Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / ITextView.cs / 1 / ITextView.cs
//---------------------------------------------------------------------------- // // File: ITextView.cs // // Description: An interface representing the presentation of an ITextContainer. // // History: // 9/17/2004 : benwest - Created // //--------------------------------------------------------------------------- using System.ComponentModel; // AsyncCompletedEventArgs using System.Collections.ObjectModel; // ReadOnlyCollection using System.Windows.Media; // GlyphRun namespace System.Windows.Documents { ////// The TextView class exposes presentation information for /// a TextContainer. Its methods reveal document structure, including /// line layout, hit testing, and character bounding boxes. /// /// Layouts that support TextView must implement the IServiceProvider /// interface, and support GetService(typeof(TextView)) method calls. /// internal interface ITextView { //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Returns an ITextPointer that matches the supplied Point /// in this TextView. /// /// /// Point in pixel coordinates to test. /// /// /// If true, this method should return the closest position as /// calculated by the control's heuristics. /// If false, this method should return null position, if the test /// point does not fall within any character bounding box. /// ////// A text position and its orientation matching or closest to the point. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// If there is no position that matches the supplied point and /// snapToText is True, this method returns an ITextPointer /// that is closest to the Point. /// However, If snapToText is False, the method returns NULL if the /// supplied point does not fall within the bounding box of /// a character. /// ITextPointer GetTextPositionFromPoint(Point point, bool snapToText); ////// Retrieves the height and offset of the object or character /// represented by the given TextPointer. /// /// /// Position of an object/character. /// ////// The height and offset of the object or character /// represented by the given TextPointer. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// Throws InvalidEnumArgumentException if an invalid enum value for /// direction is passed. /// ////// The Width of the returned rectangle is always 0. /// /// If the content at the specified position is empty, then this /// method will return the expected height of a character placed /// at the specified position. /// Rect GetRectangleFromTextPosition(ITextPointer position); ////// Retrieves the height and offset of the object or character /// represented by the given TextPointer. /// /// /// Position of an object/character. /// /// /// Transform to be applied to returned Rect /// ////// The height and offset of the object or character /// represented by the given TextPointer. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// Throws InvalidEnumArgumentException if an invalid enum value for /// direction is passed. /// ////// The Width of the returned rectangle is always 0. /// If the content at the specified position is empty, then this /// method will return the expected height of a character placed /// at the specified position. /// This rectangle returned is completely untransformed to any ancestors. /// The transform parameter contains the aggregate of transforms that must be /// applied to the rectangle. /// Rect GetRawRectangleFromTextPosition(ITextPointer position, out Transform transform); ////// Returns tight bounding geometry for the given text range. /// /// Start position of the range. /// End position of the range. ///Geometry object containing tight bound. Geometry GetTightBoundingGeometryFromTextPositions(ITextPointer startPosition, ITextPointer endPosition); ////// Returns an ITextPointer matching the given position /// advanced by the given number of lines. /// /// /// Initial text position of an object/character. /// /// /// The suggestedX parameter is the suggested X offset, in pixels, of /// the TextPointer on the destination line; the function returns the /// position whose offset is closest to suggestedX. /// /// /// Number of lines to advance. Negative means move backwards. /// /// /// newSuggestedX is the offset at the position moved (useful when moving /// between columns or pages). /// /// /// linesMoved indicates the number of lines moved, which may be less /// than count if there is no more content. /// ////// ITextPointer matching the given position advanced by the /// given number of lines. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// Throws InvalidEnumArgumentException if an invalid enum value for /// direction is passed. /// ////// The count parameter may be negative, which means move backwards. /// /// If count is larger than the number of available lines in that /// direction, then the returned position will be on the last line /// (or first line if count is negative). /// /// If suggestedX is Double.NaN, then it will be ignored. /// ITextPointer GetPositionAtNextLine(ITextPointer position, double suggestedX, int count, out double newSuggestedX, out int linesMoved); ////// ITextPointer GetPositionAtNextPage(ITextPointer position, Point suggestedOffset, int count, out Point newSuggestedOffset, out int pagesMoved); /// /// Determines if the given position is at the edge of a caret unit /// in the specified direction. /// /// /// Position to test. /// ////// Returns true if the specified position precedes or follows /// the first or last code point of a caret unit, respectively. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// "Caret Unit" is a group of one or more Unicode code points that /// map to a single rendered glyph. /// bool IsAtCaretUnitBoundary(ITextPointer position); ////// Finds the next position at the edge of a caret unit in /// specified direction. /// /// /// Initial text position of an object/character. /// /// /// If Forward, this method returns the "caret unit" position following /// the initial position. /// If Backward, this method returns the caret unit" position preceding /// the initial position. /// ////// The next caret unit break position in specified direction. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// Throws InvalidEnumArgumentException if an invalid enum value for /// direction is passed. /// ////// "Caret Unit" is a group of one or more Unicode code points that /// map to a single rendered glyph. /// /// If the given position is located between two caret units, this /// method returns a new position located at the opposite edge of /// the caret unit in the indicated direction. /// If position is located within a group of Unicode code points that /// map to a single caret unit, this method returns a new position at /// the edge of the caret unit indicated by direction. /// If position is located at the beginning or end of content -- there /// is no content in the indicated direction -- then this method returns /// position at the same location as the given position. /// ITextPointer GetNextCaretUnitPosition(ITextPointer position, LogicalDirection direction); ////// Returns the position at the edge of a caret unit after backspacing. /// /// /// Initial text position of an object/character. /// ////// The the position at the edge of a caret unit after backspacing. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// Throws InvalidEnumArgumentException if an invalid enum value for /// direction is passed. /// ITextPointer GetBackspaceCaretUnitPosition(ITextPointer position); ////// Returns a TextRange that spans the line on which the given /// position is located. /// /// /// Any oriented text position on the line. /// ////// TextRange that spans the line on which the given position is located. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// Throws InvalidEnumArgumentException if an invalid enum value for /// direction is passed. /// TextSegment GetLineRange(ITextPointer position); ////// Provides a collection of glyph properties corresponding to runs /// of Unicode code points. /// /// /// A position preceding the first code point to examine. /// /// /// A position following the last code point to examine. /// ////// A collection of glyph property runs. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// A "glyph" in this context is the lowest level rendered representation /// of text. Each entry in the output array describes a constant run /// of properties on the glyphs corresponding to a range of Unicode /// code points. With this array, it's possible to enumerate the glyph /// properties for each code point in the specified text run. /// ReadOnlyCollectionGetGlyphRuns(ITextPointer start, ITextPointer end); /// /// Returns whether the position is contained in this view. /// /// /// A position to test. /// ////// True if TextView contains specified text position. /// Otherwise returns false. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// Throws InvalidEnumArgumentException if an invalid enum value for /// direction is passed. /// ////// This method is used for multi-view (paginated) scenarios, /// when a position is not guaranteed to be in the current view. /// bool Contains(ITextPointer position); ////// void BringPositionIntoViewAsync(ITextPointer position, object userState); /// /// void BringPointIntoViewAsync(Point point, object userState); /// /// void BringLineIntoViewAsync(ITextPointer position, double suggestedX, int count, object userState); /// /// void BringPageIntoViewAsync(ITextPointer position, Point suggestedOffset, int count, object userState); /// /// Cancels all asynchronous calls made with the given userState. /// If userState is NULL, all asynchronous calls are cancelled. /// /// Unique identifier for the asynchronous task. void CancelAsync(object userState); ////// Ensures the TextView is in a clean layout state and that it is /// possible to retrieve layout data. /// ////// This method may be expensive, because it may lead to a full /// layout update. /// bool Validate(); ////// Ensures this ITextView has a clean layout at the specified Point. /// /// /// Location to validate. /// ////// True if the Point is validated, false otherwise. /// ////// Use this method before calling GetTextPositionFromPoint. /// bool Validate(Point point); ////// Ensures this ITextView has a clean layout at the specified ITextPointer. /// /// /// Position to validate. /// ////// True if the position is validated, false otherwise. /// ////// Use this method before calling any of the methods on this class that /// take a ITextPointer parameter. /// bool Validate(ITextPointer position); ////// Called by the TextEditor after receiving user input. /// Implementors of this method should balance for minimum latency /// for the next few seconds. /// ////// For example, during the next few seconds it would be /// appropriate to examine much smaller chunks of text during background /// layout calculations, so that the latency of a keystroke repsonse is /// minimal. /// void ThrottleBackgroundTasksForUserInput(); #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// A UIElement owning this text view. All coordinates are calculated relative to this element. /// UIElement RenderScope { get; } ////// The container for the text being displayed in this view. /// TextPositions refer to positions within this TextContainer. /// ITextContainer TextContainer { get; } ////// Whether the TextView object is in a valid layout state. /// ////// If False, Validate must be called before calling any other /// method on TextView. /// bool IsValid { get; } ////// Whether the TextView renders its own selection /// bool RendersOwnSelection { get; } ////// Collection of TextSegments representing content of the TextView. /// ReadOnlyCollectionTextSegments { get; } #endregion Internal Properties //------------------------------------------------------ // // Internal Events // //------------------------------------------------------ #region Internal Events /// /// Fired when a BringPositionIntoViewAsync call has completed. /// event BringPositionIntoViewCompletedEventHandler BringPositionIntoViewCompleted; ////// Fired when a BringPointIntoViewAsync call has completed. /// event BringPointIntoViewCompletedEventHandler BringPointIntoViewCompleted; ////// Fired when a BringLineIntoViewAsync call has completed. /// event BringLineIntoViewCompletedEventHandler BringLineIntoViewCompleted; ////// Fired when a BringPageIntoViewAsync call has completed. /// event BringPageIntoViewCompletedEventHandler BringPageIntoViewCompleted; ////// Fired when TextView is updated and becomes valid. /// event EventHandler Updated; #endregion Internal Events } ////// BringPositionIntoViewCompleted event handler. /// internal delegate void BringPositionIntoViewCompletedEventHandler(object sender, BringPositionIntoViewCompletedEventArgs e); ////// BringPointIntoViewCompleted event handler. /// internal delegate void BringPointIntoViewCompletedEventHandler(object sender, BringPointIntoViewCompletedEventArgs e); ////// BringLineIntoViewCompleted event handler. /// internal delegate void BringLineIntoViewCompletedEventHandler(object sender, BringLineIntoViewCompletedEventArgs e); ////// BringLineIntoViewCompleted event handler. /// internal delegate void BringPageIntoViewCompletedEventHandler(object sender, BringPageIntoViewCompletedEventArgs e); ////// Event arguments for the BringPositionIntoViewCompleted event. /// internal class BringPositionIntoViewCompletedEventArgs : AsyncCompletedEventArgs { ////// Constructor. /// /// Position of an object/character. /// Whether operation was successful. /// Error occurred during an asynchronous operation. /// Whether an asynchronous operation has been cancelled. /// Unique identifier for the asynchronous task. public BringPositionIntoViewCompletedEventArgs(ITextPointer position, bool succeeded, Exception error, bool cancelled, object userState) : base(error, cancelled, userState) { //_position = position; //_succeeded = succeeded; } // Position of an object/character. //private readonly ITextPointer _position; // Whether operation was successful. //private readonly bool _succeeded; } ////// Event arguments for the BringPointIntoViewCompleted event. /// internal class BringPointIntoViewCompletedEventArgs : AsyncCompletedEventArgs { ////// Constructor. /// /// Point in pixel coordinates. /// A text position and its orientation matching or closest to the point. /// Whether operation was successful. /// Error occurred during an asynchronous operation. /// Whether an asynchronous operation has been cancelled. /// Unique identifier for the asynchronous task. public BringPointIntoViewCompletedEventArgs(Point point, ITextPointer position, bool succeeded, Exception error, bool cancelled, object userState) : base(error, cancelled, userState) { _point = point; _position = position; //_succeeded = succeeded; } ////// Point in pixel coordinates. /// public Point Point { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _point; } } ////// A text position and its orientation matching or closest to the point. /// public ITextPointer Position { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _position; } } ////// Point in pixel coordinates. /// private readonly Point _point; ////// A text position and its orientation matching or closest to the point. /// private readonly ITextPointer _position; // Whether operation was successful. //private readonly bool _succeeded; } ////// Event arguments for the BringLineIntoViewCompleted event. /// internal class BringLineIntoViewCompletedEventArgs : AsyncCompletedEventArgs { ////// Constructor. /// /// Initial text position of an object/character. /// /// The suggestedX parameter is the suggested X offset, in pixels, of /// the TextPointer on the destination line. /// /// Number of lines to advance. Negative means move backwards. /// ITextPointer matching the given position advanced by the given number of line. /// The offset at the position moved (useful when moving between columns or pages). /// Indicates the number of lines moved, which may be less than count if there is no more content. /// Whether operation was successful. /// Error occurred during an asynchronous operation. /// Whether an asynchronous operation has been cancelled. /// Unique identifier for the asynchronous task. public BringLineIntoViewCompletedEventArgs(ITextPointer position, double suggestedX, int count, ITextPointer newPosition, double newSuggestedX, int linesMoved, bool succeeded, Exception error, bool cancelled, object userState) : base(error, cancelled, userState) { _position = position; //_suggestedX = suggestedX; _count = count; _newPosition = newPosition; _newSuggestedX = newSuggestedX; //_linesMoved = linesMoved; //_succeeded = succeeded; } ////// Initial text position of an object/character. /// public ITextPointer Position { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _position; } } ////// Number of lines to advance. Negative means move backwards. /// public int Count { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _count; } } ////// ITextPointer matching the given position advanced by the given number of line. /// public ITextPointer NewPosition { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _newPosition; } } ////// The offset at the position moved (useful when moving between columns or pages). /// public double NewSuggestedX { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _newSuggestedX; } } ////// Initial text position of an object/character. /// private readonly ITextPointer _position; // The suggestedX parameter is the suggested X offset, in pixels, of // the TextPointer on the destination line. //private readonly double _suggestedX; ////// Number of lines to advance. Negative means move backwards. /// private readonly int _count; ////// ITextPointer matching the given position advanced by the given number of line. /// private readonly ITextPointer _newPosition; ////// The offset at the position moved (useful when moving between columns or pages). /// private readonly double _newSuggestedX; // Indicates the number of lines moved, which may be less than count if there is no more content. //private readonly int _linesMoved; // Whether operation was successful. //private readonly bool _succeeded; } ////// internal class BringPageIntoViewCompletedEventArgs : AsyncCompletedEventArgs { /// /// Constructor. /// /// Initial text position of an object/character. /// /// The suggestedX parameter is the suggested X offset, in pixels, of /// the TextPointer on the destination line. /// /// Number of lines to advance. Negative means move backwards. /// ITextPointer matching the given position advanced by the given number of line. /// The offset at the position moved (useful when moving between columns or pages). /// Indicates the number of pages moved, which may be less than count if there is no more content. /// Whether operation was successful. /// Error occurred during an asynchronous operation. /// Whether an asynchronous operation has been cancelled. /// Unique identifier for the asynchronous task. public BringPageIntoViewCompletedEventArgs(ITextPointer position, Point suggestedOffset, int count, ITextPointer newPosition, Point newSuggestedOffset, int pagesMoved, bool succeeded, Exception error, bool cancelled, object userState) : base(error, cancelled, userState) { _position = position; //_suggestedX = suggestedX; _count = count; _newPosition = newPosition; _newSuggestedOffset = newSuggestedOffset; //_linesMoved = linesMoved; //_succeeded = succeeded; } ////// Initial text position of an object/character. /// public ITextPointer Position { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _position; } } ////// Number of lines to advance. Negative means move backwards. /// public int Count { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _count; } } ////// ITextPointer matching the given position advanced by the given number of line. /// public ITextPointer NewPosition { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _newPosition; } } ////// The offset at the position moved (useful when moving between columns or pages). /// public Point NewSuggestedOffset { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _newSuggestedOffset; } } ////// Initial text position of an object/character. /// private readonly ITextPointer _position; // The suggestedX parameter is the suggested X offset, in pixels, of // the TextPointer on the destination line. //private readonly double _suggestedX; ////// Number of lines to advance. Negative means move backwards. /// private readonly int _count; ////// ITextPointer matching the given position advanced by the given number of line. /// private readonly ITextPointer _newPosition; ////// The offset at the position moved (useful when moving between columns or pages). /// private readonly Point _newSuggestedOffset; // Indicates the number of lines moved, which may be less than count if there is no more content. //private readonly int _linesMoved; // Whether operation was successful. //private readonly bool _succeeded; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: ITextView.cs // // Description: An interface representing the presentation of an ITextContainer. // // History: // 9/17/2004 : benwest - Created // //--------------------------------------------------------------------------- using System.ComponentModel; // AsyncCompletedEventArgs using System.Collections.ObjectModel; // ReadOnlyCollection using System.Windows.Media; // GlyphRun namespace System.Windows.Documents { ////// The TextView class exposes presentation information for /// a TextContainer. Its methods reveal document structure, including /// line layout, hit testing, and character bounding boxes. /// /// Layouts that support TextView must implement the IServiceProvider /// interface, and support GetService(typeof(TextView)) method calls. /// internal interface ITextView { //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Returns an ITextPointer that matches the supplied Point /// in this TextView. /// /// /// Point in pixel coordinates to test. /// /// /// If true, this method should return the closest position as /// calculated by the control's heuristics. /// If false, this method should return null position, if the test /// point does not fall within any character bounding box. /// ////// A text position and its orientation matching or closest to the point. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// If there is no position that matches the supplied point and /// snapToText is True, this method returns an ITextPointer /// that is closest to the Point. /// However, If snapToText is False, the method returns NULL if the /// supplied point does not fall within the bounding box of /// a character. /// ITextPointer GetTextPositionFromPoint(Point point, bool snapToText); ////// Retrieves the height and offset of the object or character /// represented by the given TextPointer. /// /// /// Position of an object/character. /// ////// The height and offset of the object or character /// represented by the given TextPointer. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// Throws InvalidEnumArgumentException if an invalid enum value for /// direction is passed. /// ////// The Width of the returned rectangle is always 0. /// /// If the content at the specified position is empty, then this /// method will return the expected height of a character placed /// at the specified position. /// Rect GetRectangleFromTextPosition(ITextPointer position); ////// Retrieves the height and offset of the object or character /// represented by the given TextPointer. /// /// /// Position of an object/character. /// /// /// Transform to be applied to returned Rect /// ////// The height and offset of the object or character /// represented by the given TextPointer. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// Throws InvalidEnumArgumentException if an invalid enum value for /// direction is passed. /// ////// The Width of the returned rectangle is always 0. /// If the content at the specified position is empty, then this /// method will return the expected height of a character placed /// at the specified position. /// This rectangle returned is completely untransformed to any ancestors. /// The transform parameter contains the aggregate of transforms that must be /// applied to the rectangle. /// Rect GetRawRectangleFromTextPosition(ITextPointer position, out Transform transform); ////// Returns tight bounding geometry for the given text range. /// /// Start position of the range. /// End position of the range. ///Geometry object containing tight bound. Geometry GetTightBoundingGeometryFromTextPositions(ITextPointer startPosition, ITextPointer endPosition); ////// Returns an ITextPointer matching the given position /// advanced by the given number of lines. /// /// /// Initial text position of an object/character. /// /// /// The suggestedX parameter is the suggested X offset, in pixels, of /// the TextPointer on the destination line; the function returns the /// position whose offset is closest to suggestedX. /// /// /// Number of lines to advance. Negative means move backwards. /// /// /// newSuggestedX is the offset at the position moved (useful when moving /// between columns or pages). /// /// /// linesMoved indicates the number of lines moved, which may be less /// than count if there is no more content. /// ////// ITextPointer matching the given position advanced by the /// given number of lines. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// Throws InvalidEnumArgumentException if an invalid enum value for /// direction is passed. /// ////// The count parameter may be negative, which means move backwards. /// /// If count is larger than the number of available lines in that /// direction, then the returned position will be on the last line /// (or first line if count is negative). /// /// If suggestedX is Double.NaN, then it will be ignored. /// ITextPointer GetPositionAtNextLine(ITextPointer position, double suggestedX, int count, out double newSuggestedX, out int linesMoved); ////// ITextPointer GetPositionAtNextPage(ITextPointer position, Point suggestedOffset, int count, out Point newSuggestedOffset, out int pagesMoved); /// /// Determines if the given position is at the edge of a caret unit /// in the specified direction. /// /// /// Position to test. /// ////// Returns true if the specified position precedes or follows /// the first or last code point of a caret unit, respectively. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// "Caret Unit" is a group of one or more Unicode code points that /// map to a single rendered glyph. /// bool IsAtCaretUnitBoundary(ITextPointer position); ////// Finds the next position at the edge of a caret unit in /// specified direction. /// /// /// Initial text position of an object/character. /// /// /// If Forward, this method returns the "caret unit" position following /// the initial position. /// If Backward, this method returns the caret unit" position preceding /// the initial position. /// ////// The next caret unit break position in specified direction. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// Throws InvalidEnumArgumentException if an invalid enum value for /// direction is passed. /// ////// "Caret Unit" is a group of one or more Unicode code points that /// map to a single rendered glyph. /// /// If the given position is located between two caret units, this /// method returns a new position located at the opposite edge of /// the caret unit in the indicated direction. /// If position is located within a group of Unicode code points that /// map to a single caret unit, this method returns a new position at /// the edge of the caret unit indicated by direction. /// If position is located at the beginning or end of content -- there /// is no content in the indicated direction -- then this method returns /// position at the same location as the given position. /// ITextPointer GetNextCaretUnitPosition(ITextPointer position, LogicalDirection direction); ////// Returns the position at the edge of a caret unit after backspacing. /// /// /// Initial text position of an object/character. /// ////// The the position at the edge of a caret unit after backspacing. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// Throws InvalidEnumArgumentException if an invalid enum value for /// direction is passed. /// ITextPointer GetBackspaceCaretUnitPosition(ITextPointer position); ////// Returns a TextRange that spans the line on which the given /// position is located. /// /// /// Any oriented text position on the line. /// ////// TextRange that spans the line on which the given position is located. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// Throws InvalidEnumArgumentException if an invalid enum value for /// direction is passed. /// TextSegment GetLineRange(ITextPointer position); ////// Provides a collection of glyph properties corresponding to runs /// of Unicode code points. /// /// /// A position preceding the first code point to examine. /// /// /// A position following the last code point to examine. /// ////// A collection of glyph property runs. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// A "glyph" in this context is the lowest level rendered representation /// of text. Each entry in the output array describes a constant run /// of properties on the glyphs corresponding to a range of Unicode /// code points. With this array, it's possible to enumerate the glyph /// properties for each code point in the specified text run. /// ReadOnlyCollectionGetGlyphRuns(ITextPointer start, ITextPointer end); /// /// Returns whether the position is contained in this view. /// /// /// A position to test. /// ////// True if TextView contains specified text position. /// Otherwise returns false. /// ////// Throws InvalidOperationException if IsValid is false. /// ////// Throws ArgumentOutOfRangeException if incoming position is not /// part of this TextView (should call Contains first to check). /// ////// Throws ArgumentNullException if position is invalid. /// ////// Throws InvalidEnumArgumentException if an invalid enum value for /// direction is passed. /// ////// This method is used for multi-view (paginated) scenarios, /// when a position is not guaranteed to be in the current view. /// bool Contains(ITextPointer position); ////// void BringPositionIntoViewAsync(ITextPointer position, object userState); /// /// void BringPointIntoViewAsync(Point point, object userState); /// /// void BringLineIntoViewAsync(ITextPointer position, double suggestedX, int count, object userState); /// /// void BringPageIntoViewAsync(ITextPointer position, Point suggestedOffset, int count, object userState); /// /// Cancels all asynchronous calls made with the given userState. /// If userState is NULL, all asynchronous calls are cancelled. /// /// Unique identifier for the asynchronous task. void CancelAsync(object userState); ////// Ensures the TextView is in a clean layout state and that it is /// possible to retrieve layout data. /// ////// This method may be expensive, because it may lead to a full /// layout update. /// bool Validate(); ////// Ensures this ITextView has a clean layout at the specified Point. /// /// /// Location to validate. /// ////// True if the Point is validated, false otherwise. /// ////// Use this method before calling GetTextPositionFromPoint. /// bool Validate(Point point); ////// Ensures this ITextView has a clean layout at the specified ITextPointer. /// /// /// Position to validate. /// ////// True if the position is validated, false otherwise. /// ////// Use this method before calling any of the methods on this class that /// take a ITextPointer parameter. /// bool Validate(ITextPointer position); ////// Called by the TextEditor after receiving user input. /// Implementors of this method should balance for minimum latency /// for the next few seconds. /// ////// For example, during the next few seconds it would be /// appropriate to examine much smaller chunks of text during background /// layout calculations, so that the latency of a keystroke repsonse is /// minimal. /// void ThrottleBackgroundTasksForUserInput(); #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// A UIElement owning this text view. All coordinates are calculated relative to this element. /// UIElement RenderScope { get; } ////// The container for the text being displayed in this view. /// TextPositions refer to positions within this TextContainer. /// ITextContainer TextContainer { get; } ////// Whether the TextView object is in a valid layout state. /// ////// If False, Validate must be called before calling any other /// method on TextView. /// bool IsValid { get; } ////// Whether the TextView renders its own selection /// bool RendersOwnSelection { get; } ////// Collection of TextSegments representing content of the TextView. /// ReadOnlyCollectionTextSegments { get; } #endregion Internal Properties //------------------------------------------------------ // // Internal Events // //------------------------------------------------------ #region Internal Events /// /// Fired when a BringPositionIntoViewAsync call has completed. /// event BringPositionIntoViewCompletedEventHandler BringPositionIntoViewCompleted; ////// Fired when a BringPointIntoViewAsync call has completed. /// event BringPointIntoViewCompletedEventHandler BringPointIntoViewCompleted; ////// Fired when a BringLineIntoViewAsync call has completed. /// event BringLineIntoViewCompletedEventHandler BringLineIntoViewCompleted; ////// Fired when a BringPageIntoViewAsync call has completed. /// event BringPageIntoViewCompletedEventHandler BringPageIntoViewCompleted; ////// Fired when TextView is updated and becomes valid. /// event EventHandler Updated; #endregion Internal Events } ////// BringPositionIntoViewCompleted event handler. /// internal delegate void BringPositionIntoViewCompletedEventHandler(object sender, BringPositionIntoViewCompletedEventArgs e); ////// BringPointIntoViewCompleted event handler. /// internal delegate void BringPointIntoViewCompletedEventHandler(object sender, BringPointIntoViewCompletedEventArgs e); ////// BringLineIntoViewCompleted event handler. /// internal delegate void BringLineIntoViewCompletedEventHandler(object sender, BringLineIntoViewCompletedEventArgs e); ////// BringLineIntoViewCompleted event handler. /// internal delegate void BringPageIntoViewCompletedEventHandler(object sender, BringPageIntoViewCompletedEventArgs e); ////// Event arguments for the BringPositionIntoViewCompleted event. /// internal class BringPositionIntoViewCompletedEventArgs : AsyncCompletedEventArgs { ////// Constructor. /// /// Position of an object/character. /// Whether operation was successful. /// Error occurred during an asynchronous operation. /// Whether an asynchronous operation has been cancelled. /// Unique identifier for the asynchronous task. public BringPositionIntoViewCompletedEventArgs(ITextPointer position, bool succeeded, Exception error, bool cancelled, object userState) : base(error, cancelled, userState) { //_position = position; //_succeeded = succeeded; } // Position of an object/character. //private readonly ITextPointer _position; // Whether operation was successful. //private readonly bool _succeeded; } ////// Event arguments for the BringPointIntoViewCompleted event. /// internal class BringPointIntoViewCompletedEventArgs : AsyncCompletedEventArgs { ////// Constructor. /// /// Point in pixel coordinates. /// A text position and its orientation matching or closest to the point. /// Whether operation was successful. /// Error occurred during an asynchronous operation. /// Whether an asynchronous operation has been cancelled. /// Unique identifier for the asynchronous task. public BringPointIntoViewCompletedEventArgs(Point point, ITextPointer position, bool succeeded, Exception error, bool cancelled, object userState) : base(error, cancelled, userState) { _point = point; _position = position; //_succeeded = succeeded; } ////// Point in pixel coordinates. /// public Point Point { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _point; } } ////// A text position and its orientation matching or closest to the point. /// public ITextPointer Position { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _position; } } ////// Point in pixel coordinates. /// private readonly Point _point; ////// A text position and its orientation matching or closest to the point. /// private readonly ITextPointer _position; // Whether operation was successful. //private readonly bool _succeeded; } ////// Event arguments for the BringLineIntoViewCompleted event. /// internal class BringLineIntoViewCompletedEventArgs : AsyncCompletedEventArgs { ////// Constructor. /// /// Initial text position of an object/character. /// /// The suggestedX parameter is the suggested X offset, in pixels, of /// the TextPointer on the destination line. /// /// Number of lines to advance. Negative means move backwards. /// ITextPointer matching the given position advanced by the given number of line. /// The offset at the position moved (useful when moving between columns or pages). /// Indicates the number of lines moved, which may be less than count if there is no more content. /// Whether operation was successful. /// Error occurred during an asynchronous operation. /// Whether an asynchronous operation has been cancelled. /// Unique identifier for the asynchronous task. public BringLineIntoViewCompletedEventArgs(ITextPointer position, double suggestedX, int count, ITextPointer newPosition, double newSuggestedX, int linesMoved, bool succeeded, Exception error, bool cancelled, object userState) : base(error, cancelled, userState) { _position = position; //_suggestedX = suggestedX; _count = count; _newPosition = newPosition; _newSuggestedX = newSuggestedX; //_linesMoved = linesMoved; //_succeeded = succeeded; } ////// Initial text position of an object/character. /// public ITextPointer Position { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _position; } } ////// Number of lines to advance. Negative means move backwards. /// public int Count { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _count; } } ////// ITextPointer matching the given position advanced by the given number of line. /// public ITextPointer NewPosition { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _newPosition; } } ////// The offset at the position moved (useful when moving between columns or pages). /// public double NewSuggestedX { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _newSuggestedX; } } ////// Initial text position of an object/character. /// private readonly ITextPointer _position; // The suggestedX parameter is the suggested X offset, in pixels, of // the TextPointer on the destination line. //private readonly double _suggestedX; ////// Number of lines to advance. Negative means move backwards. /// private readonly int _count; ////// ITextPointer matching the given position advanced by the given number of line. /// private readonly ITextPointer _newPosition; ////// The offset at the position moved (useful when moving between columns or pages). /// private readonly double _newSuggestedX; // Indicates the number of lines moved, which may be less than count if there is no more content. //private readonly int _linesMoved; // Whether operation was successful. //private readonly bool _succeeded; } ////// internal class BringPageIntoViewCompletedEventArgs : AsyncCompletedEventArgs { /// /// Constructor. /// /// Initial text position of an object/character. /// /// The suggestedX parameter is the suggested X offset, in pixels, of /// the TextPointer on the destination line. /// /// Number of lines to advance. Negative means move backwards. /// ITextPointer matching the given position advanced by the given number of line. /// The offset at the position moved (useful when moving between columns or pages). /// Indicates the number of pages moved, which may be less than count if there is no more content. /// Whether operation was successful. /// Error occurred during an asynchronous operation. /// Whether an asynchronous operation has been cancelled. /// Unique identifier for the asynchronous task. public BringPageIntoViewCompletedEventArgs(ITextPointer position, Point suggestedOffset, int count, ITextPointer newPosition, Point newSuggestedOffset, int pagesMoved, bool succeeded, Exception error, bool cancelled, object userState) : base(error, cancelled, userState) { _position = position; //_suggestedX = suggestedX; _count = count; _newPosition = newPosition; _newSuggestedOffset = newSuggestedOffset; //_linesMoved = linesMoved; //_succeeded = succeeded; } ////// Initial text position of an object/character. /// public ITextPointer Position { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _position; } } ////// Number of lines to advance. Negative means move backwards. /// public int Count { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _count; } } ////// ITextPointer matching the given position advanced by the given number of line. /// public ITextPointer NewPosition { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _newPosition; } } ////// The offset at the position moved (useful when moving between columns or pages). /// public Point NewSuggestedOffset { get { // Raise an exception if the operation failed or was cancelled. this.RaiseExceptionIfNecessary(); return _newSuggestedOffset; } } ////// Initial text position of an object/character. /// private readonly ITextPointer _position; // The suggestedX parameter is the suggested X offset, in pixels, of // the TextPointer on the destination line. //private readonly double _suggestedX; ////// Number of lines to advance. Negative means move backwards. /// private readonly int _count; ////// ITextPointer matching the given position advanced by the given number of line. /// private readonly ITextPointer _newPosition; ////// The offset at the position moved (useful when moving between columns or pages). /// private readonly Point _newSuggestedOffset; // Indicates the number of lines moved, which may be less than count if there is no more content. //private readonly int _linesMoved; // Whether operation was successful. //private readonly bool _succeeded; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
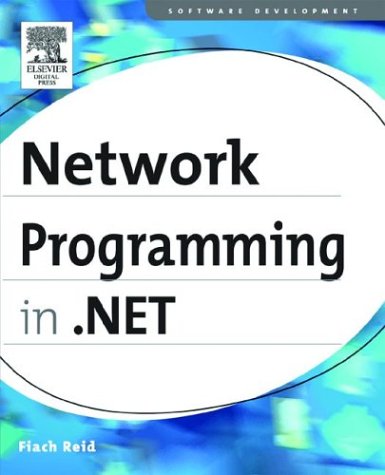
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompensatableTransactionScopeActivityDesigner.cs
- SimpleParser.cs
- ObjectStorage.cs
- CommentAction.cs
- _NestedSingleAsyncResult.cs
- AnchoredBlock.cs
- UshortList2.cs
- RuntimeWrappedException.cs
- BoundPropertyEntry.cs
- DescendantOverDescendantQuery.cs
- XmlHierarchicalDataSourceView.cs
- ImplicitInputBrush.cs
- XmlSchemaComplexContentExtension.cs
- WebPartEditVerb.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- RewritingValidator.cs
- SimpleLine.cs
- UncommonField.cs
- DomainLiteralReader.cs
- followingsibling.cs
- SiteMapDataSourceView.cs
- BitmapEffectGroup.cs
- _PooledStream.cs
- Main.cs
- SoapObjectInfo.cs
- EllipseGeometry.cs
- CodeNamespaceCollection.cs
- EntityContainerEntitySetDefiningQuery.cs
- ReadOnlyCollectionBase.cs
- ScalarType.cs
- Int16Animation.cs
- UnsafeNativeMethodsTablet.cs
- SettingsSavedEventArgs.cs
- TextEditorDragDrop.cs
- StringUtil.cs
- x509store.cs
- WebPartConnectionsCancelVerb.cs
- WpfWebRequestHelper.cs
- TypeDescriptor.cs
- UnsafeNativeMethods.cs
- TrailingSpaceComparer.cs
- SoapExtensionTypeElement.cs
- PolyLineSegmentFigureLogic.cs
- ConsumerConnectionPointCollection.cs
- WebServiceErrorEvent.cs
- DataGridViewRowPrePaintEventArgs.cs
- CrossSiteScriptingValidation.cs
- KeyInterop.cs
- BookmarkEventArgs.cs
- RealizationContext.cs
- VisualBasic.cs
- UnmanagedMemoryStream.cs
- SimpleWebHandlerParser.cs
- ChannelManager.cs
- activationcontext.cs
- HMACSHA512.cs
- Mappings.cs
- LoadedOrUnloadedOperation.cs
- WeakEventManager.cs
- ListViewDataItem.cs
- Enlistment.cs
- isolationinterop.cs
- FileVersion.cs
- HtmlInputButton.cs
- EditorBrowsableAttribute.cs
- PropertyManager.cs
- LayoutEngine.cs
- PartitionerStatic.cs
- CustomError.cs
- PropertyOverridesDialog.cs
- ElementProxy.cs
- ListViewCancelEventArgs.cs
- PropVariant.cs
- MailMessageEventArgs.cs
- SoapIncludeAttribute.cs
- control.ime.cs
- SessionStateItemCollection.cs
- TypeUtil.cs
- DrawingContextDrawingContextWalker.cs
- ClientOptions.cs
- WindowsStatic.cs
- SolidColorBrush.cs
- ipaddressinformationcollection.cs
- AtlasWeb.Designer.cs
- _ProxyChain.cs
- OdbcConnectionStringbuilder.cs
- PageAdapter.cs
- SecurityDocument.cs
- EditorPartChrome.cs
- Brushes.cs
- EntityExpressionVisitor.cs
- EntityDesignerBuildProvider.cs
- XmlQuerySequence.cs
- StringOutput.cs
- AccessDataSourceView.cs
- XmlMemberMapping.cs
- DesignerActionMethodItem.cs
- ConstructorExpr.cs
- Exceptions.cs
- RowUpdatingEventArgs.cs