Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Messaging / System / Messaging / XmlMessageFormatter.cs / 1305376 / XmlMessageFormatter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Messaging { using System; using System.IO; using System.Xml; using System.Collections; using System.Xml.Serialization; using System.ComponentModel; using System.Security.Permissions; ////// /// Formatter class that serializes and deserializes objects into /// and from MessageQueue messages using Xml. /// public class XmlMessageFormatter : IMessageFormatter { private Type[] targetTypes; private string[] targetTypeNames; Hashtable targetSerializerTable = new Hashtable(); private bool typeNamesAdded; private bool typesAdded; ////// /// Creates a new Xml message formatter object. /// public XmlMessageFormatter() { this.TargetTypes = new Type[0]; this.TargetTypeNames = new string[0]; } ////// /// Creates a new Xml message formatter object, /// using the given properties. /// public XmlMessageFormatter(string[] targetTypeNames) { this.TargetTypeNames = targetTypeNames; this.TargetTypes = new Type[0]; } ////// /// Creates a new Xml message formatter object, /// using the given properties. /// public XmlMessageFormatter(Type[] targetTypes) { this.TargetTypes = targetTypes; this.TargetTypeNames = new string[0]; } ////// /// Specifies the set of possible types that will /// be deserialized by the formatter from the /// message provided. /// [MessagingDescription(Res.XmlMsgTargetTypeNames)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1819:PropertiesShouldNotReturnArrays")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly")] public string[] TargetTypeNames { get { return this.targetTypeNames; } set { if (value == null) throw new ArgumentNullException("value"); this.typeNamesAdded = false; this.targetTypeNames = value; } } ////// /// Specifies the set of possible types that will /// be deserialized by the formatter from the /// message provided. /// [Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), MessagingDescription(Res.XmlMsgTargetTypes)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1819:PropertiesShouldNotReturnArrays")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly")] public Type[] TargetTypes { get { return this.targetTypes; } set { if (value == null) throw new ArgumentNullException("value"); this.typesAdded = false; this.targetTypes = value; } } ////// /// When this method is called, the formatter will attempt to determine /// if the contents of the message are something the formatter can deal with. /// public bool CanRead(Message message) { if (message == null) throw new ArgumentNullException("message"); this.CreateTargetSerializerTable(); Stream stream = message.BodyStream; XmlTextReader reader = new XmlTextReader(stream); reader.WhitespaceHandling = WhitespaceHandling.Significant; reader.DtdProcessing = DtdProcessing.Prohibit; bool result = false; foreach (XmlSerializer serializer in targetSerializerTable.Values) { if (serializer.CanDeserialize(reader)) { result = true; break; } } message.BodyStream.Position = 0; // reset stream in case CanRead is followed by Deserialize return result; } ////// /// This method is needed to improve scalability on Receive and ReceiveAsync scenarios. Not requiring /// thread safety on read and write. /// public object Clone() { XmlMessageFormatter formatter = new XmlMessageFormatter(); formatter.targetTypes = targetTypes; formatter.targetTypeNames = targetTypeNames; formatter.typesAdded = typesAdded; formatter.typeNamesAdded = typeNamesAdded; foreach (Type targetType in targetSerializerTable.Keys) formatter.targetSerializerTable[targetType] = new XmlSerializer(targetType); return formatter; } ///private void CreateTargetSerializerTable() { if (!this.typeNamesAdded) { for (int index = 0; index < this.targetTypeNames.Length; ++ index) { Type targetType = Type.GetType(this.targetTypeNames[index], true); if (targetType != null) this.targetSerializerTable[targetType] = new XmlSerializer(targetType); } this.typeNamesAdded = true; } if (!this.typesAdded) { for (int index = 0; index < this.targetTypes.Length; ++ index) this.targetSerializerTable[this.targetTypes[index]] = new XmlSerializer(this.targetTypes[index]); this.typesAdded = true; } if (this.targetSerializerTable.Count == 0) throw new InvalidOperationException(Res.GetString(Res.TypeListMissing)); } /// /// /// This method is used to read the contents from the given message /// and create an object. /// public object Read(Message message) { if (message == null) throw new ArgumentNullException("message"); this.CreateTargetSerializerTable(); Stream stream = message.BodyStream; XmlTextReader reader = new XmlTextReader(stream); reader.WhitespaceHandling = WhitespaceHandling.Significant; reader.DtdProcessing = DtdProcessing.Prohibit; foreach (XmlSerializer serializer in targetSerializerTable.Values) { if (serializer.CanDeserialize(reader)) return serializer.Deserialize(reader); } throw new InvalidOperationException(Res.GetString(Res.InvalidTypeDeserialization)); } ////// /// This method is used to write the given object into the given message. /// If the formatter cannot understand the given object, an exception is thrown. /// public void Write(Message message, object obj) { if (message == null) throw new ArgumentNullException("message"); if (obj == null) throw new ArgumentNullException("obj"); Stream stream = new MemoryStream(); Type serializedType = obj.GetType(); XmlSerializer serializer = null; if (this.targetSerializerTable.ContainsKey(serializedType)) serializer = (XmlSerializer)this.targetSerializerTable[serializedType]; else { serializer = new XmlSerializer(serializedType); this.targetSerializerTable[serializedType] = serializer; } serializer.Serialize(stream, obj); message.BodyStream = stream; //Need to reset the body type, in case the same message //is reused by some other formatter. message.BodyType = 0; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Messaging { using System; using System.IO; using System.Xml; using System.Collections; using System.Xml.Serialization; using System.ComponentModel; using System.Security.Permissions; ////// /// Formatter class that serializes and deserializes objects into /// and from MessageQueue messages using Xml. /// public class XmlMessageFormatter : IMessageFormatter { private Type[] targetTypes; private string[] targetTypeNames; Hashtable targetSerializerTable = new Hashtable(); private bool typeNamesAdded; private bool typesAdded; ////// /// Creates a new Xml message formatter object. /// public XmlMessageFormatter() { this.TargetTypes = new Type[0]; this.TargetTypeNames = new string[0]; } ////// /// Creates a new Xml message formatter object, /// using the given properties. /// public XmlMessageFormatter(string[] targetTypeNames) { this.TargetTypeNames = targetTypeNames; this.TargetTypes = new Type[0]; } ////// /// Creates a new Xml message formatter object, /// using the given properties. /// public XmlMessageFormatter(Type[] targetTypes) { this.TargetTypes = targetTypes; this.TargetTypeNames = new string[0]; } ////// /// Specifies the set of possible types that will /// be deserialized by the formatter from the /// message provided. /// [MessagingDescription(Res.XmlMsgTargetTypeNames)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1819:PropertiesShouldNotReturnArrays")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly")] public string[] TargetTypeNames { get { return this.targetTypeNames; } set { if (value == null) throw new ArgumentNullException("value"); this.typeNamesAdded = false; this.targetTypeNames = value; } } ////// /// Specifies the set of possible types that will /// be deserialized by the formatter from the /// message provided. /// [Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), MessagingDescription(Res.XmlMsgTargetTypes)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1819:PropertiesShouldNotReturnArrays")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly")] public Type[] TargetTypes { get { return this.targetTypes; } set { if (value == null) throw new ArgumentNullException("value"); this.typesAdded = false; this.targetTypes = value; } } ////// /// When this method is called, the formatter will attempt to determine /// if the contents of the message are something the formatter can deal with. /// public bool CanRead(Message message) { if (message == null) throw new ArgumentNullException("message"); this.CreateTargetSerializerTable(); Stream stream = message.BodyStream; XmlTextReader reader = new XmlTextReader(stream); reader.WhitespaceHandling = WhitespaceHandling.Significant; reader.DtdProcessing = DtdProcessing.Prohibit; bool result = false; foreach (XmlSerializer serializer in targetSerializerTable.Values) { if (serializer.CanDeserialize(reader)) { result = true; break; } } message.BodyStream.Position = 0; // reset stream in case CanRead is followed by Deserialize return result; } ////// /// This method is needed to improve scalability on Receive and ReceiveAsync scenarios. Not requiring /// thread safety on read and write. /// public object Clone() { XmlMessageFormatter formatter = new XmlMessageFormatter(); formatter.targetTypes = targetTypes; formatter.targetTypeNames = targetTypeNames; formatter.typesAdded = typesAdded; formatter.typeNamesAdded = typeNamesAdded; foreach (Type targetType in targetSerializerTable.Keys) formatter.targetSerializerTable[targetType] = new XmlSerializer(targetType); return formatter; } ///private void CreateTargetSerializerTable() { if (!this.typeNamesAdded) { for (int index = 0; index < this.targetTypeNames.Length; ++ index) { Type targetType = Type.GetType(this.targetTypeNames[index], true); if (targetType != null) this.targetSerializerTable[targetType] = new XmlSerializer(targetType); } this.typeNamesAdded = true; } if (!this.typesAdded) { for (int index = 0; index < this.targetTypes.Length; ++ index) this.targetSerializerTable[this.targetTypes[index]] = new XmlSerializer(this.targetTypes[index]); this.typesAdded = true; } if (this.targetSerializerTable.Count == 0) throw new InvalidOperationException(Res.GetString(Res.TypeListMissing)); } /// /// /// This method is used to read the contents from the given message /// and create an object. /// public object Read(Message message) { if (message == null) throw new ArgumentNullException("message"); this.CreateTargetSerializerTable(); Stream stream = message.BodyStream; XmlTextReader reader = new XmlTextReader(stream); reader.WhitespaceHandling = WhitespaceHandling.Significant; reader.DtdProcessing = DtdProcessing.Prohibit; foreach (XmlSerializer serializer in targetSerializerTable.Values) { if (serializer.CanDeserialize(reader)) return serializer.Deserialize(reader); } throw new InvalidOperationException(Res.GetString(Res.InvalidTypeDeserialization)); } ////// /// This method is used to write the given object into the given message. /// If the formatter cannot understand the given object, an exception is thrown. /// public void Write(Message message, object obj) { if (message == null) throw new ArgumentNullException("message"); if (obj == null) throw new ArgumentNullException("obj"); Stream stream = new MemoryStream(); Type serializedType = obj.GetType(); XmlSerializer serializer = null; if (this.targetSerializerTable.ContainsKey(serializedType)) serializer = (XmlSerializer)this.targetSerializerTable[serializedType]; else { serializer = new XmlSerializer(serializedType); this.targetSerializerTable[serializedType] = serializer; } serializer.Serialize(stream, obj); message.BodyStream = stream; //Need to reset the body type, in case the same message //is reused by some other formatter. message.BodyType = 0; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
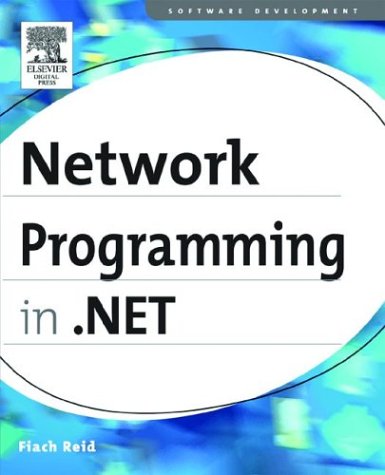
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectAnimationBase.cs
- SystemGatewayIPAddressInformation.cs
- StoreItemCollection.Loader.cs
- CodePrimitiveExpression.cs
- DecoderExceptionFallback.cs
- IUnknownConstantAttribute.cs
- XmlSchemaNotation.cs
- UserControl.cs
- RenderData.cs
- Hex.cs
- WmpBitmapEncoder.cs
- Matrix3DValueSerializer.cs
- DivideByZeroException.cs
- FamilyCollection.cs
- DocumentPageHost.cs
- AsyncOperation.cs
- wmiprovider.cs
- TreeViewImageKeyConverter.cs
- Tile.cs
- EditorPart.cs
- CqlLexerHelpers.cs
- DefaultSection.cs
- NaturalLanguageHyphenator.cs
- DbProviderServices.cs
- xamlnodes.cs
- SqlMethodAttribute.cs
- ParallelLoopState.cs
- CodeDomDecompiler.cs
- SharedHttpsTransportManager.cs
- PortCache.cs
- FlatButtonAppearance.cs
- DiagnosticStrings.cs
- UnregisterInfo.cs
- DataIdProcessor.cs
- ToolStripPanelSelectionBehavior.cs
- AdornerHitTestResult.cs
- DataTableMappingCollection.cs
- SignedInfo.cs
- Subtract.cs
- RectKeyFrameCollection.cs
- OutputCacheModule.cs
- SocketAddress.cs
- ComponentResourceKey.cs
- FieldNameLookup.cs
- SendAgentStatusRequest.cs
- ExtentCqlBlock.cs
- ParallelDesigner.cs
- HttpCapabilitiesEvaluator.cs
- GeneralTransform3D.cs
- Primitive.cs
- ServiceOperationParameter.cs
- CollectionViewGroup.cs
- MachineKeyConverter.cs
- Visual3DCollection.cs
- AttributeQuery.cs
- ConfigurationConverterBase.cs
- GenericNameHandler.cs
- WebPartUtil.cs
- GeometryGroup.cs
- WorkflowView.cs
- Permission.cs
- XamlToRtfWriter.cs
- CopyAction.cs
- StylusPointPropertyInfoDefaults.cs
- ServerIdentity.cs
- SafeEventLogReadHandle.cs
- TemplateInstanceAttribute.cs
- DomainConstraint.cs
- FreezableOperations.cs
- RequestCacheValidator.cs
- ProfileInfo.cs
- ServiceHttpModule.cs
- RuleDefinitions.cs
- ItemsPresenter.cs
- ClientConfigurationSystem.cs
- TemplateControlParser.cs
- Encoding.cs
- BuildManagerHost.cs
- TraceHelpers.cs
- SessionIDManager.cs
- Scene3D.cs
- UserControlCodeDomTreeGenerator.cs
- DataControlField.cs
- UpdatePanelControlTrigger.cs
- CounterNameConverter.cs
- Delegate.cs
- DbSource.cs
- _ListenerRequestStream.cs
- ArgIterator.cs
- UnauthorizedWebPart.cs
- SQLDouble.cs
- TrustManagerPromptUI.cs
- LoggedException.cs
- TreeNodeConverter.cs
- ObjectViewFactory.cs
- TypeDelegator.cs
- DeviceContexts.cs
- _ChunkParse.cs
- OpenTypeLayoutCache.cs
- Assert.cs