Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Common / Utils / Boolean / Sentence.cs / 1 / Sentence.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Globalization; using System.Collections.ObjectModel; using System.Diagnostics; using System.Linq; namespace System.Data.Common.Utils.Boolean { ////// Abstract base class for nodes in normal form expressions, e.g. Conjunctive Normal Form /// sentences. /// ///Type of expression leaf term identifiers. internal abstract class NormalFormNode{ private readonly BoolExpr _expr; /// /// Initialize a new normal form node representing the given expression. Caller must /// ensure the expression is logically equivalent to the node. /// /// Expression logically equivalent to this node. protected NormalFormNode(BoolExprexpr) { _expr = expr.Simplify(); } /// /// Gets an expression that is logically equivalent to this node. /// internal BoolExprExpr { get { return _expr; } } /// /// Utility method for delegation that return the expression corresponding to a given /// normal form node. /// ///Type of node /// Node to examine. ///Equivalent Boolean expression for the given node. protected static BoolExprExprSelector (T_NormalFormNode node) where T_NormalFormNode : NormalFormNode { return node._expr; } } /// /// Abstract base class for normal form sentences (CNF and DNF) /// ///Type of expression leaf term identifiers. ///Type of clauses in the sentence. internal abstract class Sentence: NormalFormNode where T_Clause : Clause , IEquatable { private readonly Set _clauses; /// /// Initialize a sentence given the appropriate sentence clauses. Produces /// an equivalent expression by composing the clause expressions using /// the given tree type. /// /// Sentence clauses /// Tree type for sentence (and generated expression) protected Sentence(Setclauses, ExprType treeType) : base(ConvertClausesToExpr(clauses, treeType)) { _clauses = clauses.AsReadOnly(); } // Produces an expression equivalent to the given clauses by composing the clause // expressions using the given tree type. private static BoolExpr ConvertClausesToExpr(Set clauses, ExprType treeType) { bool isAnd = ExprType.And == treeType; Debug.Assert(isAnd || ExprType.Or == treeType); IEnumerable > clauseExpressions = clauses.Select(new Func >(ExprSelector)); if (isAnd) { return new AndExpr (clauseExpressions); } else { return new OrExpr (clauseExpressions); } } public override string ToString() { StringBuilder builder = new StringBuilder(); builder.Append("Sentence{"); builder.Append(_clauses); return builder.Append("}").ToString(); } } /// /// Represents a sentence in disjunctive normal form, e.g.: /// /// Clause1 + Clause2 . ... /// /// Where each DNF clause is of the form: /// /// Literal1 . Literal2 . ... /// /// Each literal is of the form: /// /// Term /// /// or /// /// !Term /// ///Type of expression leaf term identifiers. internal sealed class DnfSentence: Sentence > { // Initializes a new DNF sentence given its clauses. internal DnfSentence(Set > clauses) : base(clauses, ExprType.Or) { } } /// /// Represents a sentence in conjunctive normal form, e.g.: /// /// Clause1 . Clause2 . ... /// /// Where each DNF clause is of the form: /// /// Literal1 + Literal2 + ... /// /// Each literal is of the form: /// /// Term /// /// or /// /// !Term /// ///Type of expression leaf term identifiers. internal sealed class CnfSentence: Sentence > { // Initializes a new CNF sentence given its clauses. internal CnfSentence(Set > clauses) : base(clauses, ExprType.And) { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Globalization; using System.Collections.ObjectModel; using System.Diagnostics; using System.Linq; namespace System.Data.Common.Utils.Boolean { ////// Abstract base class for nodes in normal form expressions, e.g. Conjunctive Normal Form /// sentences. /// ///Type of expression leaf term identifiers. internal abstract class NormalFormNode{ private readonly BoolExpr _expr; /// /// Initialize a new normal form node representing the given expression. Caller must /// ensure the expression is logically equivalent to the node. /// /// Expression logically equivalent to this node. protected NormalFormNode(BoolExprexpr) { _expr = expr.Simplify(); } /// /// Gets an expression that is logically equivalent to this node. /// internal BoolExprExpr { get { return _expr; } } /// /// Utility method for delegation that return the expression corresponding to a given /// normal form node. /// ///Type of node /// Node to examine. ///Equivalent Boolean expression for the given node. protected static BoolExprExprSelector (T_NormalFormNode node) where T_NormalFormNode : NormalFormNode { return node._expr; } } /// /// Abstract base class for normal form sentences (CNF and DNF) /// ///Type of expression leaf term identifiers. ///Type of clauses in the sentence. internal abstract class Sentence: NormalFormNode where T_Clause : Clause , IEquatable { private readonly Set _clauses; /// /// Initialize a sentence given the appropriate sentence clauses. Produces /// an equivalent expression by composing the clause expressions using /// the given tree type. /// /// Sentence clauses /// Tree type for sentence (and generated expression) protected Sentence(Setclauses, ExprType treeType) : base(ConvertClausesToExpr(clauses, treeType)) { _clauses = clauses.AsReadOnly(); } // Produces an expression equivalent to the given clauses by composing the clause // expressions using the given tree type. private static BoolExpr ConvertClausesToExpr(Set clauses, ExprType treeType) { bool isAnd = ExprType.And == treeType; Debug.Assert(isAnd || ExprType.Or == treeType); IEnumerable > clauseExpressions = clauses.Select(new Func >(ExprSelector)); if (isAnd) { return new AndExpr (clauseExpressions); } else { return new OrExpr (clauseExpressions); } } public override string ToString() { StringBuilder builder = new StringBuilder(); builder.Append("Sentence{"); builder.Append(_clauses); return builder.Append("}").ToString(); } } /// /// Represents a sentence in disjunctive normal form, e.g.: /// /// Clause1 + Clause2 . ... /// /// Where each DNF clause is of the form: /// /// Literal1 . Literal2 . ... /// /// Each literal is of the form: /// /// Term /// /// or /// /// !Term /// ///Type of expression leaf term identifiers. internal sealed class DnfSentence: Sentence > { // Initializes a new DNF sentence given its clauses. internal DnfSentence(Set > clauses) : base(clauses, ExprType.Or) { } } /// /// Represents a sentence in conjunctive normal form, e.g.: /// /// Clause1 . Clause2 . ... /// /// Where each DNF clause is of the form: /// /// Literal1 + Literal2 + ... /// /// Each literal is of the form: /// /// Term /// /// or /// /// !Term /// ///Type of expression leaf term identifiers. internal sealed class CnfSentence: Sentence > { // Initializes a new CNF sentence given its clauses. internal CnfSentence(Set > clauses) : base(clauses, ExprType.And) { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
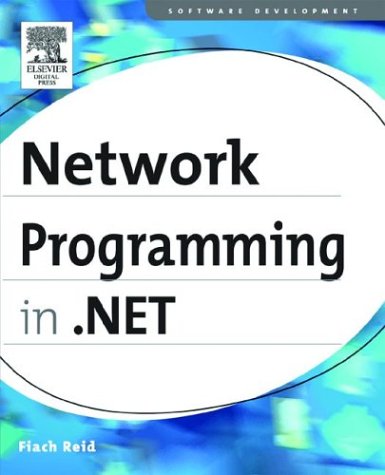
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CharStorage.cs
- Filter.cs
- ToolStripItemImageRenderEventArgs.cs
- AutomationPropertyInfo.cs
- EventDescriptor.cs
- ClientScriptManagerWrapper.cs
- ListBoxAutomationPeer.cs
- MsmqOutputMessage.cs
- ServiceChannelManager.cs
- RelationshipEnd.cs
- Compilation.cs
- LayoutDump.cs
- ImageListStreamer.cs
- Internal.cs
- GlyphRun.cs
- AssemblyCollection.cs
- ContentTextAutomationPeer.cs
- EntityDataSourceSelectingEventArgs.cs
- StatusBarItemAutomationPeer.cs
- LayoutTableCell.cs
- XmlUtf8RawTextWriter.cs
- ToolStripTextBox.cs
- SqlParameterizer.cs
- Rotation3D.cs
- ReceiveActivityDesigner.cs
- FormClosingEvent.cs
- X509IssuerSerialKeyIdentifierClause.cs
- StylusPointCollection.cs
- EntityViewGenerationAttribute.cs
- XmlElement.cs
- DataListItemEventArgs.cs
- AccessedThroughPropertyAttribute.cs
- CodeBlockBuilder.cs
- StrongNamePublicKeyBlob.cs
- CalendarAutomationPeer.cs
- ConstraintConverter.cs
- Italic.cs
- BaseCollection.cs
- PersistenceMetadataNamespace.cs
- GroupStyle.cs
- SessionChannels.cs
- RegexReplacement.cs
- InputLanguageProfileNotifySink.cs
- TextEditorTyping.cs
- WebPartConnectionsEventArgs.cs
- ReliableMessagingHelpers.cs
- StringFunctions.cs
- XmlCollation.cs
- XmlDataImplementation.cs
- DataServiceRequestOfT.cs
- BitFlagsGenerator.cs
- EntityException.cs
- CustomSignedXml.cs
- HttpWebResponse.cs
- LayoutEditorPart.cs
- XmlElementAttributes.cs
- AttachedPropertyInfo.cs
- path.cs
- TouchEventArgs.cs
- StringComparer.cs
- ProjectionPathBuilder.cs
- EntityDataSourceDataSelection.cs
- BoolExpressionVisitors.cs
- GuidelineSet.cs
- FileRecordSequenceCompletedAsyncResult.cs
- XmlNodeComparer.cs
- TextSpanModifier.cs
- LayoutDump.cs
- HtmlGenericControl.cs
- TypeConverterValueSerializer.cs
- PieceNameHelper.cs
- TraceSource.cs
- GeometryHitTestParameters.cs
- WebConfigurationManager.cs
- X509Certificate.cs
- NamedPipeHostedTransportConfiguration.cs
- HttpCacheVary.cs
- UriScheme.cs
- IgnorePropertiesAttribute.cs
- SelectedCellsCollection.cs
- SqlProfileProvider.cs
- VirtualDirectoryMappingCollection.cs
- DataGridState.cs
- DataSet.cs
- PrimitiveSchema.cs
- DataGridViewComboBoxColumn.cs
- BypassElement.cs
- SelectorItemAutomationPeer.cs
- QilTargetType.cs
- ObjectQueryState.cs
- xmlglyphRunInfo.cs
- ApplicationSettingsBase.cs
- ObjectSecurityT.cs
- XComponentModel.cs
- FrameDimension.cs
- UnsafeNativeMethods.cs
- TrackingSection.cs
- PointAnimationClockResource.cs
- MetabaseServerConfig.cs
- HandoffBehavior.cs