Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / QuaternionKeyFrameCollection.cs / 1305600 / QuaternionKeyFrameCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameQuaternionAnimation /// to animate a Quaternion property value along a set of key frames. /// public class QuaternionKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static QuaternionKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new QuaternionKeyFrameCollection. /// public QuaternionKeyFrameCollection() : base() { _keyFrames = new List< QuaternionKeyFrame>(2); } #endregion #region Static Methods ////// An empty QuaternionKeyFrameCollection. /// public static QuaternionKeyFrameCollection Empty { get { if (s_emptyCollection == null) { QuaternionKeyFrameCollection emptyCollection = new QuaternionKeyFrameCollection(); emptyCollection._keyFrames = new List< QuaternionKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this QuaternionKeyFrameCollection. /// ///The copy public new QuaternionKeyFrameCollection Clone() { return (QuaternionKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new QuaternionKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { QuaternionKeyFrameCollection sourceCollection = (QuaternionKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< QuaternionKeyFrame>(count); for (int i = 0; i < count; i++) { QuaternionKeyFrame keyFrame = (QuaternionKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { QuaternionKeyFrameCollection sourceCollection = (QuaternionKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< QuaternionKeyFrame>(count); for (int i = 0; i < count; i++) { QuaternionKeyFrame keyFrame = (QuaternionKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { QuaternionKeyFrameCollection sourceCollection = (QuaternionKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< QuaternionKeyFrame>(count); for (int i = 0; i < count; i++) { QuaternionKeyFrame keyFrame = (QuaternionKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { QuaternionKeyFrameCollection sourceCollection = (QuaternionKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< QuaternionKeyFrame>(count); for (int i = 0; i < count; i++) { QuaternionKeyFrame keyFrame = (QuaternionKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the QuaternionKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of QuaternionKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the QuaternionKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the QuaternionKeyFrames in the collection to an /// array of QuaternionKeyFrames. /// public void CopyTo(QuaternionKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a QuaternionKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((QuaternionKeyFrame)keyFrame); } ////// Adds a QuaternionKeyFrame to the collection. /// public int Add(QuaternionKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all QuaternionKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given QuaternionKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((QuaternionKeyFrame)keyFrame); } ////// Returns true of the collection contains the given QuaternionKeyFrame. /// public bool Contains(QuaternionKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given QuaternionKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((QuaternionKeyFrame)keyFrame); } ////// Returns the index of a given QuaternionKeyFrame in the collection. /// public int IndexOf(QuaternionKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a QuaternionKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (QuaternionKeyFrame)keyFrame); } ////// Inserts a QuaternionKeyFrame into a specific location in the collection. /// public void Insert(int index, QuaternionKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a QuaternionKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((QuaternionKeyFrame)keyFrame); } ////// Removes a QuaternionKeyFrame from the collection. /// public void Remove(QuaternionKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the QuaternionKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the QuaternionKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (QuaternionKeyFrame)value; } } ////// Gets or sets the QuaternionKeyFrame at a given index. /// public QuaternionKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "QuaternionKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameQuaternionAnimation /// to animate a Quaternion property value along a set of key frames. /// public class QuaternionKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static QuaternionKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new QuaternionKeyFrameCollection. /// public QuaternionKeyFrameCollection() : base() { _keyFrames = new List< QuaternionKeyFrame>(2); } #endregion #region Static Methods ////// An empty QuaternionKeyFrameCollection. /// public static QuaternionKeyFrameCollection Empty { get { if (s_emptyCollection == null) { QuaternionKeyFrameCollection emptyCollection = new QuaternionKeyFrameCollection(); emptyCollection._keyFrames = new List< QuaternionKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this QuaternionKeyFrameCollection. /// ///The copy public new QuaternionKeyFrameCollection Clone() { return (QuaternionKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new QuaternionKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { QuaternionKeyFrameCollection sourceCollection = (QuaternionKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< QuaternionKeyFrame>(count); for (int i = 0; i < count; i++) { QuaternionKeyFrame keyFrame = (QuaternionKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { QuaternionKeyFrameCollection sourceCollection = (QuaternionKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< QuaternionKeyFrame>(count); for (int i = 0; i < count; i++) { QuaternionKeyFrame keyFrame = (QuaternionKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { QuaternionKeyFrameCollection sourceCollection = (QuaternionKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< QuaternionKeyFrame>(count); for (int i = 0; i < count; i++) { QuaternionKeyFrame keyFrame = (QuaternionKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { QuaternionKeyFrameCollection sourceCollection = (QuaternionKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< QuaternionKeyFrame>(count); for (int i = 0; i < count; i++) { QuaternionKeyFrame keyFrame = (QuaternionKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the QuaternionKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of QuaternionKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the QuaternionKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the QuaternionKeyFrames in the collection to an /// array of QuaternionKeyFrames. /// public void CopyTo(QuaternionKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a QuaternionKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((QuaternionKeyFrame)keyFrame); } ////// Adds a QuaternionKeyFrame to the collection. /// public int Add(QuaternionKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all QuaternionKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given QuaternionKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((QuaternionKeyFrame)keyFrame); } ////// Returns true of the collection contains the given QuaternionKeyFrame. /// public bool Contains(QuaternionKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given QuaternionKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((QuaternionKeyFrame)keyFrame); } ////// Returns the index of a given QuaternionKeyFrame in the collection. /// public int IndexOf(QuaternionKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a QuaternionKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (QuaternionKeyFrame)keyFrame); } ////// Inserts a QuaternionKeyFrame into a specific location in the collection. /// public void Insert(int index, QuaternionKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a QuaternionKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((QuaternionKeyFrame)keyFrame); } ////// Removes a QuaternionKeyFrame from the collection. /// public void Remove(QuaternionKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the QuaternionKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the QuaternionKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (QuaternionKeyFrame)value; } } ////// Gets or sets the QuaternionKeyFrame at a given index. /// public QuaternionKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "QuaternionKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
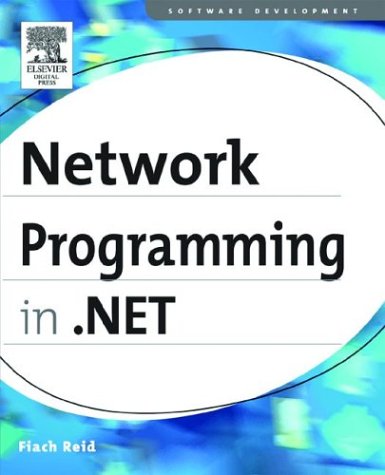
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Set.cs
- VoiceObjectToken.cs
- IntegerFacetDescriptionElement.cs
- XmlMessageFormatter.cs
- QilScopedVisitor.cs
- ResumeStoryboard.cs
- ReferenceConverter.cs
- DataObjectEventArgs.cs
- WorkflowCommandExtensionItem.cs
- smtpconnection.cs
- odbcmetadatafactory.cs
- SchemaTableColumn.cs
- Geometry.cs
- GridViewCellAutomationPeer.cs
- Timer.cs
- XmlCharCheckingWriter.cs
- Pkcs7Signer.cs
- BinaryMethodMessage.cs
- CacheEntry.cs
- PropertyAccessVisitor.cs
- MD5CryptoServiceProvider.cs
- StateBag.cs
- PathSegmentCollection.cs
- WaitHandle.cs
- hebrewshape.cs
- CustomValidator.cs
- DomainLiteralReader.cs
- FormViewInsertEventArgs.cs
- DiffuseMaterial.cs
- DecoderBestFitFallback.cs
- BamlBinaryReader.cs
- RecognitionEventArgs.cs
- TemplateBindingExpressionConverter.cs
- UserPreferenceChangingEventArgs.cs
- NameSpaceExtractor.cs
- UrlMappingsModule.cs
- HandlerBase.cs
- CrossContextChannel.cs
- HostingEnvironmentWrapper.cs
- SymDocumentType.cs
- Transform.cs
- ColumnCollection.cs
- DeferredReference.cs
- SeekStoryboard.cs
- EdmError.cs
- FixedTextContainer.cs
- Int32RectValueSerializer.cs
- BitmapEffectInputData.cs
- CharStorage.cs
- TypedDatasetGenerator.cs
- HMACSHA512.cs
- IdentitySection.cs
- Bits.cs
- ClientUIRequest.cs
- RemoveStoryboard.cs
- NodeFunctions.cs
- ProcessThread.cs
- InternalCache.cs
- XmlnsPrefixAttribute.cs
- ChangeInterceptorAttribute.cs
- UIPermission.cs
- WinFormsSecurity.cs
- MouseEventArgs.cs
- RewritingPass.cs
- HttpServerVarsCollection.cs
- OrderPreservingPipeliningSpoolingTask.cs
- SingleResultAttribute.cs
- HtmlEmptyTagControlBuilder.cs
- Lasso.cs
- MD5.cs
- AccessDataSource.cs
- FlowDocumentFormatter.cs
- TracingConnectionListener.cs
- MailAddress.cs
- SpellerStatusTable.cs
- SizeValueSerializer.cs
- MimeTypeMapper.cs
- ObjectKeyFrameCollection.cs
- ListViewGroup.cs
- QueryOutputWriter.cs
- Schema.cs
- FormViewInsertEventArgs.cs
- NativeMethodsCLR.cs
- UInt32.cs
- GeometryModel3D.cs
- BinaryFormatterWriter.cs
- Table.cs
- nulltextnavigator.cs
- UnrecognizedPolicyAssertionElement.cs
- DataError.cs
- XmlCustomFormatter.cs
- ActivityLocationReferenceEnvironment.cs
- MachinePropertyVariants.cs
- Timer.cs
- TraceContext.cs
- MethodInfo.cs
- CategoryNameCollection.cs
- NumericUpDownAccelerationCollection.cs
- RemotingServices.cs
- DataGridViewCheckBoxColumn.cs