec FindCodec(byte algoData) { byte codec = (byte)(algoData & 0x1f); //unused //if ((0x20 & algoData) != 0) //{ // int iLookup = (algoData & 0x1f); // if ((iLookup > 0) && (iLookup <= _lookupList.Count)) // { // codec = _lookupList[iLookup - 1].Byte; // } //} if (codec < AlgoModule.DefaultBAACount) { return GetDefCodec((uint)codec); } if ((int)codec >= _huffCodecs.Count + AlgoModule.DefaultBAACount) { throw new ArgumentException(StrokeCollectionSerializer.ISFDebugMessage("invalid codec computed")); } return _huffCodecs[(int)(codec - AlgoModule.DefaultBAACount)]; } ////// FindDtXf /// /// internal DataXform FindDtXf(byte algoData) { //unused //if ((0x20 & algoData) != 0) //{ // int lookupIndex = (int)(algoData & 0x1f); // if ((lookupIndex > 0) && (lookupIndex < _lookupList.Count)) // { // return _lookupList[lookupIndex].DeltaDelta; // } //} return this.DefaultDeltaDelta; } ////// Private lazy init'd /// private DeltaDelta DefaultDeltaDelta { get { if (_defaultDtxf == null) { _defaultDtxf = new DeltaDelta(); } return _defaultDtxf; } } ////// Privates /// private DeltaDelta _defaultDtxf; //unused //private List_lookupList = new List (); private List _huffCodecs = new List (); private HuffCodec[] _defaultHuffCodecs = new HuffCodec[AlgoModule.DefaultBAACount]; //unused ///// ///// Simple helper class ///// //private class CodeLookup //{ // internal CodeLookup(DeltaDelta dd, byte b) // { // if (dd == null) { throw new ArgumentNullException(); } // DeltaDelta = dd; // Byte = b; // } // internal DeltaDelta DeltaDelta; // internal Byte Byte; //} } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using MS.Utility; using System; using System.Runtime.InteropServices; using System.Security; using System.Globalization; using System.Windows; using System.Windows.Input; using System.Windows.Ink; using MS.Internal.Ink.InkSerializedFormat; using System.Collections.Generic; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink.InkSerializedFormat { ////// HuffModule /// internal class HuffModule { ////// Ctor /// internal HuffModule() { } ////// GetDefCodec /// internal HuffCodec GetDefCodec(uint index) { HuffCodec huffCodec = null; if (AlgoModule.DefaultBAACount > index) { huffCodec = _defaultHuffCodecs[index]; if (huffCodec == null) { huffCodec = new HuffCodec(index); _defaultHuffCodecs[index] = huffCodec; } } else { throw new ArgumentOutOfRangeException("index"); } return huffCodec; } ////// FindCodec /// /// internal HuffCodec FindCodec(byte algoData) { byte codec = (byte)(algoData & 0x1f); //unused //if ((0x20 & algoData) != 0) //{ // int iLookup = (algoData & 0x1f); // if ((iLookup > 0) && (iLookup <= _lookupList.Count)) // { // codec = _lookupList[iLookup - 1].Byte; // } //} if (codec < AlgoModule.DefaultBAACount) { return GetDefCodec((uint)codec); } if ((int)codec >= _huffCodecs.Count + AlgoModule.DefaultBAACount) { throw new ArgumentException(StrokeCollectionSerializer.ISFDebugMessage("invalid codec computed")); } return _huffCodecs[(int)(codec - AlgoModule.DefaultBAACount)]; } ////// FindDtXf /// /// internal DataXform FindDtXf(byte algoData) { //unused //if ((0x20 & algoData) != 0) //{ // int lookupIndex = (int)(algoData & 0x1f); // if ((lookupIndex > 0) && (lookupIndex < _lookupList.Count)) // { // return _lookupList[lookupIndex].DeltaDelta; // } //} return this.DefaultDeltaDelta; } ////// Private lazy init'd /// private DeltaDelta DefaultDeltaDelta { get { if (_defaultDtxf == null) { _defaultDtxf = new DeltaDelta(); } return _defaultDtxf; } } ////// Privates /// private DeltaDelta _defaultDtxf; //unused //private List_lookupList = new List (); private List _huffCodecs = new List (); private HuffCodec[] _defaultHuffCodecs = new HuffCodec[AlgoModule.DefaultBAACount]; //unused ///// ///// Simple helper class ///// //private class CodeLookup //{ // internal CodeLookup(DeltaDelta dd, byte b) // { // if (dd == null) { throw new ArgumentNullException(); } // DeltaDelta = dd; // Byte = b; // } // internal DeltaDelta DeltaDelta; // internal Byte Byte; //} } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
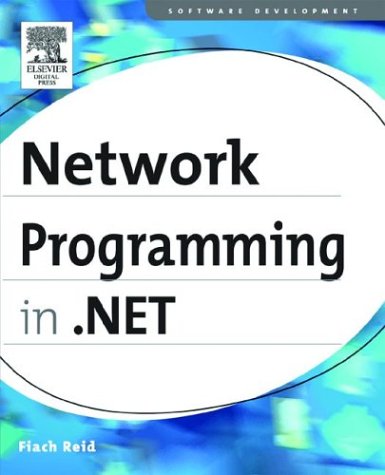
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResourceManager.cs
- OdbcStatementHandle.cs
- StreamReader.cs
- BStrWrapper.cs
- ArcSegment.cs
- SiteMembershipCondition.cs
- ProcessModule.cs
- Pool.cs
- sqlser.cs
- ProfileParameter.cs
- DesignerTransactionCloseEvent.cs
- MutexSecurity.cs
- ProxyElement.cs
- WindowsClientElement.cs
- SQLDateTime.cs
- TreeNodeCollectionEditor.cs
- SafeFindHandle.cs
- CommandHelpers.cs
- FormParameter.cs
- ImageSource.cs
- DBConnection.cs
- DynamicRouteExpression.cs
- DBParameter.cs
- GeometryDrawing.cs
- WindowsRebar.cs
- HttpPostClientProtocol.cs
- DesignerEditorPartChrome.cs
- FrameDimension.cs
- TextDecoration.cs
- RuleEngine.cs
- NewArrayExpression.cs
- CompositionCommandSet.cs
- TextElement.cs
- ExtractedStateEntry.cs
- SiteMapDataSource.cs
- PublishLicense.cs
- ImportCatalogPart.cs
- FragmentQueryProcessor.cs
- UserControl.cs
- NativeMethods.cs
- OleCmdHelper.cs
- FormattedText.cs
- ConfigXmlText.cs
- SHA1CryptoServiceProvider.cs
- StreamUpdate.cs
- HostExecutionContextManager.cs
- CryptoConfig.cs
- SchemaImporterExtensionsSection.cs
- filewebrequest.cs
- ListBox.cs
- CreateSequenceResponse.cs
- SplayTreeNode.cs
- XmlReaderSettings.cs
- ColumnMapProcessor.cs
- ToolStripPanelDesigner.cs
- codemethodreferenceexpression.cs
- UndoManager.cs
- Int32Storage.cs
- ReaderWriterLockWrapper.cs
- Environment.cs
- Perspective.cs
- FocusChangedEventArgs.cs
- VerticalAlignConverter.cs
- TranslateTransform3D.cs
- StateMachine.cs
- TextBoxBaseDesigner.cs
- XamlClipboardData.cs
- ChangeProcessor.cs
- BitmapData.cs
- SettingsContext.cs
- SmtpNtlmAuthenticationModule.cs
- AddInActivator.cs
- RuleSet.cs
- QueryCacheManager.cs
- FileSystemInfo.cs
- HttpGetServerProtocol.cs
- ProgressBarAutomationPeer.cs
- WebPartConnectionCollection.cs
- MouseWheelEventArgs.cs
- IISUnsafeMethods.cs
- FamilyMapCollection.cs
- List.cs
- TrackBar.cs
- Literal.cs
- WindowHelperService.cs
- MergeFailedEvent.cs
- TypeDelegator.cs
- CallContext.cs
- SystemIPInterfaceStatistics.cs
- GridViewColumnCollectionChangedEventArgs.cs
- XmlEnumAttribute.cs
- DetailsViewDesigner.cs
- WebPartConnectionsDisconnectVerb.cs
- MethodRental.cs
- TextBoxView.cs
- ToolBar.cs
- XmlEntity.cs
- SingleConverter.cs
- SchemaConstraints.cs
- DesignerProperties.cs