Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / RelationshipEnd.cs / 1305376 / RelationshipEnd.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Xml; using System.Data; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// Represents an End element in a relationship /// internal sealed class RelationshipEnd : SchemaElement, IRelationshipEnd { private string _unresolvedType; private RelationshipMultiplicity? _multiplicity; private SchemaEntityType _type; private List_operations; /// /// construct a Relationship End /// /// public RelationshipEnd(Relationship relationship) : base(relationship) { } ////// Type of the End /// public SchemaEntityType Type { get { return _type; } private set { _type = value; } } ////// Multiplicity of the End /// public RelationshipMultiplicity? Multiplicity { get { return _multiplicity; } set { _multiplicity = value; } } ////// The On<Operation>s defined for the End /// public ICollectionOperations { get { if (_operations == null) _operations = new List (); return _operations; } } /// /// do whole element resolution /// internal override void ResolveTopLevelNames() { base.ResolveTopLevelNames(); if (Type == null && _unresolvedType != null) { SchemaType element; if (!Schema.ResolveTypeName(this, _unresolvedType, out element)) { return; } Type = element as SchemaEntityType; if (Type == null) { AddError(ErrorCode.InvalidRelationshipEndType, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidRelationshipEndType(ParentElement.Name, element.FQName)); } } } internal override void Validate() { base.Validate(); // Check if the end has multiplicity as many, it cannot have any operation behaviour if (Multiplicity == RelationshipMultiplicity.Many && Operations.Count != 0) { AddError(ErrorCode.EndWithManyMultiplicityCannotHaveOperationsSpecified, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.EndWithManyMultiplicityCannotHaveOperationsSpecified(this.Name, ParentElement.FQName)); } } ////// Do simple validation across attributes /// protected override void HandleAttributesComplete() { // set up the default name in before validating anythig that might want to display it in an error message; if (Name == null && _unresolvedType != null) Name = Utils.ExtractTypeName(Schema.DataModel, _unresolvedType); base.HandleAttributesComplete(); } protected override bool ProhibitAttribute(string namespaceUri, string localName) { if (base.ProhibitAttribute(namespaceUri, localName)) { return true; } if (namespaceUri == null && localName == XmlConstants.Name) { return false; } return false; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.Multiplicity)) { HandleMultiplicityAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.Role)) { HandleNameAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.TypeElement)) { HandleTypeAttribute(reader); return true; } return false; } protected override bool HandleElement(XmlReader reader) { if (base.HandleElement(reader)) { return true; } else if (CanHandleElement(reader, XmlConstants.OnDelete)) { HandleOnDeleteElement(reader); return true; } return false; } ////// Handle the Type attribute /// /// reader positioned at Type attribute private void HandleTypeAttribute(XmlReader reader) { Debug.Assert(reader != null); string type; if (!Utils.GetDottedName(this.Schema, reader, out type)) return; _unresolvedType = type; } ////// Handle the Multiplicity attribute /// /// reader positioned at Type attribute private void HandleMultiplicityAttribute(XmlReader reader) { Debug.Assert(reader != null); RelationshipMultiplicity multiplicity; if (!TryParseMultiplicity(reader.Value, out multiplicity)) { AddError(ErrorCode.InvalidMultiplicity, EdmSchemaErrorSeverity.Error, reader, System.Data.Entity.Strings.InvalidRelationshipEndMultiplicity(ParentElement.Name, reader.Value)); } _multiplicity = multiplicity; } ////// Handle an OnDelete element /// /// reader positioned at the element private void HandleOnDeleteElement(XmlReader reader) { HandleOnOperationElement(reader, Operation.Delete); } ////// Handle an On<Operation> element /// /// reader positioned at the element /// the kind of operation being handled private void HandleOnOperationElement(XmlReader reader, Operation operation) { Debug.Assert(reader != null); foreach (OnOperation other in Operations) { if (other.Operation == operation) AddError(ErrorCode.InvalidOperation, EdmSchemaErrorSeverity.Error, reader, System.Data.Entity.Strings.DuplicationOperation(reader.Name)); } OnOperation onOperation = new OnOperation(this, operation); onOperation.Parse(reader); _operations.Add(onOperation); } ////// The parent element as an IRelationship /// internal new IRelationship ParentElement { get { return (IRelationship)(base.ParentElement); } } ////// Create a new Multiplicity object from a string /// /// string containing Multiplicity definition /// new multiplicity object (null if there were errors) ///try if the string was parsable, false otherwise private static bool TryParseMultiplicity(string value, out RelationshipMultiplicity multiplicity) { switch (value) { case "0..1": multiplicity = RelationshipMultiplicity.ZeroOrOne; return true; case "1": multiplicity = RelationshipMultiplicity.One; return true; case "*": multiplicity = RelationshipMultiplicity.Many; return true; default: multiplicity = (RelationshipMultiplicity)(- 1); return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Xml; using System.Data; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// Represents an End element in a relationship /// internal sealed class RelationshipEnd : SchemaElement, IRelationshipEnd { private string _unresolvedType; private RelationshipMultiplicity? _multiplicity; private SchemaEntityType _type; private List_operations; /// /// construct a Relationship End /// /// public RelationshipEnd(Relationship relationship) : base(relationship) { } ////// Type of the End /// public SchemaEntityType Type { get { return _type; } private set { _type = value; } } ////// Multiplicity of the End /// public RelationshipMultiplicity? Multiplicity { get { return _multiplicity; } set { _multiplicity = value; } } ////// The On<Operation>s defined for the End /// public ICollectionOperations { get { if (_operations == null) _operations = new List (); return _operations; } } /// /// do whole element resolution /// internal override void ResolveTopLevelNames() { base.ResolveTopLevelNames(); if (Type == null && _unresolvedType != null) { SchemaType element; if (!Schema.ResolveTypeName(this, _unresolvedType, out element)) { return; } Type = element as SchemaEntityType; if (Type == null) { AddError(ErrorCode.InvalidRelationshipEndType, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidRelationshipEndType(ParentElement.Name, element.FQName)); } } } internal override void Validate() { base.Validate(); // Check if the end has multiplicity as many, it cannot have any operation behaviour if (Multiplicity == RelationshipMultiplicity.Many && Operations.Count != 0) { AddError(ErrorCode.EndWithManyMultiplicityCannotHaveOperationsSpecified, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.EndWithManyMultiplicityCannotHaveOperationsSpecified(this.Name, ParentElement.FQName)); } } ////// Do simple validation across attributes /// protected override void HandleAttributesComplete() { // set up the default name in before validating anythig that might want to display it in an error message; if (Name == null && _unresolvedType != null) Name = Utils.ExtractTypeName(Schema.DataModel, _unresolvedType); base.HandleAttributesComplete(); } protected override bool ProhibitAttribute(string namespaceUri, string localName) { if (base.ProhibitAttribute(namespaceUri, localName)) { return true; } if (namespaceUri == null && localName == XmlConstants.Name) { return false; } return false; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.Multiplicity)) { HandleMultiplicityAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.Role)) { HandleNameAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.TypeElement)) { HandleTypeAttribute(reader); return true; } return false; } protected override bool HandleElement(XmlReader reader) { if (base.HandleElement(reader)) { return true; } else if (CanHandleElement(reader, XmlConstants.OnDelete)) { HandleOnDeleteElement(reader); return true; } return false; } ////// Handle the Type attribute /// /// reader positioned at Type attribute private void HandleTypeAttribute(XmlReader reader) { Debug.Assert(reader != null); string type; if (!Utils.GetDottedName(this.Schema, reader, out type)) return; _unresolvedType = type; } ////// Handle the Multiplicity attribute /// /// reader positioned at Type attribute private void HandleMultiplicityAttribute(XmlReader reader) { Debug.Assert(reader != null); RelationshipMultiplicity multiplicity; if (!TryParseMultiplicity(reader.Value, out multiplicity)) { AddError(ErrorCode.InvalidMultiplicity, EdmSchemaErrorSeverity.Error, reader, System.Data.Entity.Strings.InvalidRelationshipEndMultiplicity(ParentElement.Name, reader.Value)); } _multiplicity = multiplicity; } ////// Handle an OnDelete element /// /// reader positioned at the element private void HandleOnDeleteElement(XmlReader reader) { HandleOnOperationElement(reader, Operation.Delete); } ////// Handle an On<Operation> element /// /// reader positioned at the element /// the kind of operation being handled private void HandleOnOperationElement(XmlReader reader, Operation operation) { Debug.Assert(reader != null); foreach (OnOperation other in Operations) { if (other.Operation == operation) AddError(ErrorCode.InvalidOperation, EdmSchemaErrorSeverity.Error, reader, System.Data.Entity.Strings.DuplicationOperation(reader.Name)); } OnOperation onOperation = new OnOperation(this, operation); onOperation.Parse(reader); _operations.Add(onOperation); } ////// The parent element as an IRelationship /// internal new IRelationship ParentElement { get { return (IRelationship)(base.ParentElement); } } ////// Create a new Multiplicity object from a string /// /// string containing Multiplicity definition /// new multiplicity object (null if there were errors) ///try if the string was parsable, false otherwise private static bool TryParseMultiplicity(string value, out RelationshipMultiplicity multiplicity) { switch (value) { case "0..1": multiplicity = RelationshipMultiplicity.ZeroOrOne; return true; case "1": multiplicity = RelationshipMultiplicity.One; return true; case "*": multiplicity = RelationshipMultiplicity.Many; return true; default: multiplicity = (RelationshipMultiplicity)(- 1); return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
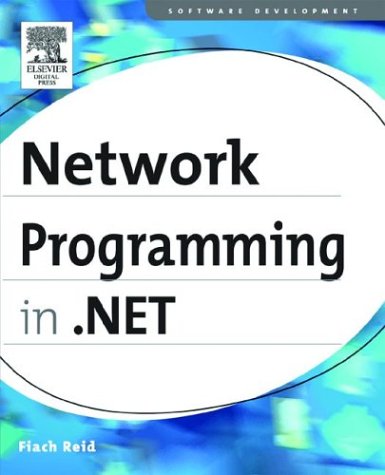
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BookmarkNameHelper.cs
- StrongNamePublicKeyBlob.cs
- FrameworkElement.cs
- PiiTraceSource.cs
- RegexGroup.cs
- PauseStoryboard.cs
- ClientBuildManager.cs
- ToggleButtonAutomationPeer.cs
- IndexerNameAttribute.cs
- CachedCompositeFamily.cs
- ProfileGroupSettings.cs
- SafeHandles.cs
- CodeArrayCreateExpression.cs
- WebColorConverter.cs
- PropertyMetadata.cs
- Message.cs
- BamlLocalizationDictionary.cs
- EncryptedKeyIdentifierClause.cs
- WebPartDisplayModeEventArgs.cs
- MenuItemAutomationPeer.cs
- Debugger.cs
- CodeArgumentReferenceExpression.cs
- EndpointAddressMessageFilterTable.cs
- XamlClipboardData.cs
- WinFormsComponentEditor.cs
- DNS.cs
- TreeNode.cs
- ITextView.cs
- __ComObject.cs
- WebZoneDesigner.cs
- EventManager.cs
- SplineKeyFrames.cs
- ToolStripPanelCell.cs
- DmlSqlGenerator.cs
- DataGridViewLinkColumn.cs
- DataGridViewRowPrePaintEventArgs.cs
- FormViewModeEventArgs.cs
- KeyConverter.cs
- PropertyChangingEventArgs.cs
- LeaseManager.cs
- DataGridItemCollection.cs
- Win32SafeHandles.cs
- ClockGroup.cs
- TimeSpanStorage.cs
- ClrProviderManifest.cs
- BaseCodePageEncoding.cs
- SByte.cs
- ExtendedPropertyInfo.cs
- ContentElement.cs
- ObjectStateEntryDbDataRecord.cs
- ReliableChannelFactory.cs
- StylusPoint.cs
- ObservableCollection.cs
- AudioFormatConverter.cs
- OperationFormatStyle.cs
- BaseCodeDomTreeGenerator.cs
- TabItemWrapperAutomationPeer.cs
- Sql8ConformanceChecker.cs
- MenuStrip.cs
- SamlAssertion.cs
- MulticastOption.cs
- CompilerState.cs
- ObfuscationAttribute.cs
- ExpressionBinding.cs
- InfoCardService.cs
- ColorAnimationUsingKeyFrames.cs
- XMLUtil.cs
- DefaultSettingsSection.cs
- PerformanceCounter.cs
- CompositeControl.cs
- SelectedPathEditor.cs
- WinFormsComponentEditor.cs
- ImageButton.cs
- InvalidComObjectException.cs
- ThemeConfigurationDialog.cs
- Vector3DValueSerializer.cs
- EventEntry.cs
- PrePrepareMethodAttribute.cs
- DataTableReader.cs
- SkewTransform.cs
- DynamicDataResources.Designer.cs
- SelectionItemProviderWrapper.cs
- WsrmTraceRecord.cs
- ExpressionBuilderContext.cs
- ImmutableObjectAttribute.cs
- ToolStripSplitButton.cs
- NodeCounter.cs
- InteropBitmapSource.cs
- DataObjectMethodAttribute.cs
- TableDetailsRow.cs
- DecimalConstantAttribute.cs
- FormsAuthenticationModule.cs
- DesignerGeometryHelper.cs
- CodeConditionStatement.cs
- ObjectParameter.cs
- ShimAsPublicXamlType.cs
- MethodCallConverter.cs
- WebPartUtil.cs
- NewExpression.cs
- EncodingInfo.cs