Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / HtmlControls / HtmlInputButton.cs / 1 / HtmlInputButton.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlInputButton.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Web; using System.Web.UI; using System.Globalization; using System.Security.Permissions; ////// [ DefaultEvent("ServerClick"), SupportsEventValidation, ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class HtmlInputButton : HtmlInputControl, IPostBackEventHandler { private static readonly object EventServerClick = new object(); /* * Creates an intrinsic Html INPUT type=button control. */ ////// The ///class defines the methods, /// properties, and events for the HTML Input Button control. This class allows /// programmatic access to the HTML <input type= /// button>, <input type= /// submit>,and <input /// type= /// reset> elements on /// the server. /// /// public HtmlInputButton() : base("button") { } /* * Creates an intrinsic Html INPUT type=button,submit,reset control. */ ///Initializes a new instance of a ///class using /// default values. /// public HtmlInputButton(string type) : base(type) { } ///Initializes a new instance of a ///class using the /// specified string. /// [ WebCategory("Behavior"), DefaultValue(true), ] public virtual bool CausesValidation { get { object b = ViewState["CausesValidation"]; return((b == null) ? true : (bool)b); } set { ViewState["CausesValidation"] = value; } } [ WebCategory("Behavior"), DefaultValue(""), WebSysDescription(SR.PostBackControl_ValidationGroup) ] public virtual string ValidationGroup { get { string s = (string)ViewState["ValidationGroup"]; return((s == null) ? String.Empty : s); } set { ViewState["ValidationGroup"] = value; } } ///Gets or sets whether pressing the button causes page validation to fire. This defaults to True so that when /// using validation controls, the validation state of all controls are updated when the button is clicked, both /// on the client and the server. Setting this to False is useful when defining a cancel or reset button on a page /// that has validators. ////// [ WebCategory("Action"), WebSysDescription(SR.HtmlControl_OnServerClick) ] public event EventHandler ServerClick { add { Events.AddHandler(EventServerClick, value); } remove { Events.RemoveHandler(EventServerClick, value); } } ////// Occurs when an HTML Input Button control is clicked on the browser. /// ///protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); if (Page != null && Events[EventServerClick] != null) { Page.RegisterPostBackScript(); } } /* * Override to generate postback code for onclick. */ /// /// /// protected override void RenderAttributes(HtmlTextWriter writer) { RenderAttributesInternal(writer); base.RenderAttributes(writer); // this must come last because of the self-closing / } // VSWhidbey 80882: It needs to be overridden by HtmlInputSubmit internal virtual void RenderAttributesInternal(HtmlTextWriter writer) { bool submitsProgramatically = Events[EventServerClick] != null; if (Page != null) { if (submitsProgramatically) { Util.WriteOnClickAttribute( writer, this, false /* submitsAutomatically */, submitsProgramatically, (CausesValidation && Page.GetValidators(ValidationGroup).Count > 0), ValidationGroup); } else { Page.ClientScript.RegisterForEventValidation(UniqueID); } } } ////// protected virtual void OnServerClick(EventArgs e) { EventHandler handler = (EventHandler)Events[EventServerClick]; if (handler != null) handler(this, e); } /* * Method of IPostBackEventHandler interface to raise events on post back. * Button fires an OnServerClick event. */ ///Raises the ///event. /// /// void IPostBackEventHandler.RaisePostBackEvent(string eventArgument) { RaisePostBackEvent(eventArgument); } ////// /// protected virtual void RaisePostBackEvent(string eventArgument) { ValidateEvent(UniqueID, eventArgument); if (CausesValidation) { Page.Validate(ValidationGroup); } OnServerClick(EventArgs.Empty); } } }
Link Menu
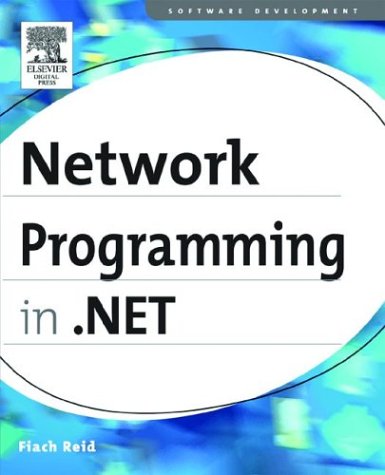
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyFilterAttribute.cs
- CounterSample.cs
- ListCommandEventArgs.cs
- SQLBinary.cs
- DataGridViewIntLinkedList.cs
- login.cs
- FilterException.cs
- CookieHandler.cs
- MemberDescriptor.cs
- HttpHandlerAction.cs
- RouteItem.cs
- InvalidEnumArgumentException.cs
- AutomationProperty.cs
- SqlDataRecord.cs
- ConnectorDragDropGlyph.cs
- GridViewColumnCollection.cs
- FlowPanelDesigner.cs
- LogSwitch.cs
- Message.cs
- HtmlControl.cs
- MetadataUtilsSmi.cs
- configsystem.cs
- XmlBinaryReader.cs
- WebPartEditVerb.cs
- SetUserLanguageRequest.cs
- DataGridTableCollection.cs
- QuinticEase.cs
- shaper.cs
- DBSchemaTable.cs
- NullReferenceException.cs
- EUCJPEncoding.cs
- ScriptControl.cs
- HttpCachePolicy.cs
- ListBoxItem.cs
- DisplayNameAttribute.cs
- DesignerRegionCollection.cs
- SqlEnums.cs
- DataGridViewRowPostPaintEventArgs.cs
- AssemblyResourceLoader.cs
- PagePropertiesChangingEventArgs.cs
- RichTextBoxConstants.cs
- TextTreeTextBlock.cs
- FontFamilyIdentifier.cs
- entityreference_tresulttype.cs
- EditorZone.cs
- XPathDescendantIterator.cs
- ProjectionCamera.cs
- SspiSafeHandles.cs
- WindowsFormsSynchronizationContext.cs
- IdentitySection.cs
- CodeTypeParameter.cs
- DecimalAnimationUsingKeyFrames.cs
- WinEventHandler.cs
- ConnectionConsumerAttribute.cs
- ButtonChrome.cs
- DmlSqlGenerator.cs
- SharedStatics.cs
- SourceItem.cs
- ClientConfigPaths.cs
- CommonDialog.cs
- CodeTypeDeclaration.cs
- ViewStateException.cs
- MimeParameterWriter.cs
- GroupQuery.cs
- SerialPort.cs
- RelationshipConverter.cs
- XmlPropertyBag.cs
- BitSet.cs
- InfoCardBaseException.cs
- ControlCollection.cs
- RecordManager.cs
- _DomainName.cs
- WorkflowPrinting.cs
- WebBrowserHelper.cs
- WindowsScroll.cs
- UIntPtr.cs
- Decimal.cs
- ButtonPopupAdapter.cs
- ItemChangedEventArgs.cs
- DynamicPropertyHolder.cs
- GraphicsPathIterator.cs
- RawStylusInputCustomDataList.cs
- DesignerDataRelationship.cs
- ProcessStartInfo.cs
- EventLog.cs
- ArcSegment.cs
- HtmlInputFile.cs
- SourceFileInfo.cs
- TouchEventArgs.cs
- X509Certificate.cs
- EventLogPermission.cs
- RequestCacheEntry.cs
- SingleAnimationBase.cs
- ToolStripContainerActionList.cs
- ConnectionInterfaceCollection.cs
- OutputCacheModule.cs
- DeclarationUpdate.cs
- BindingWorker.cs
- ConstraintEnumerator.cs
- ShaderRenderModeValidation.cs